持续学习&持续更新中…
守破离
【Java从零到架构师第1季】【并发 Concurrent 03】线程间通信_ReentrantLock_线程池
线程间通信
- 调用wait、notify、notifyAll方法的obj对象必须是同一个
- 调用wait、notify、notifyAll方法的线程必须拥有该obj对象的内部锁
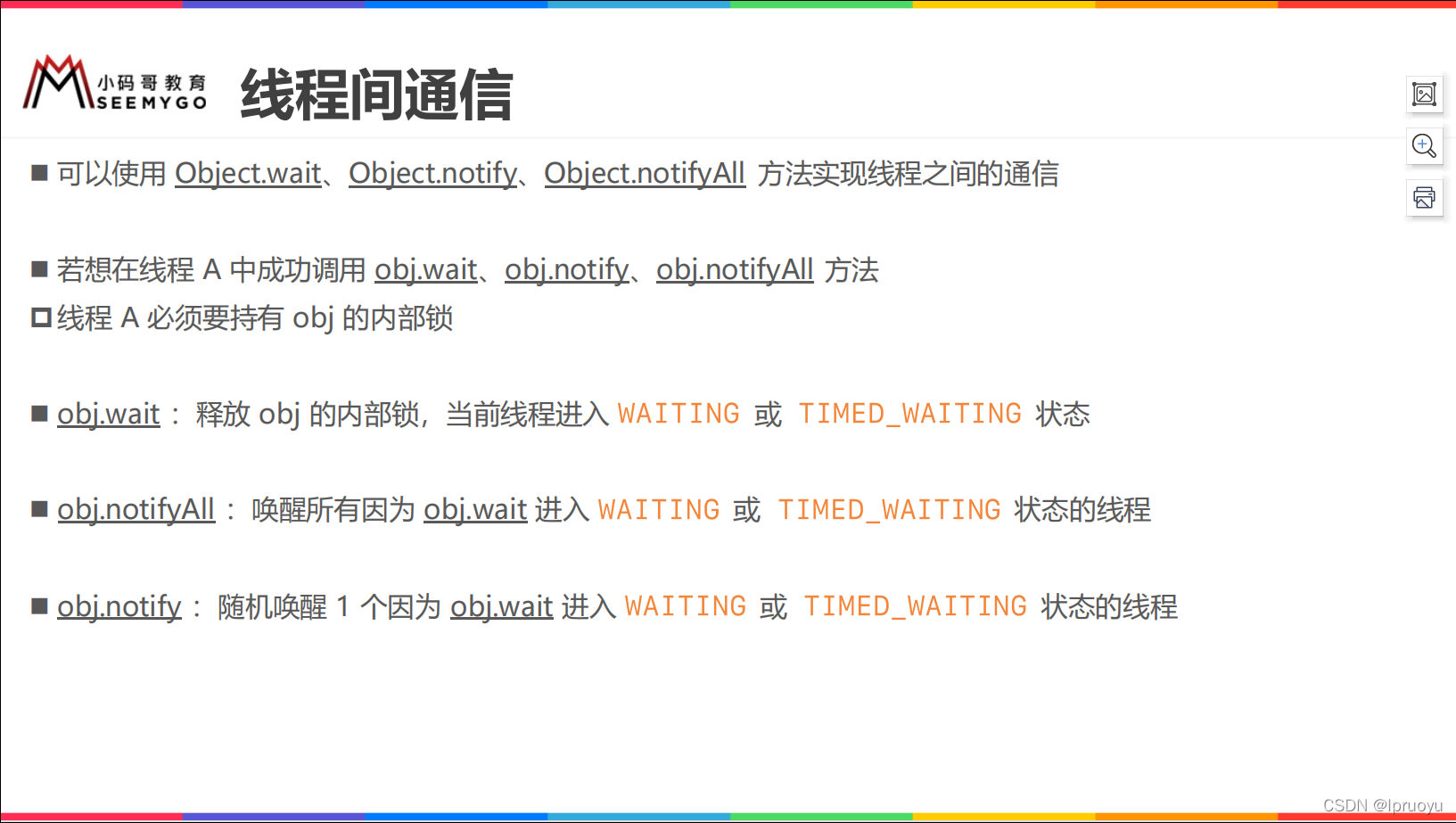
线程间通信—示例
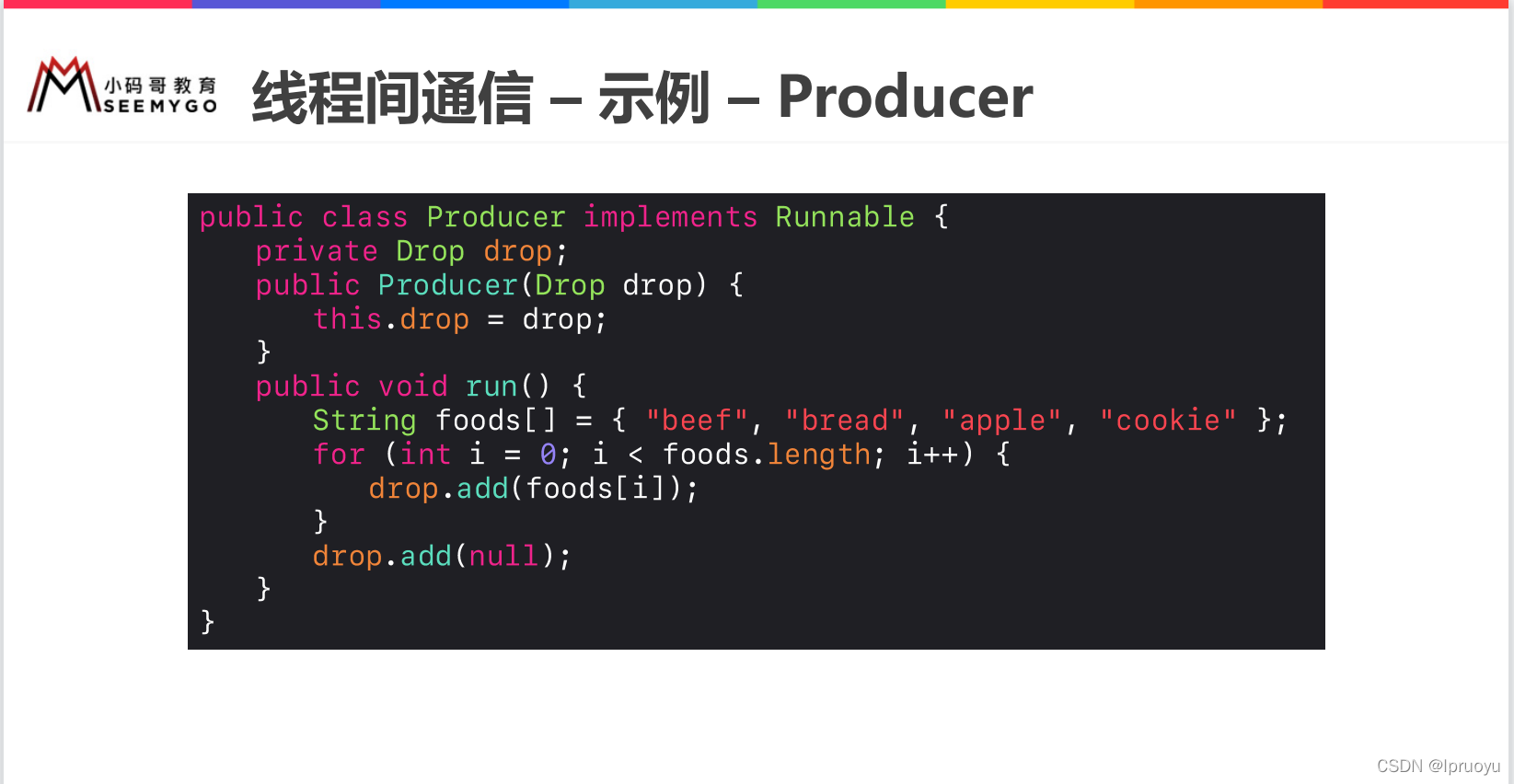
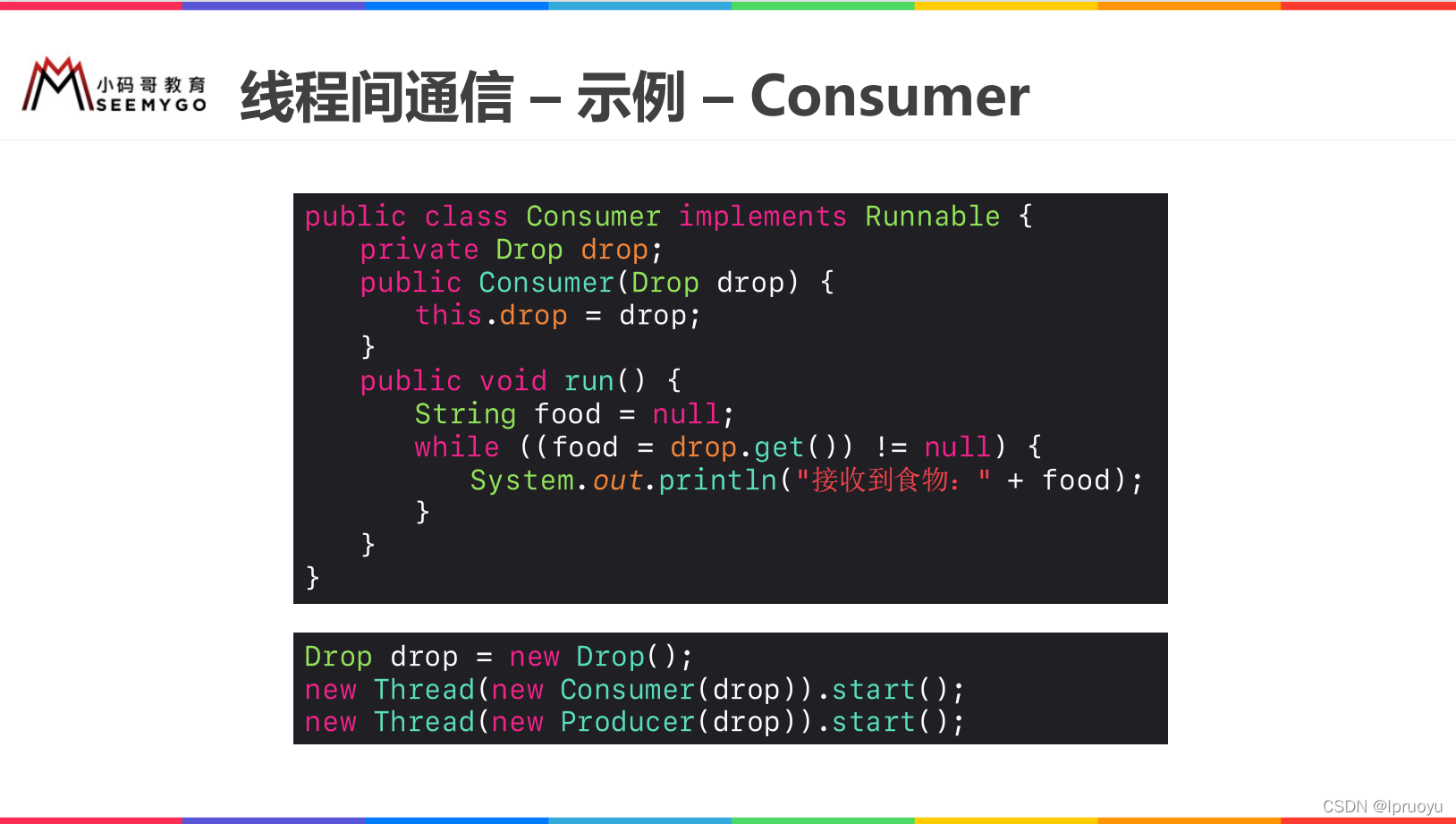
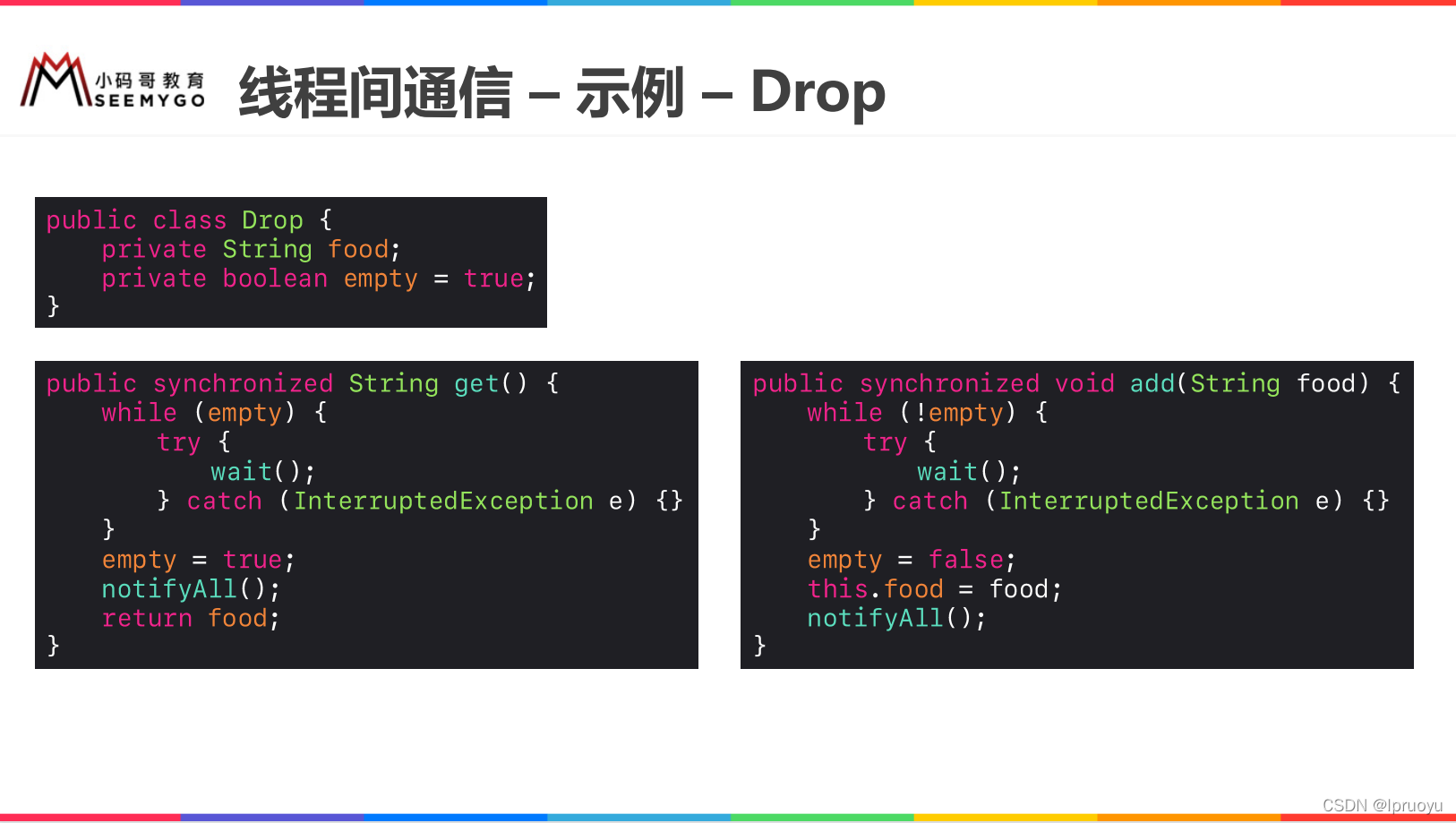
注意:
代码:
Producer:
public class Producer implements Runnable{
private Drop drop;
public Producer(Drop drop) {
this.drop = drop;
}
@Override
public void run() {
String[] foods = {"beef", "bread", "apple", "cookie", "banana"};
for (String food : foods) {
try {
Thread.sleep(1500);
} catch (InterruptedException e) {}
drop.add(food);
}
drop.add(null);
}
}
Consumer:
public class Consumer implements Runnable {
private Drop drop;
public Consumer(Drop drop) {
this.drop = drop;
}
@Override
public void run() {
String food;
while ((food = drop.get()) != null) {
System.out.println("消费了:" + food);
}
}
}
Drop:
version1:
public class Drop {
private String food;
private boolean isEmpty = true;
public synchronized void add(String food) {
if(isEmpty) {
this.food = food;
isEmpty = false;
notifyAll();
}else {
try {
wait();
} catch (InterruptedException e) {}
this.food = food;
isEmpty = false;
notifyAll();
}
}
public synchronized String get() {
if(isEmpty) {
try {
wait();
} catch (InterruptedException e) {}
}
isEmpty = true;
notifyAll();
return food;
}
}
老师课件上写的while(empty) 是为了防止wait时出现异常(如果wait时出现了异常并且不做任何处理的话,那么程序就会按顺序执行下去,那样的话程序就会出现bug;因此使用while循环:如果wait时出现了异常还会继续wait)
在wait时应该使用while循环来防止wait失败(如果wait出现异常,还得继续wait)
version2(使用while循环代替if循环):
public class Drop {
private String food;
private boolean isEmpty = true;
public synchronized void add(String food) {
if(isEmpty) {
this.food = food;
isEmpty = false;
notifyAll();
}else {
while(!isEmpty) {
try {
wait();
} catch (InterruptedException e) {}
}
this.food = food;
isEmpty = false;
notifyAll();
}
}
public synchronized String get() {
while(isEmpty) {
try {
wait();
} catch (InterruptedException e) {}
}
isEmpty = true;
notifyAll();
return food;
}
}
测试:
public static void main(String[] args) {
Drop drop = new Drop();
Consumer consumer = new Consumer(drop);
Producer producer = new Producer(drop);
new Thread(consumer).start();
new Thread(producer).start();
}
可重入锁ReentrantLock
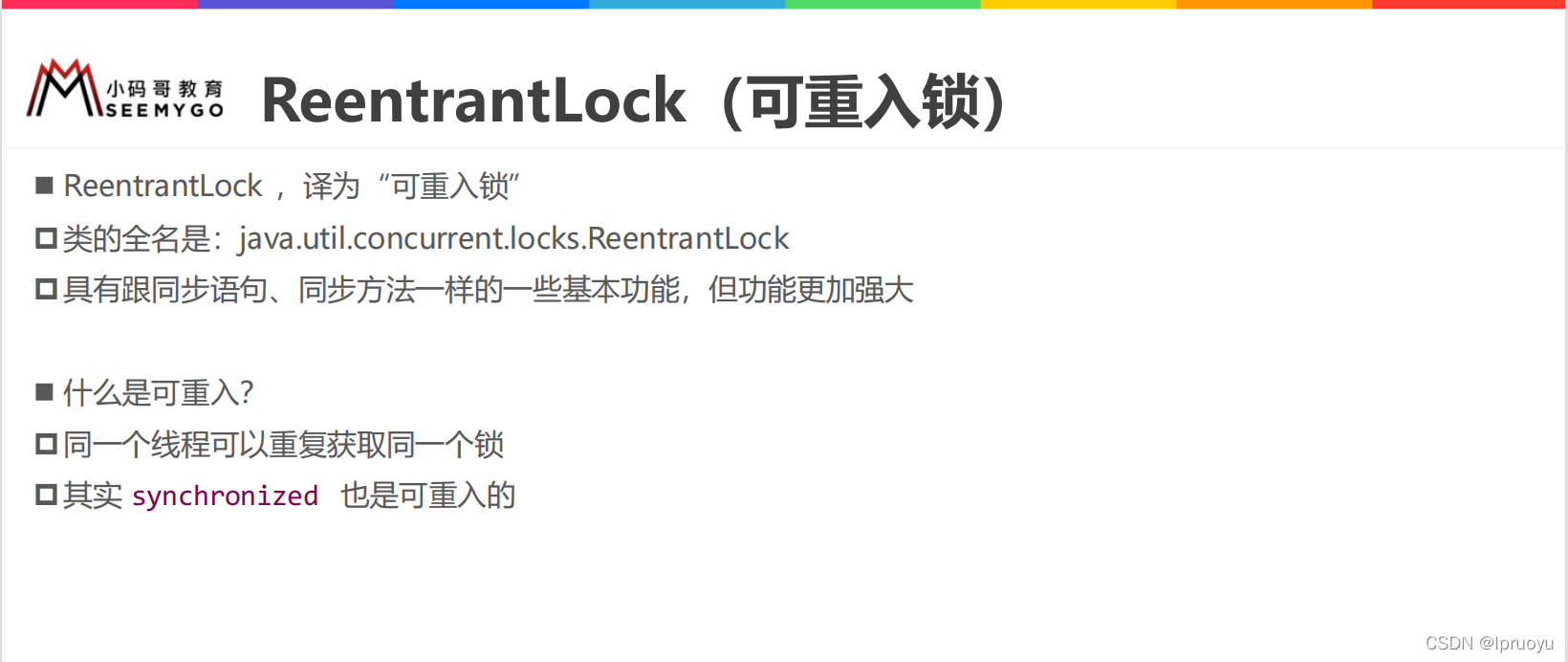
public static void main(String[] args) {
synchronized ("1") {
synchronized ("1") {
System.out.println("1");
}
}
}
我们可以认为:每一个线程都有属于自己的锁持有计数器
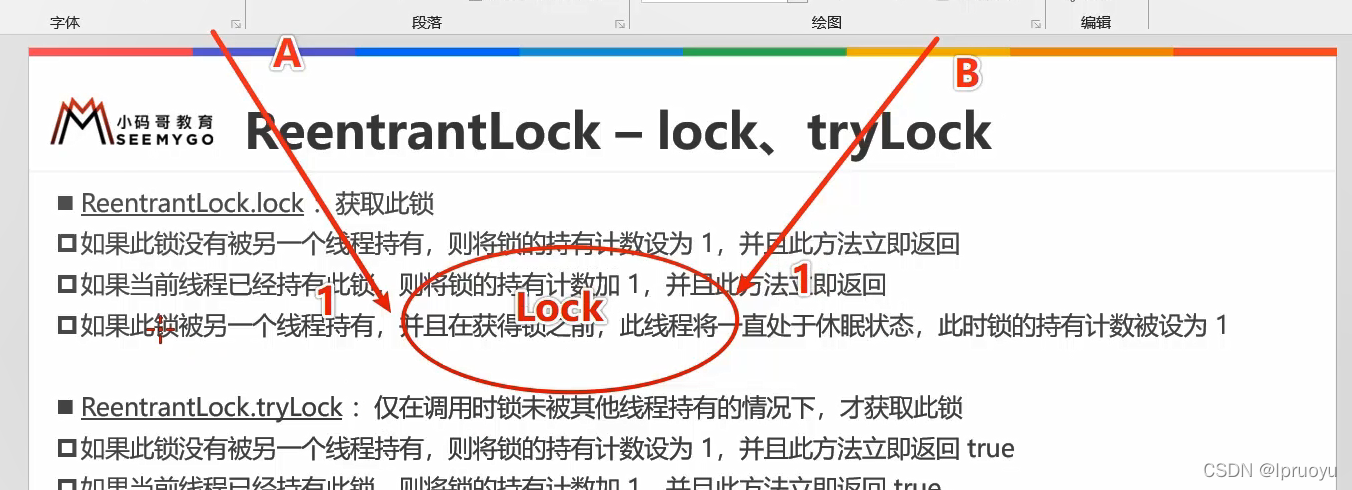
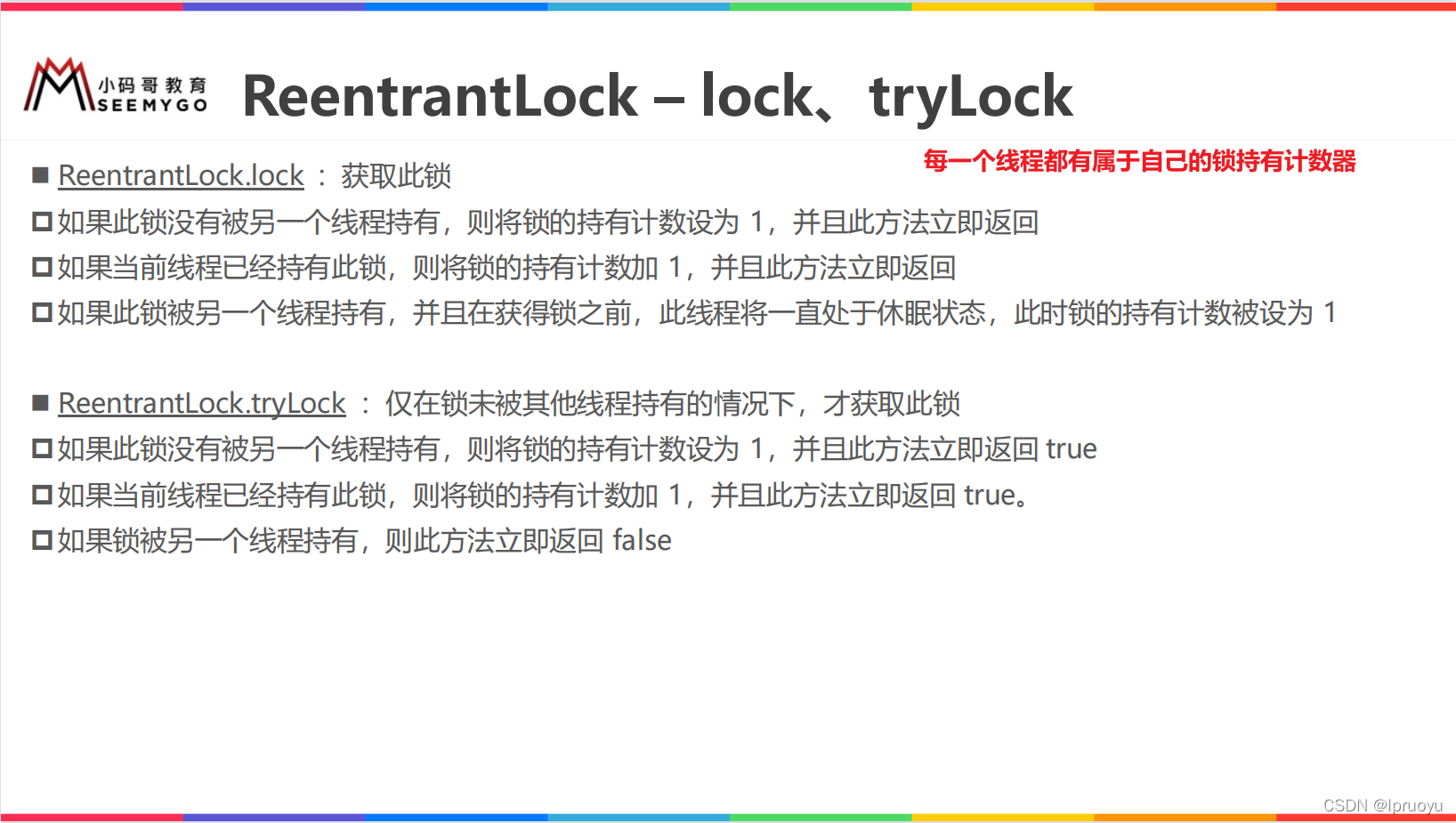
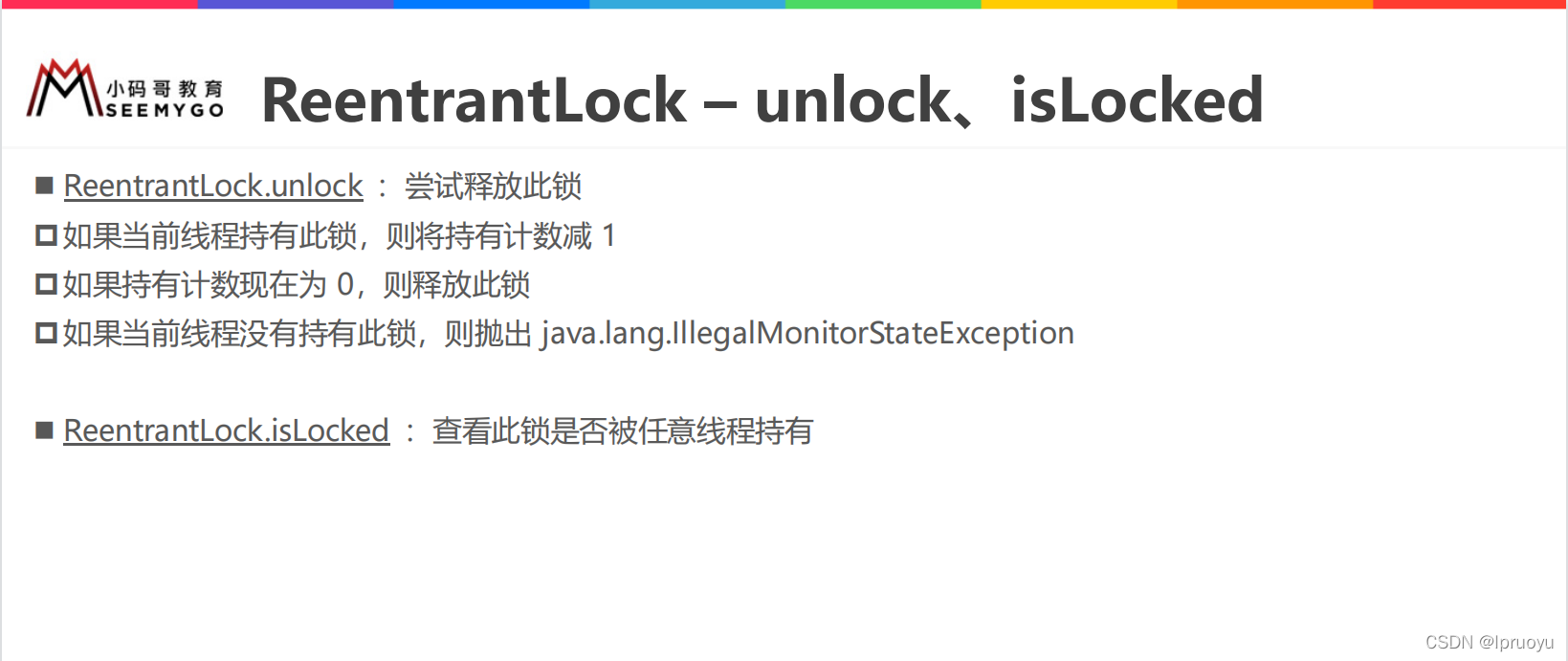
ReentrantLock的使用
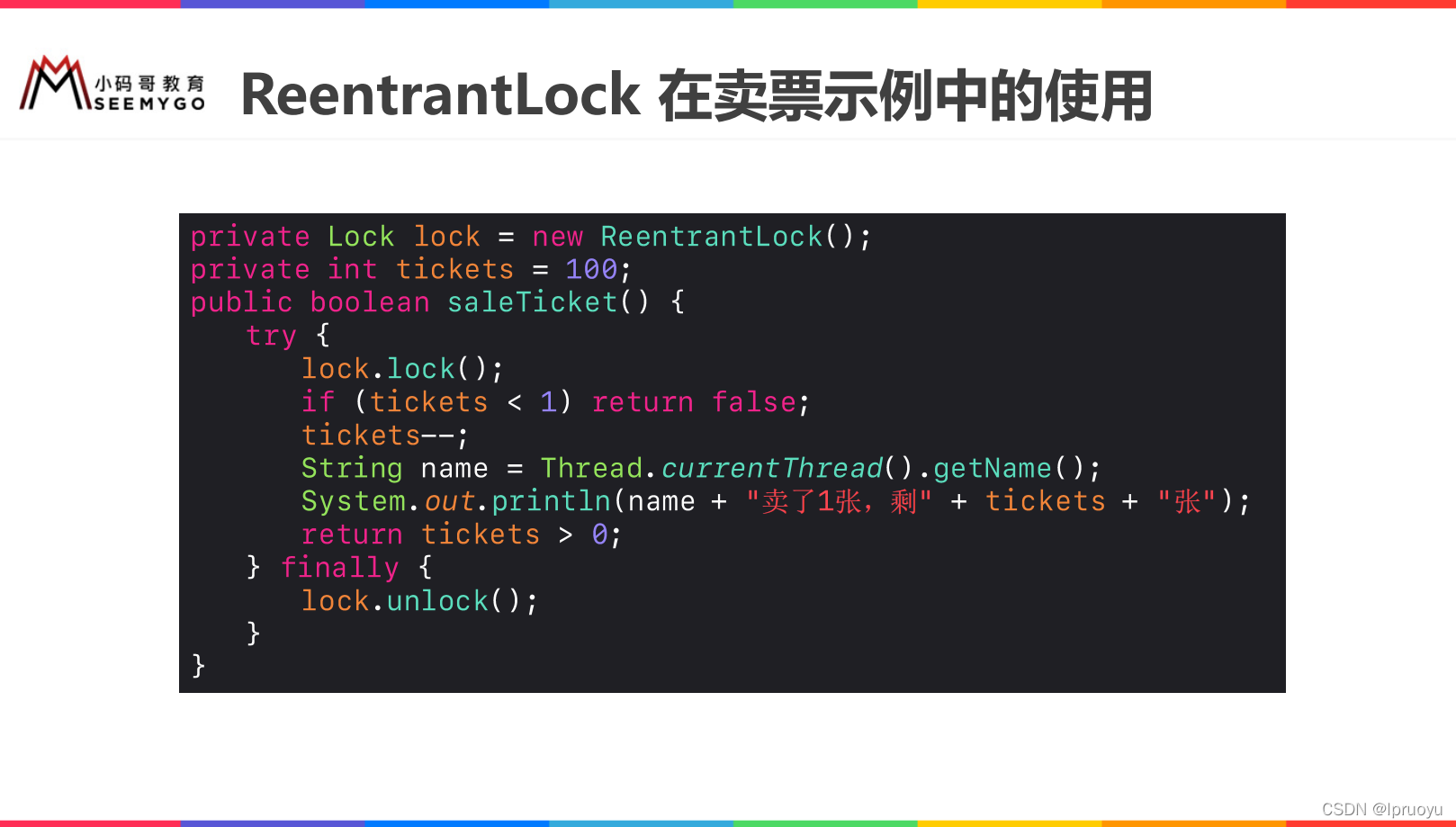
public class Station implements Runnable {
private final ReentrantLock lock = new ReentrantLock();
private int tickets = 100;
public boolean saleTicket() {
try{
lock.lock();
if (tickets < 1) return false;
tickets--;
System.out.println(Thread.currentThread().getName() + "卖了一张票,还剩" + tickets + "张票。");
return tickets > 0;
}finally {
lock.unlock();
}
}
@Override
public void run() {
while (saleTicket()) ;
}
}
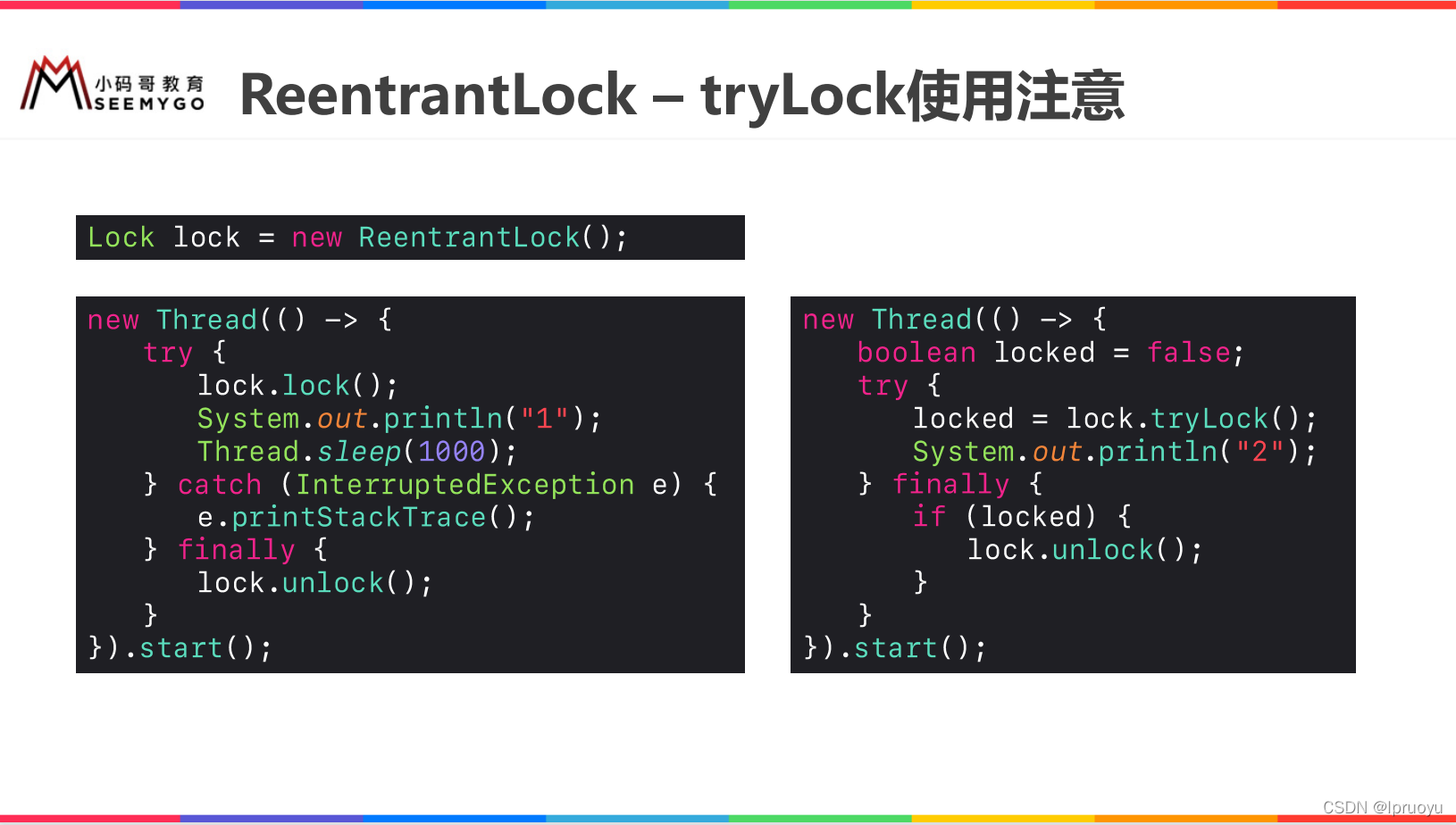
线程池
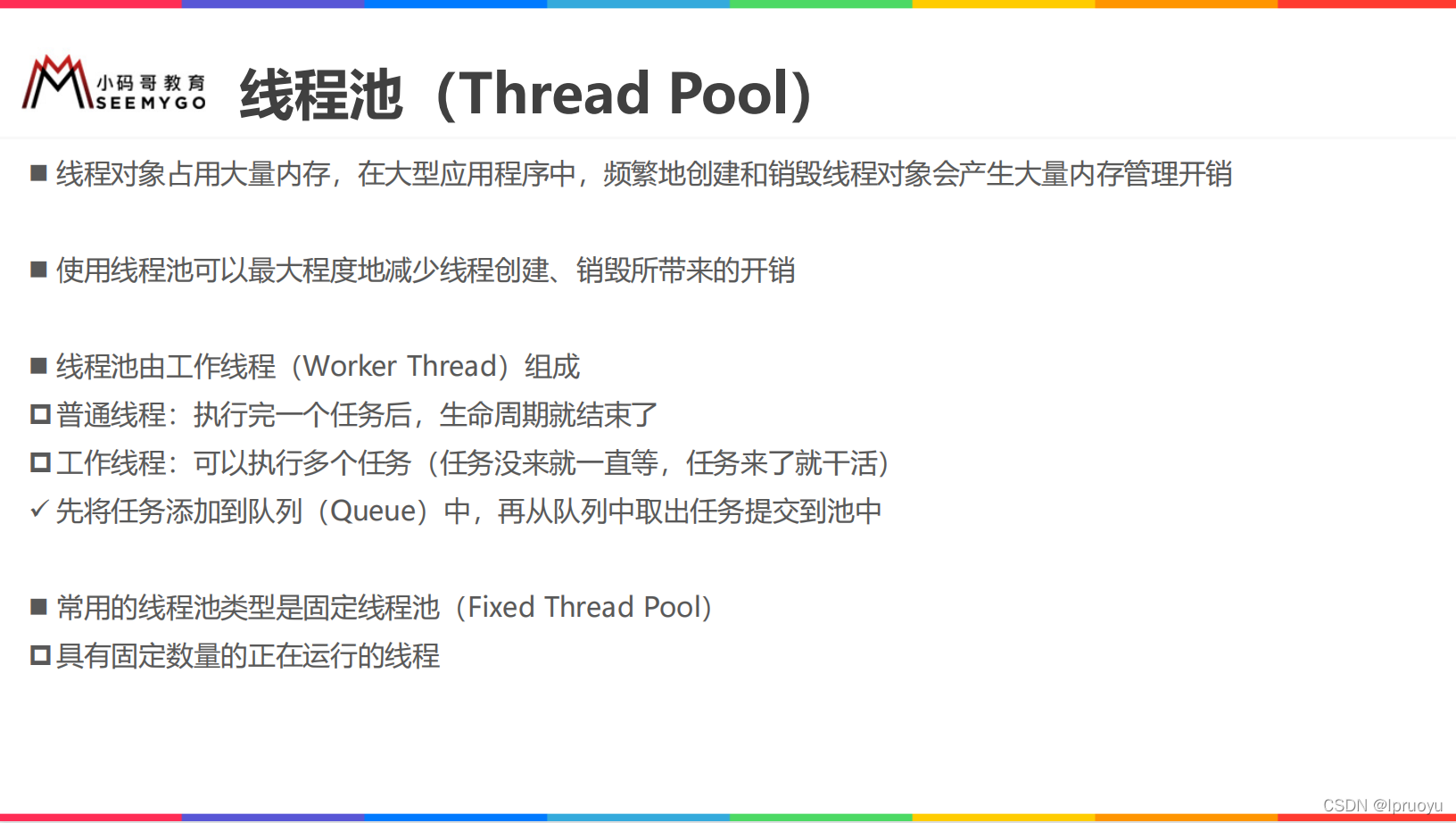
线程池—基本使用
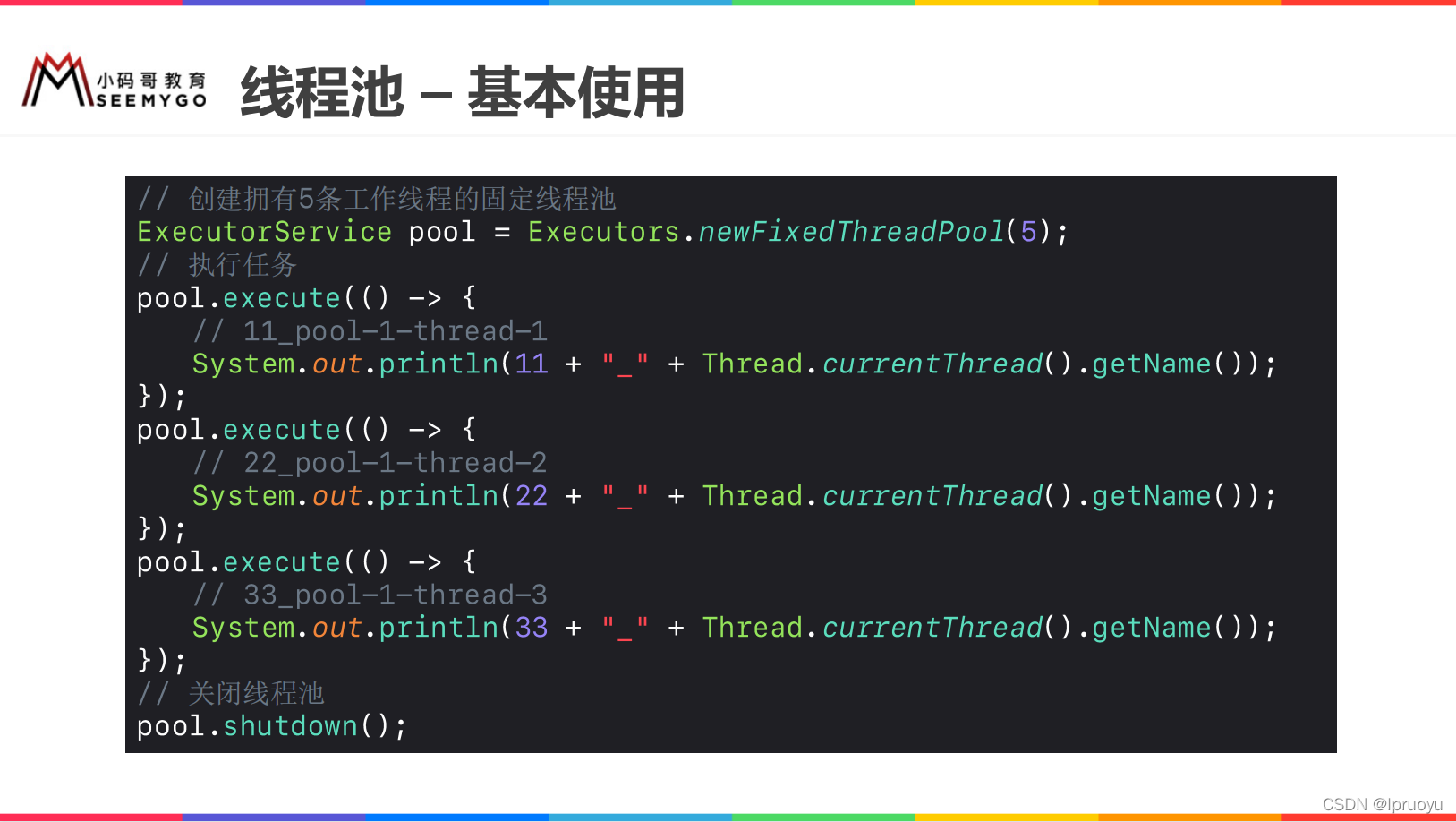
public static void main(String[] args) {
final ExecutorService pool = Executors.newFixedThreadPool(5);
for (int i = 1; i <= 10; i++) {
pool.execute(() -> {
System.out.println(Thread.currentThread().getName());
});
}
pool.shutdown();
}
参考
小码哥-李明杰: Java从0到架构师①零基础高效率入门.
本文完,感谢您的关注支持!
|