Hello,各位。这个专栏,我会帮助大家通过简单易懂的方式学习,学懂,学精Java这门编程语言!欢迎大家与我积极探讨Java以及其他的知识。
?wechat:Kepler-Antonia
?QQ:162196770
?gitee: https://gitee.com/jialebihaitao
?上一篇文章:https://blog.csdn.net/m0_53117341/article/details/123307420
?文章专栏:https://blog.csdn.net/m0_53117341/category_11551619.html
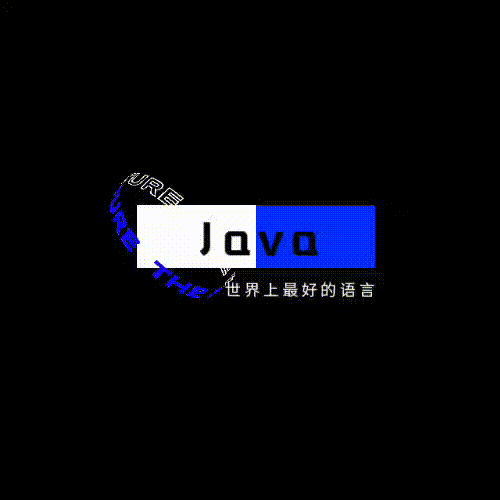
1、字面常量
1.1 常量的概念
常量即程序运行期间,固定不变的量称为常量
1.2 分类
- 字符串常量:
"12345" - 整形常量:
316 - 浮点数常量:
3.14 - 字符常量:
‘a’ - 布尔常量:
true/false - 空常量:
null (注意:小写的null)
2、数据类型
2.1 分类:
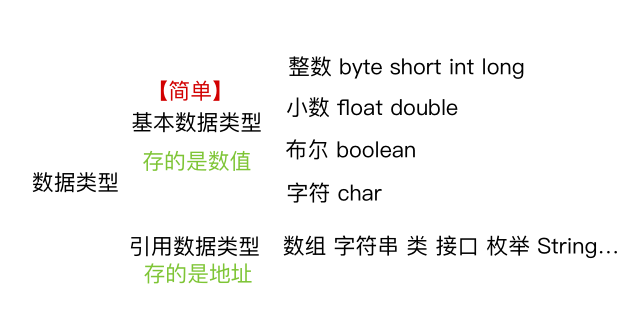
注意:string不是基本的数据类型
2.2 它们的大小
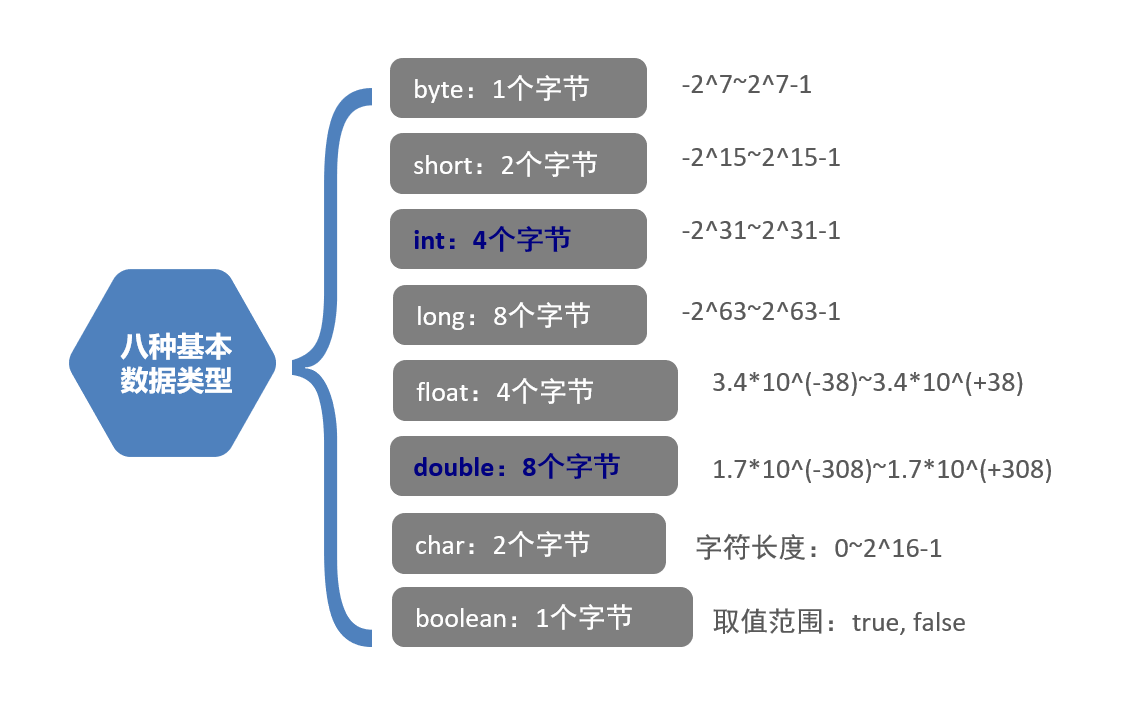
注意:
- 不论是在16位系统还是32位系统,int都占用4个字节,long都占8个字节
- 整形和浮点型都是带有符号的
- 整型默认为int型,浮点型默认为double
2.2.1 计算机存储数据的形式
计算机中最小的存储单元是字节(Byte,通常用B表示),每个字节包含8个位(bit,又叫“比特位”通常用b表示,值为0或1)。
- 1Byte = 8bit
- 1KB = 1024B
- 1MB = 1024KB
- 1GB = 1024MB
- 1TB = 1024GB
2.2.2局部变量和成员变量
- 局部变量:?法内部的变量
- 成员变量:我们在类和方法会讲到
举个栗子:
public class Demo1 {
public static void main(String[] args) {
System.out.println("欢迎你来看我的博客!");
}
}
3、变量
3.1 概念
在程序执行的过程中,其值可以在某个范围内发生改变的量。
3.2 本质
内存中的一小块区域
3.3 定义格式
数据类型 变量名 = 初始化值;
举个栗子:
public class Demo2 {
public static void main(String[] args) {
int a = 10;
double d = 3.14;
char ch = 'A';
boolean b = true;
System.out.println(a);
System.out.println(d);
System.out.println(ch);
System.out.println(b);
}
}
3.4 整型变量
3.4.1 整型
- 错误定义:
public class Demo3 {
public static void main(String[] args) {
int a;
System.out.println(a);
}
}
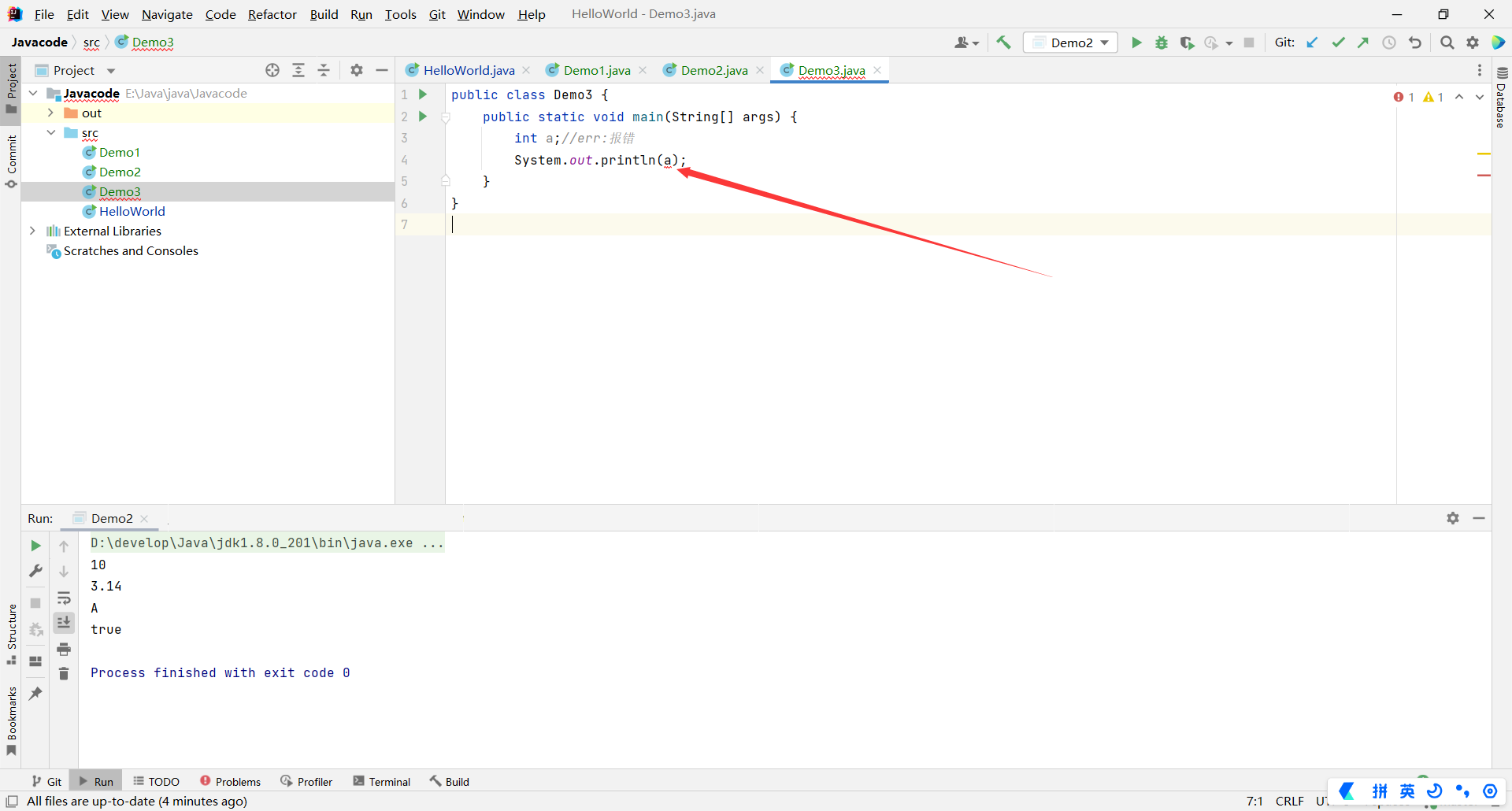
- 正确定义方法:
public class Demo3 {
public static void main(String[] args) {
int a = 10;
System.out.println(a);
int b;
b = 10;
System.out.println(b);
}
}
- 整型所能表达的范围:
public class Demo4 {
public static void main(String[] args) {
System.out.println(Integer.MAX_VALUE);//2147483647
System.out.println(Integer.MIN_VALUE);//-2147483648
}
}
//这里出现的概念:包装类
//基本数据类型 所对应的 类类型
//int->Integer[可以当成int的加强版本]
-
int的大小: 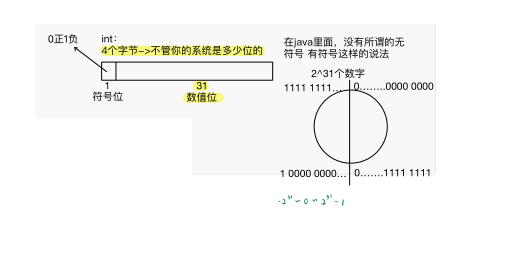
3.4.2 长整型
-
正确定义: public class longDemo {
public static void main(String[] args) {
long a = 10;
long b = 10L;
long c = 10l;
}
}
-
数据范围:-2^63~2^63-1 public class longDemo {
public static void main(String[] args) {
long a = 10;
long b = 10L;
long c = 10l;
System.out.println(Long.MAX_VALUE);
System.out.println(Long.MIN_VALUE);
}
}
3.4.3 短整型
-
正确定义 public class shortDemo {
public static void main(String[] args) {
short sh = 10;
System.out.println(sh);
}
}
-
数据范围 public class shortDemo {
public static void main(String[] args) {
System.out.println(Short.MAX_VALUE);
System.out.println(Short.MIN_VALUE);
}
}
-
利用包装类也可以创建变量(以后具体讲) public class shortDemo {
public static void main(String[] args) {
Short sh1 = 20;
System.out.println(sh1);
}
}
3.4.4 字节型(C语言未出现过的)
-
正确定义 public class byteDemo {
public static void main(String[] args) {
byte b1 = 124;
System.out.println(b1);
}
}
-
表示范围(重点):-128~+127 public class byteDemo {
public static void main(String[] args) {
System.out.println(Byte.MAX_VALUE);//127
System.out.println(Byte.MIN_VALUE);//-128
}
}
//包装类:Byte
那这样的话,我们就要格外的注意一下对于byte类型的变量的赋值了,不要超出byte的数据范围。 public class byteDemo {
public static void main(String[] args) {
byte b1 = 128;
System.out.println(b1);
}
}
为了方便理解,我们可以看这张图:
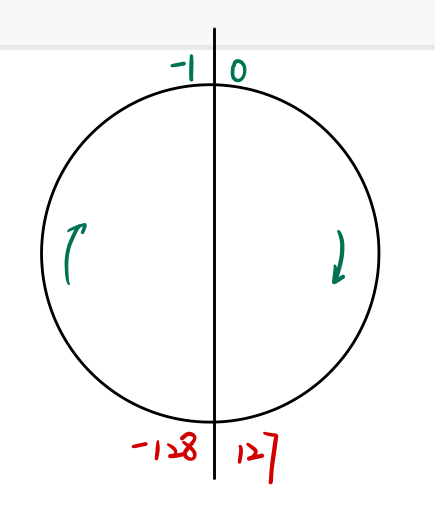
3.5 浮点型变量
3.5.1 双精度浮点型数据
-
正确使用: public class doubleDemo {
public static void main(String[] args) {
double d1=3.14;
System.out.println(d1);
}
}
-
注意事项:
-
会输出0.5吗? public class doubleDemo {
public static void main(String[] args) {
int a = 1;
int b = 2;
System.out.println(1 / 2);
}
}
答案是不会! 在Java中,int除以int的值依然是int(会把小数部分舍弃掉)。想要得到小数,需要使用double类型计算 public class doubleDemo {
public static void main(String[] args) {
double a = 1.0;
double b = 2.0;
System.out.println(a / b);
}
}
或者这样 public class doubleDemo {
public static void main(String[] args) {
int a = 1;
int b = 2;
System.out.println(a * 1.0 / b);
}
}
-
会输出1.21吗? public class doubleDemo {
public static void main(String[] args) {
double num = 1.1;
System.out.println(num * num);
}
}
我们看输出结果: 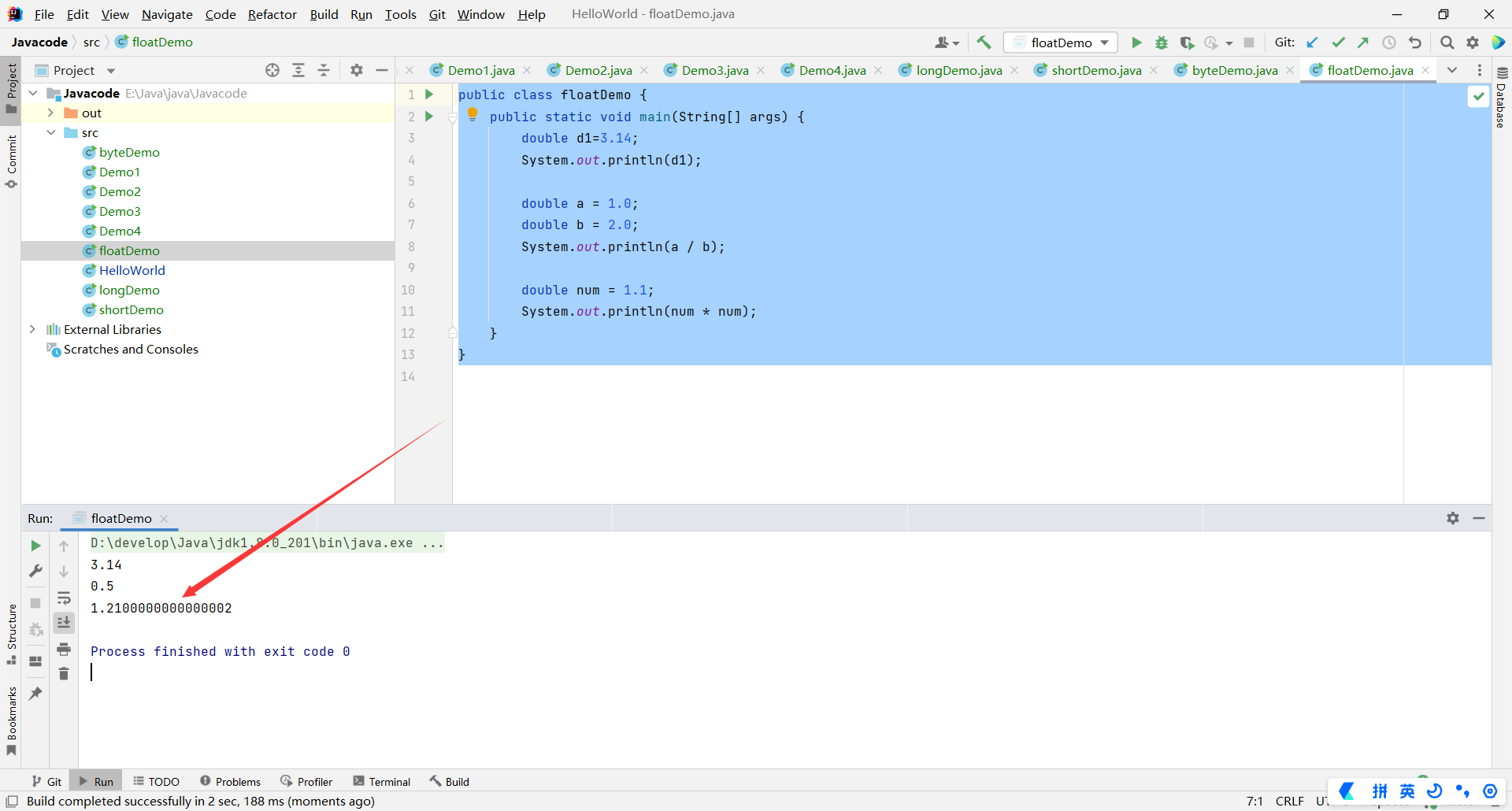 实际上,对于?数,是没有?个精确的数字,只能精确到?数点后?位。 -
表示范围: public class doubleDemo {
public static void main(String[] args) {
double d1=3.14;
System.out.println(d1);
double a = 1.0;
double b = 2.0;
System.out.println(a / b);
double num = 1.1;
System.out.println(num * num);
System.out.println(Double.MAX_VALUE);
System.out.println(Double.MIN_VALUE);
}
}
3.5.2 单精度浮点型数据
-
正确写法 public class floatDemo {
public static void main(String[] args) {
float f1 = 1.0f;
float f2 = 1.0F;
}
}
-
数据范围: public class floatDemo {
public static void main(String[] args) {
float f1 = 1.0f;
float f2 = 1.0F;
System.out.println(Float.MAX_VALUE);
System.out.println(Float.MIN_VALUE);
}
}
-
?oat 类型在 Java 中占四个字节, 同样遵守 IEEE 754 标准. 由于表示的数据精度范围较小, 一般在工程上用到浮点数都 优先考虑 double, 不太推荐使用 ?oat.
3.6 字符变量
-
正确使用: public class charDemo {
public static void main(String[] args) {
char ch = '帅';
System.out.println(ch);
}
}
-
注意事项:char数据类型不能存放负数 public class charDemo {
public static void main(String[] args) {
char ch1 = -100;
}
}
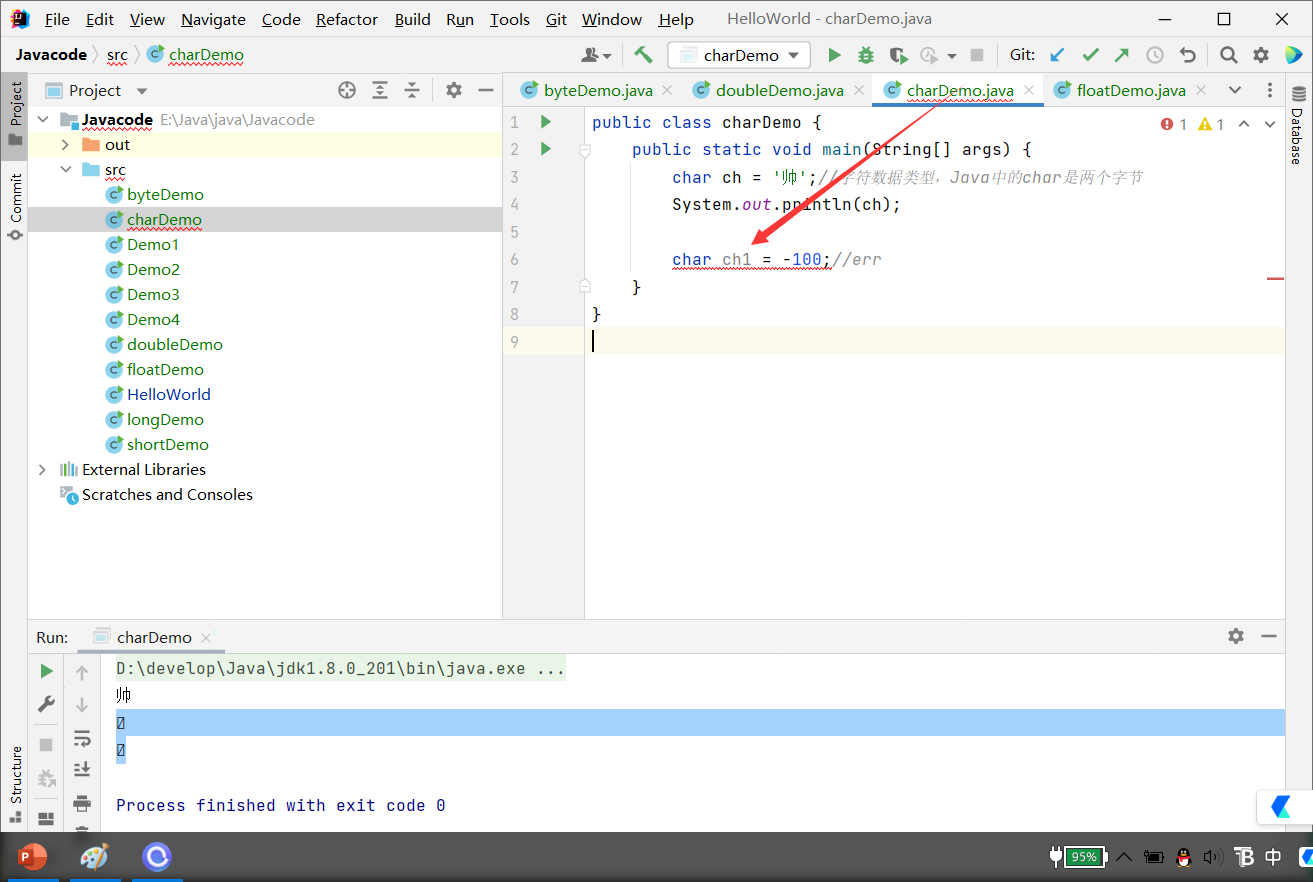
3.7 布尔型变量
-
使用:只有true和false两个取值 public class boolDemo {
public static void main(String[] args) {
boolean flg = true;
System.out.println(flg);
}
}
-
错误使用: public class boolDemo {
public static void main(String[] args) {
boolean flg1 = 1;
int a = 1;
if(a)
if(a!=0) {
System.out.println("正确写法");
}
}
}
-
boolean的包装类是Boolean -
boolean的大小:在JVM中,没有明确给定??(有些书上,1bit/1byte),但是说布尔数组会被JVM识别为字节数组,数组中每个布尔元素是8位->1个字节 在选择题中,所谓的?个boolean占1个字节/1个?特位
3.8 类型转换
在Java中,当参与运算数据类型不一致时,就会进行类型转换。Java中类型转换主要分为两类:自动类型转换( 隐式) 和强制类型转换(显式)。
3.8.1 自动类型转换
-
特点:数据范围小的转为数据范围大的时会自动进行。 -
举个栗子: public class typeConversion {
public static void main(String[] args) {
int a = 10;
long b = 10L;
a = b;
b = a;
}
}
3.8.2 强制类型转换(显式)
-
特点:数据范围大的到数据范围小的。 -
举个栗子: public class typeConversion {
public static void main(String[] args) {
int a = 10;
long b = 100L;
b = a;
a = (int)b;
}
}
3.9 数据提升
不同类型的数据之间相互运算时,数据类型小的会被提升到数据类型大的。
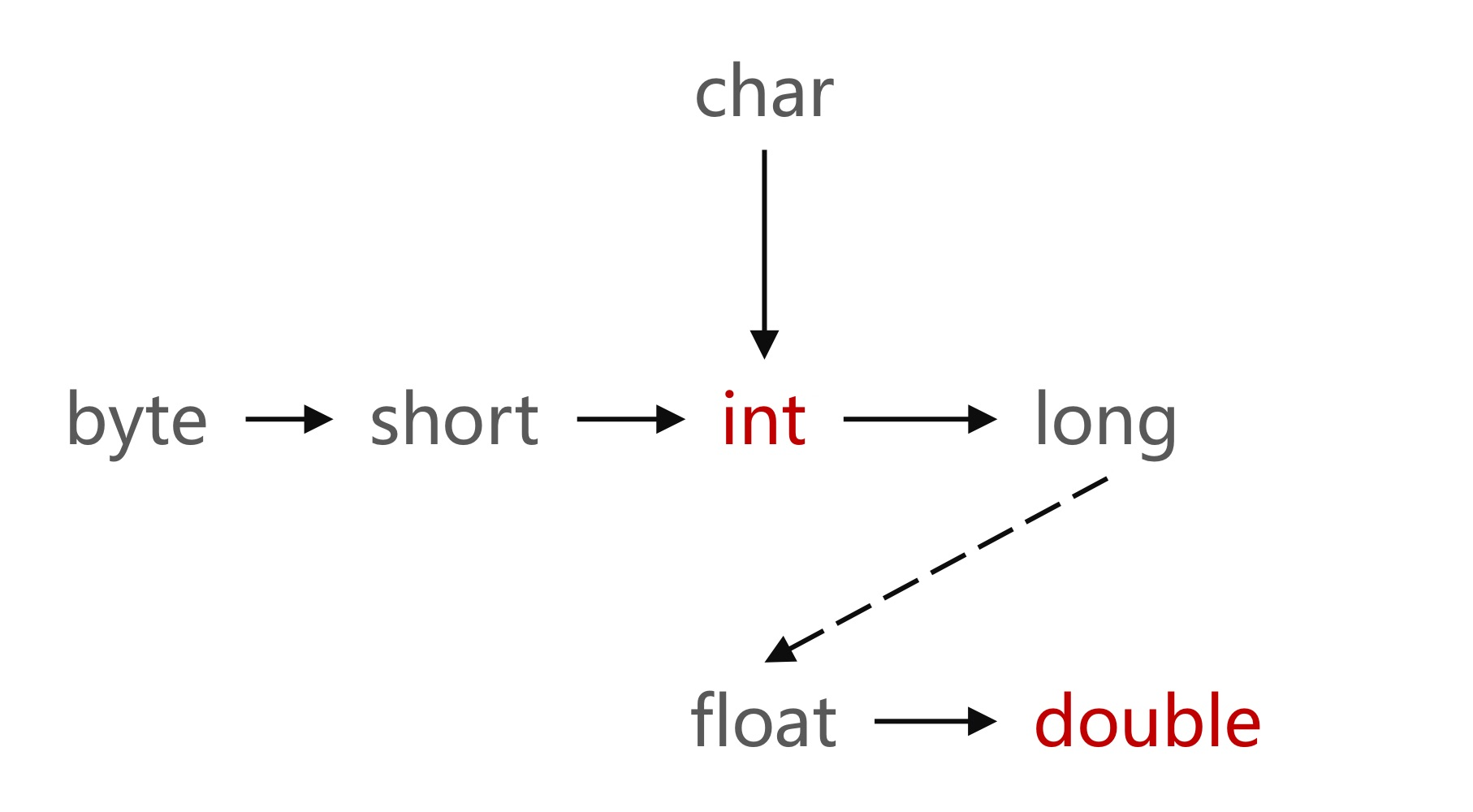
-
int->long: public class dataImprove {
public static void main(String[] args) {
int a = 10;
long b = 20;
int c = (int)(a+b);
long d = a + b;
}
}
public class dataImprove {
public static void main(String[] args) {
int a = 10;
long b = 10L;
a = (int)b;
}
}
-
byte<->byte: public class dataImprove {
public static void main(String[] args) {
byte b1 = 30;
byte b2 =20;
byte b3 = (byte)(b1 + b2);
}
}
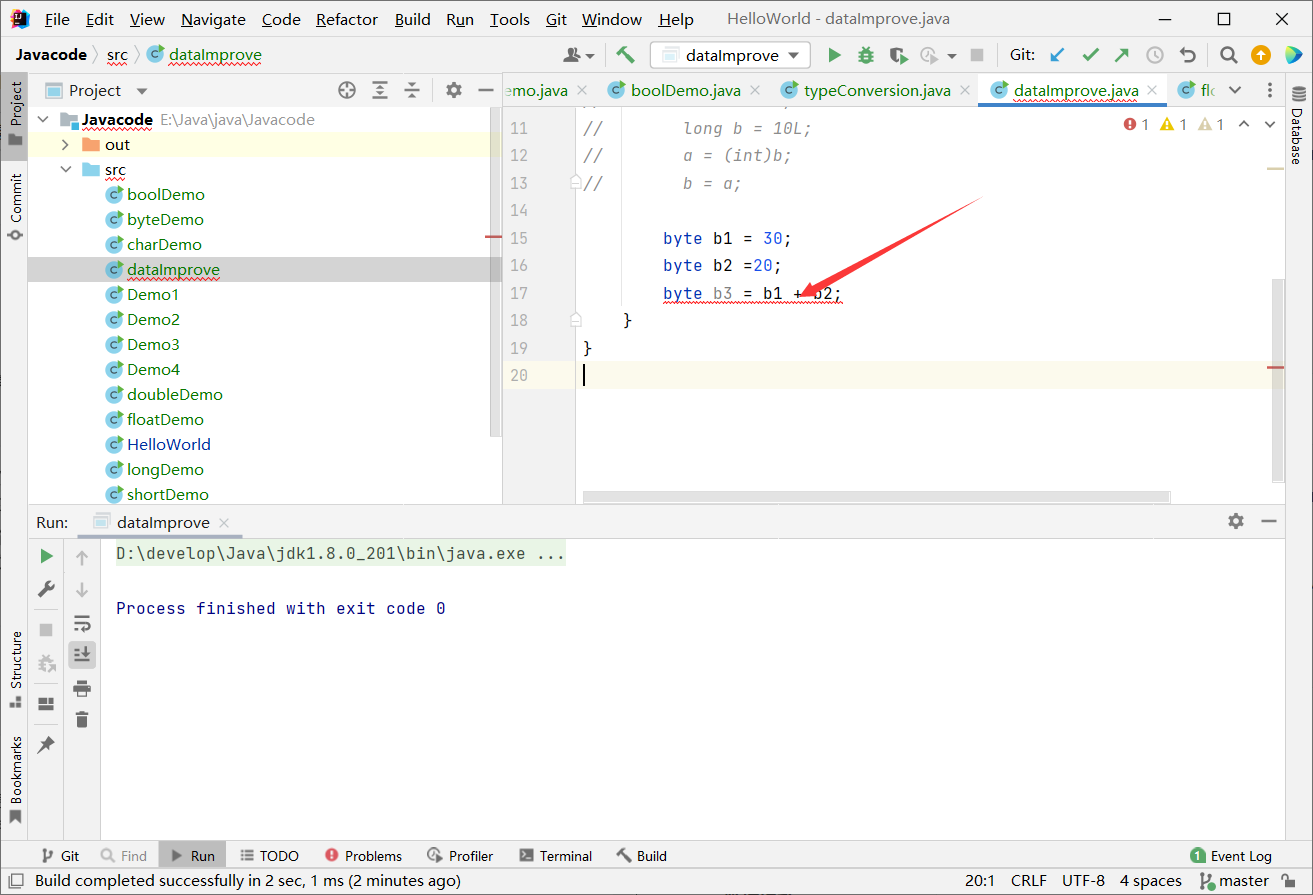
4.字符串类型
在Java中,是存在字符串这个类型的
举个栗子:
public class stringDemo {
public static void main(String[] args) {
String str1 = "您好";
String str2 = "欢迎来看我的博客";
System.out.println(str1+str2);
}
}
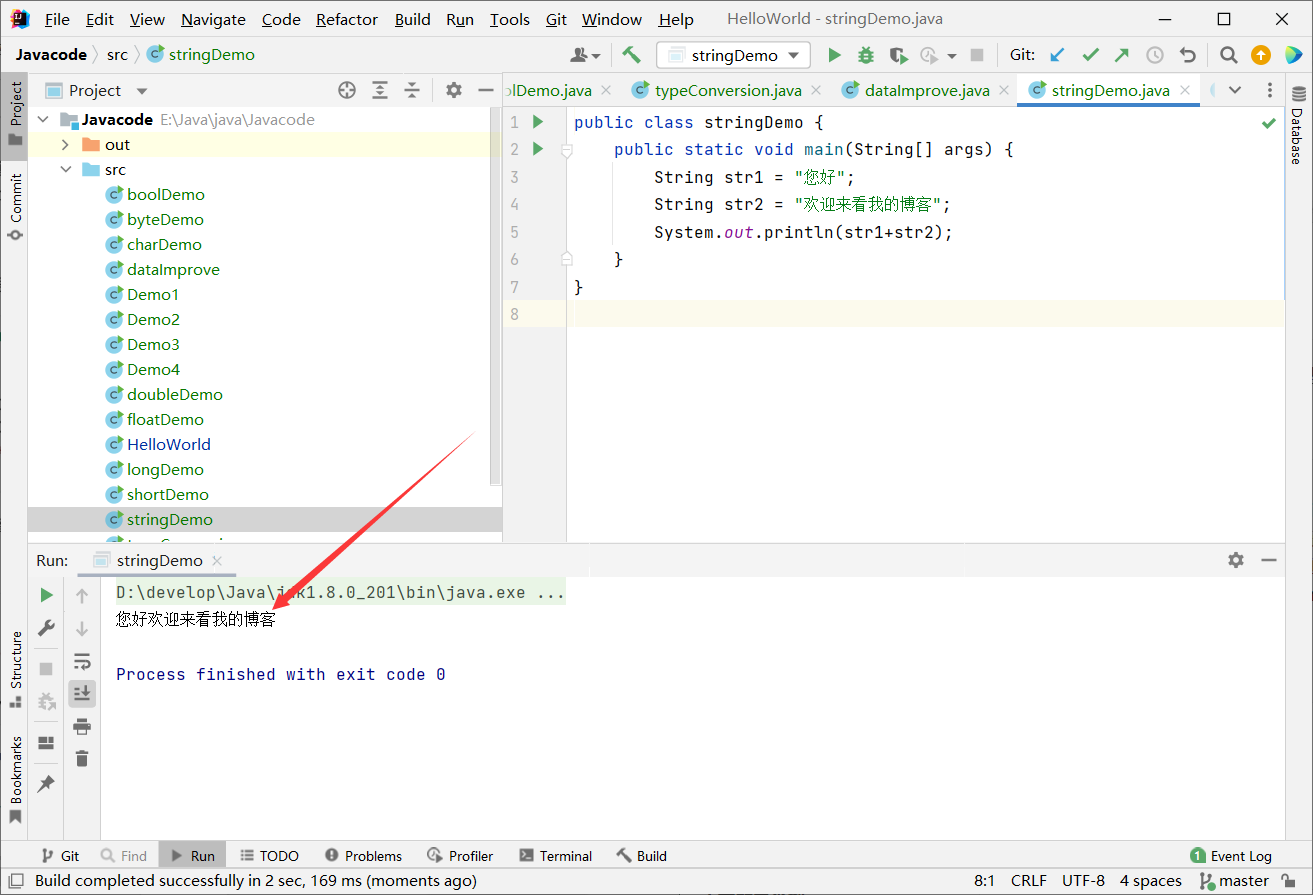
再举个栗子:
public class stringDemo {
public static void main(String[] args) {
int a = 10;
int b = 20;
System.out.println("a="+a+"b="+b);
System.out.println("a+b="+a+b);
System.out.println("a+b="+(a+b));
System.out.println(a+b+"<-a+b");
}
}
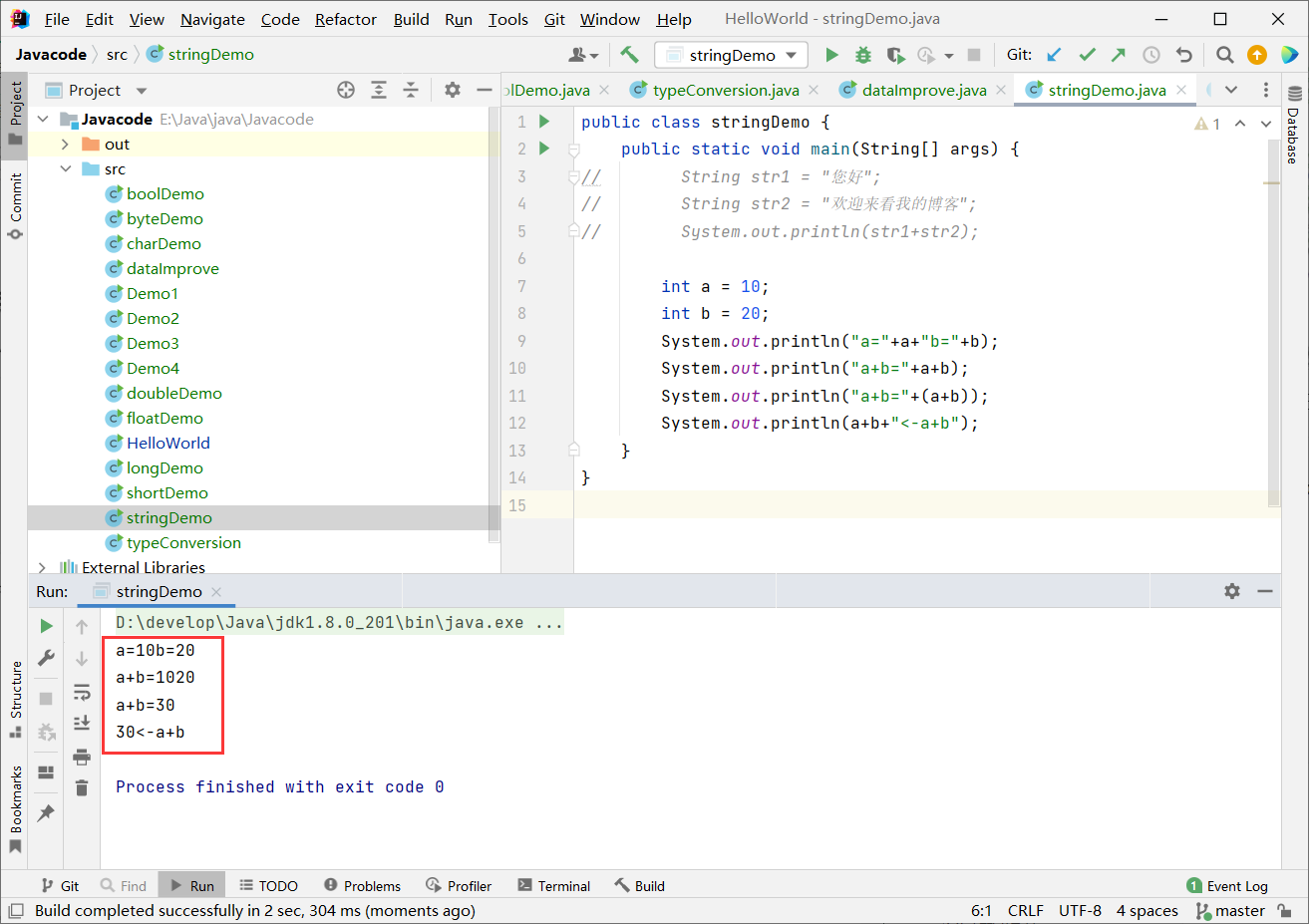
解释一下:只要是跟字符串拼接,结果就是字符串,自上至下,自左至右
那再再举个栗子:
public class stringDemo {
public static void main(String[] args) {
//字符串的加法运算
System.out.println("hello" + "world"); //"helloworld"
System.out.println("hello" + 10); //"hello10"
System.out.println("hello" + 10 + 20); //"hello10" + 20 --> "hello1020"
System.out.println(10 + 20 + "hello"); //30 + "hello" --> "30hello"
}
}
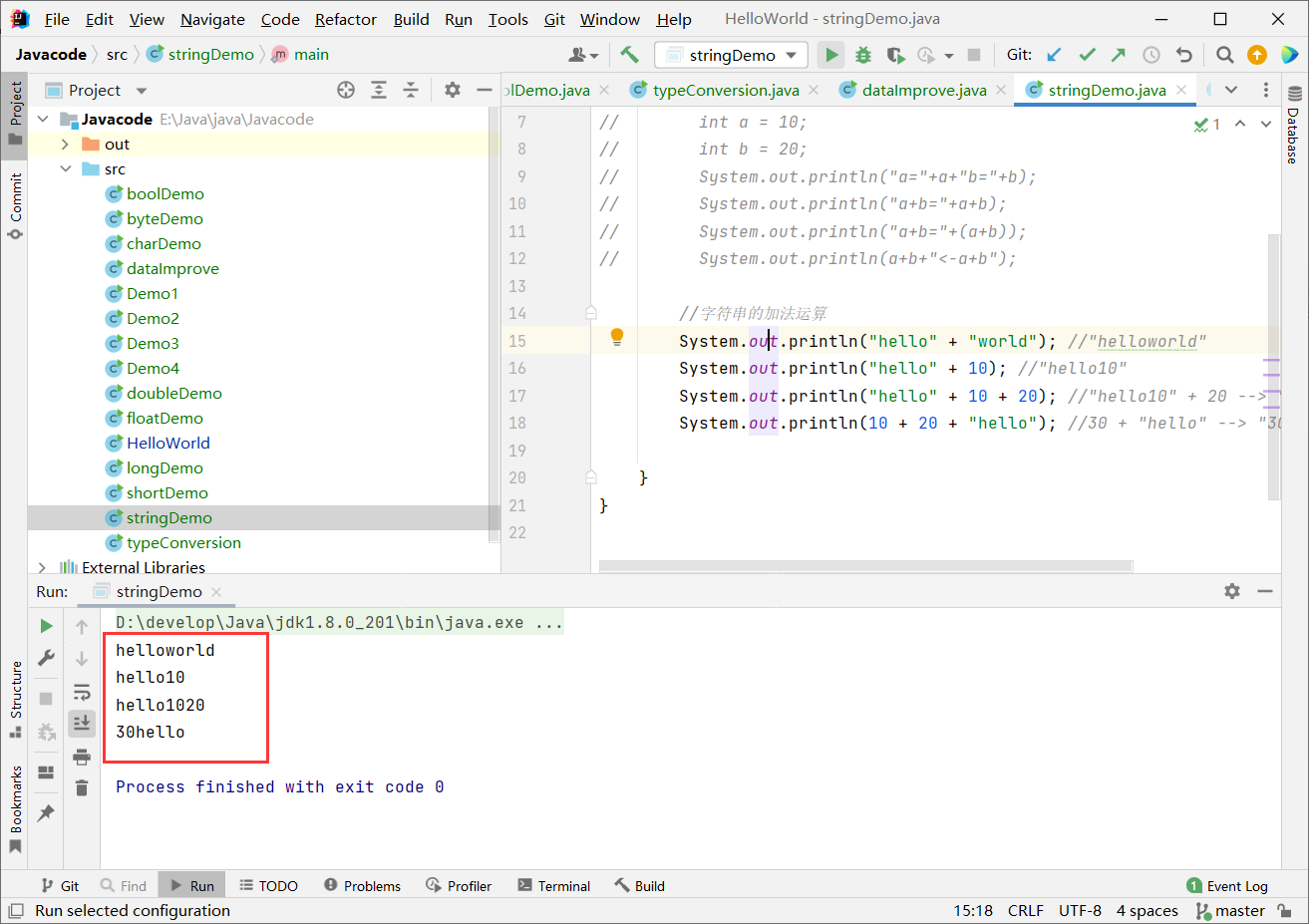
-
整数变字符串 public class stringExchange {
public static void main(String[] args) {
int num = 10;
//方法一
String str1 = num + "";
System.out.println(str1);
//方法二
String str2 = String.valueOf(num);
System.out.println(str2);
}
}
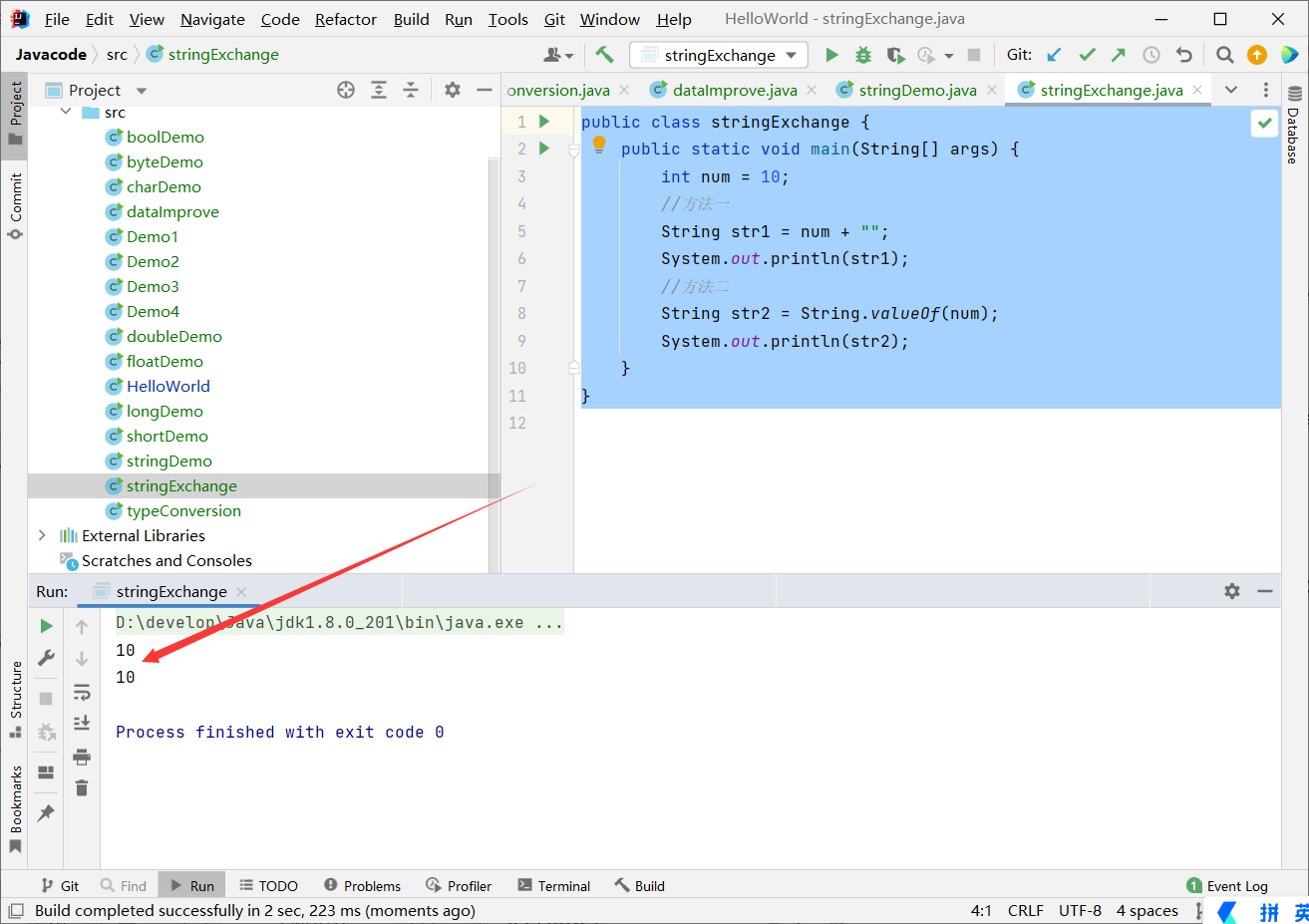 -
字符串变整数 public class stringExchange {
public static void main(String[] args) {
String str1 = "123";
int a = Integer.valueOf(str1);
System.out.println(str1);
int b = Integer.parseInt(str1);
System.out.println(b);
}
}
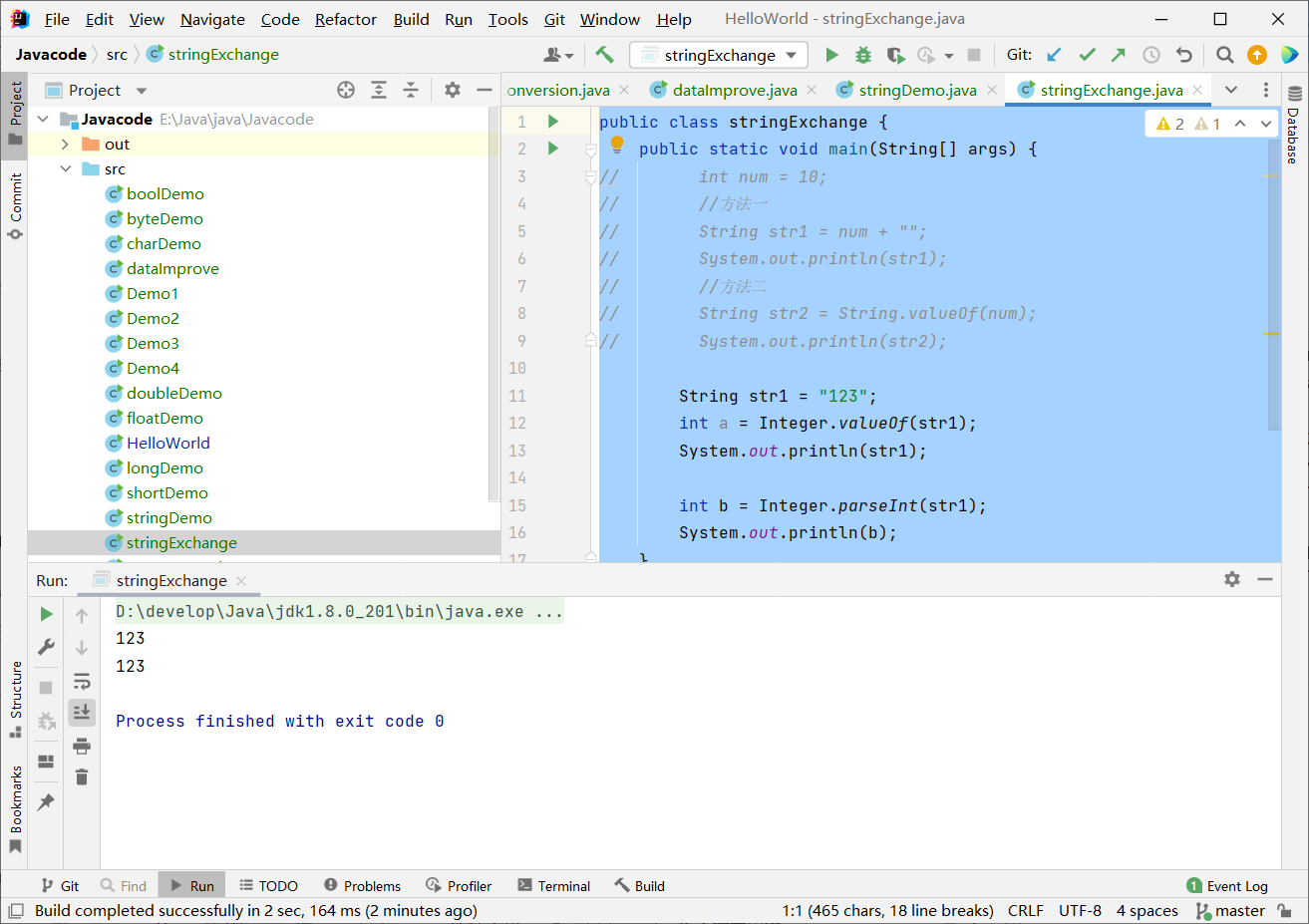
这两个知识点先了解即可,以后会详细讲解的
至此,关于数据类型与变量的相关知识点已介绍完毕。喜欢的话,一键三联熬~
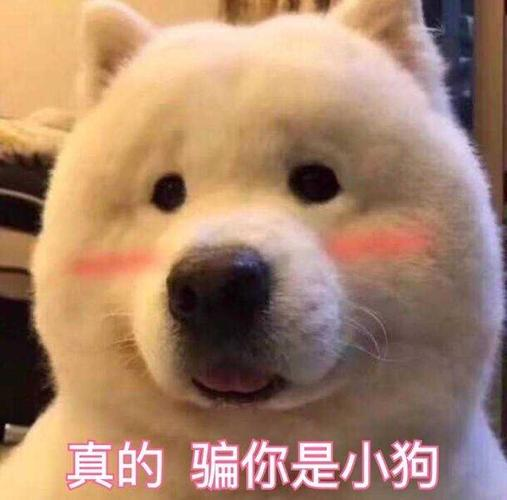
|