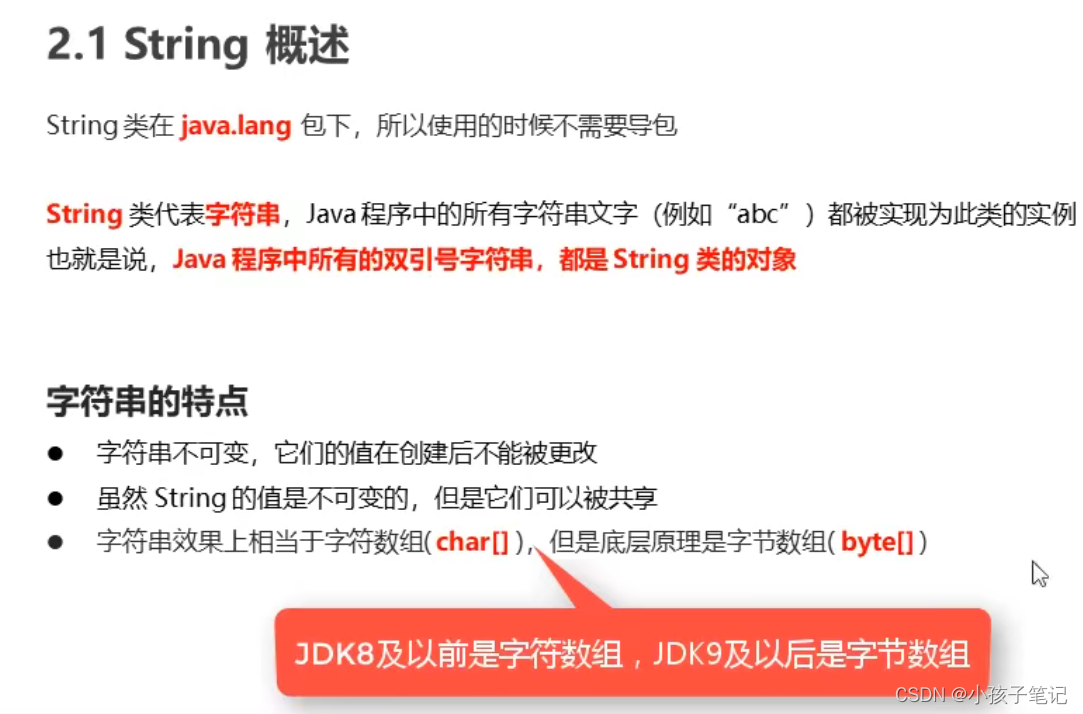 字符串的构造方法: 因为是字符串,且a=97,b=98,数字是计算机中存储的,而我们看到的字符是字母,所以s3显示的是数字转字符字母abc 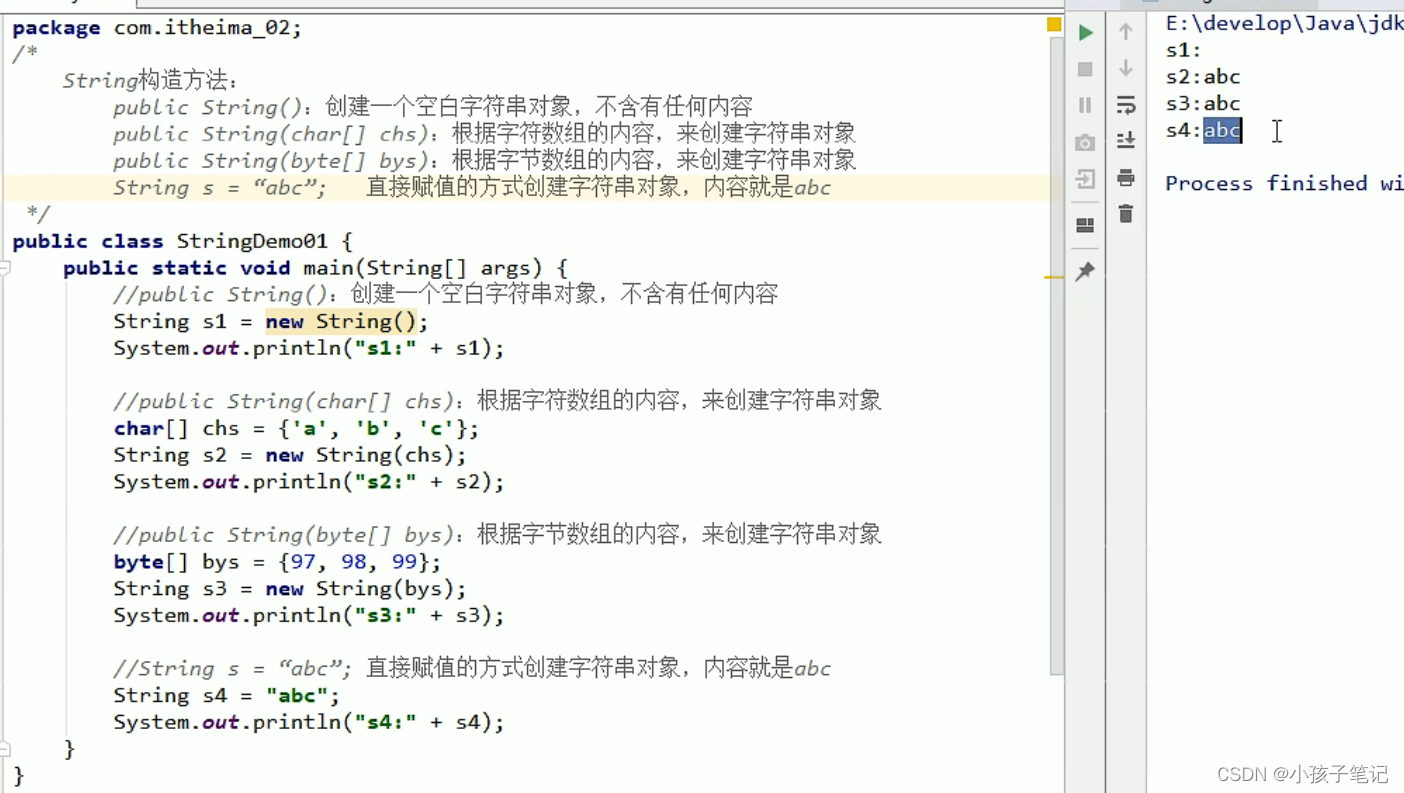
用 == 号做比较,要看数据类型 基本数据类型:比较的是数据值是否相同 引用数据类型: 比较的是地址值是否相同
所以比较String的地址值是否相同时,可以用 == 而比较数据值是否相同时,使用一个方法equals() 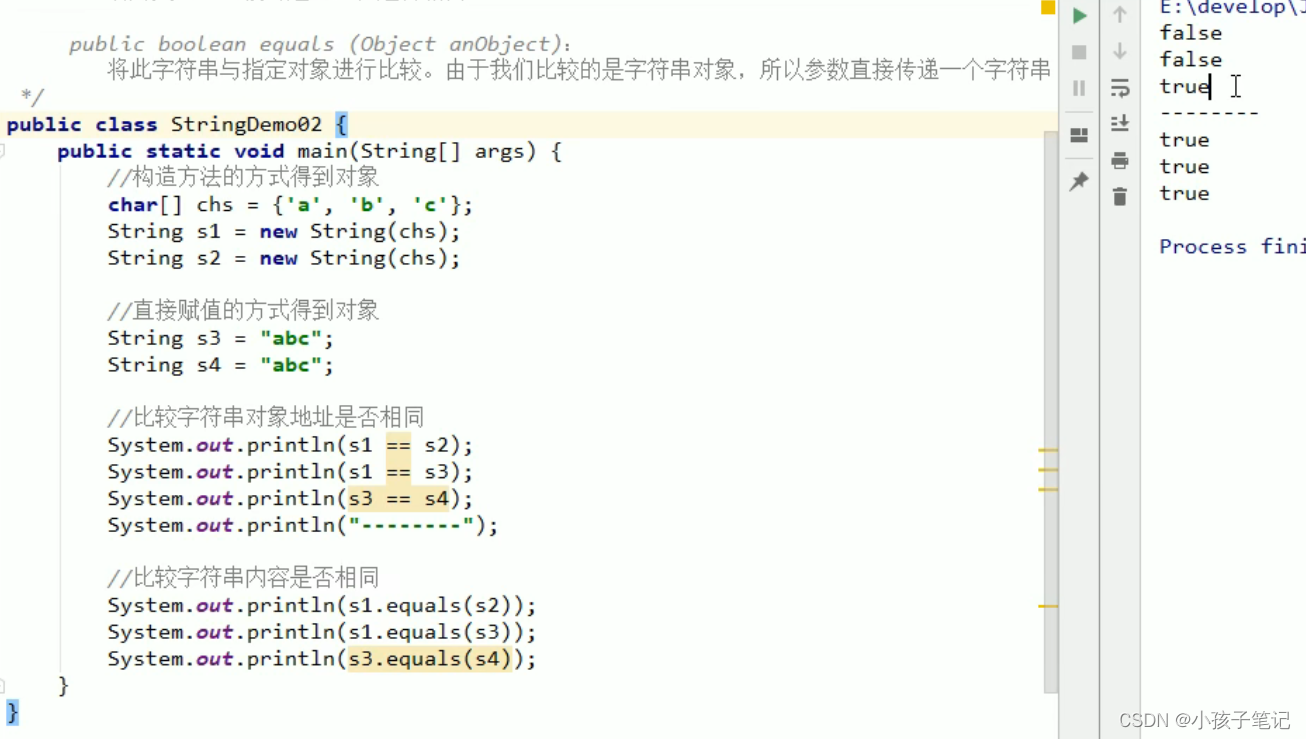 统计Sring中的字符个数: 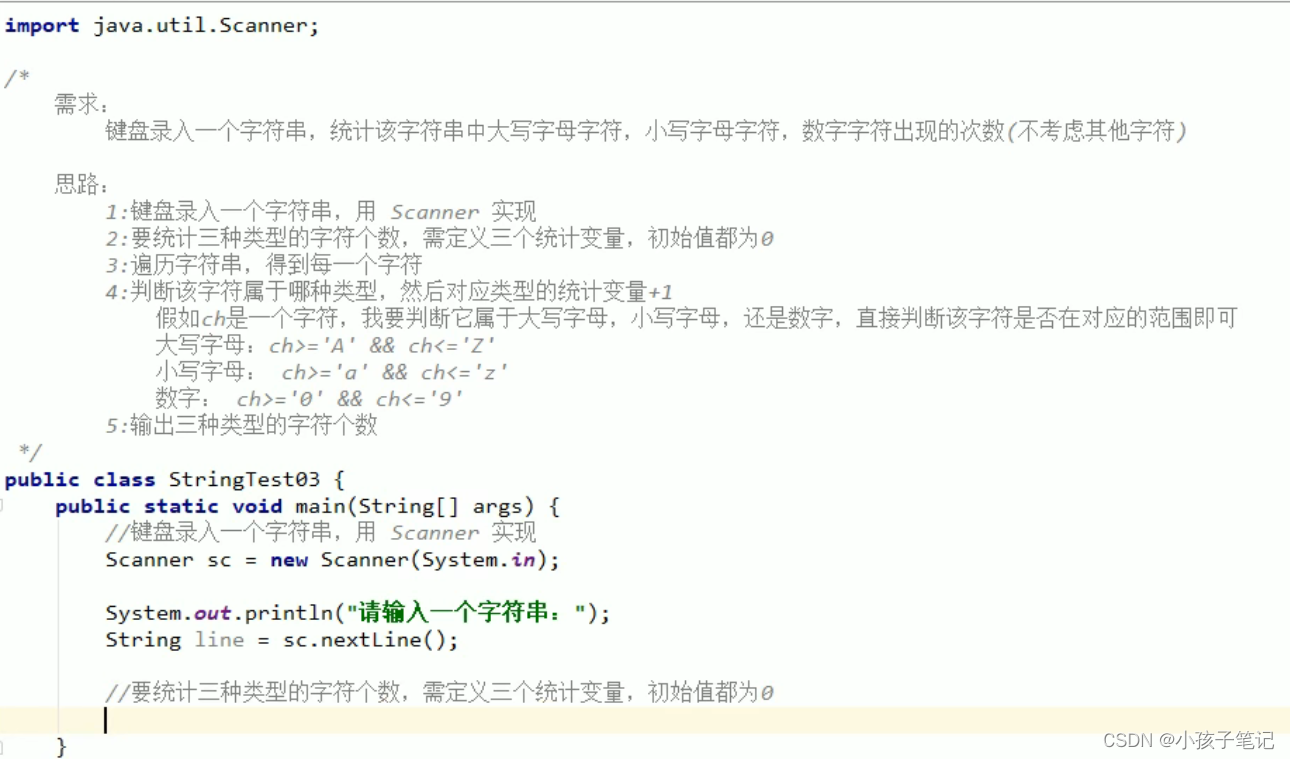 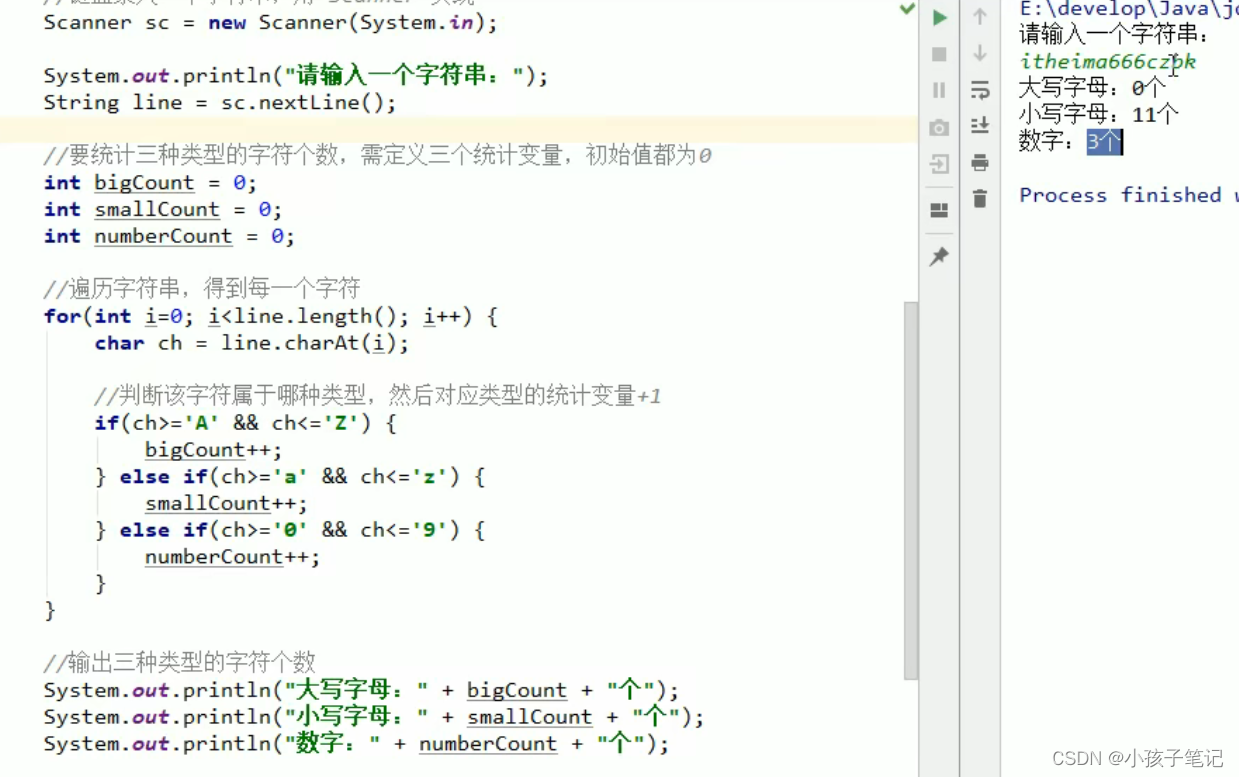 普通String字符串拼接: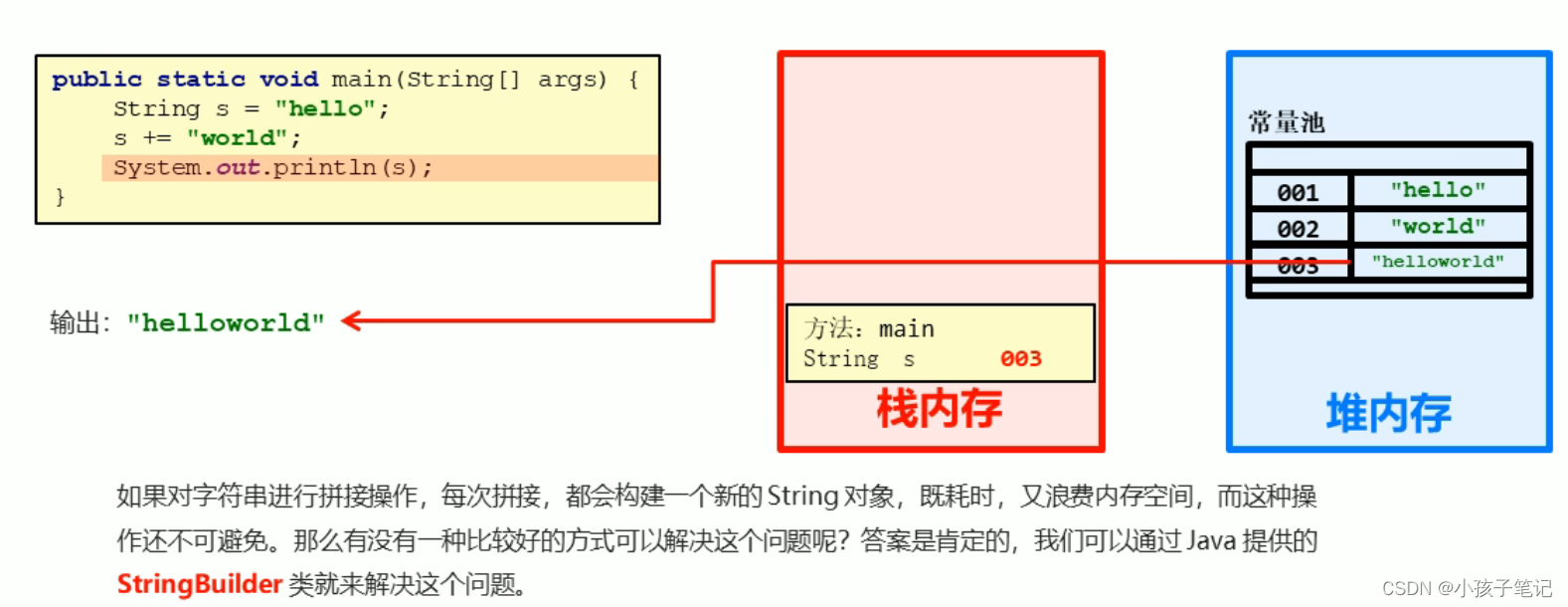
StringBuilder:
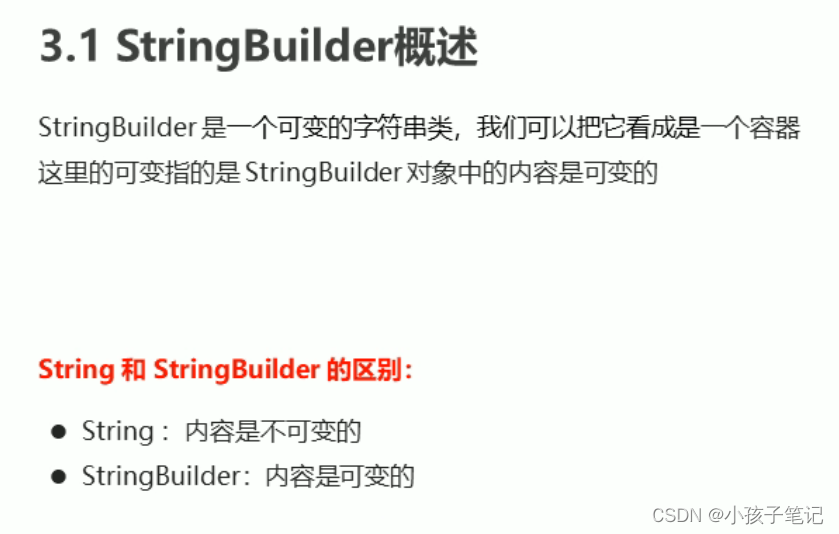 StringBuilder的构方法: 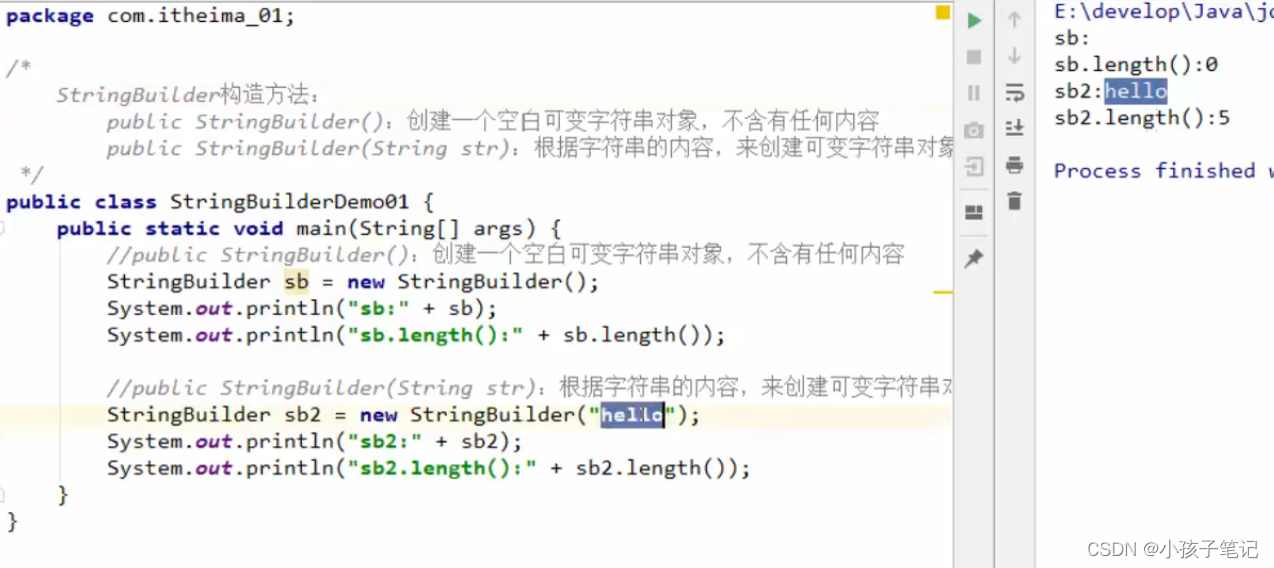 StringBuilder的添加append()与反转方法reverse(): 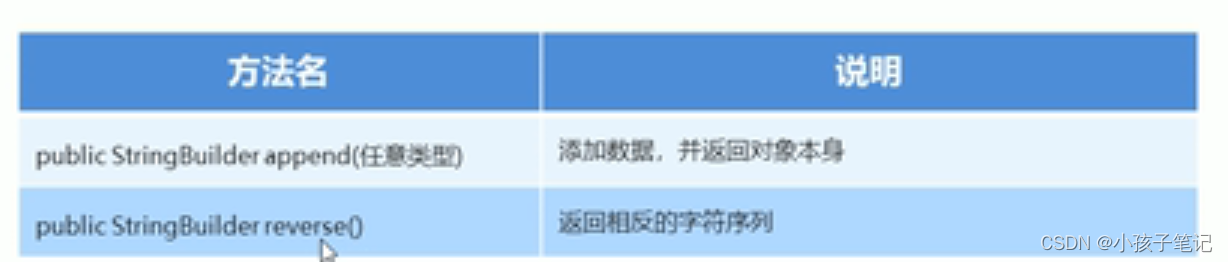 String与StringBuilder的相互转换: 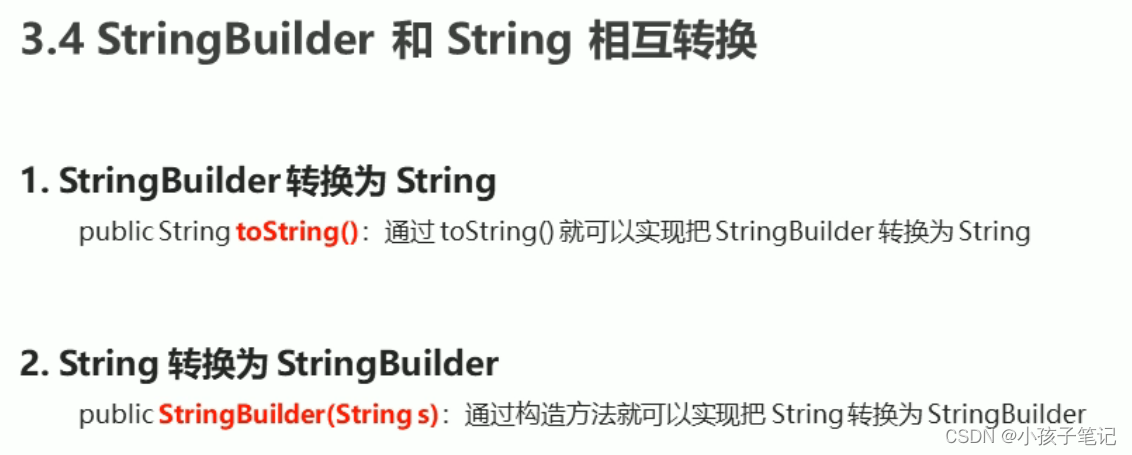
String的整理:
package com.company;
//===============================String 类===========================================
/*
字符串:在 Java 中字符串属于对象,Java 提供了 String 类来创建和操作字符串
注:String 创建的字符串存储在公共池中,而 new 创建的字符串对象在堆上;
String 类是不可改变的,所以你一旦创建了 String 对象,那它的值就无法改变了
创建字符串:String str = "aba"; // String 直接创建
用构造函数创建字符串:String str2 = new String("aba"); // String 对象创建
字符串长度:
访问器方法:获取有关对象的信息的方法
length() 方法,它返回字符串对象包含的字符数
String site = "www.runoob.com";
int len = site.length();
System.out.println( "菜鸟教程网址长度 : " + len ); //菜鸟教程网址长度 : 14
连接字符串:
concat(),连接两个字符串的方法
+连接
"我的名字是 ".concat("Runoob"); //我的名字是Runoob
"Hello," + " runoob" + "!" //Hello, runoob!
创建格式化字符串:
输出格式化数字可以使用 printf() 和 format() 方法;
String 类使用静态方法 format() 返回一个String 对象而不是 PrintStream 对象;
String 类的静态方法 format() 能用来创建可复用的格式化字符串,而不仅仅是用于一次打印输出
System.out.printf("浮点型变量的值为 " +
"%f, 整型变量的值为 " +
" %d, 字符串变量的值为 " +
"is %s", floatVar, intVar, stringVar);
或者
String fs;
fs = String.format("浮点型变量的值为 " +
"%f, 整型变量的值为 " +
" %d, 字符串变量的值为 " +
" %s", floatVar, intVar, stringVar);
String方法:
charAt():返回指定索引处的字符;索引范围为从 0 到 length() - 1;
String s = "www.runoob.com";
char result = s.charAt(6);
System.out.println(result); //n
compareTo():两种方式的比较
字符串与对象进行比较
按字典顺序比较两个字符串
如果参数字符串等于此字符串,则返回值 0;
如果此字符串小于字符串参数,则返回一个小于 0 的值;
如果此字符串大于字符串参数,则返回一个大于 0 的值。
String str1 = "Strings";
String str2 = "Strings";
String str3 = "Strings123";
int result = str1.compareTo( str2 );
System.out.println(result); //0
result = str2.compareTo( str3 );
System.out.println(result); //-3
result = str3.compareTo( str1 );
System.out.println(result); //3
compareToIgnoreCase():按字典顺序比较两个字符串,不考虑大小写
如果参数字符串等于此字符串,则返回值 0;
如果此字符串小于字符串参数,则返回一个小于 0 的值;
如果此字符串大于字符串参数,则返回一个大于 0 的值。
String str1 = "STRINGS";
String str2 = "Strings";
int result = str1.compareToIgnoreCase( str2 ); //0
System.out.println(result);
String str1="javDscrspt";
String str2="jAvascript";
str1.compareToIgnoreCase(str2); //此时返回值为3,是d的ASCII码(100)减去了a的ASCII码值(97)得到或者D与A相差得到的。
String str1="javAScript";
String str2="JaVa";
str1.compareToIgnoreCase(str2); //此时返回值为6,是str1相比str2多出来的字符个数。
此时只比较位数,而无关ASCII码值,并非是S(s)的ASCII码值减去0的ASCII码值,在参数字符串前面字符和原字符串一样时,
返回值就是两者相差的字符个数,即使改变后面的字符也不会影响到返回的值,比如String str1="jAva233666",此时结果仍是6。
concat():连接字符串
String s = "菜鸟教程:";
s = s.concat("www.runoob.com");
contentEquals():相同的字符序列,则返回 true;否则返回 false
String str1 = "String1";
String str2 = "String2";
StringBuffer str3 = new StringBuffer( "String1");
boolean result = str1.contentEquals( str3 );
System.out.println(result); //true
result = str2.contentEquals( str3 );
System.out.println(result); //false
copyValueOf():
char[] Str1 = {'h', 'e', 'l', 'l', 'o', ' ', 'r', 'u', 'n', 'o', 'o', 'b'};
String Str2 = "";
Str2 = Str2.copyValueOf( Str1 );
System.out.println("返回结果:" + Str2); //hello runoob
Str2 = Str2.copyValueOf( Str1, 2, 6 ); //Str1字符串数组 2初始偏移量 6子数组长度,0到lenght-1
System.out.println("返回结果:" + Str2); //llo ru
endsWith():测试字符串是否以指定的后缀结束。
String Str = new String("菜鸟教程:www.runoob.com");
boolean retVal;
retVal = Str.endsWith( "runoob" );
System.out.println("返回值 = " + retVal ); //false
retVal = Str.endsWith( "com" );
System.out.println("返回值 = " + retVal ); //true
equals() 方法用于将字符串与指定的对象比较,比较两个字符串的内容是否相等
注意:
== 比较引用地址是否相同;
equals() 比较字符串的内容是否相同;
String s1 = "Hello"; // String 直接创建
String s2 = "Hello"; // String 直接创建
String s3 = s1; // 相同引用
String s4 = new String("Hello"); // String 对象创建
String s5 = new String("Hello"); // String 对象创建
s1 == s1; // true, 相同引用
s1 == s2; // true, s1 和 s2 都在公共池中,引用相同
s1 == s3; // true, s3 与 s1 引用相同
s1 == s4; // false, 不同引用地址
s4 == s5; // false, 堆中不同引用地址
s1.equals(s3); // true, 相同内容
equalsIgnoreCase():将字符串与指定的对象比较,不考虑大小写
注意:equals() 会判断大小写区别;equalsIgnoreCase() 不会判断大小写区别
String Str1 = new String("runoob");
String Str4 = new String("RUNOOB");
boolean retVal;
retVal = Str1.equalsIgnoreCase( Str4 );
System.out.println("返回值 = " + retVal ); //true
getBytes():
getBytes(String charsetName): 使用指定的字符集将字符串编码为 byte 序列,并将结果存储到一个新的 byte 数组中;
getBytes(): 使用平台的默认字符集将字符串编码为 byte 序列,并将结果存储到一个新的 byte 数组中;
String Str1 = new String("runoob");
try{
byte[] Str2 = Str1.getBytes();
System.out.println("返回值:" + Str2 ); //返回值:[B@7852e922
Str2 = Str1.getBytes( "UTF-8" );
System.out.println("返回值:" + Str2 ); //返回值:[B@4e25154f
Str2 = Str1.getBytes( "ISO-8859-1" );
System.out.println("返回值:" + Str2 ); //返回值:[B@70dea4e
} catch ( UnsupportedEncodingException e){
System.out.println("不支持的字符集");
}
getChars():将字符从字符串复制到目标字符数组
String Str1 = new String("www.runoob.com");
char[] Str2 = new char[6];
try {
Str1.getChars(4, 10, Str2, 0); //从4开始复制到10,(0到lenght-1,开始的4包含,结束的10不包含),复制出来的数据保存在目标数组Str2中,目标数组中起始偏移量为0;
System.out.print("拷贝的字符串为:" );
System.out.println(Str2 ); //runoob
} catch( Exception ex) {
System.out.println("触发异常...");
}
hashCode():返回字符串的哈希码;空字符串的哈希值为 0
String Str = new String("www.runoob.com");
System.out.println("字符串的哈希码为 :" + Str.hashCode() ); //字符串的哈希码为 :321005537
indexOf():
public int indexOf(int ch): 返回指定字符在字符串中第一次出现处的索引,如果此字符串中没有这样的字符,则返回 -1。
public int indexOf(int ch, int fromIndex): 返回从 fromIndex 位置开始查找指定字符在字符串中第一次出现处的索引,如果此字符串中没有这样的字符,则返回 -1。
int indexOf(String str): 返回指定字符在字符串中第一次出现处的索引,如果此字符串中没有这样的字符,则返回 -1。
int indexOf(String str, int fromIndex): 返回从 fromIndex 位置开始查找指定字符在字符串中第一次出现处的索引,如果此字符串中没有这样的字符,则返回 -1。
String string = "aaa456ac";
// 从第四个字符位置开始往后继续查找,包含当前位置
System.out.println(string.indexOf("a",3));//indexOf(String str, int fromIndex); 返回结果:6
length():返回字符串长度,空字符串的长度为0;
matches():检测字符串是否匹配给定的正则表达式
String Str = new String("www.runoob.com");
System.out.print("返回值 :" );
System.out.println(Str.matches("(.*)google(.*)")); //false 匹配.google.的字符串
System.out.print("返回值 :" );
System.out.println(Str.matches("www(.*)")); //true 匹配www.的字符串
regionMatches():检测两个字符串在一个区域内是否相等
String Str1 = new String("www.runoob.com");
String Str2 = new String("runoob");
String Str3 = new String("RUNOOB");
System.out.print("返回值 :" );
System.out.println(Str1.regionMatches(4, Str2, 0, 5)); //true
System.out.print("返回值 :" );
System.out.println(Str1.regionMatches(4, Str3, 0, 5)); //false
System.out.print("返回值 :" );
System.out.println(Str1.regionMatches(true, 4, Str3, 0, 5)); //true(检测Stri1中的第4个字符开始,偏移量为0,比较5个字符,true不区分大小写,是否和Str3一样;
replace():替换;返回替换后生成的新字符串
String Str = new String("Runoob");
System.out.println(Str.replace('o', 'T')); //RunTTb (把Str字符串所有的o替换成T
replaceAll():替换;成功则返回替换的字符串,失败则返回原始字符串
String Str = new String("www.google.com");
System.out.println(Str.replaceAll("(.*)google(.*)", "runoob" )); //runoob(把Str符合(.*)google(.*)的替换成runoob;)
replaceFirst():替换;成功则返回替换的字符串,失败则返回原始字符串
String Str = new String("hello runoob,I am from runoob。");
System.out.println(Str.replaceFirst("(.*)runoob(.*)", "google" )); //google(把Str符合(.*)runoob(.*)的第一个字符串替换成google;)
split():根据匹配给定的正则表达式来拆分字符串
注意: . 、 $、 | 和 * 等转义字符,必须得加 \\。
注意:多个分隔符,可以用 | 作为连字符。
返回字符串数组
String str = new String("Welcome-to-Runoob");
for (String retval: str.split("-")){ //根据Str字符串的-分割字符
System.out.println(retval); //Welcome to Runoob
}
for (String retval: str.split("-", 2)){ //根据Str字符串的-分割字符,分割成两个字符,即只找前面一次-分割
System.out.println(retval); //Welcome to-Runoob
}
String str2 = new String("www.runoob.com");
for (String retval: str2.split("\\.", 3)){ //根据Str字符串的.分割字符,分割成三个字符,即只找前面两个.分割
System.out.println(retval); //www runoob com
}
String str3 = new String("acount=? and uu =? or n=?");
for (String retval: str3.split("and|or")){ //根据Str字符串的and或or分割字符
System.out.println(retval); //acount=? uu =? n=?
}
startsWith():检测字符串是否以指定的前缀开始
String Str = new String("www.runoob.com");
System.out.println(Str.startsWith("www") ); //true 字符串Str是否以www开始
System.out.println(Str.startsWith("runoob", 4) ); //true 字符串Str是否在第四个字符开始,以runoob开始
subSequence():截取
String Str = new String("www.runoob.com");
System.out.println(Str.subSequence(4, 10) ); //runoob 返回Str中第4到第10的字符,开始包含,结束不包含
substring():截取
String Str = new String("This is text");
System.out.println(Str.substring(4) ); //is text 返回字符串Str中的第4个字符开始到最后一个(包含第四个)
System.out.println(Str.substring(4, 10) ); //is te 返回字符串Str中的第4个字符开始到第10给结束(包含第四个,不包含第10个)
toCharArray():将字符串转换为字符数组,没有参数
String Str = new String("www.runoob.com");
System.out.println( Str.toCharArray() ); //把Str转为字符数组
toLowerCase():字符串转为小写
String Str = new String("TTT");
System.out.println( Str.toLowerCase() ); //TTT 字符串Str转为小写
toUpperCase():字符串小写字符转换为大写
String Str = new String("ttt");
System.out.println( Str.toUpperCase() ); //ttt 字符串Str转为大写
toString():返回此对象本身(它已经是一个字符串)
String Str = new String("WWW.RUNOOB.COM");
System.out.println( Str.toString() ); //WWW.RUNOOB.COM
trim():删除字符串头尾空白符
String Str = new String(" www.runoob.com ");
System.out.println( Str.trim() ); //www.runoob.com 删除Str首尾空白符
valueOf():
double d = 1100.00;
boolean b = true;
long l = 1234567890;
char[] arr = {'r', 'u', 'n', 'o', 'o', 'b' };
System.out.println("返回值 : " + String.valueOf(d) ); //1100.0
System.out.println("返回值 : " + String.valueOf(b) ); //true
System.out.println("返回值 : " + String.valueOf(l) ); //1234567890
System.out.println("返回值 : " + String.valueOf(arr) ); //runoob
contains():判断字符串中是否包含指定的字符或字符串;包含返回 true,否则返回 false
String myStr = "Runoob";
System.out.println(myStr.contains("Run")); //true
System.out.println(myStr.contains("s")); //false
isEmpty():判断字符串是否为空
字符串为空返回 true,否则返回 false(字符串通过 length() 方法计算字符串长度,如果返回 0,即为空字符串)
String myStr1 = "Runoob";
String myStr2 = ""; // 空字符串
String myStr3 = " "; // 多个空格,length() 不为 0
System.out.println("myStr1 是否为空:" + myStr1.isEmpty()); // myStr1 是否为空:false
System.out.println("myStr2 是否为空:" + myStr2.isEmpty()); //myStr2 是否为空:true
System.out.println("myStr3 长度:" + myStr3.length()); //myStr3 长度:4
System.out.println("myStr3 是否为空:" + myStr3.isEmpty()); //myStr3 是否为空:false
*/
public class Stringa {
}
|