Spring Boot简介与案例
https://spring.io/
概述
Spring的缺点:配置过于繁琐
Spring Boot开发团队:Pivotal
Spring Boot简介
简化初始搭建以及开发过程
不再需要定义样板化的配置
快速应用开发领域
Spring、Spring MVC和Spring Boot
Spring最初利用IOC和AOP解耦
按照这种模式搞了MVC框架
写很多样板代码很麻烦,就有了Spring Boot
Spring Cloud是在Spring Boot基础上诞生的
Spring Boot核心特点
开箱即用
约定优先于配置
版本介绍
不需要选择最新的版本,而是要选择最适合,稳定的,因为新版本可能很多其他配套的组件还没有应用
CURRENT GA最新的GA版本
GA 发布版本 面向大众的稳定版本,不会被更改
SNAPSHOT 快照版本 经常修改
Spring Boot2.0
Java 8+
tomcat 8+
Thymeleaf 3
HIBERNATE 5.2
默认软件的优化,比如检查到Spring Security安全组件存在会自动进行默认配置
支持HTTP2
新建Spring Boot项目演示(官网和IDEA两种方式)
官网方式
https://start.spring.io/ 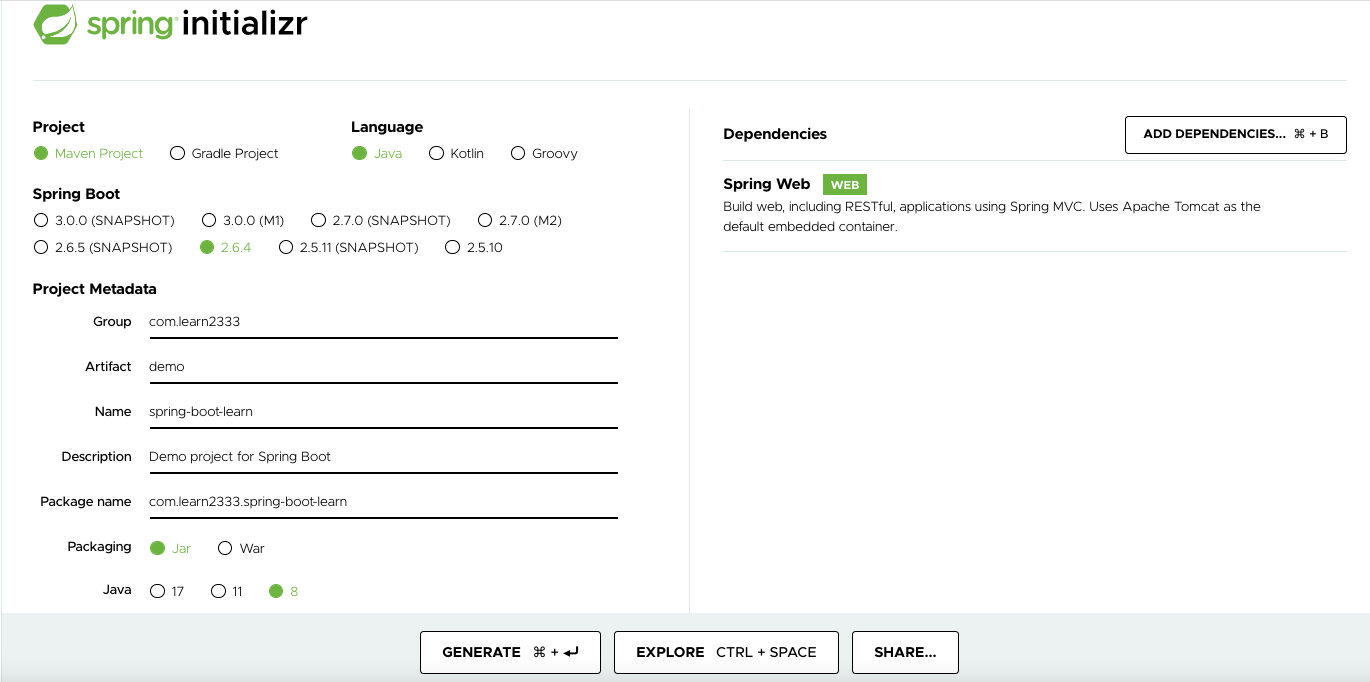
Spring Boot版本显示不全,之后可以在项目中改
点击GENERATE会下载一个zip包,解压后用IDEA打开,等待导入加载即可
IDEA方式
新建项目选择Spring Initializr进行配置即可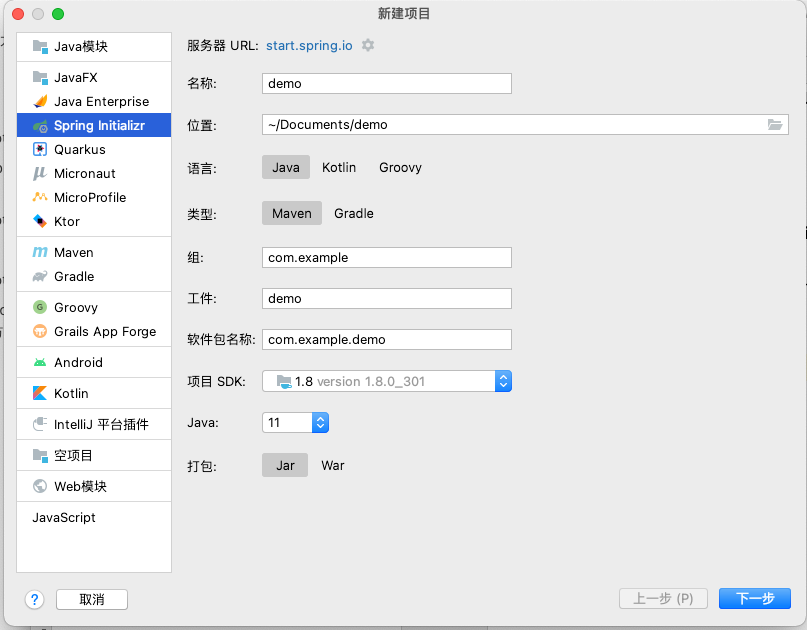
案例
Java 1.8
MySQL 8.0
Maven 3
Springn Boot 2.2.1版本需要严格一致
项目结构解析
Spring Boot的基础结构共三个文件:入口、配置文件、测试入口
生成的Application和ApplicationTests类都可以直接运行来启动当前创建的项目
pom.xml Spring Boot的版本改为和项目的一致:2.2.1RELEASE然后重新加载maven
点击入口类启动即可成功启动Spring Boot,开箱即用
Get请求传参方式
ParaController
package com.learn2333.springbootlearn;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/prefix")
public class ParaController {
@GetMapping({"/firstpequest"})
public String firstRequest() {
return "第一个Spring Boot接口";
}
@GetMapping({"/requestpara"})
public String requestPara(@RequestParam Integer num) {
return "para from request:" + num;
}
@GetMapping({"/para/{num}"})
public String pathPara(@PathVariable Integer num) {
return "para from path:" + num;
}
@GetMapping({"/multiurl1", "multiurl2"})
public String multiurl(@PathVariable Integer num) {
return "para from path:" + num;
}
@GetMapping({"/required"})
public String required(@RequestParam(required = false, defaultValue = "0") Integer num) {
return "para from request:" + num;
}
}
Web项目三层结构
Controller 对外暴露接口
Service 在复杂业务场景下对业务逻辑做一层抽象封装,保持Controller层的简洁和独立
DAO层 数据相关处理
配置文件简介
properties
端口、项目统一前缀…
server.port=8081
server.servlet.context-path=/first
上面是等号写法,还有yml写法
https://toyaml.com/index.html 在线转换
yml:分层级,冒号后需要空格
server:
port: 8081
servlet:
context-path: /first
配置自定义属性
application.properties
school.grade=3
school.classnum=6
方法一:使用Value注入
PropertiesController
package com.learn2333.springbootlearn;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class PropertiesController {
@Value("${school.grade}")
Integer grade;
@Value("${school.classnum}")
Integer classnum;
@GetMapping("gradeclass")
public String gradeClass() {
return "年级" + grade + "班级" + classnum;
}
}
方法二:使用Autowired对象注入
在类中配置绑定(需要get/set方法)
SchoolConfig
package com.learn2333.springbootlearn;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.web.bind.annotation.RestController;
@RestController
@ConfigurationProperties(prefix = "school")
public class SchoolConfig {
Integer grade;
Integer classnum;
public Integer getGrade() {
return grade;
}
public void setGrade(Integer grade) {
this.grade = grade;
}
public Integer getClassnum() {
return classnum;
}
public void setClassnum(Integer classnum) {
this.classnum = classnum;
}
}
ConfigController
package com.learn2333.springbootlearn;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ConfigController {
@Autowired
SchoolConfig schoolConfig;
@GetMapping({"/gradefromconfig"})
public String gradeClass() {
return "年级" + schoolConfig.grade + "班级" + schoolConfig.classnum;
}
}
Service和DAO的编写–学生信息查询案例
数据库名springbootlearn内有表student包含学号和姓名2个字段
pom引入mybatis和连接池依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
application.properties配置
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.username=root
spring.datasource.password=root1024
spring.datasource.url=jdbc:mysql://localhost:3306/springbootlearn?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=true
Student实体类
package com.learn2333.springbootlearn;
public class Student {
Integer id;
String name;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
StudentController控制层
package com.learn2333.springbootlearn;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class StudentController {
@Autowired
StudentService studentService;
@GetMapping("/student")
public String student(@RequestParam Integer num) {
Student student = studentService.findStudent(num);
return student.toString();
}
}
StudentService服务层
package com.learn2333.springbootlearn;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class StudentService {
@Autowired
StudentMapper studentMapper;
public Student findStudent(Integer id) {
return studentMapper.findById(id);
}
}
StudentMapper接口
package com.learn2333.springbootlearn;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import org.springframework.stereotype.Repository;
@Mapper
@Repository
public interface StudentMapper {
@Select("select * from students where id = #{id}")
Student findById(Integer id);
}
|