一、跨多行文件
Spring Batch之读数据—Flat格式文件(二十四)_人……杰的博客-CSDN博客_flat文件
二、项目实例
1.项目框架
?
2.代码实现
(1)BatchMain.java
package com.xj.demo22;
import org.springframework.batch.core.Job;
import org.springframework.batch.core.JobExecution;
import org.springframework.batch.core.JobParameters;
import org.springframework.batch.core.launch.JobLauncher;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* @Author : xjfu
* @Date : 2021/10/26 20:01
* @Description : demo22 读记录跨多行文件
*/
public class BatchMain {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("demo22/job/demo22-job.xml");
//Spring Batch的作业启动器,
JobLauncher launcher = (JobLauncher) context.getBean("jobLauncher");
//在batch.xml中配置的一个作业
Job job = (Job)context.getBean("billJob");
try{
//开始执行这个作业,获得处理结果(要运行的job,job参数对象)
JobExecution result = launcher.run(job, new JobParameters());
System.out.println(result.toString());
}catch (Exception e){
e.printStackTrace();
}
}
}
(2)CreditBill.java
package com.xj.demo22;
/**
* @Author : xjfu
* @Date : 2021/10/26 19:27
* @Description :
*/
public class CreditBill {
//银行卡账户ID
private String accountID = "";
//持卡人姓名
private String name = "";
//消费金额
private double amount = 0;
//消费日期
private String date = "";
//消费场所
private String address = "";
public String getAccountID() {
return accountID;
}
public void setAccountID(String accountID) {
this.accountID = accountID;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getAmount() {
return amount;
}
public void setAmount(double amount) {
this.amount = amount;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return this.accountID + "," + this.name + "," + this.amount + "," + this.date + "," + this.address;
}
}
(3)CreditBillFieldSetMapper.java
package com.xj.demo22;
import org.springframework.batch.item.file.mapping.FieldSetMapper;
import org.springframework.batch.item.file.transform.FieldSet;
import org.springframework.validation.BindException;
public class CreditBillFieldSetMapper implements FieldSetMapper<CreditBill> {
public CreditBill mapFieldSet(FieldSet fieldSet) throws BindException {
CreditBill result = new CreditBill();
result.setAccountID(fieldSet.readString("accountID"));
result.setName(fieldSet.readString("name"));
result.setAmount(fieldSet.readDouble("amount"));
result.setDate(fieldSet.readString("date"));
result.setAddress(fieldSet.readString("address"));
return result;
}
}
(4)CreditBillProcessor.java
package com.xj.demo22;
import org.springframework.batch.item.ItemProcessor;
public class CreditBillProcessor implements ItemProcessor<CreditBill, CreditBill> {
public CreditBill process(CreditBill bill) throws Exception {
System.out.println(bill.toString());
return bill;
}
}
(5)MultiLineRecordSeparatorPolicy.java
package com.xj.demo22;
import org.springframework.batch.item.file.separator.RecordSeparatorPolicy;
public class MultiLineRecordSeparatorPolicy implements RecordSeparatorPolicy {
//定义读取的分隔符号
private String delimiter = ",";
//分割符总数,给定的字符串包含的分隔符个数等于此值,则认为是一条完整记录
private int count = 0;
//定义一条记录的完整规则
public boolean isEndOfRecord(String line) {
return countDelimiter(line) == count;
}
public String postProcess(String record) {
return record;
}
public String preProcess(String record) {
return record;
}
//统计给定内容包含分隔符的个数
private int countDelimiter(String s) {
String tmp = s;
int index = -1;
int count = 0;
while ((index=tmp.indexOf(","))!=-1) {
tmp = tmp.substring(index+1);
count++;
}
return count;
}
public String getDelimiter() {
return delimiter;
}
public int getCount() {
return count;
}
public void setDelimiter(String delimiter) {
this.delimiter = delimiter;
}
public void setCount(int count) {
this.count = count;
}
}
(6)demo22-job.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:batch="http://www.springframework.org/schema/batch"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/batch http://www.springframework.org/schema/batch/spring-batch.xsd">
<!--导入文件-->
<import resource="classpath:demo22/job/demo22-jobContext.xml"/>
<!--定义名字为billJob的作业-->
<batch:job id="billJob">
<!--定义名字为billStep的作业步-->
<batch:step id="billStep">
<batch:tasklet transaction-manager="transactionManager">
<!--定义读、处理、写操作,规定每处理两条数据,进行一次写入操作,这样可以提高写的效率-->
<batch:chunk reader="csvItemReader" processor="creditBillProcessor" writer="csvItemWriter" commit-interval="2">
</batch:chunk>
</batch:tasklet>
</batch:step>
</batch:job>
</beans>
(7)demo22-jobContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--定义作业仓库 Job执行期间的元数据存储在内存中-->
<bean id="jobRepository" class="org.springframework.batch.core.repository.support.MapJobRepositoryFactoryBean">
</bean>
<!--定义作业调度器,用来启动job-->
<bean id="jobLauncher" class="org.springframework.batch.core.launch.support.SimpleJobLauncher">
<!--注入jobRepository-->
<property name="jobRepository" ref="jobRepository"/>
</bean>
<!--定义事务管理器,用于Spring Batch框架中对数据操作提供事务能力-->
<bean id="transactionManager" class="org.springframework.batch.support.transaction.ResourcelessTransactionManager"/>
<!--使用FlatFileItemReader读文本文件-->
<bean id="csvItemReader" class="org.springframework.batch.item.file.FlatFileItemReader" scope="step">
<!--指定读取的资源文件-->
<property name="resource" value="classpath:demo22/data/demo22-inputFile.csv"/>
<property name="recordSeparatorPolicy" ref="multiLineRecordSeparatorPolicy"/>
<property name="lineMapper" ref="lineMapper"/>
</bean>
<bean id="multiLineRecordSeparatorPolicy" class="com.xj.demo22.MultiLineRecordSeparatorPolicy">
<!--定义分隔符号-->
<property name="delimiter" value=","/>
<!--定义4个分隔符为一条完整记录-->
<property name="count" value="4"/>
</bean>
<!--声明行转换策略-->
<bean id="lineMapper" class="org.springframework.batch.item.file.mapping.DefaultLineMapper" >
<property name="lineTokenizer" ref="lineTokenizer" />
<property name="fieldSetMapper" ref="creditBillFieldSetMapper"/>
</bean>
<bean id="lineTokenizer" class="org.springframework.batch.item.file.transform.DelimitedLineTokenizer">
<property name="delimiter" value=","/>
<property name="names" value="accountID,name,amount,date,address" />
</bean>
<bean id="creditBillFieldSetMapper" class="com.xj.demo22.CreditBillFieldSetMapper">
</bean>
<!--注入实体类-->
<bean id="creditBill" class="com.xj.demo22.CreditBill" scope="prototype"/>
<bean id="creditBillProcessor" class="com.xj.demo22.CreditBillProcessor" scope="step"/>
<!--写信用卡账单文件,CSV格式-->
<bean id="csvItemWriter" class="org.springframework.batch.item.file.FlatFileItemWriter" scope="step">
<!--要写入的文件位置,因为[classpath:]不是一个具体的目录,这里应当用[file:](从项目根目录开始)指明输出位置-->
<property name="resource" value="file:src/main/resources/demo22/data/demo22-outputFile.csv"/>
<!--[lineAggregator成员]指明行聚合器,用来将对象输出到文件时构造文件中的每行的格式-->
<property name="lineAggregator">
<!--这里使用Spring Batch自带的DelimitedLineAggregator来作为行聚合器(可以拼接一个个属性形成行)-->
<bean class="org.springframework.batch.item.file.transform.DelimitedLineAggregator">
<!--使用","拼接-->
<property name="delimiter" value=","/>
<!--fieldExtractor成员用来将Java类的属性组成的数组拼接成行字符串-->
<property name="fieldExtractor">
<bean class="org.springframework.batch.item.file.transform.BeanWrapperFieldExtractor">
<property name="names" value="accountID,name,amount,date,address">
</property>
</bean>
</property>
</bean>
</property>
</bean>
</beans>
(8)demo22-inputFile.csv
4047390012345678,tom,100.00,2013-2-2 12:00:08
,Lu Jia Zui road
4047390012345678,tom,320.00,2013-2-3 10:35:21
,Lu Jia Zui road
4047390012345678,tom,674.70,2013-2-6 16:26:49
,South Linyi road
4047390012345678,tom,793.20,2013-2-9 15:15:37
,Longyang road
4047390012345678,tom,360.00,2013-2-11 11:12:38
,Longyang road
4047390012345678,tom,893.00,2013-2-28 20:34:19
,Hunan road
3.运行结果
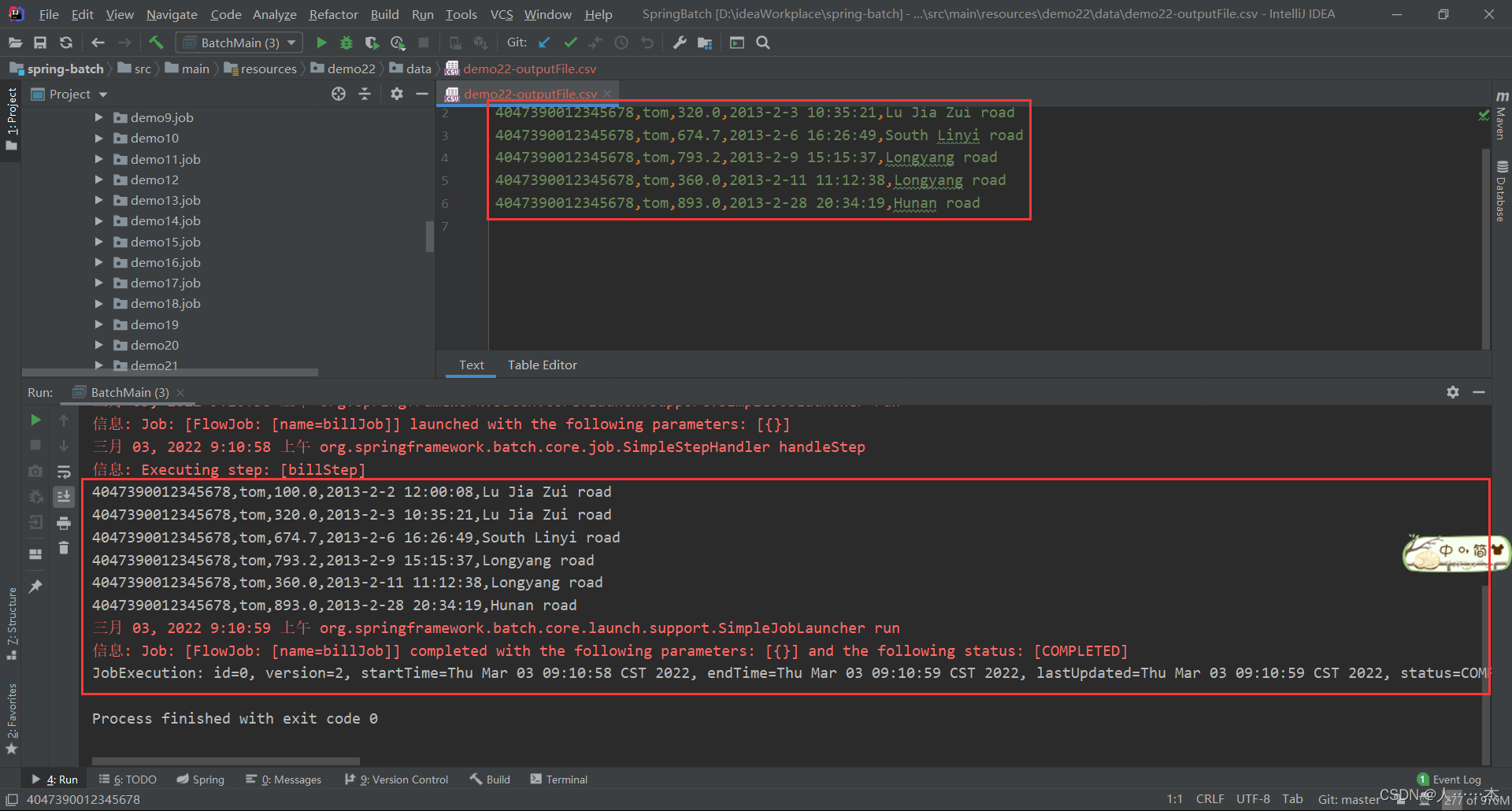
?
|