Spring 使用 XML 作为容器配置文件, 在 3.0 以后加入了 JavaConfig,使用 Java 类做配置文件使用。
一、什么是 JavaConfig ?
JavaConfig :是 Spring 提供的使用 java 类配置容器。 配置 Spring IOC 容器的纯 Java 方法
优点 :
- 可以使用面向对象的方式,一个配置类可以继承配置类,可以重写方法
- 避免繁琐的 xml 配置
二、XML 配置容器
1、新建 pre-boot 项目
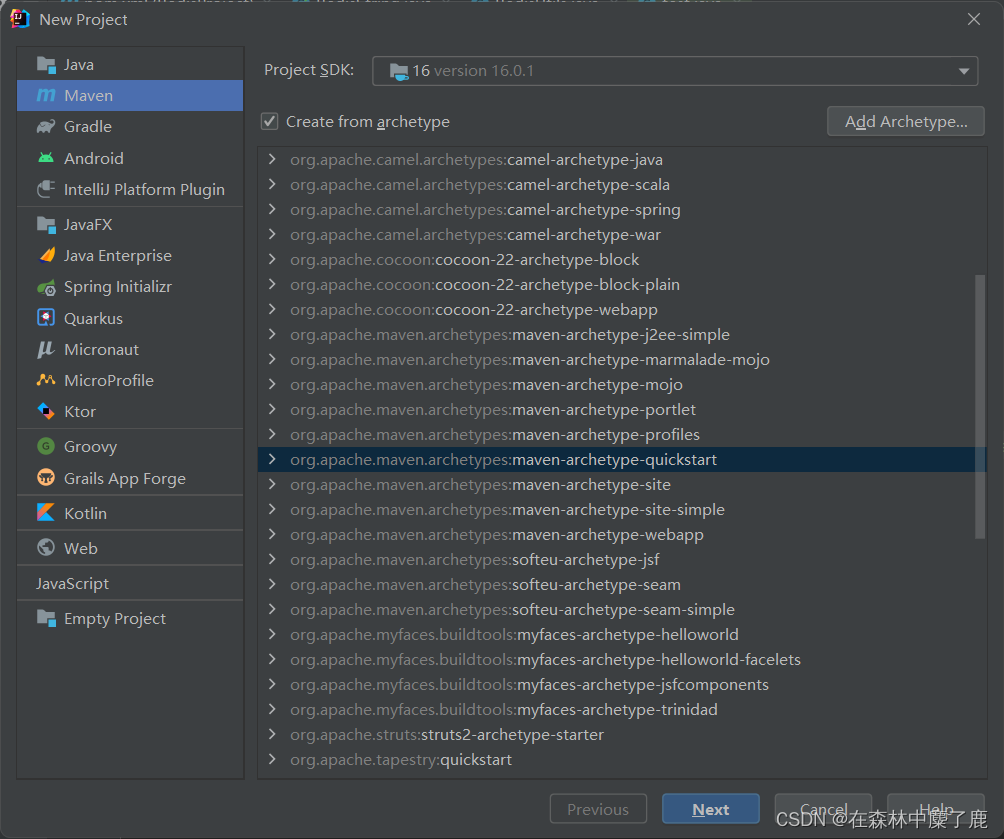
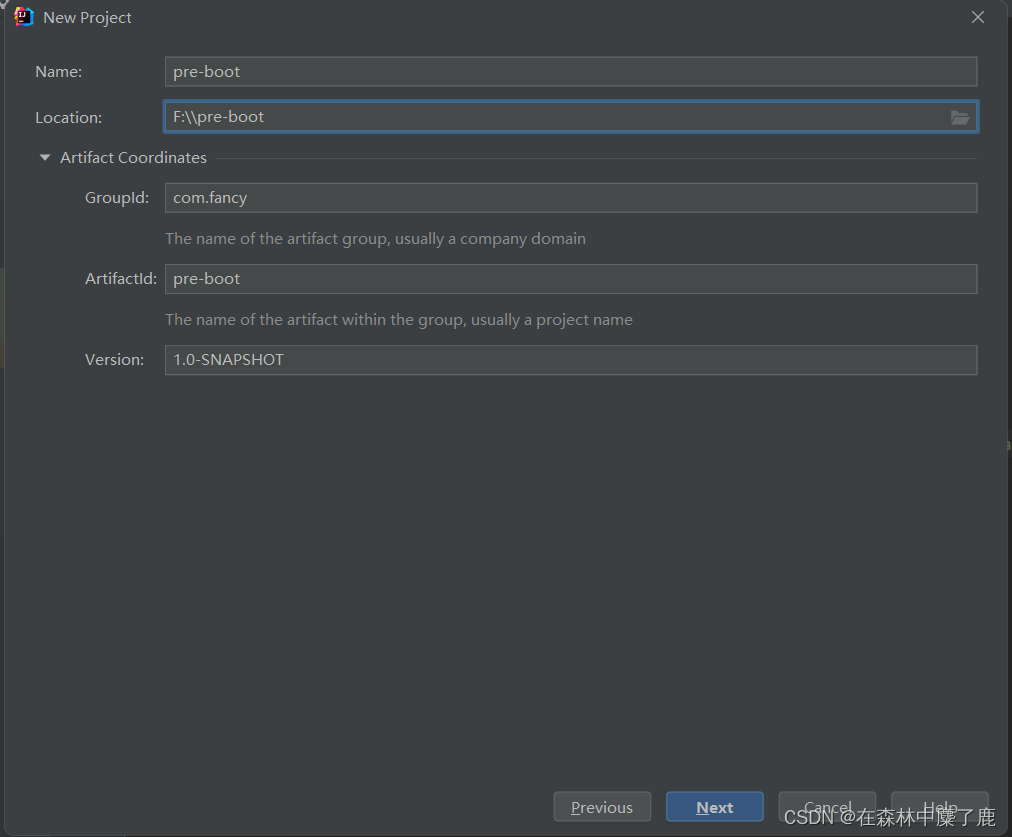 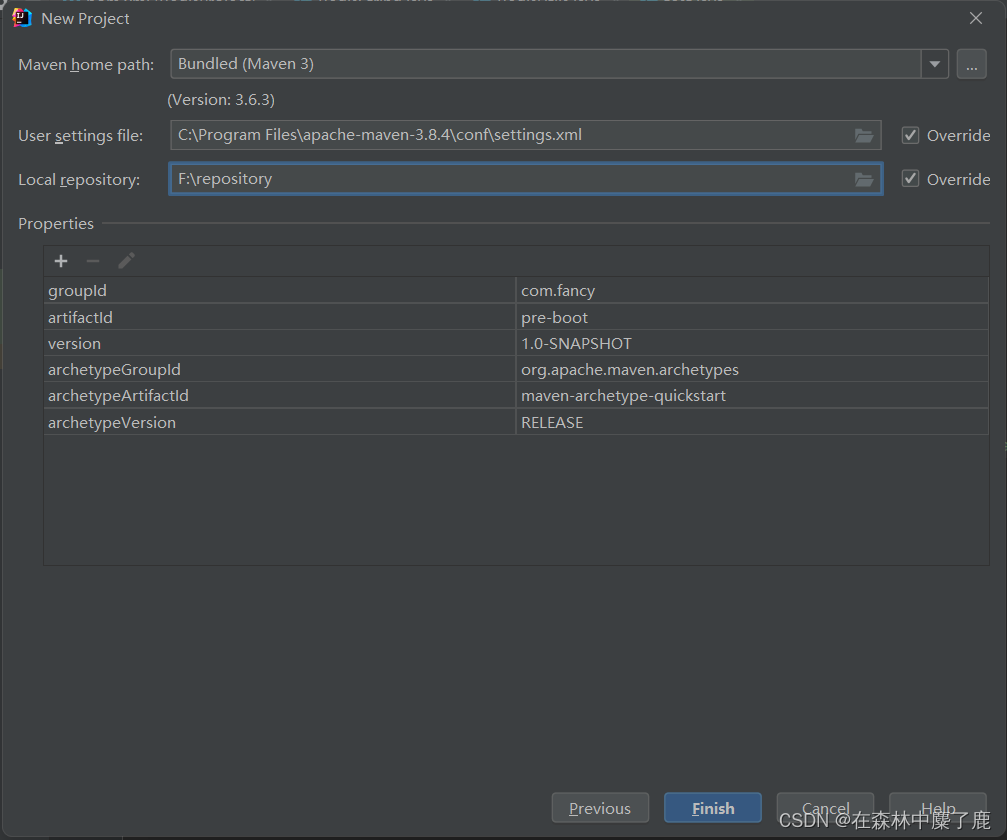
2、pom.xml
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.1</version>
</dependency>
3、创建数据类 Student
新建 vo 包,在 vo 包下创建学生类
vo :值对象(Value Object)
用new关键字创建,有GC回收通常用于业务层之间的数据传递,一般是抽象出的业务对象,可以和数据表相对应,也可以不。在web层,对应一个web页面或者swt界面,用一个VO对象对应一个界面的值。
package com.fancy.vo;
public class Student {
private Integer id;
private String name;
private Integer age;
public Student() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
4、配置文件
resources 目录下创建 Spring 的配置文件 applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myStudent" class="com.fancy.vo.Student">
<property name="id" value="101"></property>
<property name="name" value="张三"></property>
<property name="age" value="18"></property>
</bean>
</beans>
5、测试方法
@Test
public void test01 () {
String config = "applicationContext.xml";
ApplicationContext ctx = new ClassPathXmlApplicationContext(config);
Student student = (Student) ctx.getBean("myStudent");
System.out.println("student = " + student);
}

三、JavaConfig 配置容器
JavaConfig 主要使用的注解 :
1、创建配置类(等同于 xml 配置文件)
新建 config 包,并把配置类 SpringConfig 放于此包下
package com.fancy.config;
import com.fancy.vo.Student;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.Date;
@Configuration
public class SpringConfig {
@Bean
public Student createStudent() {
Student student = new Student();
student.setId(1002);
student.setName("李四");
student.setAge(18);
return student;
}
@Bean
public Student makeStudent() {
Student student = new Student();
student.setId(1003);
student.setName("王五");
student.setAge(19);
return student;
}
@Bean
public Date myDate() {
return new Date();
}
}
2、测试方法
@Test
public void test02() {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringConfig.class);
Student student = (Student) ctx.getBean("createStudent");
System.out.println("student === " + student);
}
@Test
public void test03() {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringConfig.class);
Student student = (Student) ctx.getBean("myStudent2");
System.out.println("myStudent2 ==== " + student );
Object myDate = ctx.getBean("myDate");
System.out.println("myDate = " + myDate);
}
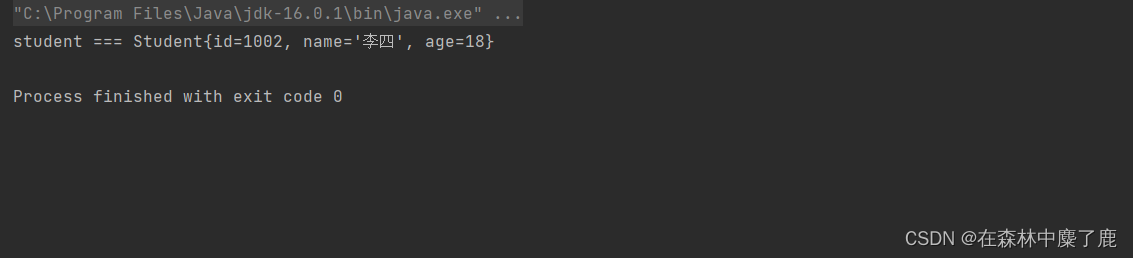 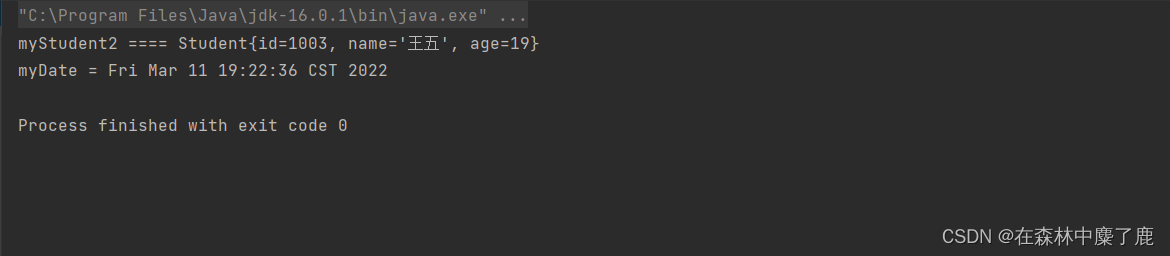
四、@ImportResource
@ImportResource 是导入 xml 配置,等同于 xml 文件的 resources
1、创建数据类
package com.fancy.vo;
public class Cat {
private String cardId;
private String name;
private Integer age;
public Cat() {
}
public Cat(String cardId, String name, Integer age) {
this.cardId = cardId;
this.name = name;
this.age = age;
}
public String getCardId() {
return cardId;
}
public void setCardId(String cardId) {
this.cardId = cardId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Cat{" +
"cardId='" + cardId + '\'' +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
2、创建配置文件 beans.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myCat" class="com.fancy.vo.Cat">
<property name="cardId" value="200101"></property>
<property name="name" value="tomcat"></property>
<property name="age" value="3"></property>
</bean>
</beans>
3、创建配置类
package com.fancy.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.ImportResource;
@Configuration
@ImportResource(value = {"classpath:beans.xml", "classpath:applicationContext.xml"})
public class SystemConfig {
}
4、创建测试方法
@Test
public void test04() {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SystemConfig.class);
Cat cat = (Cat)ctx.getBean("myCat");
System.out.println("cat == " + cat);
}
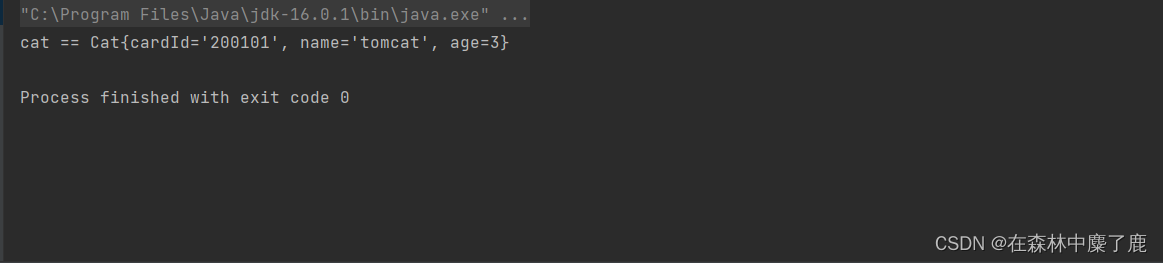
五、@PropertyResource
@PropertyResource 是读取 properties 属性配置文件
1、创建 config.properties
tiger.name=hhh
tiger.age=6
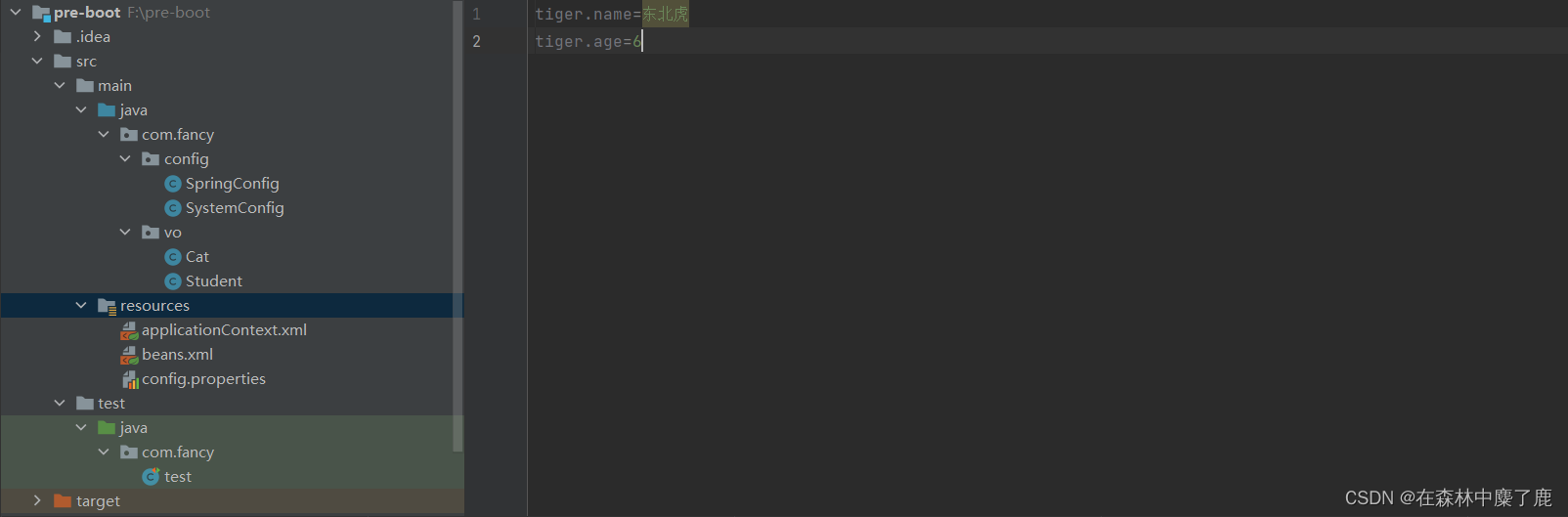
2、创建数据类 Tiger
package com.fancy.vo;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component("tiger")
public class Tiger {
@Value("${tiger.name}")
private String name;
@Value("${tiger.age}")
private Integer age;
@Override
public String toString() {
return "Tiger{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
3、修改 SystemConfig 配置文件
package com.fancy.config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.ImportResource;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySource(value = "classpath:config.properties")
@ComponentScan(value = "com.fancy.vo")
public class SystemConfig {
}
4、测试方法
@Test
public void test05() {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SystemConfig.class);
Tiger tiger = (Tiger) ctx.getBean("tiger");
System.out.println("Tiger === " + tiger);
}
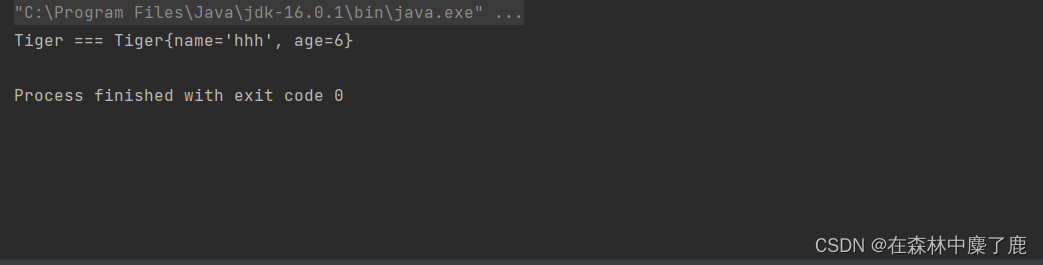
|