简单说明
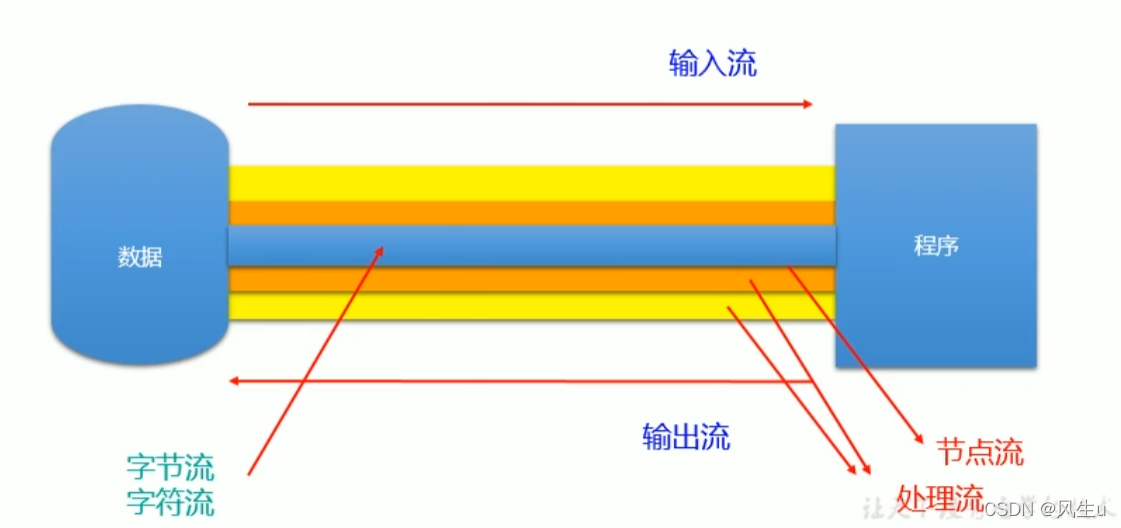
流的分类:
1.操作数据单位:字节流、字符流2.数据的流向:输入流、输出流3.流的角色:节点流、处理流
二、流的体系结构
抽象基类 节点流 缓冲流(处理流的一种)
InputStream FileInputStream BufferedInputStream
Outputstream FileOutputStream BufferedOutputstream
Reader FileReader BufferedReader
Writer FileWriter BufferedWriter
- 测试FiLeInputStream和FileoutpuStream的使用
- 结论:
- 1.对于文本文件(.txt,.java,.c,.cpp),使用字符流处理
- 2.对于非文本文件( .jpg,.mp3,.mp4,.avi,.doc,.ppt,…),使用字节流处理
- 使用字节流FiLeInputStream处理文本文件,可能出现乱码。
处理流之二:转换流的使用 1.转换流:属于字符流
InputStreamReader:将一个字节的输入流转换为字符的输入流OutputStreamwriter:将一个字符的输出流转换为字节的输出流 作用:提供字节流与字符流之间的转换
3.解码:字节、字节数组—>字符数组、字符串 编码:字符数组、字符串—>字节、字节数组
4.字符集 ASCII:美国标准信息交换码。用一个字节的7位可以表示. IS 08859-1:拉丁码表.欧洲码 表用一个字节的8位表示. GB2312:中国的中文编码表。最多两个字节编码所有字符 GBK:中国的中文编码表升级,融合了更多的中文文字符号。最多两个字节编码 Unicode:国际标准码,融合了目前人类使用的所有字符。为每个字符分配唯一的字符码。所有的字符,为每一个字符分配唯一的字符码,所有的文字都用两个字节来表示 UTF-8:变长的编码方式,可用1-4个字节来表示一个字符。
注意
public static void main(String[] args){
File file=new File("hello .txt");
System.out.println(file.getAbsolutePath());
File file1=new File("Java测试\\hello.txt");
System.out.println(file1);
}
File类的使用
- File类的一个对象,代表一个文件或一个文件目录(俗称:文件夹)
2.File类声明在java.io包下
3.File类中涉及到关于文件或文件目录的创建、删除、重命名、修改时间、文件大小等方法,并未涉及到写入或读取文件内容的操作。如果需要读取或写入文件内容,必须使用Io流来完成。
4.后续FiLe类的对象常会作为参数传递到流的构造器中,指明读取或写入的"终点".
File类的构造器
public class FileTest {
@Test
public void test1(){
File file1=new File("hello.txt");
File file2=new File("E:\\he.txt");
System.out.println(file1);
System.out.println(file2);
File file3=new File("E:","ff");
System.out.println(file3);
File file4=new File(file3,"hi.txt");
}
File类中的常用方法
对于文件
@Test
public void test2(){
File file1 = new File("hello.txt" );
File file2 =new File("E:\\ff\\hi.txt");
System.out.println(file1.getAbsolutePath()) ;
System.out.println(file1.getPath());
System.out.println(file1.getName( ));System.out.println(file1.getParent());System.out.println(file1.length());
System.out.println(new Date(file1.lastModified()));
System.out.println();
System.out.println(file2.getAbsolutePath());
System.out.println(file2.getPath());
System.out.println(file2.getName( ));System.out.println(file2.getParent( ));
System. out.println(file2.length());
System.out.println(file2.lastModified());
}
对于文件路径
@Test
public void test3(){
File file= new File("D:\\OneDrive\\桌面\\DemoTest\\Java常用类\\FengSheng\\src");
String list[] = file.list();
for(String f:list){
System.out.println(f);
}
System.out.println();
File[] files=file.listFiles();
for(File f:files){
System.out.println(f);
}
}
把文件重命名为指定的文件路径
@Test
public void test4(){
File file2=new File("hello.txt");
File file1=new File("E:\\ff\\aa.txt");
boolean rename=file1.renameTo(file2);
System.out.println(rename);
}
判断文件属性的方法
@Test
public void test5(){
File file1=new File("hello.txt");
System.out.println(file1.isDirectory());
System.out.println(file1.isFile());
System.out.println(file1.exists());
System.out.println(file1.canRead());
System.out.println(file1.canWrite());
System.out.println(file1.isHidden());
System.out.println();
File file2=new File("E:\\ff");
System.out.println(file2.isDirectory());
System.out.println(file2.isFile());
System.out.println(file2.exists());
System.out.println(file2.canRead());
System.out.println(file2.canWrite());
System.out.println(file2.isHidden());
}
创建和删除文件或文件目录的方法
@Test
public void test6() throws IOException {
File file1=new File("hi.txt");
if(!file1.exists()){
file1.createNewFile();
System.out.println("创建成功");
}else{
file1.delete();
System.out.println("删除成功");
}
}
@Test
public void test7(){
File file1=new File("E:\\ff\\aa");
boolean mkdir=file1.mkdir();
if(mkdir){
System.out.println("创建成功1");
}
File file2=new File("E:\\ff\\aa");
boolean mkdirs=file2.mkdirs();
if(mkdirs){
System.out.println("创建成功2");
}
}
FileReader
@Test
public void test1() {
FileReader fr= null;
try {
File file=new File("hello.txt");
fr = new FileReader(file);
int data;
while((data=fr.read())!=-1){
System.out.println((char)data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(fr!=null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void testFileReader1( ){
FileReader fr= null;
try {
File file=new File("hello.txt");
fr = new FileReader(file);
char[] cbuf=new char[5];
int len;
while((len=fr.read(cbuf))!=-1){
String str=new String(cbuf,0,len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(fr!=null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
FileWriter
@Test
public void testFilewriter() throws IOException {
File file = new File( "hello1.txt" );
FileWriter fw = new FileWriter(file,true);
fw.write( "I have a dream ! \n" );
fw.write( "you need to have a dream! " );
fw.close();
}
@Test
public void test2(){
FileReader fr= null;
FileWriter fw= null;
try {
File f1=new File("hello.txt");
File f2=new File("hello1.txt");
fr = new FileReader(f1);
fw = new FileWriter(f2);
char[] cubf=new char[5];
int len;
while((len=fr.read(cubf))!=-1){
fw.write(cubf,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fw.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
FileInputStream和FileOutputStream
@Test
public void testFileInputStream()throws IOException {
File file=new File("hello.txt");
FileInputStream fis=new FileInputStream(file);
byte[] by=new byte[5];
int len;
while((len=fis.read(by))!=-1){
String str=new String(by,0,len);
System.out.print(str);
}
}
@Test
public void testFileInputOutputStream() throws IOException {
File file=new File("src\\aa.jpg");
FileInputStream fis=new FileInputStream(file);
FileOutputStream fos=new FileOutputStream("少女.jpg");
byte[] by=new byte[5];
int len;
while((len=fis.read(by))!=-1){
fos.write(by,0,len);
}
fis.close();
fos.close();
}
缓冲流
- 处理流之一:缓冲流的使用
- 1.缓冲流: 套接在已有的流的基础之上
- BufferedInputStream
- BufferedoutputStream
- BufferedReader
- Bufferedwriter
- 2.作用:提供流的读取、写入的速度
- 提高读写速度的原因:内部提供了一个缓冲区
@Test
public void bufferedStreamTest() throws IOException{
File srcFile = new File( "src\\bb.jpg");
File destFile = new File( "呱呱.jpg" );
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
BufferedInputStream bis = new BufferedInputStream(fis );
BufferedOutputStream bos = new BufferedOutputStream(fos);
byte[] by=new byte[10];
int len;
while((len=bis.read(by))!=-1){
bos.write(by,0,len);
}
bis.close();
bos.close();
fis.close();
fos.close();
}
@Test
public void test1()throws Exception{
BufferedReader br=new BufferedReader(new FileReader(new File("hello.txt")));
BufferedWriter bw=new BufferedWriter(new FileWriter(new File("hello1.txt")));
char[] cbuf = new char[ 1024];int len;
String str;
while((str=br.readLine())!=null){
bw.write(str);
bw.newLine();
}
bw.close();
br.close();
}
转换流(修改文件字符集)
@Test
public void test1() throws Exception{
FileInputStream fis = new FileInputStream("hello.txt");
InputStreamReader isr = new InputStreamReader(fis,"UTF-8");
char[] cbuf = new char[ 20];
int len;
while((len = isr.read(cbuf)) != -1){
String str = new String( cbuf,0,len);
System.out.print(str);
}
isr.close();
}
@Test
public void test2() throws Exception{
FileInputStream fis=new FileInputStream("hello.txt");
FileOutputStream fos=new FileOutputStream("hello1.txt");
InputStreamReader is=new InputStreamReader(fis,"utf-8");
OutputStreamWriter os=new OutputStreamWriter(fos,"gbk");
char[] arr=new char[20];
int len;
while((len=is.read(arr))!=-1){
os.write(arr,0,len);
}
is.close();
os.close();
}
数据流
1 DataInputStream和DataoutputStream 作用:用于读取或写出基本数据类型的变量或字符串
练习:将内存中的字符串、基本数据类型的变量写出到文件中
注意:处理异常的话,仍然应该使用try-catch-finally
@Test
public void test1() throws Exception{
DataOutputStream dos = new DataOutputStream( new FileOutputStream("dd.txt"));
dos.writeUTF( "刘建辰");
dos.flush( );
dos.writeInt( 23);
dos.flush( );
dos.writeBoolean( true);
dos.flush( );
dos.close();
}
@Test
public void test2() throws Exception{
DataInputStream dis = new DataInputStream(new FileInputStream( "D:\\OneDrive\\桌面\\DemoTest\\Java测试\\dd.txt"));
String name = dis.readUTF();int age = dis.readInt();
boolean isMale = dis.readBoolean();
System.out.println( "name = " +name);System.out.println( "age = " + age);
System.out.println( "isMale = " + isMale);
dis.close();
}
标准的输入输出流
1.标准的输入、输出流
1.1 System.in:标准的输入流,默认从键盘输入System.out:标准的输出流,默认从控制台输出
1.2 system类的setIn(InputStream is) / setOut(PrintStream ps)方式重新指定输入和输出的流
1.3练习: 从键盘输入字符串,要求将读取到的整行字符串转成大写输出。然后继续进行输入操作, 直至当输入“e”或者"exit”时,退出程序。
方法一:使用Scanner实现,调用next()返回一个字符串 方法二:使用System.in实现。System.in—>转换流—>BufferedReader的readLine()
@Test
public void test1()throws Exception{
InputStreamReader isr=new InputStreamReader(System.in);
BufferedReader br=new BufferedReader(isr);
while(true){
String data=br.readLine();
if("e".equalsIgnoreCase(data)||"exit".equalsIgnoreCase(data))
break;
String upper=data.toUpperCase();
System.out.println(upper);
}
br.close();
}
自定义输入类
public class MyInput {
public static String readString() {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String string = "";
try {
string = br.readLine();
} catch (IOException ex) {
System.out.println(ex);
}
return string;
}
public static int readInt() {
return Integer.parseInt(readString());
}
public static double readDouble() {
return Double.parseDouble(readString());
}
public static double readByte() {
return Byte.parseByte(readString());
}
public static double readShort() {
return Short.parseShort(readString());
}
public static double readLong() {
return Long.parseLong(readString());
}
public static double readFloat() {
return Float.parseFloat(readString());
}
}
对象流
对象流的使用: 1.ObjectInputStream 2.ObjectOutPutStream 用于存储和读取基本数据类型数据或对象的处理流。它的强大之处就是可以把Java中的对象写入到数据源中,也能把对象从数据源中还原回来。 对象序列化机制允许把内存中的Java对象转换成平台无关的二进制流,从而允许把这种二进制流持久地保存在磁盘上,或通过网络将这种二进制流传输到另一个网络节点。 当其它程序获取了这种二进制流,就可以恢复成原来的Java对象
自定义类实现序列化和反序列化
Person需要满足如下的要求,方可序列化 1.需要实现接口: SerializabLe 2.当前类提供一个全局常量: serialVersionUID: 如果类没有显示定义这个静态常量,它的值是Java运行时环境根据类的内部细节自动生成的。 若类的实例变量做了修改,serialVersionUID可能发生变化。故建议,显式声明。 ObjectOutputStream和ObjectInputStream不能序列化static和transient修饰的成员变量 因为static修饰的属性不归类所有,二transient关键字是专门保证其修饰的属性不被序列化
public class Person implements Serializable {
public static final long serialVersionUID=653213154688678L;
String name;
int age;
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public Person() {
}
序列化
@Test
public void test1() throws IOException {
ObjectOutputStream oos=new ObjectOutputStream(new FileOutputStream("object.dat"));
oos.writeObject(new String("呱呱"));
oos.flush();
oos.writeObject(new Person("aaa",18));
oos.flush();
oos.close();
}
反序列化
@Test
public void test2()throws Exception{
ObjectInputStream ois=new ObjectInputStream(new FileInputStream("object.dat"));
String str=(String)ois.readObject();
Person p=(Person)ois.readObject();
System.out.println(str);
System.out.println(p);
ois.close();
}
随机存储文件流RandomAccessFile
public class Demo {
@Test
public void test1()throws Exception{
RandomAccessFile raf1 = new RandomAccessFile(new File( "D:\\OneDrive\\桌面\\DemoTest\\Java测试\\少女.jpg"),"r");
RandomAccessFile raf2 = new RandomAccessFile(new File( "D:\\OneDrive\\桌面\\DemoTest\\Java测试\\少女1.jpg"), "rw");
byte[] buffer = new byte[1024];
int len;
while(( len = raf1.read ( buffer)) != -1){
raf2.write(buffer,0,len);
}
raf1.close();
raf2.close();
}
@Test
public void test2() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("hello.txt"),"rw");
raf1.write("xyz".getBytes());
raf1.close();
}
@Test
public void test3()throws Exception{
RandomAccessFile raf1 = new RandomAccessFile( "hello.txt","rw");
raf1.seek(3);
StringBuilder builder = new StringBuilder((int) new File("hello.txt").length());
byte[] buffer = new byte[20];
int len;
while((len = raf1.read(buffer)) != -1){
builder.append(new String(buffer,0,len)) ;
}
raf1.seek(3);
raf1.write( "xyz".getBytes());
raf1.write( builder.toString().getBytes());
raf1.close();
}
|