Spring配置
使用spring创建对象,spring中称为Bean 类型 变量名 = new 类型(); Hello hello = new Hello();
在spring中 id = 变量名 class = new的对象 property 相当于给属性赋值
<bean id="hello" class="pojo.Hello">
<property name="str" value="Spring"/>
</bean>
1.别名

2.Bean的配置
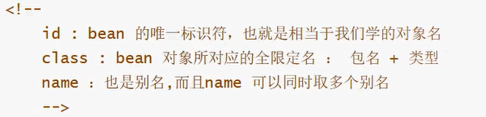
<bean id="hello" class="pojo.Hello" name="hello1 hello2,h3;h4">
<property name="str" value="Spring"/>
</bean>
3.Import
这个import,一般用于团队开发使用,他可以将多个配置文件,导入合并为一个 假设,现在项目中有多个人开发,这三个人复制不同的类开发,不同的类需要注册在不同的bean中,我们可以利用import将所有人的beans.xml合并为一个总的!
如:在总的配置文件applicationConfig.xml中
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<import resource="spring-dao.xml"/>
<import resource="spring-service.xml"/>
<beans>
DI(依赖注入)
1.构造器注入
见 Spring学习总结【二】—IoC(控制反转) 中的 IoC创建对象的方式
2.set方式注入【重点】
。依赖: bean对象的创建依赖于容器! 。注入:bean对象中的所有属性,由容器来注入!
步骤如下:
- 1.在 Bean 中提供一个默认的无参构造函数(在没有其他带参构造函数的情况下,可省略),并为所有需要注入的属性提供一个 setXxx() 方法;
- 2.在 Spring 的 XML 配置文件中,使用 及其子元素 对 Bean 进行定义;
- 3.在
<bean> 元素内使用 <property> 元素对各个属性进行赋值。
public class Student {
private String name;
private Address address;
private String[] book;
private List<String> hobby;
private Map<String, String> card;
private Set<String> games;
private String wife;
private Properties info;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public String[] getBook() {
return book;
}
public void setBook(String[] book) {
this.book = book;
}
public List<String> getHobby() {
return hobby;
}
public void setHobby(List<String> hobby) {
this.hobby = hobby;
}
public Map<String, String> getCard() {
return card;
}
public void setCard(Map<String, String> card) {
this.card = card;
}
public Set<String> getGames() {
return games;
}
public void setGames(Set<String> games) {
this.games = games;
}
public String getWife() {
return wife;
}
public void setWife(String wife) {
this.wife = wife;
}
public Properties getInfo() {
return info;
}
public void setInfo(Properties info) {
this.info = info;
}
@Override
public String toString() {
return "Student{" + "\n" +
"name='" + name + "\n" +
"address=" + address.toString()+ "\n" +
"book=" + Arrays.toString(book) + "\n" +
"hobby=" + hobby + "\n" +
"card=" + card + "\n" +
"games=" + games + "\n" +
"wife='" + wife + "\n" +
"info=" + info + "\n" +
'}';
}
}
<bean id="student" class="pojo.Student">
<property name="name" value="张三"/>
<property name="address" ref="address"/>
<property name="book">
<array>
<value>红楼梦</value>
<value>西游记</value>
<value>水浒传</value>
</array>
</property>
<property name="hobby">
<list>
<value>篮球</value>
<value>足球</value>
</list>
</property>
<property name="card">
<map>
<entry key="111" value="aaa"/>
<entry key="222" value="ddd"/>
</map>
</property>
<property name="games">
<set>
<value>cf</value>
<value>lol</value>
</set>
</property>
<property name="wife">
<null/>
</property>
<property name="info">
<props>
<prop key="违纪记录">无违纪记录</prop>
<prop key="性别">男</prop>
</props>
</property>
</bean>
3.拓展方式注入(短命名空间注入)

<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:c="http://www.springframework.org/schema/c"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="user" class="pojo.User" p:name="aa" p:age="12"/>
<bean id="user2" class="pojo.User" c:age="123" c:name="dddd"/>
</beans>
|