文档
package queue
type Queue []int
func (queue *Queue) Push(val int) {
*queue = append(*queue, val)
}
func (queue *Queue) Pop() int {
popVal := (*queue)[0]
*queue = (*queue)[1:]
return popVal
}
func (queue *Queue) IsEmpty() bool {
return len(*queue) == 0
}
- 对上面的代码生成文档进行查看;我们主要使用到的是godoc命令
- go 1.13版本后移除了godoc相关的一些命令,因此需要手动安装
-
第一步配置相关代理 go env -w GO111MODULE=on
go env -w GOPROXY="https://goproxy.io,direct"
-
第二步命令行输入,安装godoc go get golang.org/x/tools/cmd/godoc
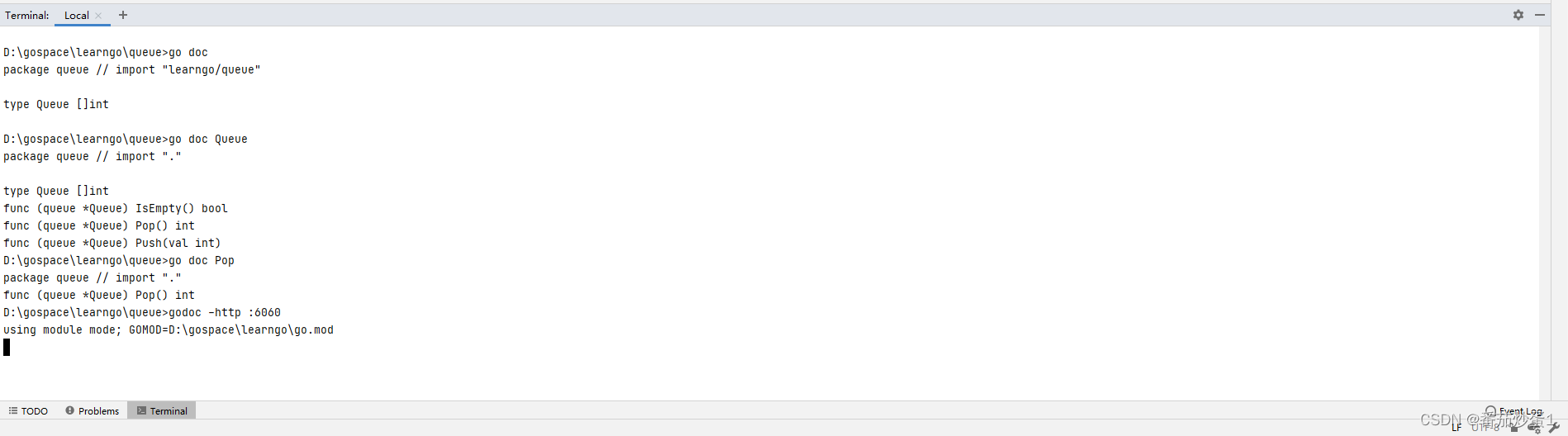 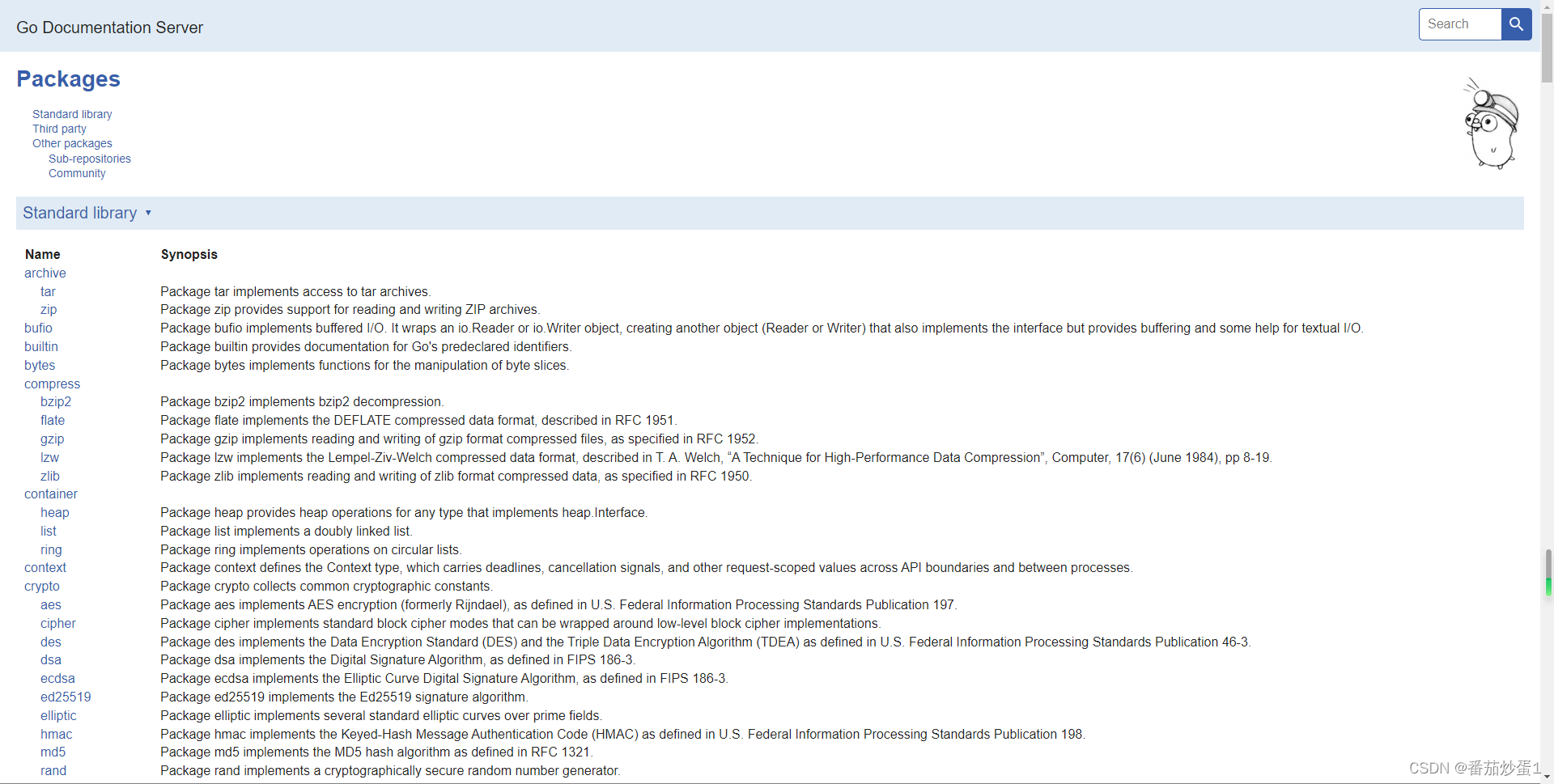
package queue
type Queue []int
func (queue *Queue) Push(val int) {
*queue = append(*queue, val)
}
func (queue *Queue) Pop() int {
popVal := (*queue)[0]
*queue = (*queue)[1:]
return popVal
}
func (queue *Queue) IsEmpty() bool {
return len(*queue) == 0
}
- 随便写一点注释;重新生成文档
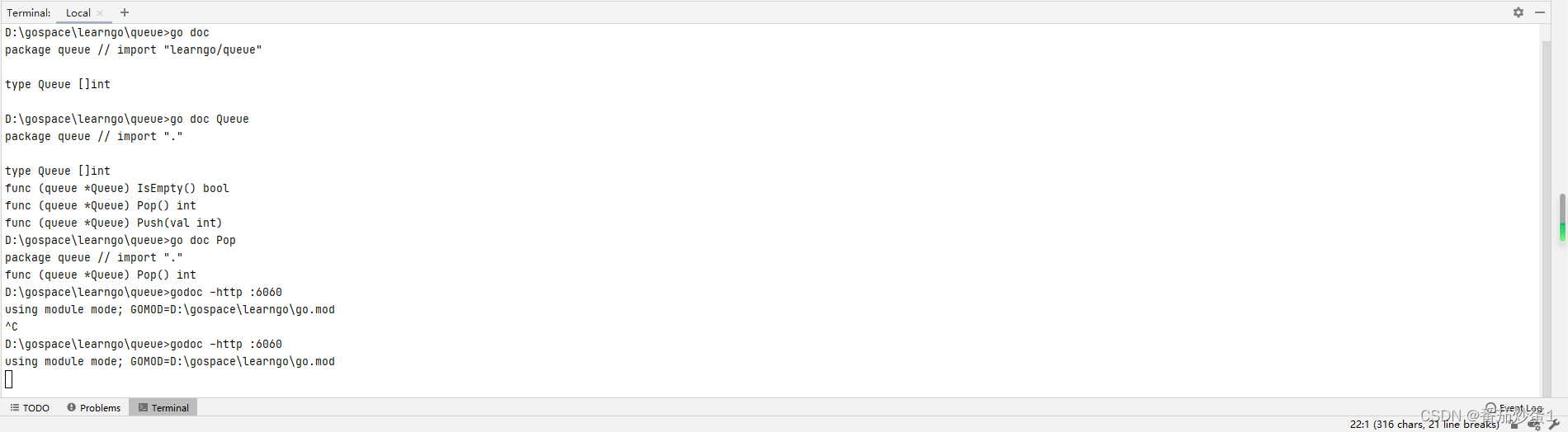 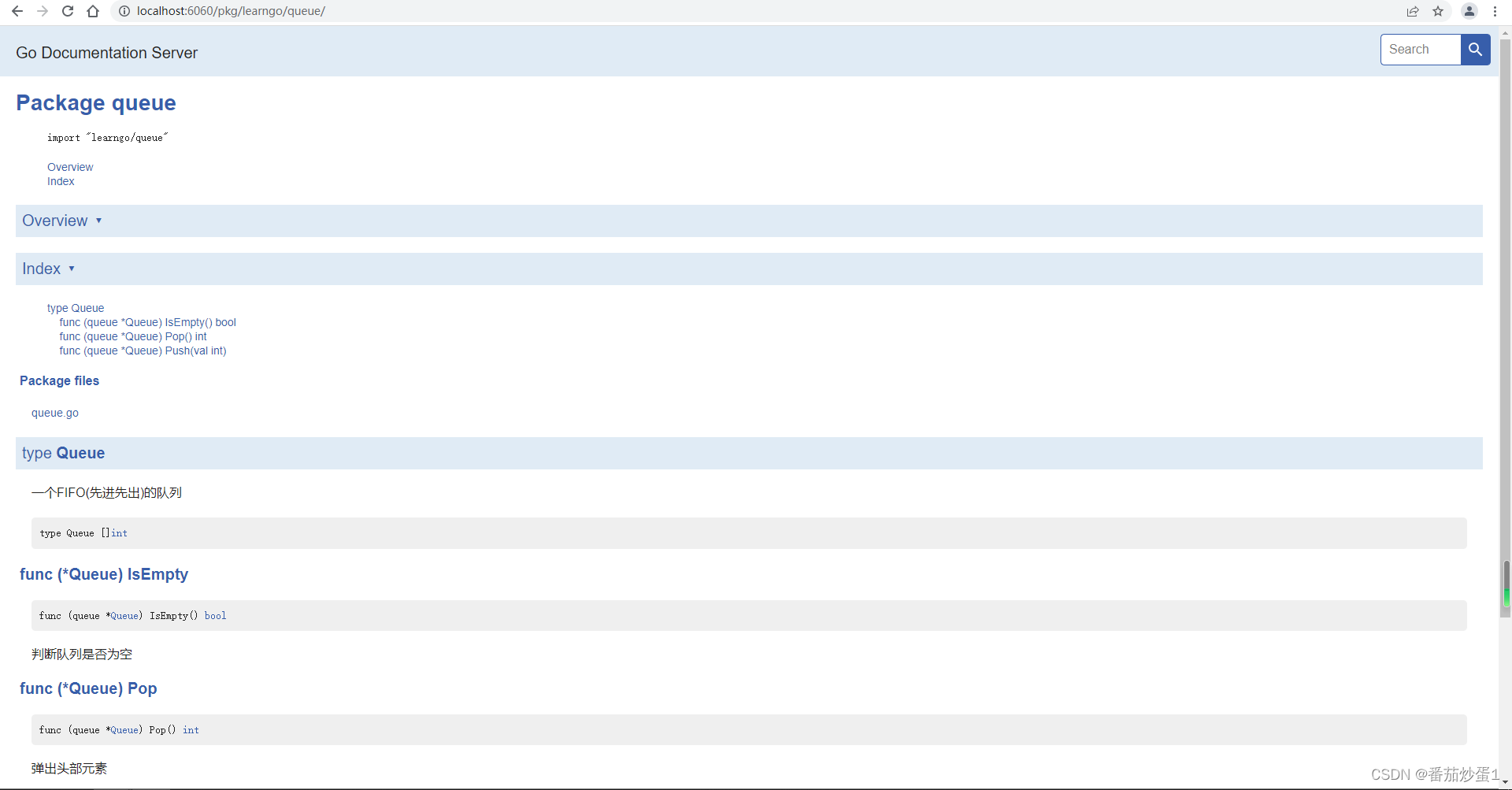
- 再go语言中,我们可以随意的写注释,不想java或者其他的语言,即使是注释也有自己比较复杂的语法
示例代码
package queue
import "fmt"
func ExampleQueue_Push() {
queue := Queue{1}
queue.Push(2)
queue.Push(3)
fmt.Println(queue.Pop())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
}
 我们执行代码的时候没有输出我们想要的结果,返回的却是testing: warning: no tests to run
package queue
import "fmt"
func ExampleQueue_Push() {
queue := Queue{1}
queue.Push(2)
queue.Push(3)
fmt.Println(queue.Pop())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
}
- 使用OutPut:把结果都注释再代码下面;就可以正常输出了
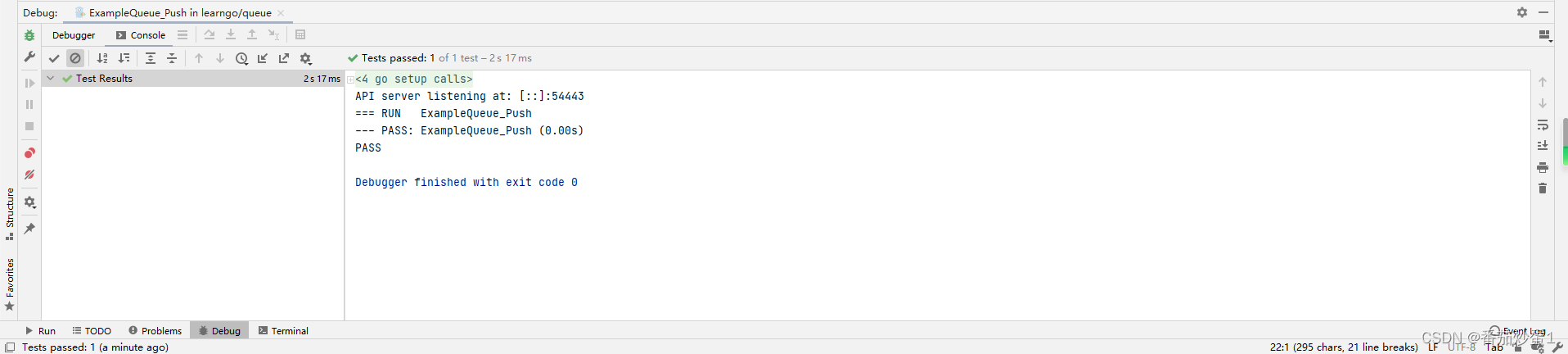 - 故意写错一个答案试一下
package queue
import "fmt"
func ExampleQueue_Push() {
queue := Queue{1}
queue.Push(2)
queue.Push(3)
fmt.Println(queue.Pop())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
fmt.Println(queue.Pop())
fmt.Println(queue.IsEmpty())
}
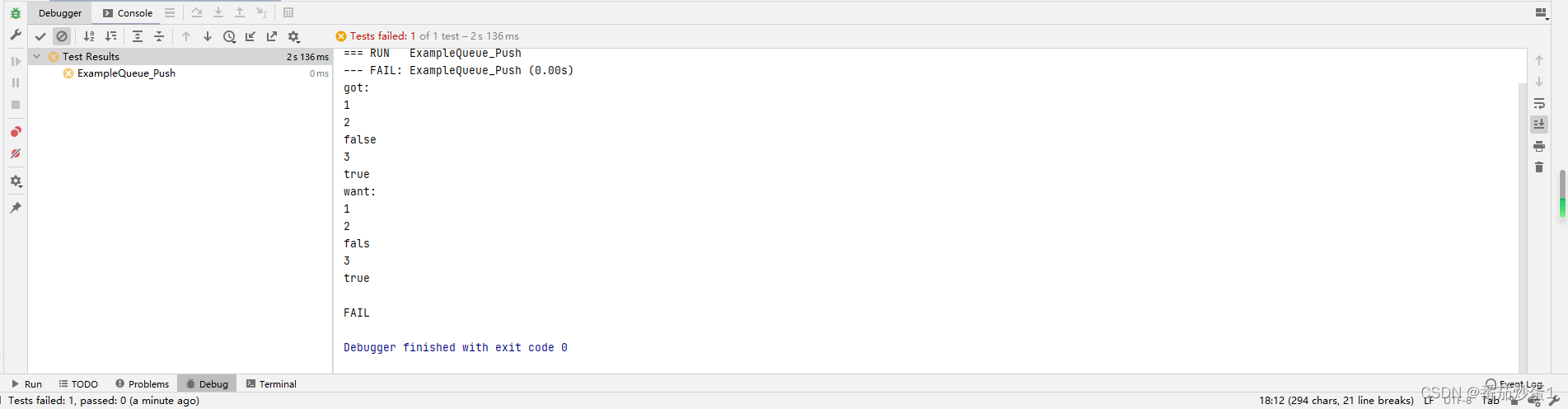
go语言的示例代码,不仅可以执行;而且还会检查一下你的期望值是否跟结果一样;如果不一样会有反馈;示例代码也可以作为测试,但是不仅仅是测试;还可以作为用户的example
文档&示例代码
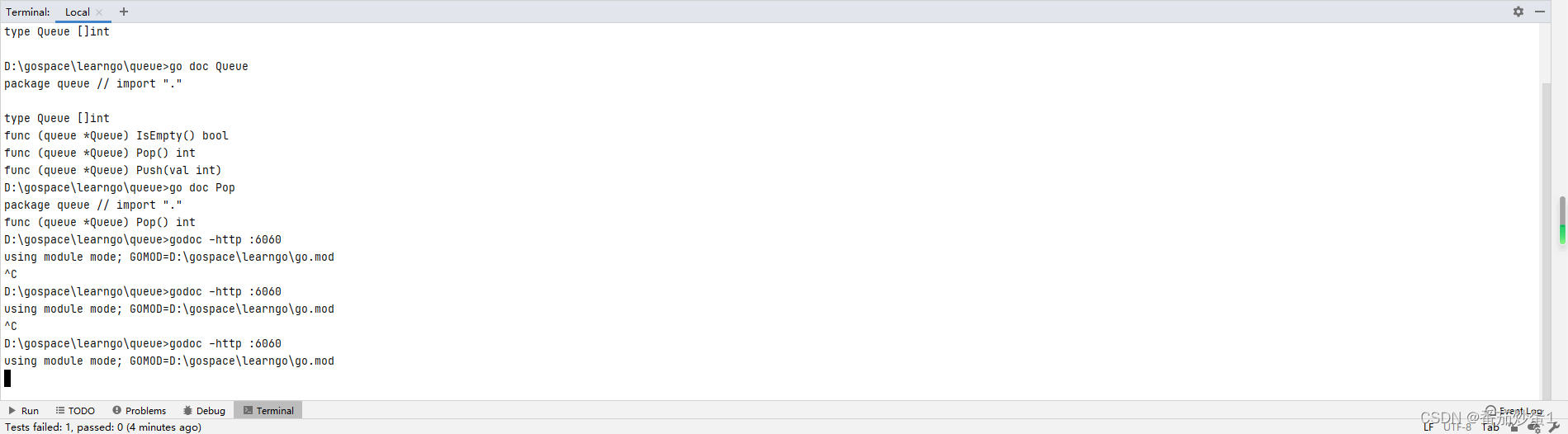 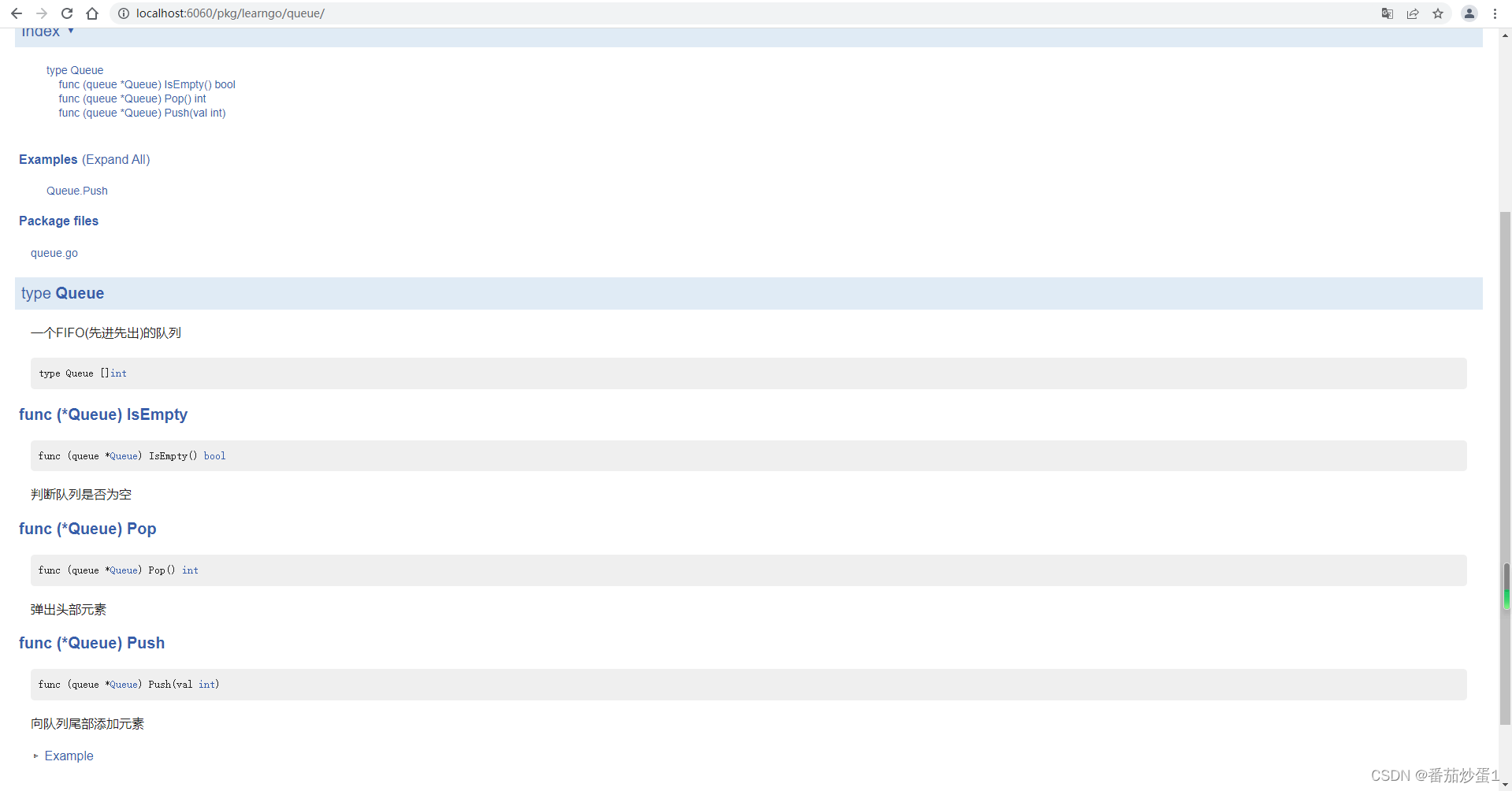 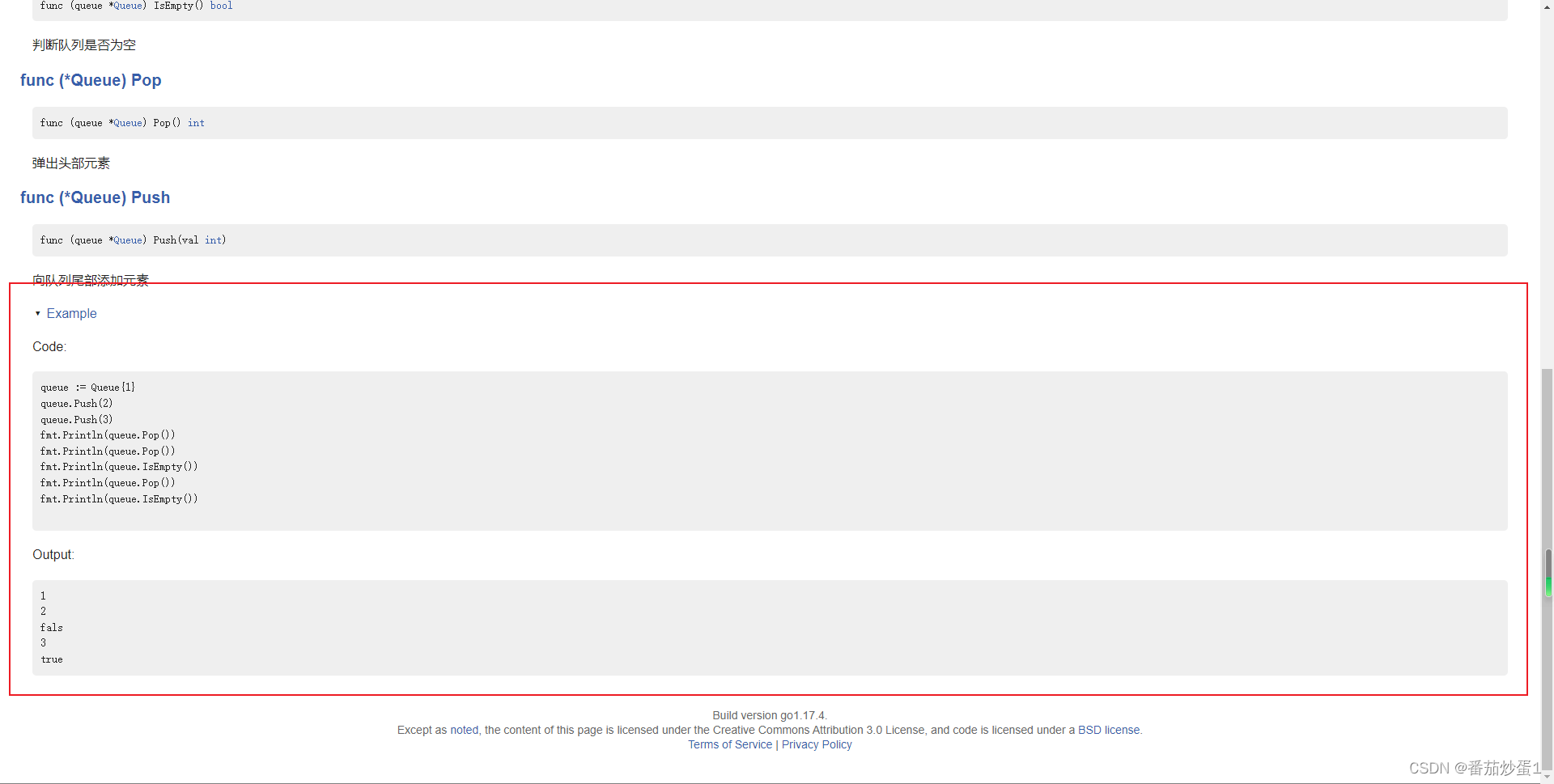
- 用注释写文档
- 再测试中加入Example
- 使用go doc/godoc来查看/生成文档
|