1.SimpleDateFormat
主要就是用来规范我们输出的时间日期格式
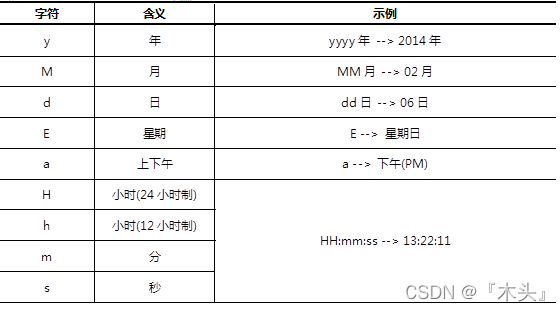
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
2.获取当前时间的毫秒值
Date date = new Date();
System.out.println(date.getTime());
System.out.println(System.currentTimeMillis());
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String current = sdf.format(System.currentTimeMillis());
System.out.println(current);
3.日期转字符串、字符串转日期
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
Calendar calendar = Calendar.getInstance();
Date date = calendar.getTime();
String dateStringParse = sdf.format(date);
System.out.println(dateStringParse);
String dateString = "2022-02-22 22:22:22";
Date dateParse = sdf.parse(dateString);
System.out.println(dateParse);
} catch (ParseException e) {
e.printStackTrace();
}
4.输入时间,转为日期
public static String getFormatBySeconds(long seconds) {
String timeStr = seconds + "秒";
if (seconds > 60) {
long second = seconds % 60;
long min = seconds / 60;
timeStr = min + "分" + second + "秒";
if (min > 60) {
min = (seconds / 60) % 60;
long hour = (seconds / 60) / 60;
timeStr = hour + "小时" + min + "分" + second + "秒";
if (hour > 24) {
hour = ((seconds / 60) / 60) % 24;
long day = (((seconds / 60) / 60) / 24);
timeStr = day + "天" + hour + "小时" + min + "分" + second + "秒";
}
}
}
return timeStr;
}
5.计算时间差
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String previousTime = "2022-01-01 01:01:01";
Calendar calender = Calendar.getInstance();
long currentDate = calendar.getTime().getTime();
try {
long previousDate = sdf.parse(previousTime).getTime();
long betweenDate = (currentDate - previousDate) / (1000 * 60 * 60 * 24);
System.out.print(betweenDate);
} catch (ParseException e) {
e.printStackTrace();
}
|