前提声明:我有跟着周阳老师来做项目,不是只过来写文章的!!里边有一些踩坑点和配置文件代码,按照b站视频顺序来写的。也不是来授课的。Alibaba的springcloud还没学,那是另一个阶段了。只是按照这篇文章给使用者包括我自己提醒顺序、配置、具体操作等等。墙裂建议没看过的小伙伴还是去学习一下,小白新手很合适!!
B站尚硅谷springcloud视频:
https://www.bilibili.com/video/BV18E411x7eT
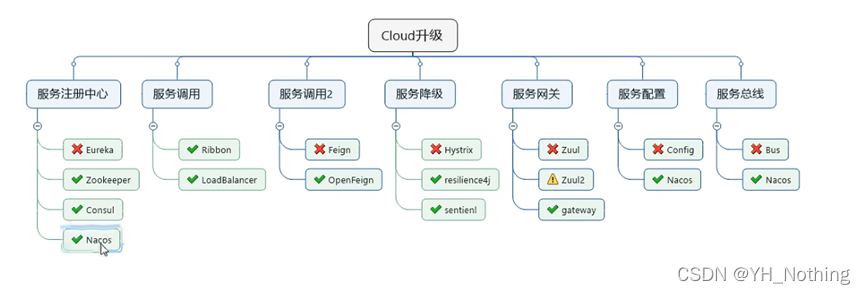 SpringBoot 2.0版和SpringCloud H版 强烈建议使用SpringBoot 2.0以上
SpringBoot和SpringCloud之间版本有约束 H版对应2.2 G版对应2.1
总工程创建步骤
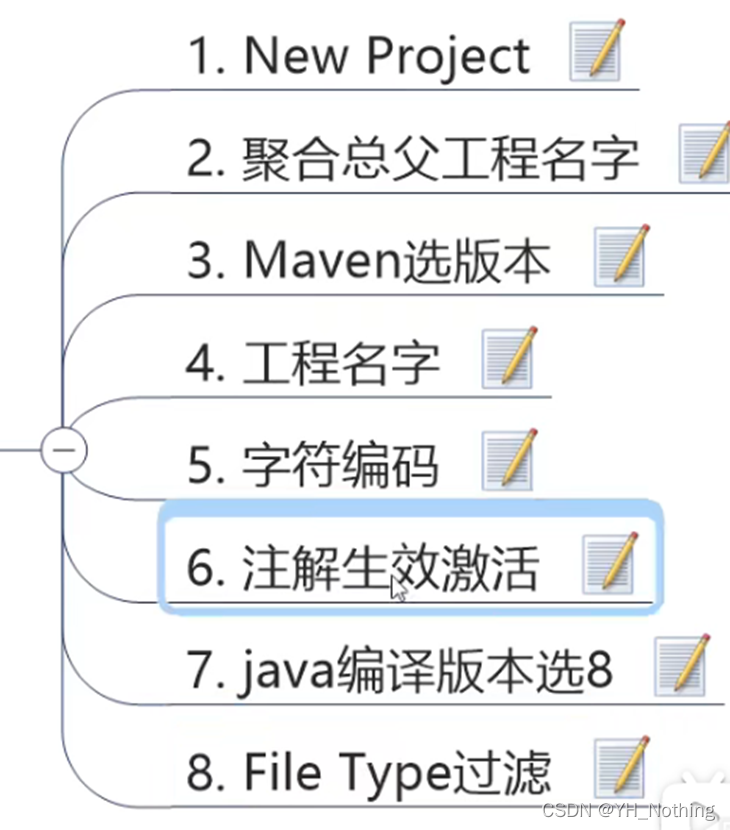
注册中心:Eureka
(貌似还有个在电脑host文件中添加修改的操作,但是具体是啥我忘了,配置了两个ip指向eureka7001.com:7001和eureka7002.com:7002)
依赖(父模块版本已经引入了,不会报错,这里单写展示一下)
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
<version>2.2.1.RELEASE</version>
</dependency>
添加yml配置文件
server:
port: 7001
eureka:
instance:
hostname: eureka7001.com
client:
register-with-eureka: false
fetch-registry: false
service-url:
defaultZone: http://eureka7001.com:7001/eureka/
编写启动类
@SpringBootApplication
@EnableEurekaServer
public class EurekaMain7001 {
public static void main(String[] args) {
SpringApplication.run(EurekaMain7001.class,args);
}
}
提供者模块
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
server:
port: 8001
spring:
application:
name: cloud-payment-service
zipkin:
base-url: http://localhost:9411
sleuth:
sampler:
probability: 1
datasource:
type: com.alibaba.druid.pool.DruidDataSource
driver-class-name: org.gjt.mm.mysql.Driver
url: jdbc:mysql://localhost:3306/db2019?useUnicode=true&characterEncoding=utf-8&useSSL=false
username: root
password: 123456
eureka:
client:
register-with-eureka: true
fetchRegistry: true
service-url:
defaultZone: http://localhost:7001/eureka
instance:
instance-id: payment8001
prefer-ip-address: true
mybatis:
mapperLocations: classpath:mapper/*.xml
type-aliases-package: com.atguigu.springcloud.entities
启动类
@EnableEurekaClient
消费者模块
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
server:
port: 80
spring:
application:
name: cloud-order-service
zipkin:
base-url: http://localhost:9411
sleuth:
sampler:
probability: 1
eureka:
client:
register-with-eureka: false
fetchRegistry: true
service-url:
defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka
动态获取已经注册进注册中心的服务端口
public static final String PAYMENT_URL = "http://CLOUD-PAYMENT-SERVICE";
启动类
@EnableEurekaClient
devtool热部署
子工程xml文件
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
父工程pom.xml文件
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<fork>true</fork>
<addResources>true</addResources>
</configuration>
</plugin>
</plugins>
</build>
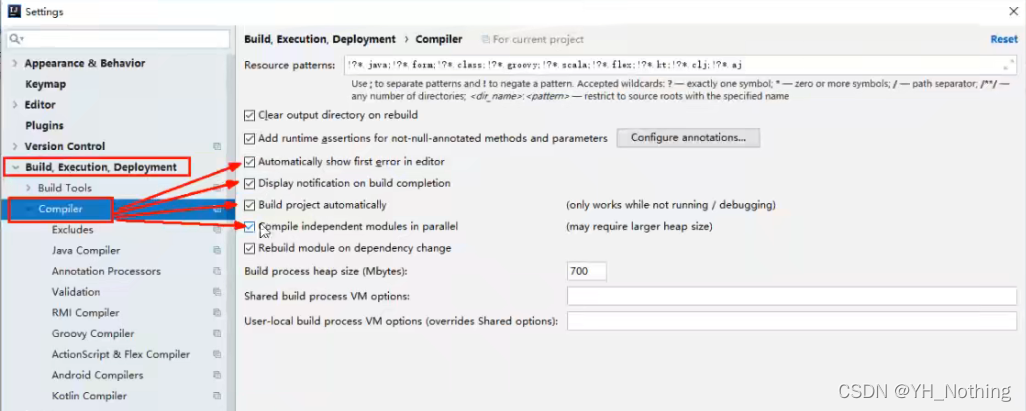 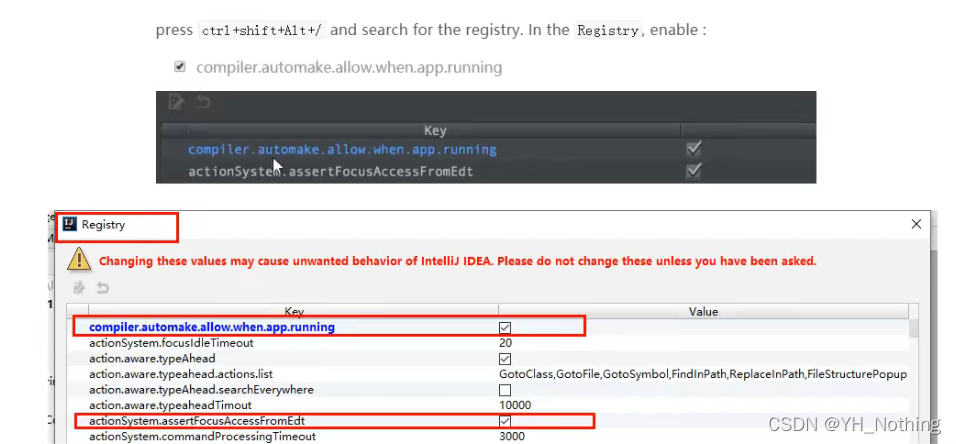 顺序不一样,找一找 重启IDEA
自定义api通用包
引入自己定义的api通用包,可以使用Payment支付Entity 把相同实体类取出来放进统一管理的这个包内,不用每个子工程但凡用到都要去写一份相同的实体类 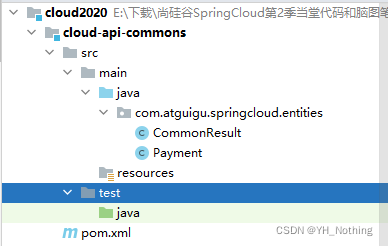 两个groupId要对应,这里边我写自己代码的时候出错了一直爆红!
<parent>
<groupId>com.atguigu.springcloud</groupId>
<artifactId>cloud2020</artifactId>
<version>1.0-SNAPSHOT</version>
</parent>
<dependency>
<groupId>com.atguigu.springcloud</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
还有一个点,但凡遇到后边别的子工程或者业务或者配置文件yaml/xml用到关于实体类的,一定要看好自己的引用包名和这个创建的统一管理子工程实体的包名,切记要对应!!
zookeeper和consul
原理和eureka一样,只是消费者提供者注册中心三角图原先eureka的地方换成相对应的注册中心即可
Ribbon
80消费者 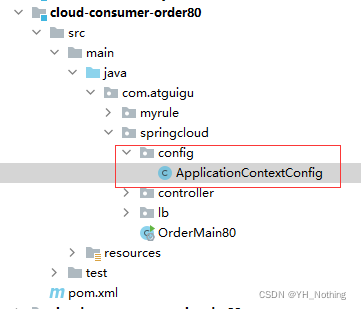
@Configuration
public class ApplicationContextConfig
{
@Bean
@LoadBalanced
public RestTemplate getRestTemplate()
{
return new RestTemplate();
}
}
也可以自定义规则 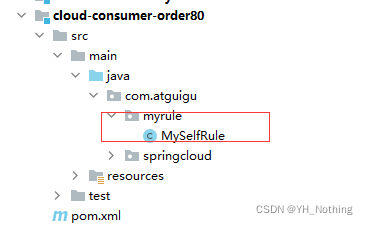
@Configuration
public class MySelfRule
{
@Bean
public IRule myRule()
{
return new RandomRule();
}
}
openFeign
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
server:
port: 80
eureka:
client:
register-with-eureka: false
service-url:
defaultZone: http://eureka7001.com:7001/eureka/,http://eureka7002.com:7002/eureka/
ribbon:
ReadTimeout: 5000
ConnectTimeout: 5000
logging:
level:
com.atguigu.springcloud.service.PaymentFeignService: debug
启动类
@EnableFeignClients
监控日志,定义配置类 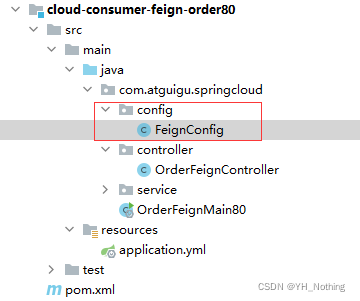
@Configuration
public class FeignConfig
{
@Bean
Logger.Level feignLoggerLevel()
{
return Logger.Level.FULL;
}
}
Hystrix
服务降级
:有兜底解决方案,返回一个友好提示,而不是把错误代码返回给用户
提供者
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
server:
port: 8001
spring:
application:
name: cloud-provider-hystrix-payment
eureka:
client:
register-with-eureka: true
fetch-registry: true
service-url:
defaultZone: http://eureka7001.com:7001/eureka
业务层:fallbackMethod里边标明的方法给所注释的方法兜底
@HystrixCommand(fallbackMethod = "paymentInfo_TimeOutHandler",commandProperties = {
@HystrixProperty(name="execution.isolation.thread.timeoutInMilliseconds",value="5000")
})
public String paymentInfo_TimeOut(Integer id)
{
try { TimeUnit.MILLISECONDS.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); }
return "线程池: "+Thread.currentThread().getName()+" id: "+id+"\t"+"O(∩_∩)O哈哈~"+" 耗时(秒): ";
}
public String paymentInfo_TimeOutHandler(Integer id)
{
return "线程池: "+Thread.currentThread().getName()+" 8001系统繁忙或者运行报错,请稍后再试,id: "+id+"\t"+"o(╥﹏╥)o";
}
消费者
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
server:
port: 80
eureka:
client:
register-with-eureka: false
service-url:
defaultZone: http://eureka7001.com:7001/eureka/
feign:
hystrix:
enabled: true
@SpringBootApplication
@EnableFeignClients
@EnableHystrix
interface PaymentHystrixService业务层
@Component
@FeignClient(value = "CLOUD-PROVIDER-HYSTRIX-PAYMENT")
public interface PaymentHystrixService
{
@GetMapping("/payment/hystrix/ok/{id}")
public String paymentInfo_OK(@PathVariable("id") Integer id);
@GetMapping("/payment/hystrix/timeout/{id}")
public String paymentInfo_TimeOut(@PathVariable("id") Integer id);
}
controller业务层:fallbackMethod里边标明的方法给所注释的方法兜底
@HystrixCommand(fallbackMethod = "paymentTimeOutFallbackMethod",commandProperties = {
@HystrixProperty(name="execution.isolation.thread.timeoutInMilliseconds",value="1500")
})
public String paymentInfo_TimeOut(@PathVariable("id") Integer id)
{
int age = 10/0;
String result = paymentHystrixService.paymentInfo_TimeOut(id);
return result;
}
public String paymentTimeOutFallbackMethod(@PathVariable("id") Integer id)
{
return "我是消费者80,对方支付系统繁忙请10秒钟后再试或者自己运行出错请检查自己,o(╥﹏╥)o";
}
不能一百个方法一百个注解一百个兜底方法哎,所以就有全局兜底fallback方法,别的没添加注解的默认走这个兜底方法降级
@DefaultProperties(defaultFallback = "payment_Global_FallbackMethod")
public class OrderHystirxController
{
...
public String payment_Global_FallbackMethod()
{
return "Global异常处理信息,请稍后再试,/(ㄒoㄒ)/~~";
}
}
仔细观察上边都是controller引入service接口调用里边的方法,兜底都是在controller里边自己写,但是不能调用一次service接口方法写一遍兜底啊,所以写了个service接口实现类来实现这个接口,定义兜底 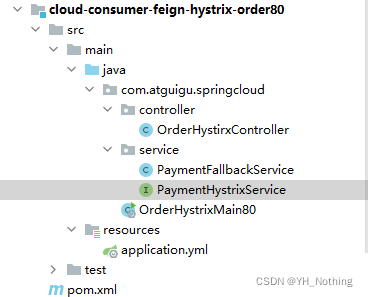
@Component
public class PaymentFallbackService implements PaymentHystrixService
{
@Override
public String paymentInfo_OK(Integer id)
{
return "-----PaymentFallbackService fall back-paymentInfo_OK ,o(╥﹏╥)o";
}
@Override
public String paymentInfo_TimeOut(Integer id)
{
return "-----PaymentFallbackService fall back-paymentInfo_TimeOut ,o(╥﹏╥)o";
}
}
fallback = PaymentFallbackService.class 调用我接口里边的方法不去在controller再写兜底,出问题根据fallback = PaymentFallbackService.class找这个PaymentFallbackService类
@FeignClient(value = "CLOUD-PROVIDER-HYSTRIX-PAYMENT" ,fallback = PaymentFallbackService.class)
服务熔断
:如果服务器故障,调用服务多次未响应时,不应该继续调用服务器的服务,增加被调用的服务器压力 启动类的需要额外添加的注解 @EnableCircuitBreaker 提供者:
@SpringBootApplication
@EnableEurekaClient
@EnableCircuitBreaker
public class PaymentHystrixMain8001
{
public static void main(String[] args) {
SpringApplication.run(PaymentHystrixMain8001.class, args);
}
}
业务层(@HystrixCommand类似于swagger的注解,写在方法上边)
@HystrixCommand(fallbackMethod = "paymentCircuitBreaker_fallback",commandProperties = {
@HystrixProperty(name = "circuitBreaker.enabled",value = "true"),
@HystrixProperty(name = "circuitBreaker.requestVolumeThreshold",value = "10"),
@HystrixProperty(name = "circuitBreaker.sleepWindowInMilliseconds",value = "10000"),
@HystrixProperty(name = "circuitBreaker.errorThresholdPercentage",value = "60"),
})
豪猪哥监控图形化界面::还有一个配置:这边是豪猪哥图形化监控界面需要的设置,具体原因看下边,说是springcloud的一个问题,不兼容还是咋地,需要手动配置
@Bean
public ServletRegistrationBean getServlet() {
HystrixMetricsStreamServlet streamServlet = new HystrixMetricsStreamServlet();
ServletRegistrationBean registrationBean = new ServletRegistrationBean(streamServlet);
registrationBean.setLoadOnStartup(1);
registrationBean.addUrlMappings("/hystrix.stream");
registrationBean.setName("HystrixMetricsStreamServlet");
return registrationBean;
}
}
新建项目豪猪哥界面dashboard9001
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix-dashboard</artifactId>
</dependency>
@EnableHystrixDashboard
访问路径 localhost:9001/hystrix
GateWay、Spring Config、Bus消息中心、SpringCloud Sleuth
博客地址 详见b站视频或者上方博客链接 66_GateWay是什么 67_GateWay非阻塞异步模型 68_Gateway工作流程 69_Gateway9527搭建 70_Gateway配置路由的两种方式 71_GateWay配置动态路由 72_GateWay常用的Predicate 73_GateWay的Filter 74_Config分布式配置中心介绍 75_Config配置总控中心搭建 76_Config客户端配置与测试 77_Config动态刷新之手动版 78_Bus消息总线是什么 79_Bus之RabbitMQ环境配置 80_Bus动态刷新全局广播的设计思想和选型 81_Bus动态刷新全局广播配置实现 82_Bus动态刷新定点通知 83_Stream为什么被引入 84_Stream是什么及Binder介绍 85_Stream的设计思想 86_Stream编码常用注解简介 87_Stream消息驱动之生产者 88_Stream消息驱动之消费者 89_Stream之消息重复消费 90_Stream之group解决消息重复消费 91_Stream之消息持久化 92_Sleuth是什么 93_Sleuth之zipkin搭建安装 94_Sleuth链路监控展现
|