1、Collection
Java类集框架中提供了两大核心接口:Collection接口和Map接口,这两个接口是相对独立的。Collection接口是集合单值操作最大的父接口。
-
boolean add(E e) 添加一个元素。添加成功返回true,如果不允许重复并且已经包含指定的元素。返回false。 -
boolean addAll(Collection<? extends E> c) 将指定集合中的所有元素添加到此集合中。 -
void clear() 清空掉集合中的所有元素 -
boolean contains(Object o) 如果集合中包含指定元素那么返回true。 -
boolean containsAll(Collection<?> c) 如果该集合中包含指定集合中的所有元素的时候返回true。 -
boolean isEmpty() 如果集合中没有元素返回true。 -
boolean remove(Object o) 删除集合中的指定的元素。如果存在NULL,也删除。 -
boolean removeAll(Collection<?> c) 删除当前集合中所有等于指定集合中的元素。 -
boolean retainAll(Collection<?> c) 仅保留该指定集合中存在的所有元素。其余删除 -
int size() 返回该集合中元素的个数。如果超过了Integer.MAX_VALUE,那么返回Integer.MAX_VALUE。 -
Object[] toArray() 这个方法是集合和数组转化的桥梁。 -
Iterator iterator() 将集合变为Iterator接口输出。
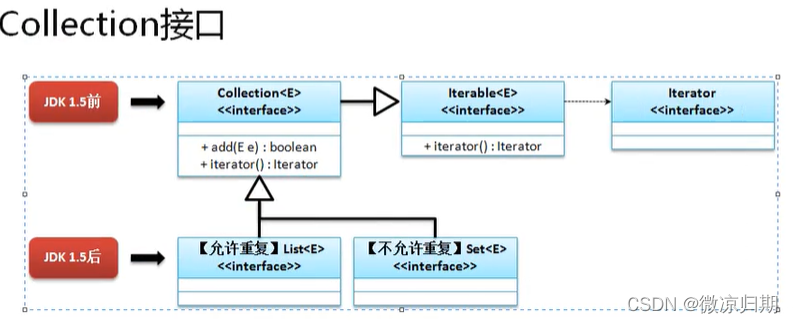 Collection中两个重要的方法add()和iterator(),子接口中都有。
2、List
(1)List接口是collection子接口,对collectio接口进行了进一步扩充,允许保存重复元素。List接口有两个重要的扩充方法:
- E get(int index)
根据索引取得保存数据。 - E set(int index,E element)
修改数据
(2)List有三个子类:ArrayList,Vector,LinkedList
public class TestArrayList {
public static void main(String[] args) {
List<String > list=new ArrayList<String>();
list.add("hello");
list.add("hello");
list.add("Java");
System.out.println("集合大小为:"+list.size()+" 集合是否为空:"+list.isEmpty());
for (int i=0;i<list.size();i++){
System.out.println(list.get(i));
}
System.out.println("删除hello后");
list.remove("hello");
System.out.println("集合大小为:"+list.size()+" 集合是否为空:"+list.isEmpty());
for (int i=0;i<list.size();i++){
System.out.println(list.get(i));
}
System.out.println(list.contains("hello"));
System.out.println(list.contains("ABC"));
System.out.println(list);
}
}
List允许保存重复数据。另外,get()方法是List子接口提供的。如果现在操作的是Collection接口,那么对于此时的数据取出只能够将集合变为对象数组操作。如下:
Collection<String > list=new ArrayList<String>();
list.add("hello");
list.add("hello");
list.add("Java");
Object[] objects=list.toArray();
System.out.println(Arrays.toString(objects));
用Collection接口很容易产生ClassCastException异常,因此在开发过程中尽量少用。
List<String> list = new LinkedList<>() ;
list.add("hello") ;
list.add("hello") ;
list.add("bit") ;
System.out.println(list) ;
list.remove("hello") ;
System.out.println(list) ;
ArrayList和LinkedList的区别
ArrayList | LinkedList |
---|
封装的是数组 | 封装的是链表 | 复杂度为1 | LinkedList的复杂度为n |
List<String > list = new Vector<String>();
list.add("hello");
list.add("hello");
list.add("Java");
System.out.println(list);
ArrayList和Vector的区别
ArrayList | Vector |
---|
异步处理,性能较高 | 同步处理,性能较低 | 线程不安全 | 线程安全 | 支持Iterator、ListIterator、foreach | 支持Iterator、ListIterator、foreach、Enumeration |
3、Set
Set也有两个子接口:HashSet(无序存储)和TreeSet(有序存储) (1)HashSet子类
Set<String> set=new HashSet<String>();
set.add("hello");
set.add("hello");
set.add("Java");
System.out.println(set);
从输出可以得到是不允许重复且无序的。
(2)TreeSet子类
Set<Integer> set=new TreeSet<Integer>();
set.add(1);
set.add(1);
set.add(2);
set.add(3);
set.add(4);
set.add(5);
System.out.println(set);
TreeSet同样不允许重复,输出是升序的。 对象判断必须两个方法equals()、hashCode()返回值都相同才判断为相同。
Set与List区别
set | list |
---|
不允许重复 | 允许重复 | 没有对Collection接口进行扩充 | 对Collection接口进行扩充 | 没有get()方法 | 有get()方法 | 无序:存储与添加顺序无关,无下标 | 有序:存储与添加顺序相同,可下标访问 |
4、 输出
(1)Iterator迭代输出 使用List,Set的时候,为了实现对其数据的遍历,我们经常使用到了Iterator
List<String> list = new ArrayList<>();
list.add("hello");
list.add("java");
Iterator<String> iterator = list.iterator();
while(iterator.hasNext()){
if(iterator.next().equals("hello")){
iterator.remove();
}
System.out.println(iterator.next());
}
System.out.println(list);
}
进行迭代输出时候使用collection的romove方法会导致并发更新异常,需要使用interator的romove方法删除。
(2)ListIterator双向迭代输出
Iterator只能实现由前到后输出,需要双向迭代输出ListIterator,它继承于Iterator接口,只能用于各种List类型的访问。
List<String> list = new ArrayList<>();
list.add("hello");
list.add("java");
ListIterator<String> iterator = list.listIterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
while(iterator.hasPrevious()){
System.out.println(iterator.previous());
}
Iterator和ListIterator主要区别
- ListIterator有add()方法向List中添加对象,而Iterator不能;
- 都有hasNext()和next()方法向后遍历,但是ListIterator有hasPrevious()和previous()方法,逆向遍历
- ListIterator可以定位当前的索引位置,nextIndex()和previousIndex()可以实现。Iterator没有此功能。
- 都可删除对象,但是ListIterator可以实现对象的修改,set()方法可以实现。Iierator仅能遍历,不能修改。
(3)Enumeration枚举输出 主要是为了Vector类提供输出服务的。如果想要获取Enumeration的接口对象,就必须依靠Vector类提供的方法。
- 获取Enumeration:public Enumeration elements();
- 在Enumeration接口中定义有两个操作方法:
判断是否有下一个元素:pubilc boolean hasMoreElements();
获取当前元素;public E nextElement();
package com.iterator.demo;
import java.util.Enumeration;
import java.util.Vector;
public class IteratorDemo {
public static void main(String[] args) {
Vector<String> all = new Vector<String>();
all.add("hello");
all.add("world");
all.add("sina");
all.add("sohu");
Enumeration<String> enu = all.elements();
while (enu.hasMoreElements()) {
String string = enu.nextElement();
System.out.print(string+"、");
}
}
}
(4)foreach输出
增强for循环。
public static void main(String[] args) {
List<String> arr = new ArrayList<String>();
arr.add("你好");
arr.add("我好");
arr.add("大家好");
for(String str : arr){
System.out.println(str);
}
}
5、Map
- Collection保存是为了输出,Map是为了key的查找;
- Map中的集合的键不能重复,值可以重复;每个键只能对应一个值;
- Collection中的集合称为单列集合,Map中的集合称为双列集合。
(1)Map接口中的常用方法
-
get方法:获取指定键(key)所对应的值(value); -
put方法:将指定的键与值对应起来添加到集合中,返回null,若该键已存在,把指定键所对应的值替换成新值并返回旧的值; -
remove方法:根据指定的键(key)删除元素,返回被删除元素的值(value); -
keySet():将集合中的key转为set集合;
public class MapDemo {
public static void main(String[] args) {
Map<String, String> map = new HashMap<String,String>();
map.put("邓1", "孙1");
map.put("李2", "范2");
map.put("刘3", "柳3");
Set<String> keySet = map.keySet();
Iterator<String> it =keySet.iterator(); **
while(it.hasNext()){
String key = it.next();
String value = map.get(key);
System.out.println(key+"="+value);
}
}
}
(2)HashMap子类
- 遍历顺序是不确定的;
- 最多只允许一条记录的键为null,允许多条记录的值为null;
- 非线程安全。如果需要满足线程安全,可用 Collections的synchronizedMap方法,或者使用ConcurrentHashMap;
- 默认最大保存16个元素;
HashMap<Integer, String> Sites = new HashMap<Integer, String>();
Sites.put(1, "Google");
Sites.put(2, "Runoob");
Sites.put(3, "Taobao");
Sites.put(4, "Zhihu");
for (Integer i : Sites.keySet()) {
System.out.println("key: " + i + " value: " + Sites.get(i));
}
for (String value : Sites.values()) {
System.out.print(value + ", ");
}
Sites.remove(4);
System.out.println(Sites.size());
System.out.println(Sites.get(1));
HashMap由数组+链表组成的,数组是HashMap的主体,链表则是主要为了解决哈希冲突而存在的。阈值小于8为链表,超过8转为红黑树。
(3)LinkedHashMap子类 HashMap的子类,为了实现有序,基于链表实现。
HashMap<Integer, String> Sites = new LinkedHashMap<>();
Sites.put(1, "Google");
Sites.put(2, "Runoob");
Sites.put(3, "Taobao");
Sites.put(4, "Zhihu");
for (Integer i : Sites.keySet()) {
System.out.println("key: " + i + " value: " + Sites.get(i));
}
for (String value : Sites.values()) {
System.out.print(value + ", ");
}
Sites.remove(4);
System.out.println(Sites.size());
System.out.println(Sites.get(1));
(4)Hashtable子类 HashMap与Hashtable区别
HashMap | Hashtable |
---|
k-v允许为空 | k-v不允许为空 | 异步操作,非线程安全 | 同步操作、线程安全 |
(5)Map.Entry内部接口 通过集合中每个键值对(Entry)对象,获取键值对(Entry)对象中的键与值
public class MapDemo {
public static void main(String[] args) {
Map<String, String> map = new HashMap<String,String>();
map.put("邓1", "孙1");
map.put("李2", "范2");
map.put("刘3", "柳3");
Set<Map.Entry<String,String>> entrySet = map.entrySet();
Iterator<Map.Entry<String,String>> it =entrySet.iterator();
while(it.hasNext()){
Map.Entry<String,String> entry = it.next();
String key = entry.getKey();
String value = entry.getValue();
System.out.println(key+"="+value);
}
}
}
(6)利用Iterator输出Map集合
- Map集合不能直接使用迭代器或者foreach进行遍历。但是转成Set之后就可以使用了。
Map<Integer,String> map = new HashMap<Integer,String>();
map.put(1,"a");
map.put(2,"b");
map.put(3,"c");
map.get(1);
Iterator<String> iter = map.keySet().iterator();
while (iter.hasNext()) {
int key = iter.next();
String value = map.get(key);
}
(7)自定义Map的key类型
- get 查询时候需要使用hashcode查找,自定义类型需要覆写equals与hashcode方法,否则无法查到。
- HashMap的Hash冲突:为了保证程序正常执行,会将冲突位置的内容转为链表保存。
6、 工具类
(1)Stack栈操作
栈(顶和底):先进后出,是vector的子类,空栈出栈抛出异常;
Stack<String> strings = new Stack<>();
strings.push("1");
strings.push("2");
System.out.println(strings.pop());
System.out.println(strings.pop());
System.out.println(strings.pop());
}
(2)Queue队列
队列(头和尾):先进先出; LinkedList类实现了Queue接口,因此我们可以把LinkedList当成Queue来用;
Queue<String> queue = new LinkedList<>();
queue.offer("a");
queue.offer("X");
queue.offer("b");
for(String q : queue){
System.out.println(q);
}
System.out.println("poll=" + queue.poll());
System.out.println("element=" + queue.element());
System.out.println("peek=" + queue.peek());
执行结果:
a
X
b
poll=a
element=X
peek=X
- PriorityQueue
优先队列PriorityQueue是Queue接口的实现,可以对其中元素进行排序; 可以放基本数据类型的包装类(如:Integer,Long等)或自定义的类; 对于基本数据类型的包装器类,优先队列中元素默认排列顺序是升序排列;
Queue<String> queue = new PriorityQueue<>();
queue.offer("a");
queue.offer("X");
queue.offer("b");
for(String q : queue){
System.out.println(q);
}
System.out.println("poll=" + queue.poll());
System.out.println("element=" + queue.element());
System.out.println("peek=" + queue.peek());
执行结果:
X
a
b
poll=X
element=a
peek=a
(3)Properties属性操作 Properties类是Hashtable,只能操作String类型; 它提供了几个主要的方法:
-
getProperty ( String key),用指定的键搜索属性。也就是通过 key 得到 value。 -
load ( InputStream inStream),从输入流中读取属性列表(键和元素对)。获取该文件中的所有键 - 值对。以供 getProperty ( String key) 来搜索。 -
setProperty ( String key, String value) ,调用 Hashtable 的方法 put 。调用基类的put方法来设置 键 - 值对。 -
store ( OutputStream out, String comments),以适合使用 load 方法加载到 Properties 表中的格式,将此 Properties 表中的属性列表(键和元素对)写入输出流。与 load 方法相反,该方法将键 - 值对写入到指定的文件中去。 -
clear (),清除所有装载的 键 - 值对。
实例:
Properties pps = System.getProperties();
- 根据key读取value,读取properties的全部信息,写入新的properties信息
name=JJ
Weight=4444
Height=3333
public class TestProperties {
public static String GetValueByKey(String filePath, String key) {
Properties pps = new Properties();
try {
InputStream in = new BufferedInputStream (new FileInputStream(filePath));
pps.load(in);
String value = pps.getProperty(key);
System.out.println(key + " = " + value);
return value;
}catch (IOException e) {
e.printStackTrace();
return null;
}
}
public static void GetAllProperties(String filePath) throws IOException {
Properties pps = new Properties();
InputStream in = new BufferedInputStream(new FileInputStream(filePath));
pps.load(in);
Enumeration en = pps.propertyNames();
while(en.hasMoreElements()) {
String strKey = (String) en.nextElement();
String strValue = pps.getProperty(strKey);
System.out.println(strKey + "=" + strValue);
}
}
public static void WriteProperties (String filePath, String pKey, String pValue) throws IOException {
Properties pps = new Properties();
InputStream in = new FileInputStream(filePath);
pps.load(in);
OutputStream out = new FileOutputStream(filePath);
pps.setProperty(pKey, pValue);
pps.store(out, "Update " + pKey + " name");
}
public static void main(String [] args) throws IOException{
WriteProperties("Test.properties","long", "212");
}
}
执行结果:
Test.properties中文件的数据为:
name=JJ
Weight=4444
long=212
Height=3333
(4)Collections工具类
Queue<String> queue = new LinkedList<>();
Collections.addAll(queue,"1","2");
List<String> queue = new LinkedList<>();
Collections.addAll(queue,"1","2");
Collections.sort(queue);
System.out.println(Collections.binarySearch(queue,"2"));
Collection与Collections区别 Collection是集合接口,Collections是集合操作工具类,二者没有本质联系;
7、关系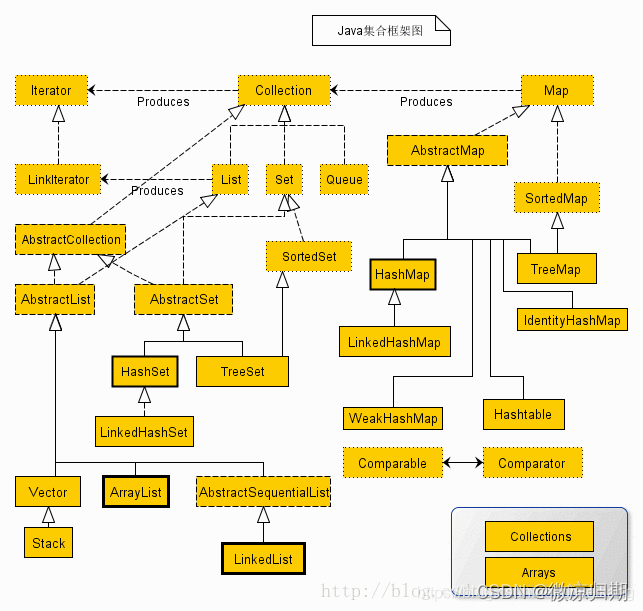
|