目录
一、注解开发
1、介绍
2、相关标签、属性介绍
3、步骤
1、在核心配置文件中配置映射关系
2、创建接口 和 查询方法:
3、创建测试类:
二、注解实现多表操作
一对一
1、相关属性 标签介绍
2、实现步骤
一对多
1、数据准备
1、实现步骤
多对多
1、数据准备
?2、实现步骤
一、注解开发
1、介绍
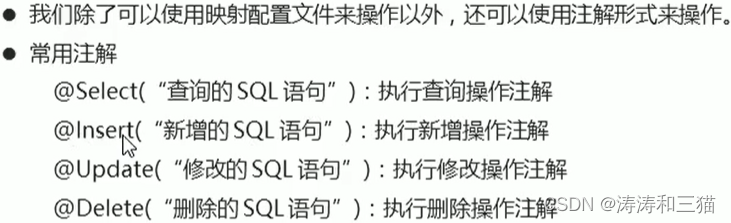
2、相关标签、属性介绍
3、步骤
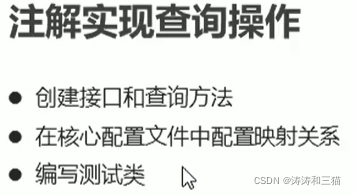
1、在核心配置文件中配置映射关系
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<!--核心根标签-->
<configuration>
<!--引入数据库连接的配置文件-->
<properties resource="jdbc.properties"/>
<!--配置LOG4J-->
<settings>
<setting name="logImpl" value="log4j"/>
</settings>
<!--起别名-->
<typeAliases>
<package name="com.itheima.bean"/>
</typeAliases>
<!-- 配置数据库环境, 环境可以有多个, defult属性是指哪一个 -->
<environments default="mysql">
<environment id="mysql">
<!--transactionManager事务管理。-->
<transactionManager type="JDBC"></transactionManager>
<dataSource type="POOLED">
<!--property获取数据库连接的配置信息-->
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<!--配置映射关系-->
<mappers>
<package name="com.itheima.mapper"/>
</mappers>
</configuration>
2、创建接口 和 查询方法:
package com.itheima.mapper;
import com.itheima.bean.Student;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import java.util.List;
public interface StudentMapper {
/**
* 单表操作
*/
//增
@Insert("insert into student values (#{id}, #{name}, #{age})")
public abstract Integer insert(Student student);
//删
@Delete("delete from student where id=#{id}")
public abstract Integer delete(Integer id);
//改
@Update("update student set name=#{name}, age=#{age} where id=#{id};")
public abstract Integer update(Student student);
//查
@Select("select * from student")
public abstract List<Student> selsctAll();
/**
* 多表操作
*/
}
3、创建测试类:
package com.itheima.test;
import com.itheima.bean.Student;
import com.itheima.mapper.StudentMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class Test01 {
@Test
public void selectAll() throws IOException {
//1、加载核心配置文件
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2、获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3、获取到SqlSession对象
SqlSession sqlSession = sqlSessionFactory.openSession(true);
//4、获取StudentMapper接口实现对象
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
//5、调用实现类对象中方法,接受结果
List<Student> students = mapper.selsctAll();
//6、处理结果
for (Student student : students) {
System.out.println(student);
}
//7、释放
sqlSession.close();
is.close();
}
@Test
public void insert() throws IOException {
//1、加载核心配置文件
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2、获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3、获取到SqlSession对象
SqlSession sqlSession = sqlSessionFactory.openSession(true);
//4、获取StudentMapper接口实现对象
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
//5、调用实现类对象中方法,接受结果
Student student = new Student(4, "赵六", 15);
Integer result = mapper.insert(student);
//6、处理结果
System.out.println(result);
//7、释放
sqlSession.close();
is.close();
}
@Test
public void update() throws IOException {
//1、加载核心配置文件
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2、获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3、获取到SqlSession对象
SqlSession sqlSession = sqlSessionFactory.openSession(true);
//4、获取StudentMapper接口实现对象
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
//5、调用实现类对象中方法,接受结果
Student student = new Student(4, "赵六", 99);
Integer result = mapper.update(student);
//6、处理结果
System.out.println(result);
//7、释放
sqlSession.close();
is.close();
}
@Test
public void delete() throws IOException {
//1、加载核心配置文件
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2、获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3、获取到SqlSession对象
SqlSession sqlSession = sqlSessionFactory.openSession(true);
//4、获取StudentMapper接口实现对象
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
//5、调用实现类对象中方法,接受结果
Integer id = 2;
Integer result = mapper.delete(id);
//6、处理结果
System.out.println(result);
//7、释放
sqlSession.close();
is.close();
}
}
二、注解实现多表操作
一对一
1、相关属性 标签介绍
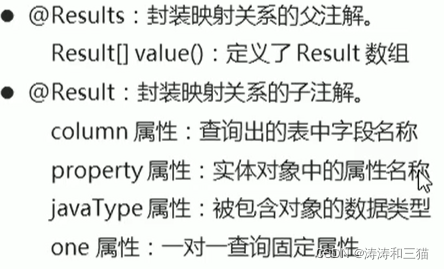
2、实现步骤
①创建接口和查询方法
package com.itheima.one_to_one;
import com.itheima.bean.Card;
import com.itheima.bean.Person;
import org.apache.ibatis.annotations.One;
import org.apache.ibatis.annotations.Result;
import org.apache.ibatis.annotations.Results;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface CardMapper {
//查询全部
@Select("select * from card")
@Results({
@Result(column = "id", property = "id"),
@Result(column = "number", property = "number"),
@Result(
property = "p", //被包含对象的变量名
javaType = Person.class, //被包含对象的实际数据类型
column = "pid", //代表根据查询出的card表中的pid字段来查询person表
/*
one、@One 一对一固定写法
select属性: 指调用哪个接口中的哪个方法
*/
one = @One(select = "com.itheima.one_to_one.PersonMapper.selectById")
)
})
public abstract List<Card> selectAll();
}
package com.itheima.one_to_one;
import com.itheima.bean.Person;
import org.apache.ibatis.annotations.Select;
public interface PersonMapper {
@Select("select * from person where id=#{id}")
public abstract Person selectById(Integer id);
}
?② 配置映射文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<!--核心根标签-->
<configuration>
<!--引入数据库连接的配置文件-->
<properties resource="jdbc.properties"/>
<!--配置LOG4J-->
<settings>
<setting name="logImpl" value="log4j"/>
</settings>
<!--起别名-->
<typeAliases>
<!--用于指定要配置别名的包,当指定后,该报下的实体类都会注册别名,并且类名就是别名,不再区分大小写-->
<package name="com.itheima.bean"/>
</typeAliases>
<!--集成分页助手插件-->
<!-- <plugins>-->
<!-- <plugin interceptor="com.github.pagehelper.PageInterceptor"/>-->
<!-- </plugins>-->
<!-- 配置数据库环境, 环境可以有多个, defult属性是指哪一个 -->
<environments default="mysql">
<environment id="mysql">
<!--transactionManager事务管理。-->
<transactionManager type="JDBC"></transactionManager>
<dataSource type="POOLED">
<!--property获取数据库连接的配置信息-->
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<!--配置映射关系-->
<mappers>
<package name="com.itheima.one_to_one"/>
</mappers>
</configuration>
③ 编写测试类
package com.itheima.one_to_one;
import com.itheima.bean.Card;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class Test01 {
@Test
public void selectAll() throws IOException {
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
SqlSession sqlSession = sqlSessionFactory.openSession(true);
CardMapper mapper = sqlSession.getMapper(CardMapper.class); // 实现类对象
List<Card> list = mapper.selectAll();
for (Card card : list){
System.out.println(card);
}
sqlSession.close();
is.close();
}
}
一对多
1、数据准备
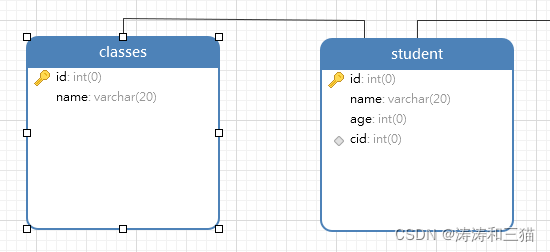
班级-学生 :一对多
要实现的查询:
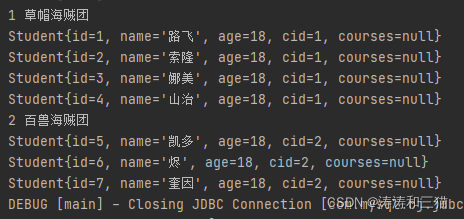
?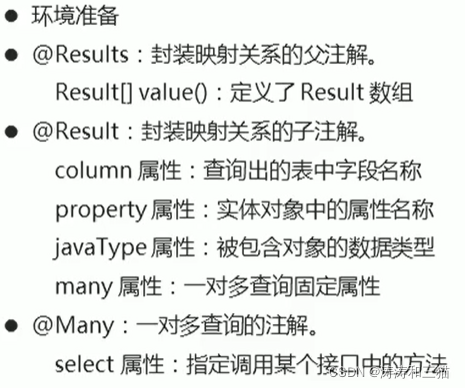
1、实现步骤
①配置映射文件
②创建接口和查询方法
public interface ClassesMapper {
@Select("select * from classes")
@Results({
@Result(column = "id", property = "id"),
@Result(column = "name", property = "name"),
@Result(
property = "students",
javaType = List.class,
column = "id", // 根据查询出来的classes表的id字段来查询student表
/**
* many = @Many:一对多查询的固定写法
* select属性: 指调用哪个接口中的哪个查询方法
*/
many = @Many(select = "com.itheima.one_to_many.StudentMapper.selectByCid")
)
})
public abstract List<Classes> selectAll();
}
package com.itheima.one_to_many;
import com.itheima.bean.Student;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface StudentMapper {
@Select("select * from student where cid = #{cid}")
public abstract List<Student> selectByCid(Integer cid);
}
③创建测试类
package com.itheima.one_to_many;
import com.itheima.bean.Card;
import com.itheima.bean.Classes;
import com.itheima.bean.Student;
import com.itheima.one_to_one.CardMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class Test01 {
@Test
public void selectAll() throws IOException {
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
SqlSession sqlSession = sqlSessionFactory.openSession(true);
ClassesMapper mapper = sqlSession.getMapper(ClassesMapper.class); // 实现类对象
List<Classes> list = mapper.selectAll();
for (Classes classes : list) {
System.out.println(classes.getId() +" "+ classes.getName());
List<Student> students = classes.getStudents();
for (Student student : students) {
System.out.println(student);
}
}
sqlSession.close();
is.close();
}
}
多对多
1、数据准备
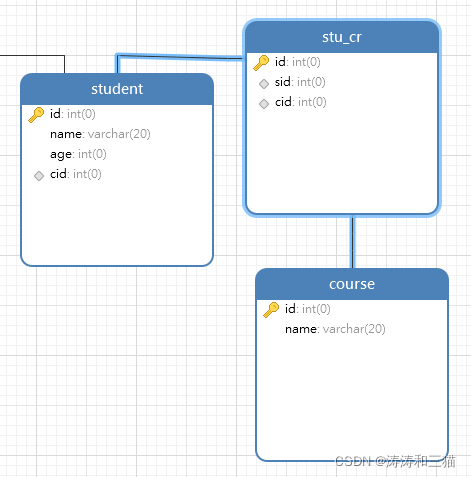
?2、实现步骤
①配置映射文件
②创建接口和查询方法
注意:这里是多对多可能会出现以下情况:比如我们要查询每一个学生的信息,包括他所有选的课程。有的学生没有选课也会被查询出来。这时候要借助中间表,将没有选课的学生过滤掉。
package com.itheima.many_to_many;
import com.itheima.bean.Student;
import org.apache.ibatis.annotations.Many;
import org.apache.ibatis.annotations.Result;
import org.apache.ibatis.annotations.Results;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface StudentMapper {
// 查询全部
@Select("select distinct s.id, s.name, s.age from student s, stu_cr sc where sc.sid = s.id")
@Results({
@Result(column = "id", property = "id"),
@Result(column = "name", property = "name"),
@Result(column = "age", property = "age"),
@Result(
property = "courses", //被包含对象的变量名
javaType = List.class, //被包含对象的实际数据类型
column = "id", //根绝查询出student表的id作为关联条件,去查询中间表和课程表
many = @Many(select = "com.itheima.many_to_many.CourseMapper.selectBySid")
)
})
public abstract List<Student> selectAll();
}
package com.itheima.many_to_many;
import com.itheima.bean.Course;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface CourseMapper {
@Select("select c.id,c.name from stu_cr sc, course c where sc.cid=c.id and sc.sid = #{id}")
public abstract List<Course> selectBySid(Integer id);
}
③编写测试类
package com.itheima.many_to_many;
import com.itheima.bean.Classes;
import com.itheima.bean.Course;
import com.itheima.bean.Student;
import com.itheima.one_to_many.ClassesMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class Test01 {
@Test
public void selectAll() throws IOException {
InputStream is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
SqlSession sqlSession = sqlSessionFactory.openSession(true);
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class); // 实现类对象
List<Student> list = mapper.selectAll();
for (Student student : list) {
System.out.println(student.getId() +" "+ student.getName() +" "+ student.getAge());
List<Course> courses = student.getCourses();
for (Course course : courses) {
System.out.println(course);
}
}
sqlSession.close();
is.close();
}
}
|