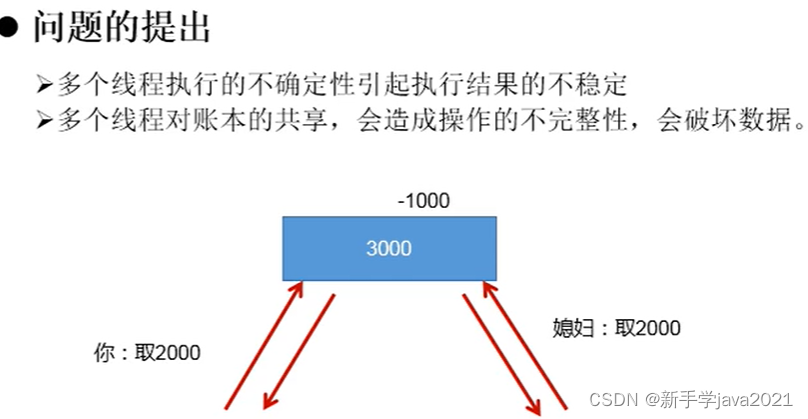
package com.www.java02;
class MyThread1 implements Runnable{
private int sum = 100;
@Override
public void run() {
while(true){
if(sum > 0){
System.out.println(Thread.currentThread().getName() + "卖票:" + sum);
sum--;
}else break;
}
}
}
public class WindowTest1 {
public static void main(String[] args) {
MyThread1 mt = new MyThread1();
Thread t1 = new Thread(mt);
t1.setName("线程一");
Thread t2 = new Thread(mt);
t2.setName("线程二");
Thread t3 = new Thread(mt);
t3.setName("线程三");
t1.start();
t2.start();
t3.start();
}
}
package com.www.java02;
public class WindowTest {
public static void main(String[] args) {
MyThread mt = new MyThread();
mt.setName("线程1");
mt.start();
MyThread mt1 = new MyThread();
mt1.setName("线程2");
mt1.start();
MyThread mt2 = new MyThread();
mt2.setName("线程3");
mt2.start();
}
}
class MyThread extends Thread{
private static int sum = 100;
@Override
public void run() {
while(true){
if(sum > 0){
System.out.println(getName() + "卖票:" + sum);
sum--;
}else break;
}
}
}
线程不安全 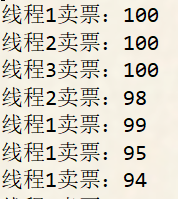 解决线程安全问题方法一:同步代码块
package com.www.java;
class MyThread1 implements Runnable{
private int sum = 100;
Object obj = new Object();
@Override
public void run() {
while(true){
synchronized (this){
if(sum > 0){
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "卖票:" + sum);
sum--;
}else break;
}
}
}
}
public class WindowTest1 {
public static void main(String[] args) {
MyThread1 mt = new MyThread1();
Thread t1 = new Thread(mt);
t1.setName("线程一");
Thread t2 = new Thread(mt);
t2.setName("线程二");
Thread t3 = new Thread(mt);
t3.setName("线程三");
t1.start();
t2.start();
t3.start();
}
}
package com.www.java;
public class WindowTest2 {
public static void main(String[] args) {
MyThread mt = new MyThread();
mt.setName("线程1");
mt.start();
MyThread mt1 = new MyThread();
mt1.setName("线程2");
mt1.start();
MyThread mt2 = new MyThread();
mt2.setName("线程3");
mt2.start();
}
}
class MyThread extends Thread{
private static int sum = 100;
static Object obj = new Object();
@Override
public void run() {
while(true){
synchronized (obj){
if(sum > 0){
try {
sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(getName() + "卖票:" + sum);
sum--;
}else break;
}
}
}
}
方法二:同步方法
package com.www.java;
class MyThread2 implements Runnable{
private int sum = 100;
@Override
public void run() {
while(true){
run1();
if(sum < 1)break;
}
}
private synchronized void run1(){
if(sum > 0) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "卖票:" + sum);
sum--;
}
}
}
public class WindowTest3 {
public static void main(String[] args) {
com.www.java.MyThread2 mt = new com.www.java.MyThread2();
Thread t1 = new Thread(mt);
t1.setName("线程一");
Thread t2 = new Thread(mt);
t2.setName("线程二");
Thread t3 = new Thread(mt);
t3.setName("线程三");
t1.start();
t2.start();
t3.start();
}
}
package com.www.java;
public class WindowTest4 {
public static void main(String[] args) {
MyThread3 mt = new MyThread3();
mt.setName("线程1");
mt.start();
MyThread3 mt1 = new MyThread3();
mt1.setName("线程2");
mt1.start();
MyThread3 mt2 = new MyThread3();
mt2.setName("线程3");
mt2.start();
}
}
class MyThread3 extends Thread{
private static int sum = 100;
@Override
public void run() {
while(true){
run1();
if(sum < 1)break;
}
}
private static synchronized void run1(){
if(sum > 0){
try {
sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "卖票:" + sum);
sum--;
}
}
}
|