JDBC的本质
是一堆接口,sun公司定义的一套操作所有关系型数据库的规则。各个数据库厂商去实现这套接口,提供数据库驱动jar包。我们可以使用这套接口(JDBC)编程,真正执行的代码是驱动jar包中的实现类。
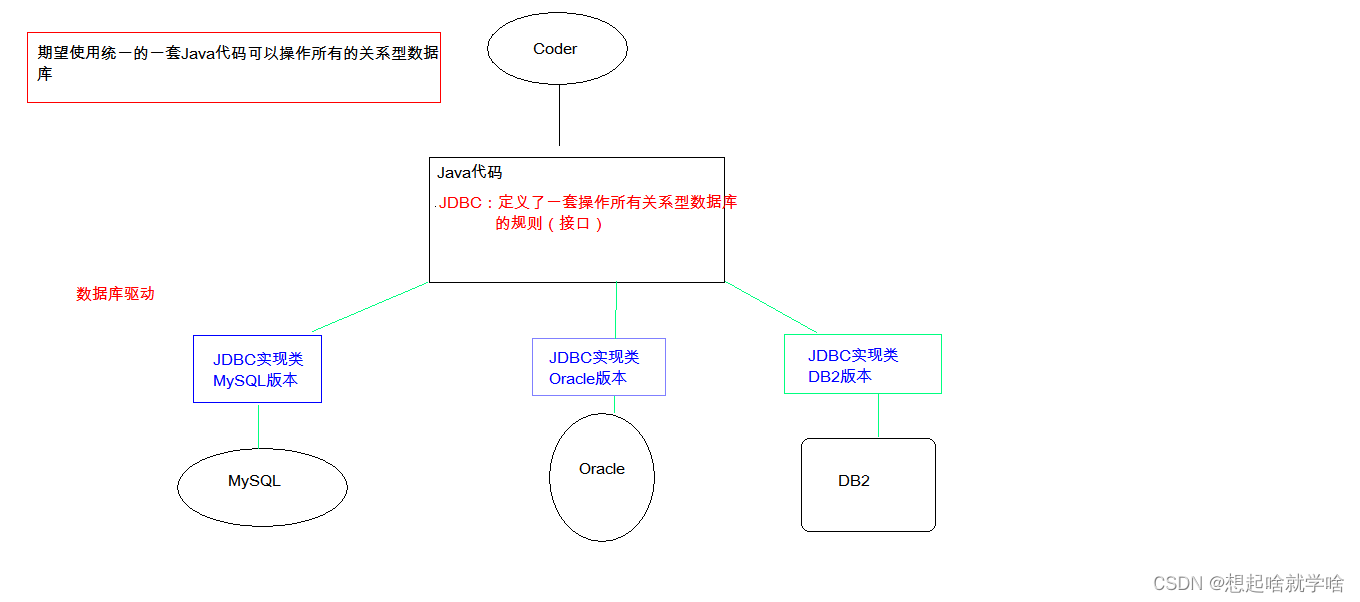
JDBC使用步骤
????????1.注册驱动
????????2.获取数据库链接
????????3.获取数据库操作对象
????????4.执行sql语句
????????5.处理查询结果集(查询语句才需要)
????????6.关闭资源
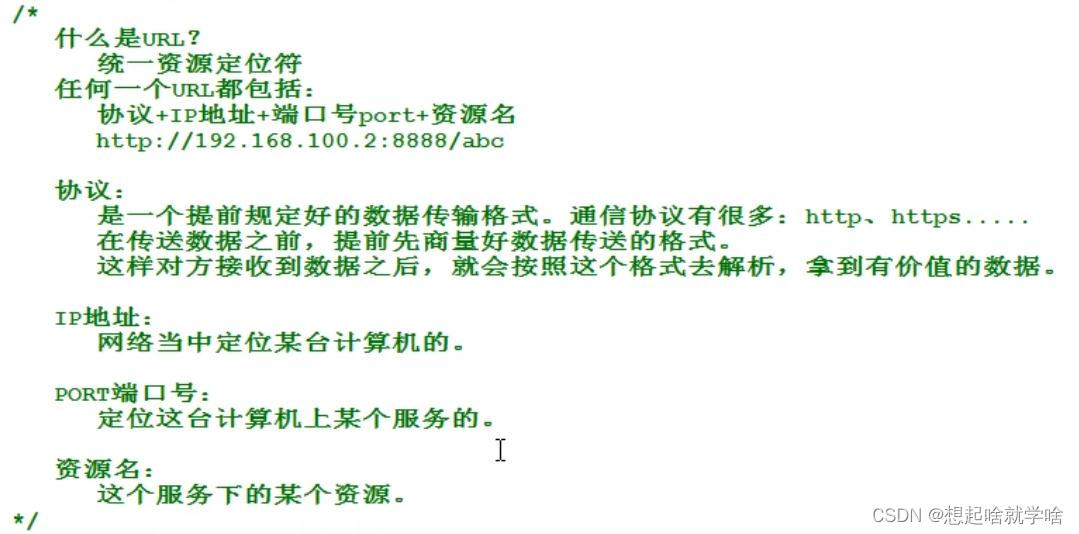
增删改操作?
public class JdbcDemo1 {
public static void main(String[] args) {
try {
// 注册驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 获取数据库链接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/companytest?serverTimezone=UTC&characterEncoding=utf8&useUnicode=true&useSSL=false", "root", "123456");
// 获取数据库操作对象
Statement statement = conn.createStatement();
// 执行sql语句
int i = statement.executeUpdate("insert into dept (deptno,dname,loc) values (50,'销售部','上海')");
System.out.println(i);
// 关闭资源
statement.close();
conn.close();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
查询操作?
@Test
public void testRead(){
Connection conn = null;
Statement statement = null;
ResultSet rs = null;
try {
// 加载驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 获取数据库链接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/companytest?serverTimezone=UTC&characterEncoding=utf8&useUnicode=true&useSSL=false", "root", "123456");
// 获取数据库对象
statement= conn.createStatement();
// 执行sql语句
rs = statement.executeQuery("select deptno,dname,loc from dept ");
// 处理结果集
while (rs.next()){
System.out.println(rs.getString("deptno") + "--" + rs.getString("dname") + "--" + rs.getString("loc"));
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally { // 关闭资源
if (rs != null){
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(statement != null){
try {
statement.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
|