概念
工厂模式(Factory Pattern)是 Java 中最常用的设计模式之一。 这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。 ?
?
意图
- 定义一个创建对象的接口,让其子类自己决定实例化哪一个工厂类,
- 工厂模式使其创建过程延迟到子类进行。
?
工厂一般分类有以下:
工厂模式实践
简单工厂
实现一个国家工厂,不同的国家人讲不同的hello ?
?
1、先创建一个接口,用来表示国家
package factory;
public interface Country {
void hello();
}
2、实现三个不同的国家类
package factory;
public class American implements Country {
@Override
public void hello() {
System.out.println("hello American");
}
}
package factory;
public class Chinese implements Country {
@Override
public void hello() {
System.out.println("你好");
}
}
package factory;
public class English implements Country {
@Override
public void hello() {
System.out.println("hello english");
}
}
3、创建一个工厂类和创建实例方法
package factory;
import javax.print.DocFlavor;
public class CountryFactory {
public Country getCountry(String type){
type = type.toLowerCase();
switch (type){
case "chinese":
return new Chinese();
case "american":
return new American();
case "english":
return new English();
default:
return null;
}
}
}
package factory;
import java.util.HashMap;
public class CountryFactory2 {
private static HashMap<String,Country> map=new HashMap<>();
static{
map.put("chinese",new Chinese());
map.put("american",new Chinese());
map.put("english",new Chinese());
}
public Country getCountry(String type){
type = type.toLowerCase();
return map.getOrDefault(type,null);
}
}
4、测试结果
package factory;
public class Test {
public static void main(String[] args) {
CountryFactory countryFactory = new CountryFactory();
countryFactory.getCountry("Chinese").hello();
countryFactory.getCountry("American").hello();
countryFactory.getCountry("English").hello();
}
}
?
优点
1.解耦 添加新的对象,只需要找工厂类中添加即可,即也不需要关注业务逻辑 2.帮助封装,使面向接口编程;
缺点
1.不方便子工厂的扩展
工厂方法
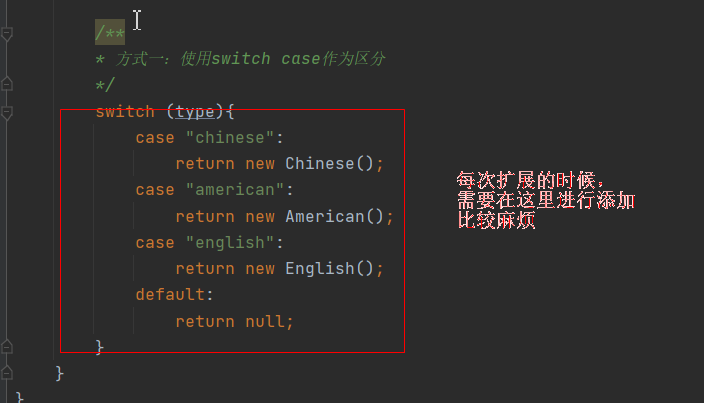
案例:创建一个人种的工厂方法 1、创建一个人种父类
package factory.factoryMethod;
import lombok.Data;
@Data
public class Human {
String type;
String skin;
public Human(String type, String skin) {
this.type = type;
this.skin = skin;
}
public void desc(){
System.out.println("我是"+type+" 肤色是:"+skin);
}
}
2、创建三个人种,都继承human类
package factory.factoryMethod;
public class BlackRace extends Human{
public BlackRace(String type, String skin) {
super(type, skin);
}
}
package factory.factoryMethod;
public class WhiteRace extends Human{
public WhiteRace(String type, String skin) {
super(type, skin);
}
}
package factory.factoryMethod;
public class YellowRace extends Human{
public YellowRace(String type, String skin) {
super(type, skin);
}
}
3、创建工厂抽象类
package factory.factoryMethod;
public abstract class HumanAbstractFactory {
public Human createHuman(){
return null;
}
}
4、创建三个具体人种的工厂类,都继承工厂抽象类。这里是扩展的关键
package factory.factoryMethod;
public class WhiteFactory extends HumanAbstractFactory{
@Override
public Human createHuman() {
return new WhiteRace("白种人","白色");
}
}
package factory.factoryMethod;
public class YellowFactory extends HumanAbstractFactory{
@Override
public Human createHuman() {
return new YellowRace("黄种人","黄色");
}
}
package factory.factoryMethod;
public class BlackFactory extends HumanAbstractFactory{
@Override
public Human createHuman() {
return new BlackRace("黑种人","黑色");
}
}
5、测试
package factory.factoryMethod;
public class Test {
public static void main(String[] args) {
new BlackFactory().createHuman().desc();
new WhiteFactory().createHuman().desc();
new YellowFactory().createHuman().desc();
}
}
工厂方法优势
1.满足JAVA的开闭原则,即如果需要新增加产品,只需要简单继承即可 2.每一种工厂只负责生产一种产品,保证单独处理业务逻辑,减少了简单工厂中switch判断
缺点
1.只能创建单一的产品 2.每新增加产品,都需要继承父类 增加系统复杂性
参考案例:https://www.cnblogs.com/zhucj-java/p/13598013.html
|