?????????Elasticsearch是一个开源的高扩展的分布式全文检索引擎,它可以近乎实时的存储、检索数据;本身扩展性很好,可以扩展到上百台服务器,处理PB级别的数据。? Elasticsearch也使用Java开发并使用Lucene作为其核心来实现所有索引和搜索的功能,但是它的目的是通过简单的RESTful API来隐藏Lucene的复杂性,从而让全文搜索变得简单。
1.安装Elasticsearch服务并进行相关配置,启动Elasticsearch服务
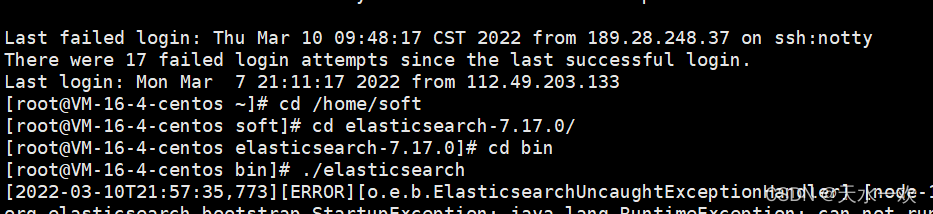
2. 安装Elasticsearch Header? 可视查看相关记录信息
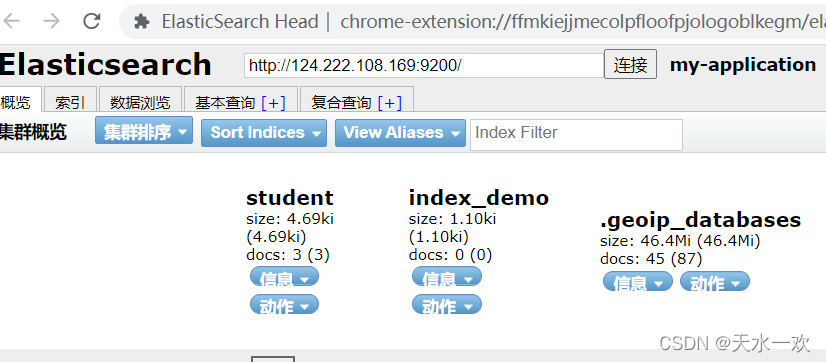
3. pom.xml
<!-- elasticsearch -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>io.searchbox</groupId>
<artifactId>jest</artifactId>
</dependency
1.application.yml
spring:
elasticsearch:
jest:
uris:
- http://124.222.108.169:9200
read-timeout: 5000
2.StudentController
**
*
*/
@Slf4j
@RestController
@RequestMapping("/student")
@Api(tags = "学生信息")
public class StudentController {
@Autowired
private StudentService studentService;
@ApiOperation(value = "保存学生数据")
@RequestMapping(path="/saveStudent")
public JSONResult saveStudent( HttpServletRequest request, HttpServletResponse response){
List<Student> list=new ArrayList<Student>();
Student student=new Student( 1L,"张三",5);
list.add(student);
student=new Student( 2L,"王五",8);
list.add(student);
student=new Student( 4L,"赵六",10);
list.add(student);
studentService.saveEntity(list);
return JSONResult.ok();
}
@ApiOperation(value = "删除学生数据")
@RequestMapping(path="/delStudent")
public JSONResult delStudent( HttpServletRequest request, HttpServletResponse response){
String id=request.getParameter("id");
studentService.delEntity(id);
return JSONResult.ok();
}
@ApiOperation(value = "搜索")
@RequestMapping(path="/searchStudent")
public JSONResult searchStudent( HttpServletRequest request){
List<Student> result=studentService.searchEntity("张");
return JSONResult.ok(result);
}
/**
* 创建索引
* @param indexName
* @throws Exception
*/
@ApiOperation(value = "创建索引")
@RequestMapping(path="/createIndex")
public JSONResult createIndex(@RequestParam("indexName")String indexName) throws Exception{
studentService.createIndex(indexName);
return JSONResult.ok();
}
/**
* 删除索引
* @param indexName
* @throws Exception
*/
@ApiOperation(value = "刪除索引")
@RequestMapping(path="/deleteIndex")
public JSONResult deleteIndex(@RequestParam("indexName")String indexName) throws Exception{
studentService.deleteIndex(indexName);
return JSONResult.ok();
}
}
3.StudentServiceImpl
@Service
public class StudentServiceImpl implements StudentService {
private static final Logger LOGGER = LoggerFactory.getLogger(StudentServiceImpl.class);
@Autowired
private JestClient jestClient;
@Override
public void saveEntity(Student entity) {
Index index = new Index.Builder(entity).index(Student.INDEX_NAME).type(Student.TYPE).build();
try {
jestClient.execute(index);
LOGGER.info("插入完成");
} catch (IOException e) {
e.printStackTrace();
LOGGER.error(e.getMessage());
}
}
@Override
public void delEntity(String id) {
Delete index = new Delete.Builder(id).index(Student.INDEX_NAME).type(Student.TYPE).build();
try {
jestClient.execute(index);
LOGGER.info("删除完成");
} catch (IOException e) {
e.printStackTrace();
LOGGER.error(e.getMessage());
}
}
@Override
public void saveEntity(List<Student> entityList) {
Bulk.Builder bulk = new Bulk.Builder();
for(Student entity : entityList) {
Index index = new Index.Builder(entity).id(String.valueOf(entity.getId())).index(Student.INDEX_NAME).type(Student.TYPE).build();
bulk.addAction(index);
}
try {
jestClient.execute(bulk.build());
LOGGER.info("批量插入完成");
} catch (IOException e) {
e.printStackTrace();
LOGGER.error(e.getMessage());
}
}
/**
* 在ES中搜索内容
*/
@Override
public List<Student> searchEntity(String searchContent){
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.matchQuery("studentName",searchContent));
Search search = new Search.Builder(searchSourceBuilder.toString())
.addIndex(Student.INDEX_NAME).addType(Student.TYPE).build();
try {
JestResult result = jestClient.execute(search);
return result.getSourceAsObjectList(Student.class);
} catch (IOException e) {
LOGGER.error(e.getMessage());
e.printStackTrace();
}
return null;
}
/**
* 创建索引
* @param indexName
*
*/
@Override
public void createIndex(String indexName) {
try{
CreateIndex createIndex = new CreateIndex.Builder(indexName).build();
JestResult result = jestClient.execute(createIndex);
LOGGER.info("result",result.getJsonString());
}catch (Exception e){
LOGGER.error(e.getMessage());
e.printStackTrace();
}
}
/**
* 删除索引
* @param indexName
*
*/
@Override
public void deleteIndex(String indexName) {
try{
DeleteIndex deleteIndex = new DeleteIndex.Builder(indexName).build();
JestResult result = jestClient.execute(deleteIndex);
LOGGER.info("result",result.getJsonString());
}catch (Exception e){
LOGGER.error(e.getMessage());
e.printStackTrace();
}
}
|