spring+mybatis
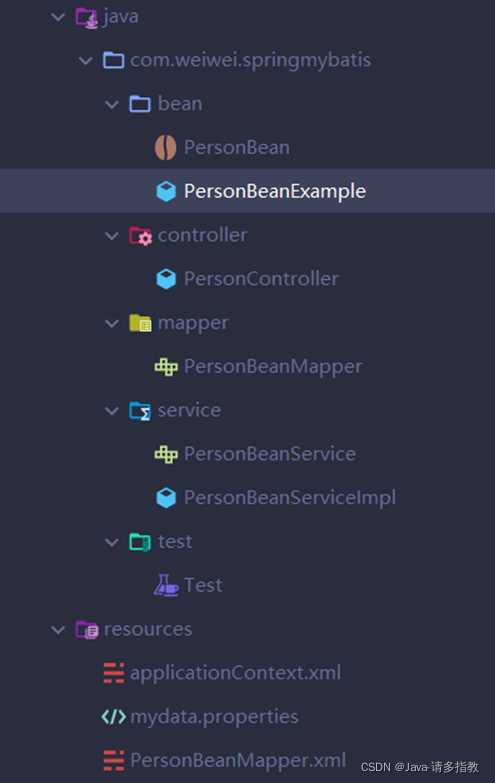
PersonBean和PersonBeanExample和PersonBeanMapper和PersonBeanMapper为mybatis逆向工程工具生产的。直接复制进。
需要导入的依赖包:
<!-- https://mvnrepository.com/artifact/org.springframework/spring-context -->
??? <dependency>
????? <groupId>org.springframework</groupId>
????? <artifactId>spring-context</artifactId>
????? <version>5.3.16</version>
??? </dependency>
??? <!-- spring-jdbc -->
??? <!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc -->
??? <dependency>
????? <groupId>org.springframework</groupId>
????? <artifactId>spring-jdbc</artifactId>
????? <version>5.3.16</version>
??? </dependency>
??? <!-- spring_tx -->
??? <!-- https://mvnrepository.com/artifact/org.springframework/spring-tx -->
??? <dependency>
????? <groupId>org.springframework</groupId>
????? <artifactId>spring-tx</artifactId>
????? <version>5.3.16</version>
??? </dependency>
??? <!-- MyBatis依赖 -->
??? <!-- https://mvnrepository.com/artifact/org.mybatis/mybatis -->
??? <dependency>
????? <groupId>org.mybatis</groupId>
????? <artifactId>mybatis</artifactId>
????? <version>3.5.9</version>
??? </dependency>
??? <!-- mybatis-spring 整合包 -->
??? <dependency>
????? <groupId>org.mybatis</groupId>
????? <artifactId>mybatis-spring</artifactId>
????? <version>2.0.7</version>
??? </dependency>
??? <!-- mysql数据库驱动 -->
??? <!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
??? <dependency>
????? <groupId>mysql</groupId>
????? <artifactId>mysql-connector-java</artifactId>
????? <version>8.0.25</version>
??? </dependency>
??? <!--druid 阿里的连接池-->
??? <dependency>
????? <groupId>com.alibaba</groupId>
????? <artifactId>druid</artifactId>
????? <version>1.2.8</version>
</dependency>
mydata.properties
driver=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/student_db?serverTimezone=UTC
jdbc.username=root
password=123456
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
?????? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
?????? xmlns:context="http://www.springframework.org/schema/context"
?????? xsi:schemaLocation="http://www.springframework.org/schema/beans
?????? http://www.springframework.org/schema/beans/spring-beans.xsd
?????? http://www.springframework.org/schema/context
?????? http://www.springframework.org/schema/context/spring-context.xsd">
<!--??? 配置自动扫描包-->
??? <context:component-scan base-package="com.weiwei.springmybatis"/>
<!-- 配置加载mydata.properties-->
??? <context:property-placeholder location="classpath:mydata.properties"/>
<!-- 配置数据源-->
??? <bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
??????? <property name="driverClassName" value="${driver}"/>
??????? <property name="url" value="${url}"/>
??????? <property name="username" value="${jdbc.username}"/>
??????? <property name="password" value="${password}"/>
??? </bean>
<!--??? 配置SqlSessionFactoryBean-->
??? <bean class="org.mybatis.spring.SqlSessionFactoryBean">
??????? <property name="dataSource" ref="dataSource"/>
??????? <property name="mapperLocations" value="classpath:PersonBeanMapper.xml"/>
??? </bean>
<!--??? 创建数据库访问接口对象-->
??? <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
??????? <property name="basePackage" value="com.weiwei.springmybatis.mapper"/>
??? </bean>
</beans>
PersonBeanService
package com.weiwei.springmybatis.service;
import com.weiwei.springmybatis.bean.PersonBean;
import java.util.List;
public interface PersonBeanService {
??? void insertPerson(PersonBean personBean);
??? void updatePerson(PersonBean personBean);
??? void deletePerson(int perid);
??? PersonBean selectPersonById(int perid);
??? List<PersonBean> selectPerson();
??? List<PersonBean> selectPersonByNameAge(PersonBean personBean);
}
PersonBeanServiceImpl
package com.weiwei.springmybatis.service;
import com.weiwei.springmybatis.bean.PersonBean;
import com.weiwei.springmybatis.bean.PersonBeanExample;
import com.weiwei.springmybatis.mapper.PersonBeanMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class PersonBeanServiceImpl implements PersonBeanService{
??? @Autowired
??? private PersonBeanMapper personBeanMapper;
??? @Override
??? public void insertPerson(PersonBean personBean) {
??????? personBeanMapper.insert(personBean);
??? }
??? @Override
??? public void updatePerson(PersonBean personBean) {
??????? personBeanMapper.updateByPrimaryKey(personBean);
??? }
??? @Override
??? public void deletePerson(int perid) {
??????? personBeanMapper.deleteByPrimaryKey(perid);
??? }
??? @Override
??? public PersonBean selectPersonById(int perid) {
??????? return personBeanMapper.selectByPrimaryKey(perid);
??? }
??? @Override
??? public List<PersonBean> selectPerson() {
??????? return personBeanMapper.selectByExample(null);
??? }
??? @Override
?? ?public List<PersonBean> selectPersonByNameAge(PersonBean personBean) {
??????? PersonBeanExample personBeanExample = new PersonBeanExample();
??????? PersonBeanExample.Criteria criteria = personBeanExample.createCriteria();
??????? if(personBean.getPersonName()!=null || personBean.getPersonName().length()>0) {
??????????? criteria.andPersonNameLike("%" + personBean.getPersonName() + "%");
??????? }
??????? if (personBean.getPersonAge()!=null){
??????????? criteria.andPersonAgeEqualTo(personBean.getPersonAge());
??????? }
??????? List<PersonBean> personBeanList = personBeanMapper.selectByExample(personBeanExample);
??????? return personBeanList;
??? }
}
PersonController
package com.weiwei.springmybatis.controller;
import com.weiwei.springmybatis.bean.PersonBean;
import com.weiwei.springmybatis.service.PersonBeanService;
import com.weiwei.springmybatis.service.PersonBeanServiceImpl;
import org.omg.CORBA.PUBLIC_MEMBER;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import java.util.List;
@Controller("percontrol")
public class PersonController {
??? @Autowired
??? private PersonBeanService personBeanService;
??? public Boolean addPerson(PersonBean personBean){
??????? boolean falg=false;
??????? try{
??????????? personBeanService.insertPerson(personBean);
??????????? falg=true;
??????? }catch (Exception e){
??????????? e.printStackTrace();
??????? }
??????? return falg;
??? }
??? public Boolean updatePerson(PersonBean personBean){
??????? boolean falg=false;
??????? try{
??????????? personBeanService.updatePerson(personBean);
??????????? falg=true;
??????? }catch (Exception e){
??????????? e.printStackTrace();
??????? }
??????? return? falg;
??? }
??? public Boolean deletePerson(int perid){
??????? boolean falg=false;
??????? try{
??????????? personBeanService.deletePerson(perid);
??????????? falg=true;
??????? }catch (Exception e){
??????????? e.printStackTrace();
??????? }
??????? return? falg;
??? }
???? public PersonBean selectPersonByid(int perid){
???????? PersonBean personBean=null;
???????? try{
????????????? personBean = personBeanService.selectPersonById(perid);
???? ????}catch (Exception e){
???????????? e.printStackTrace();
???????? }
??????? return? personBean;
???? }
??? public List<PersonBean> selectPerson(){
??????? return? personBeanService.selectPerson();
??? }
??? public List<PersonBean> selectPersonByAgeName(PersonBean personBean){
??????? return? personBeanService.selectPersonByNameAge(personBean);
??? }
}
Test
package com.weiwei.springmybatis.test;
import com.weiwei.springmybatis.bean.PersonBean;
import com.weiwei.springmybatis.controller.PersonController;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
public class Test {
??? public static void main(String[] args) {
??????? ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
??????? PersonController percontrol = applicationContext.getBean("percontrol", PersonController.class);
??????? /*添加
??? ????PersonBean personBean1 = new PersonBean();
??????? personBean1.setPersonName("孙悟空");
??????? personBean1.setPersonAge(1000);
??????? personBean1.setPersonAddress("花果山");
??????? percontrol.addPerson(personBean1);
??????? */
??????? /*修改
??????? PersonBean personBean2 = new PersonBean();
??????? personBean2.setPersonId(15);
??????? personBean2.setPersonName("唐僧");
??????? personBean2.setPersonAddress("东土大唐");
??????? personBean2.setPersonAge(200);
??????? percontrol.updatePerson(personBean2);
???????? */
??????? /*
??????? 根据id查询
??????? PersonBean personBean2 = new PersonBean();
??????? PersonBean personBean = percontrol.selectPersonByid(3);
??????? System.out.println("id====3,name===="+personBean.getPersonName()+"age====="+personBean.getPersonAge());
???????? */
??????? /*删除
??????? PersonBean personBean2 = new PersonBean();
??????? Boolean boo = percontrol.deletePerson(3);
??????? if (boo){
??????????? System.out.println("删除成功");
??????? }
???????? */
??????? //查询所有
??????? /*
??????? List<PersonBean> personBeanList = percontrol.selectPerson();
??????? for (PersonBean personBean:personBeanList) {
??????????? System.out.println(personBean.getPersonName());
??????? }
???????? */
??????? //按名字和年龄查询
??????? PersonBean personBean = new PersonBean();
??????? personBean.setPersonName("孙");
??????? personBean.setPersonAge(1000);
??????? List<PersonBean> personBeans = percontrol.selectPersonByAgeName(personBean);
??????? for (PersonBean personBean1:personBeans) {
??????????? System.out.println(personBean1.getPersonName()+personBean1.getPersonAge());
??????? }
??? }
}
|