异常Exception
引出异常
运行下面的代码,看看有什么问题 —》引出异常和异常处理机制Exception01.java
public static void main(String[] args){
int num1 = 10;
int num2 = 0;
int result = num1 / num2;
System.out.println("程序继续运行...");
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-wXbhdQaN-1646999494450)(E:\Typora笔记\java笔记\img\image-20220203123354131.png)]](https://img-blog.csdnimg.cn/71d78eccac1640f6a07adb908d8068fd.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
异常介绍
基本概念:
JAVA 语言中,将程序执行中发生的不正常的情况称为“异常”。(开发过程中的语法错误和逻辑错误不是异常)
执行过程中所发生的异常事件可分为两大类:
1.Error (错误):java 虚拟机无法解决的严重问题。如:jvm 系统内部错误,资源耗尽等严重情况,比如:StackOverflowError 【栈溢出】和内存不足OOM (out of memory ),Error 是严重错误,程序会崩溃
2.Exception :其它因编程错误或偶然的外在因素导致的一般性问题,可以使用针对性的代码进行处理。例如空指针访问,试图读取不存在的文件,网络连接中继等,Excption 分为两大类:运行时异常【程序运行时,发生的异常】和编译异常【编程时,编译器检查出的异常】
异常体系图【重点】
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-htDCPfUm-1646999494451)(E:\Typora笔记\java笔记\img\image-20220203174701457.png)]](https://img-blog.csdnimg.cn/0eb6dfbaa3844122b8291629cf65a797.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
异常体系图的小结:【必须记住】
- 异常分为两大类,运行时异常和编译时异常
- 运行时异常,编译器检查不出来。一般是指编程时的逻辑错误,是程序员应该避免其出现的异常。
java.lang.RuntimeException 类及它的子类都是运行时异常 - 对于运行时异常,可以不作处理,因为这类异常很普通,若全处理可以会对程序的可读性和运行效率产生影响
- 编译时异常,是编译器要求必须处理的异常
常见的运行时异常
NullPointerException :空指针异常ArithmeicException :数学运算异常ArrayIndexOutOfBoundsException :数组下标越界异常ClassCastException :类型转换异常NumberFormatException :数字格式不正确异常
空指针异常NullPointException
当应用程序试图在需要对象的地方使用null 时,抛出该异常,看案例演示:
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-yVRdtQXh-1646999494451)(E:\Typora笔记\java笔记\img\image-20220203181224821.png)]](https://img-blog.csdnimg.cn/ebc855db35e14d95b8c328e938e916d7.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
package exception;
public class NullPointException_ {
public static void main(String[] args) {
String name = "海康";
System.out.println(name);
String resume = null;
System.out.println(resume.length());
}
}
ArithmeticException 数学运算异常
当出现异常的运算条件时,抛出此异常。例如,一个整数"除以零"时,抛出此类的一个实例,案例演示当出现异常的运算条件时,抛出异常。
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-hO8DLM8i-1646999494452)(E:\Typora笔记\java笔记\img\image-20220203181956303.png)]) 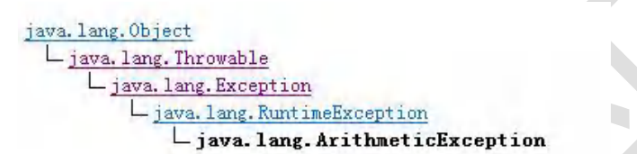
ArrarIndexOutOfBoundException 数组下标越界异常
数组下标越界异常,用非法索引访问数组的异常。如果索引为负或大于等于数组大小,则该索引为非法索引
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-hPgTV7yv-1646999494453)(E:\Typora笔记\java笔记\img\image-20220203185251092.png)]](https://img-blog.csdnimg.cn/853d3f68a63649929f1c018c38ee23f7.png)
ClassCastException 类型转换异常
当试图将对象强制转换为不是实例的子类时,抛出该异常。如下,以下代码将生成一个ClassCastException.java
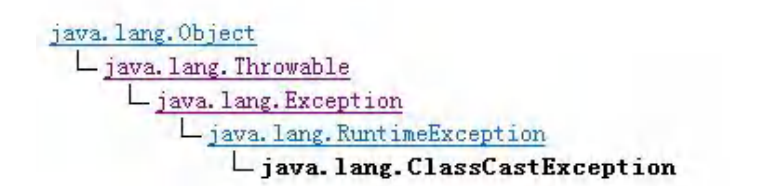
package exception;
public class ClassCastException {
public static void main(String[] args) {
Animal animal = new Cat();
Dog dog = (Dog) animal;
}
}
class Animal {}
class Cat extends Animal{}
class Dog extends Animal{}
NumberFormatException 数字格式不正确异常
当应用程序试图将字符串转换成一种数值类型,但该字符串不能转换为适当格式时,抛出该异常 —》抛出该异常 —》使用异常我们可以确保输入是满足条件数字
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-0T0bLedD-1646999494454)(E:\Typora笔记\java笔记\img\image-20220204112602504.png)]](https://img-blog.csdnimg.cn/c402ec08fe004dd4a85e6ef4d08e0eaf.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
package exception;
public class NumberFormatException {
public static void main(String[] args) {
String name = "光良";
int num = Integer.getInteger(name);
System.out.println(num);
}
}
编译异常
介绍:编译异常是指在编译期间,就必须处理的异常,否则代码不能通过编译
常见的编译异常:
SQLException :操作数据库时,查询表可能发生异常
IOException :操作文件时,发生的异常
FileNotFoundException :当操作一个不存在的文件时,发生异常
ClassNotFoundException :加载类,而该类不存在时,异常
EOFException :操作文件,到文件未尾,发生异常
ILLegaIArguementException :参数异常
练习题目:
1.
String firends[] = {"tom","jack","milan"};
for(int i=0;i<4;i++){
System.out.println(firends[i]);
}
2.
Cat c = new Cat();
cat = null;
System.out.println(cat.name);
3.
public calss A{
int x;
public static void main(String[] args){
int y;
A a = new A();
y=3/a.x;
System.out.println("program ends ok!");
}
}
4.
class Person{
public static void main(String[] args){
Object obj = new Date();
Person person;
person = (Person) obj;
System.out.println("person");
}
}
异常处理[必须掌握]
异常处理就是当异常发生时,对异常处理的方式
异常处理的方式有两种:
1.try-catch-finally
程序员在代码中捕获发生的异常,自行处理
2.throws
将发生异常抛出,交给调用者(方法)处理,最顶级的处理者就是JVM ,JVM 的处理机制是将异常直接打印,退出异常
try-catch-finally 处理机制
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-WfuvBzl5-1646999494454)(E:\Typora笔记\java笔记\img\image-20220204122431663.png)]](https://img-blog.csdnimg.cn/8c69ecdcd5cb472ead779c4ca5b1ac0b.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
throws处理机制
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-EaioaDsB-1646999494455)(E:\Typora笔记\java笔记\img\image-20220204122524156.png)]](https://img-blog.csdnimg.cn/39f33c8859e240dfa2fa5d379b3a50d7.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
try-catch异常
try-catch方式处理异常说明
1.java提供try和catch块来处理异常,try块用于包含可能出错的代码。catch块用于处理try块中发生的异常。可以根据需要在程序中有多个try...catch块。
2.基本语法
try{
}catch(异常){
}
try-catch 方式处理异常-快速入门
package exception;
public class Exception01 {
public static void main(String[] args) {
int num1 = 10;
int num2 = 0;
try {
int result = num1 / num2;
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("程序继承运行......");
}
}
try-catch 方式处理异常-注意事项
1.如果异常发生了,则异常发生后面的代码不会执行,直接进入到catch 块
2.如果异常没有发生,则顺序执行try 的代码块,不会进入到catch 块
3.如果希望不管是否发生异常,都执行某段代码(比如关闭连接,释放资源等),则使用到finally
4.可以有多个catch 语句,捕获不同的异常(进行不同的业务处理),要求父类异常在后,子类异常在前,比如(Exception 在后,NullPointerException 在前),如果发生异常,只会匹配一个catch
5.可以进行try-finally 配合使用,这种用法相当于没有捕获异常,因此程序会直接崩掉(退出)。应用场景,就是执行一段代码,不管是否发生异常,都必须执行某个业务逻辑【就是如果没有异常正常执行,并执行finally中的代码,如果有异常就是先执行finally中的代码,再抛出异常信息】
package exception.tryCatchDetail;
import exception.NullPointException_;
public class TryCatchDetail01 {
public static void main(String[] args) {
try {
String str = "海康";
int a = Integer.getInteger(str);
System.out.println("数字:"+a);
Person person = new Person("湛江");
person = null;
System.out.println(person.getName());
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (NullPointerException exception){
System.out.println("空指针异常");
exception.printStackTrace();
}catch (Exception e1){
System.out.println("异常");
e1.printStackTrace();
}finally {
System.out.println("finally就是一个干活的");
}
}
}
class Person{
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Person(String name) {
this.name = name;
}
}
package exception.tryCatchDetail;
public class TryCatchDetail02 {
public static void main(String[] args) {
try {
int num1 = 10;
int num2 = 0;
int result = num1 / num2;
System.out.println(result);
} finally {
System.out.println("奴才命啊");
}
}
}
练习题目
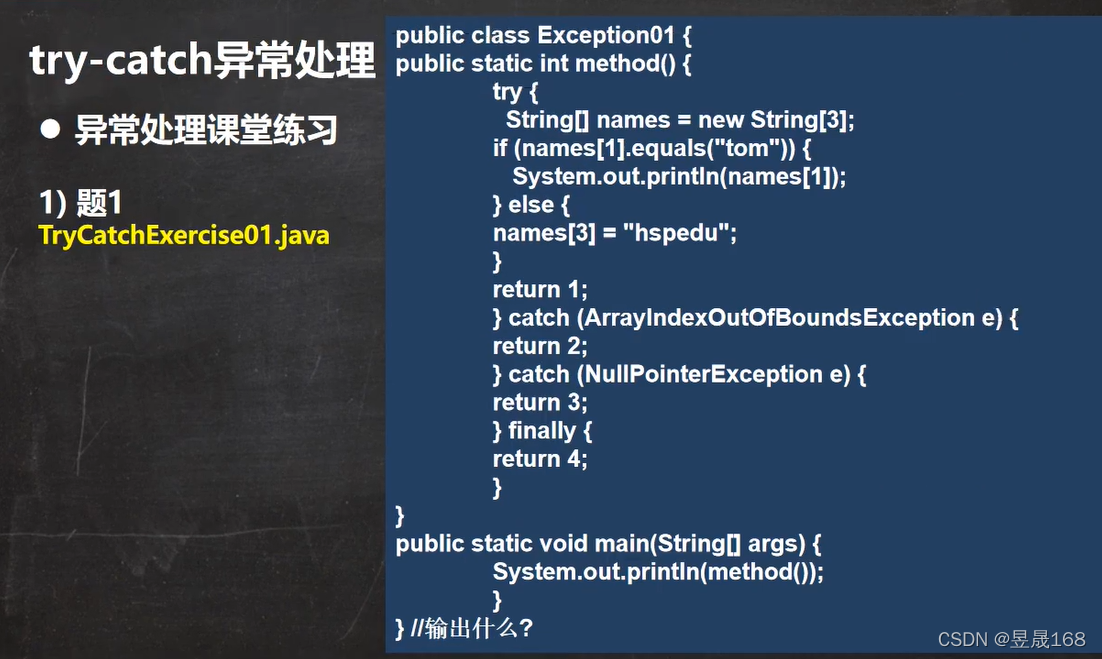
由于return 语句执行就是倒致整个方法退出栈【所以:catch中NullPointerException 只会捕获到异常,并没有返回3,由于finally 是必须要执行的所以在finally 中返回4,整个方法结束】
输出:4
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-YRqWEj9Q-1646999494456)(E:\Typora笔记\java笔记\img\image-20220204181838610.png)]](https://img-blog.csdnimg.cn/373459f525c240609110a84323e0ec3a.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
输出:4
由于空指针异常到了NullPointerException 中,但return 语句没有执行,++i 还是要执行可以此时变成了3,最后执行finally 变成了4
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-n4HqBz63-1646999494456)(E:\Typora笔记\java笔记\img\image-20220205220701389.png)]](https://img-blog.csdnimg.cn/817ca9a01faf4ad8b7f4c6694530769c.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
输出:i=4,3
由于空指针异常直接跳到了NullPointerException 中执行return 并没有直接返回而是先执行到++i ,i 变成了3,并用一个临时变量保存这个3,再到finally中执行的代码,++i 后变成了4,并输出了4,最后要跳到NullPointerException 中执行最后return 语句的返回保存临时变时3
题目4:
如果用户输入的不是一个整数,就提示他反复输入,直到输入一个整数
package exception.tryCatchDetail;
import java.util.Scanner;
public class TryCatchDetail03 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String line = "";
boolean loop = true;
while (loop){
try {
System.out.println("请输入一个整数:");
line = sc.next();
int integer = Integer.parseInt(line);
System.out.println("num="+integer);
loop = false;
} catch (Exception e) {
System.out.println("输入的格式不对,请重新输入!");
}
}
}
}
try-catch-finally 执行顺序的小结:
- 如果没有出现异常,则执行
try 块中所有语句,不执行catch 块中语句,如果有finally ,最后还需要执行finally 里面的语句 - 如果出现异常,则
try 块中异常发生后,try 块剩下的语句不再执行。将执行catch 块中的语句,如果有finally ,最后还需要执行finally 里面的语句
throws 异常处理
基本介绍:
- 如果一个方法(中的语句执行时)可能生成某种异常,但是并不能确定如何处理这种异常,则此方法应显示地声明抛出异常,表明该方法将不对这些异常进行处理,而由该方法的调用者负责处理
- 在方法声明中用
throws 语句可以声明抛出异常的列表(意思是可以抛出多个异常信息),throws 后面的异常类型可以是方法中产生的异常类型,也可以是它的父类
快速入门案例:
package exception.throws_;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class Throws01 {
public static void main(String[] args) {
}
public void myInput() throws FileNotFoundException,Exception {
FileInputStream fileInputStream =
new FileInputStream("d://eeje.txt");
}
}
throws 注意事项和使用细节
-
对于编译异常,程序中必须处理,比如try-catch 或者throws -
对于运行时异常,程序中如果没有处理,默认就是throws 的方式处理 -
子类重写父类的方法时,对抛出异常的规定:子类重写的方法,所抛出的异常类型要么和父类抛出的异常一致,要么为父类抛出的异常的类型的子类型 -
在throws 过程中,如果有方法try-catch ,就相当于处理异常,就可以不必须throws 下面代码必须理解:
package exception.throws_;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class ThrowsDetail01 {
public static void main(String[] args) {
}
public void arithmetic(){
int num1 = 8;
int num2 = 0;
int result = num1 / num2;
System.out.println("result="+result);
}
public void myInput() throws FileNotFoundException {
FileInputStream fileInputStream = new FileInputStream("d://eeje.txt");
}
public static void f1() throws FileNotFoundException {
f2();
}
public static void f2() throws FileNotFoundException{
System.out.println("f2()方法");
}
public static void f3(){
f4();
}
public static void f4() throws RuntimeException{
System.out.println("f4()方法");
}
}
class Father{
public void show() throws RuntimeException{}
}
class Son extends Father{
public void show() throws RuntimeException{}
}
自定义异常
基本概念:当程序中出现了某些“错误”,但该错误信息并没有在Throwable 子类中描述处理,这个时候可以自己设计异常类,用于描述该错误信息
自定义异常的步骤:
- 定义类:自定义异常类名(程序员自己写)继承
Exception 或RuntimeException - 如果继承
Exception ,属性编译异常 - 如果继承
RuntimeException ,属性运行异常(一般来说,继承RuntimeException ,因为是运行时错误有默认的处理机制)
案例:
package exception.throws_;
public class CustomException {
public static void main(String[] args) {
int age = 188;
if (!(age>=18&&age<=120)){
throw new AgeException("年龄非法请输入一个正确年龄!");
}
System.out.println("您输入的年龄合法!");
}
}
class AgeException extends RuntimeException{
public AgeException(String message) {
super(message);
}
}
throw 和throws 的区别
| 意义 | 位置 | 后面跟的内容 |
---|
throws | 异常处理的一种方式 | 方法声明处 | 异常类型 | throw | 手动生成异常对象的关键字 | 方法体中 | 异常对象 |
练习题目:
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-uIjli5hH-1646999494457)(E:\Typora笔记\java笔记\img\image-20220205182227604.png)]](https://img-blog.csdnimg.cn/843699cf1a2d4a358d57c678a7a21946.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
输出的内容:
1.进入方法A
2.用A方法的finally
3.制造异常
4.进入方法B
5.调用B方法的finally
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-0uePy9KP-1646999494457)(E:\Typora笔记\java笔记\img\image-20220205182620476.png)]](https://img-blog.csdnimg.cn/2a78181e6acc4624ba6203d26ad9ac01.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-4uHPvh4Z-1646999494457)(E:\Typora笔记\java笔记\img\image-20220205185516616.png)]](https://img-blog.csdnimg.cn/68591efa0cf548abb2b133a12b2f9f88.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
package exception.throws_;
public class HomeWork02 {
public static void main(String[] args) {
if (args.length!=2){
throw new ArrayIndexOutOfBoundsException("缺少命令行参数!");
}
try {
int num1 = Integer.parseInt(args[0]);
int num2 = Integer.parseInt(args[1]);
double result = cal(num1, num2);
System.out.println("计算的结果result="+result);
} catch (NumberFormatException e) {
System.out.println("数据格式不正确!");
}catch (ArithmeticException e){
System.out.println("算数除0异常!");
}
}
public static double cal(int n1,int n2){
return n1 / n2;
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-KsDRwBde-1646999494458)(E:\Typora笔记\java笔记\img\image-20220205211801899.png)]](https://img-blog.csdnimg.cn/ba28b5f8810f460d96ba62747e1d2f07.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
结果:
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-pmTGkLhX-1646999494458)(E:\Typora笔记\java笔记\img\image-20220205212034456.png)]](https://img-blog.csdnimg.cn/0b79d58864044ed28c6502335f8e804c.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
第三题目
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-eRkdqJie-1646999494459)(E:\Typora笔记\java笔记\img\image-20220205212253335.png)]](https://img-blog.csdnimg.cn/6aad6592705247d6b0e58fba7336aaf4.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
输出:B,C,D
第四题目
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-4vvGURJs-1646999494459)(E:\Typora笔记\java笔记\img\image-20220205212730884.png)]](https://img-blog.csdnimg.cn/50c0a4ab903c44208c106540d690c85c.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5pix5pmfMTY4,size_20,color_FFFFFF,t_70,g_se,x_16)
输出: B,C,D
|