JSP
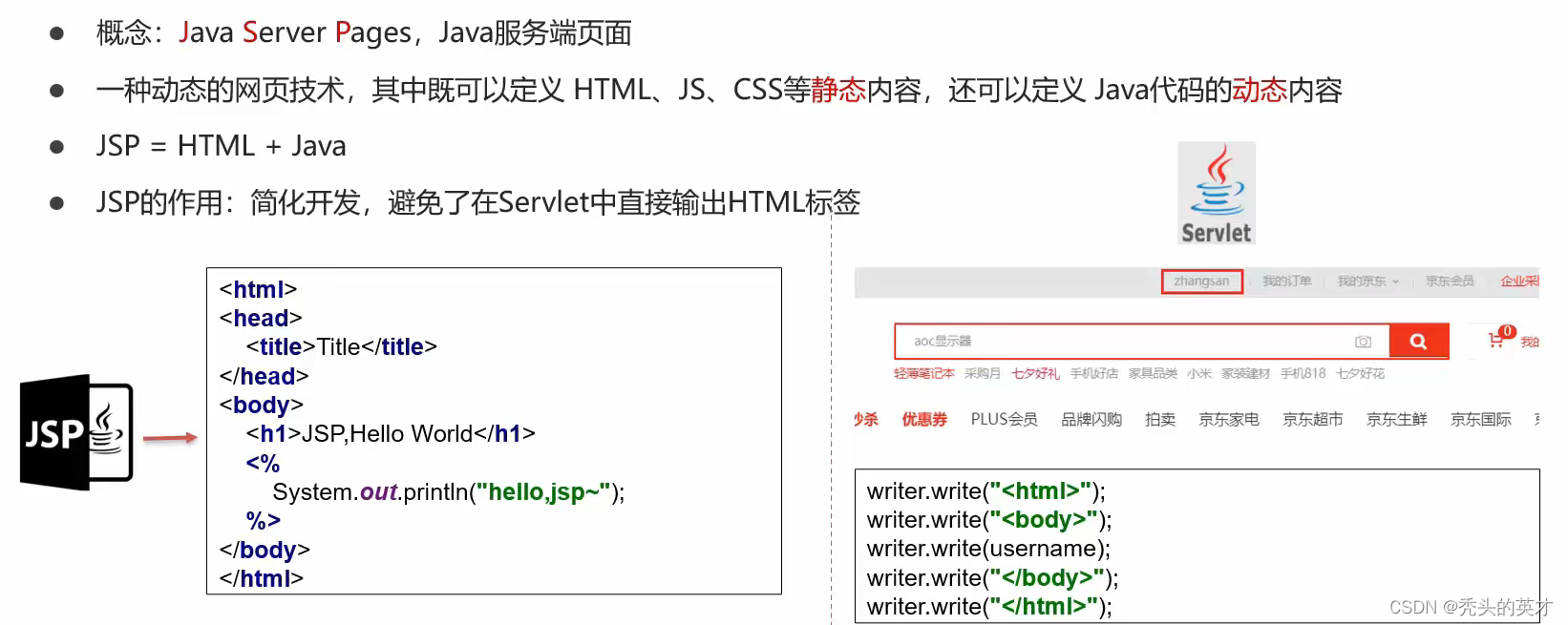
1、快速入门
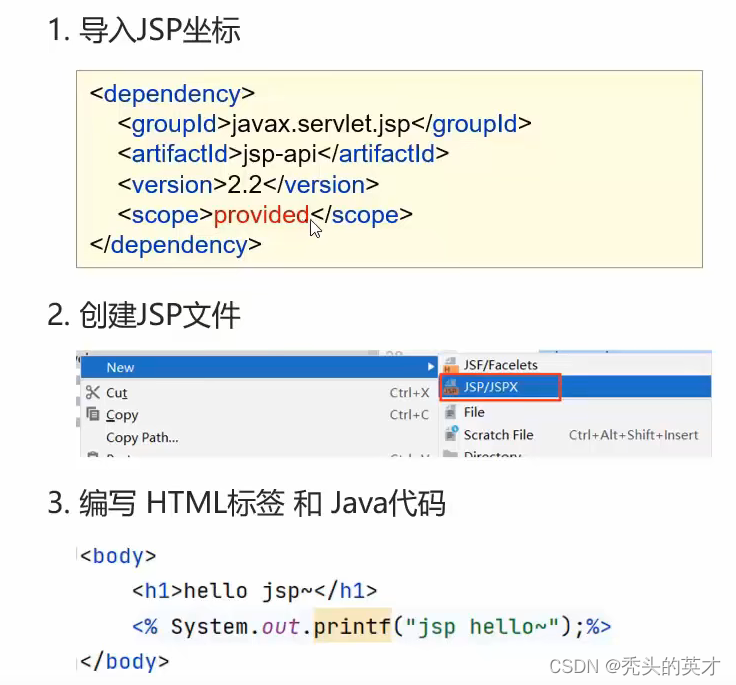
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
在webapp包下床创建文件hello.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Hello world</h1>
<%
System.out.println("hello jsp");
%>
</body>
</html>
JSP的原理
- JSP=HTML+Java 用于简化开发
- JSP本质上就是Servlet
- JSP在被访问时,由JSP容器(Tomcat)将其转换成java文件(Servlet),在由JSP容器(Tomcat)将其编译,最终对外提供服务的就是这个字节码文件
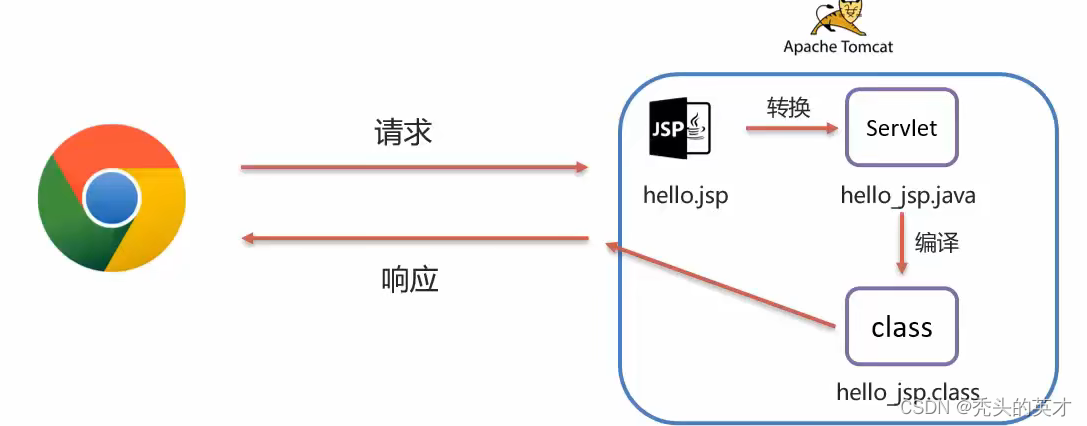
JSP的脚本
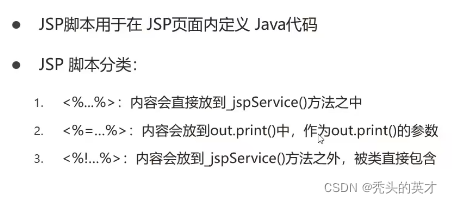
2、JSP的缺点
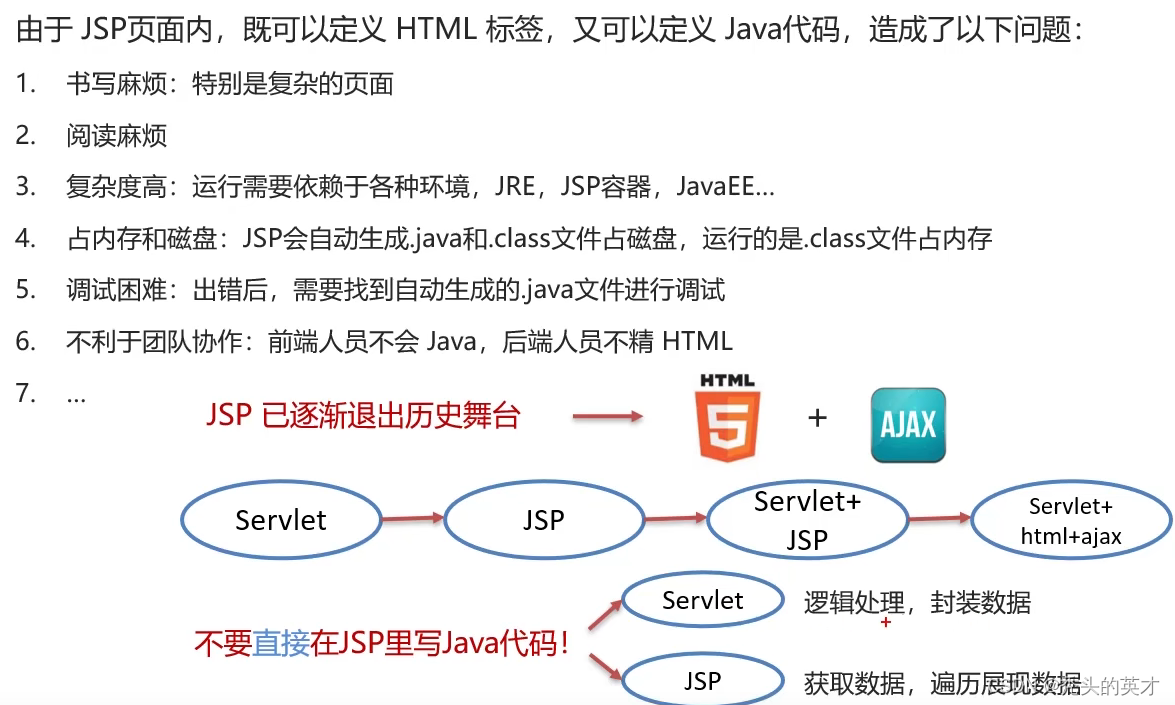
3、El表达式
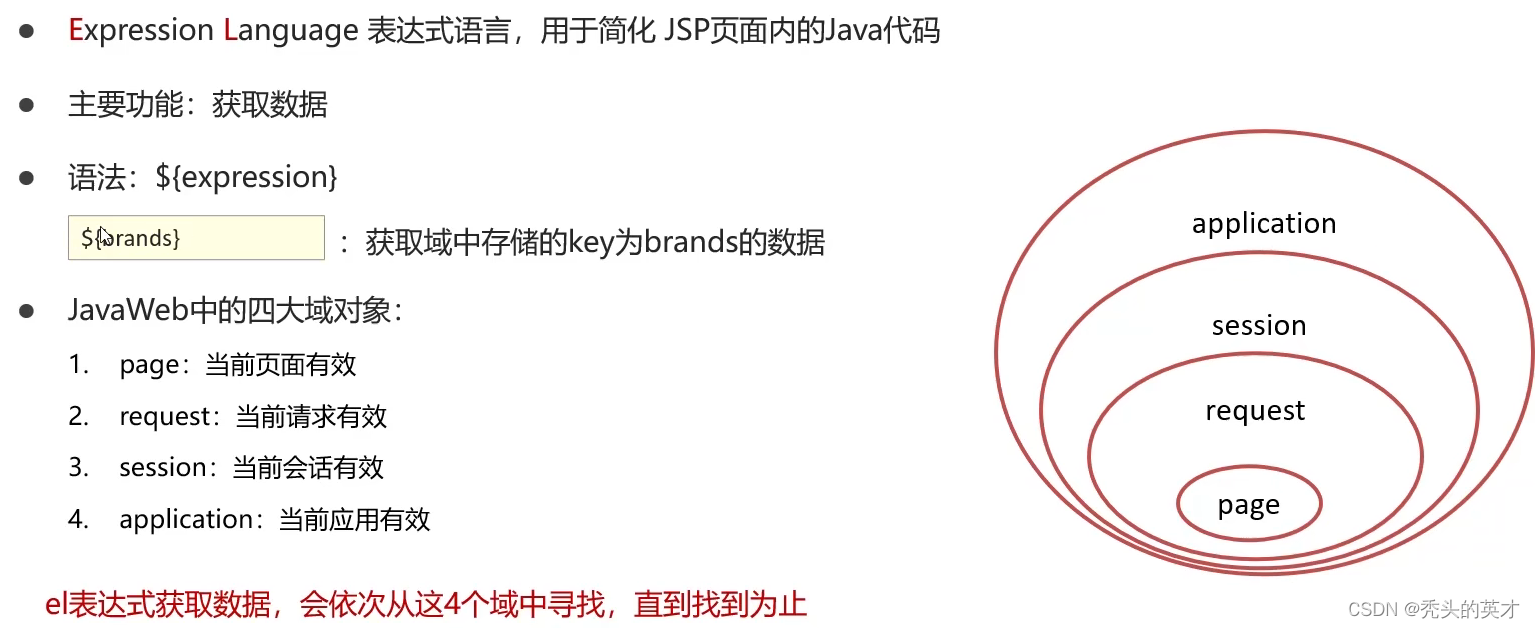
package com.web.web;
public class Brand {
private int id;
private String name;
public Brand(int i, String name) {
this.id=i;
this.name=name;
}
}
package com.web.web;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@WebServlet("/ser1")
public class ServletDemo1 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<Brand> brands=new ArrayList<Brand>();
brands.add(new Brand(1,"三只松鼠"));
brands.add(new Brand(2,"康师傅"));
brands.add(new Brand(3,"华为"));
req.setAttribute("brands",brands);
req.getRequestDispatcher("el-demo.jsp").forward(req,resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
super.doPost(req, resp);
}
}
jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
${brands}
</body>
</html>
4、JSTL标签
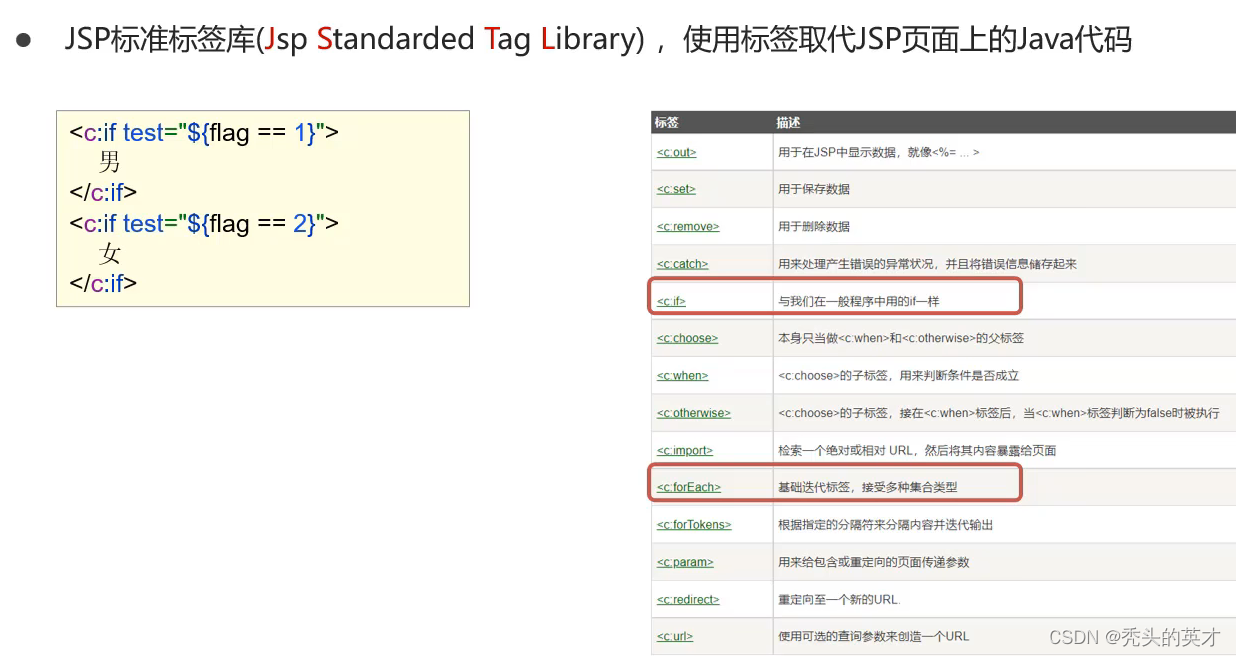 1、快速入门 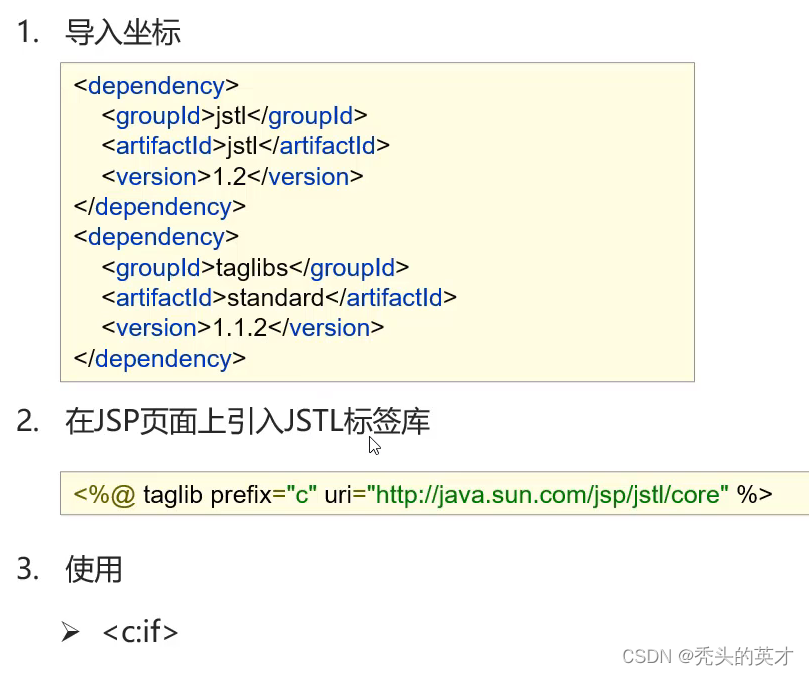
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--
c:if:来完成逻辑判断 替换java中的if else
--%>
<c:if test="true">
<h1>true</h1>
</c:if>--%>
<c:if test="false">
<h1>false</h1>
</c:if>--
</body>
</html>
用转发方式打开
package com.web.web;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@WebServlet("/ser1")
public class ServletDemo1 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<Brand> brands=new ArrayList<Brand>();
brands.add(new Brand(1,"三只松鼠"));
brands.add(new Brand(2,"康师傅"));
brands.add(new Brand(3,"华为"));
req.setAttribute("brands",brands);
req.setAttribute("status",1);
req.getRequestDispatcher("jstl-if.jsp").forward(req,resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
super.doPost(req, resp);
}
}
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--
c:if:来完成逻辑判断 替换java中的if else
--%>
<%-- <c:if test="true">--%>
<%-- <h1>true</h1>--%>
<%-- </c:if>--%>
<%-- <c:if test="false">--%>
<%-- <h1>false</h1>--%>
<%-- </c:if>--%>
<c:if test="${status==1}">
启用
</c:if>
<c:if test="${status==0}">
禁用
</c:if>
</body>
</html>
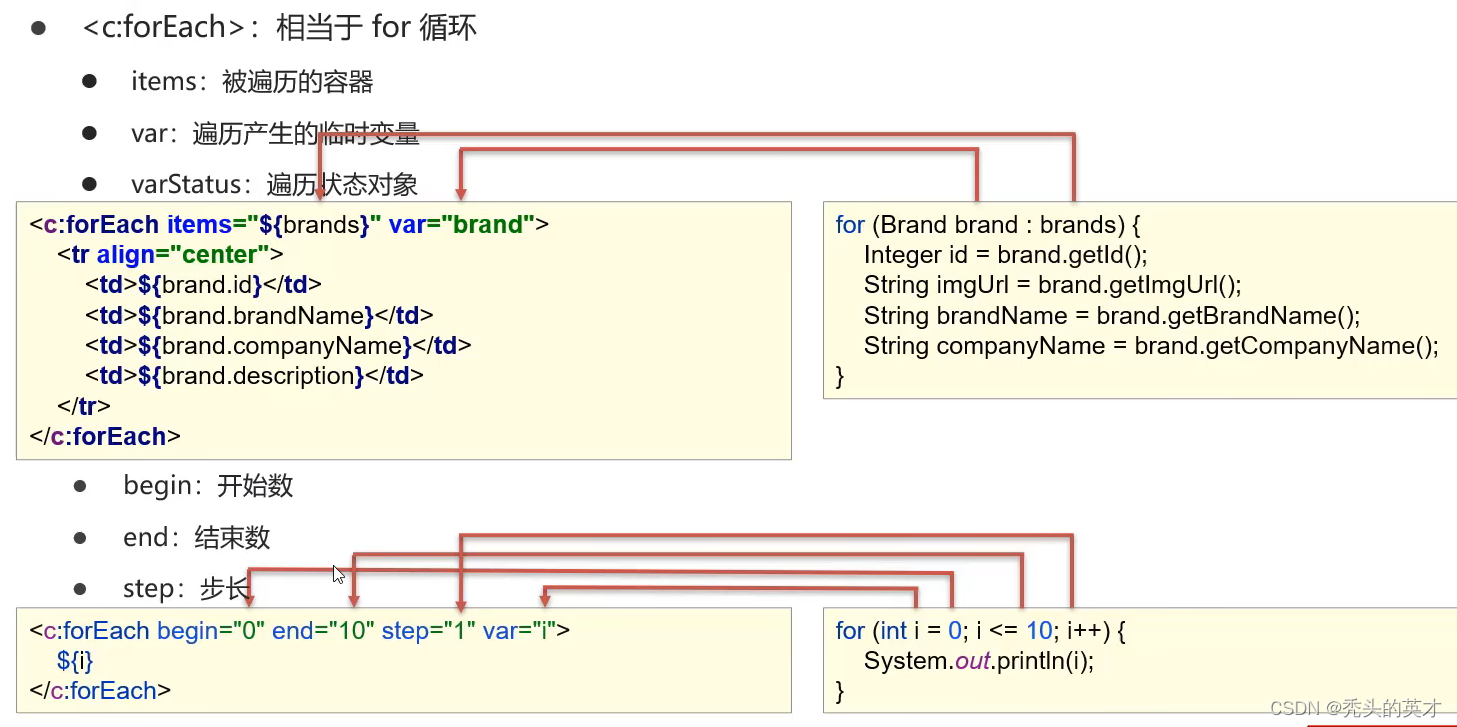
5、MVC模式
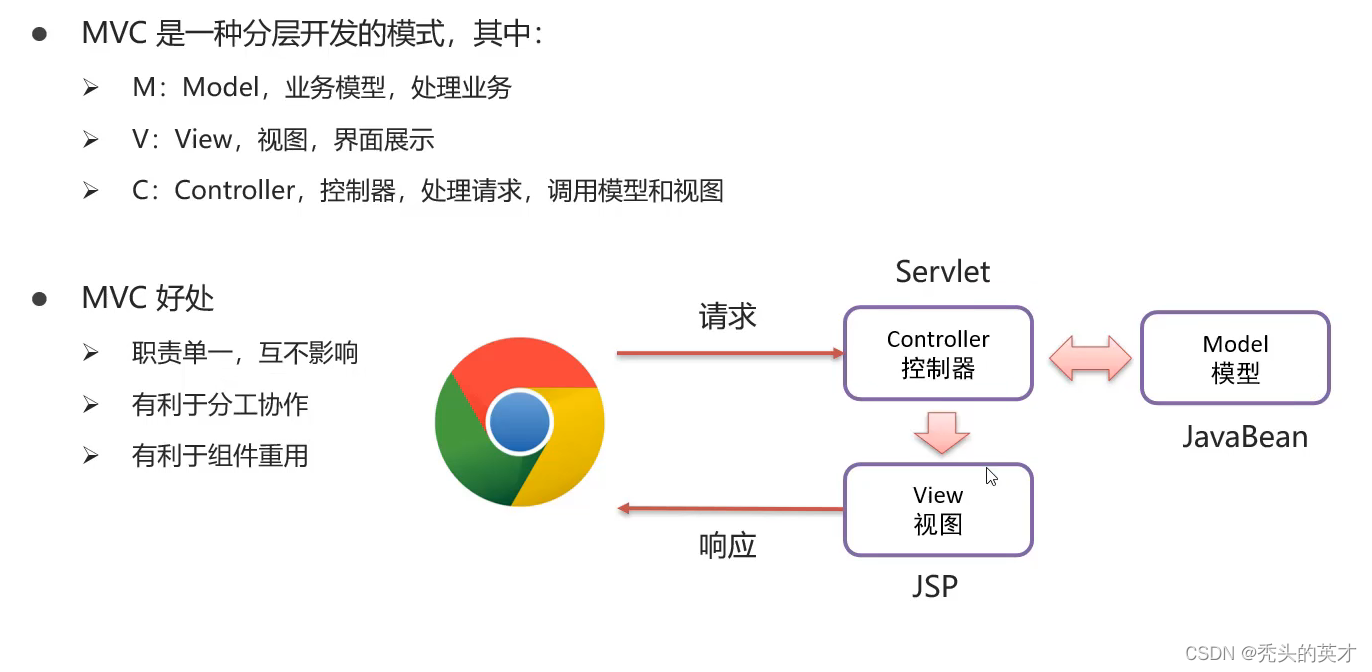 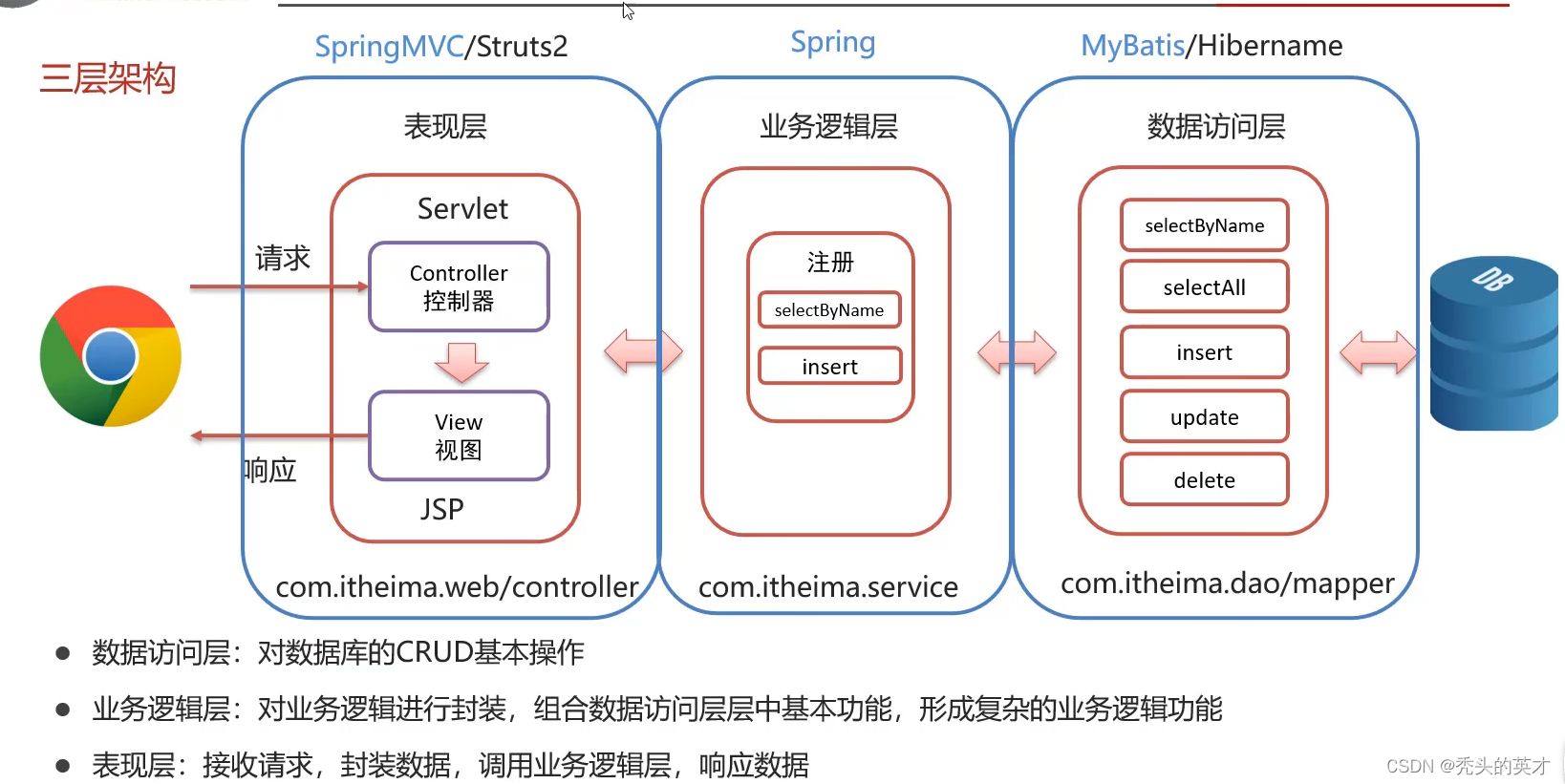
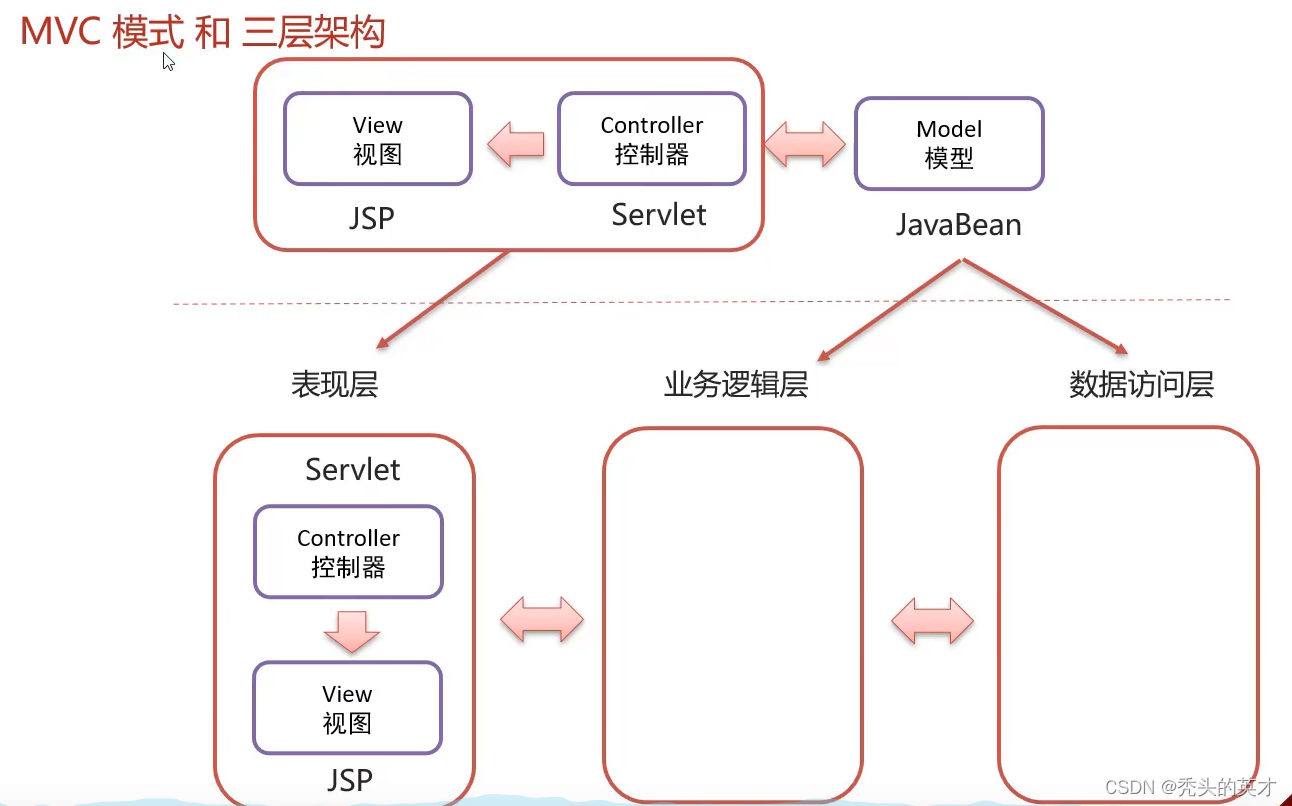
|