IOC
Spring 简介
Spring是什么
spring是分层的JavaSE/EE 应用 full-stack 轻量级 开源 框架 框架作用:
- 提高开发效率
- 增强可重用性
- 提供编写规范
- 节约维护成本
- 解耦底层实现原理
Spring优势
- 方便解耦,简化开发
- 方便继承各种优秀框架
- 方便程序的测试
- AOP编程支持
- 声明式事务支持
- 降低JavaEE API的使用难度
- Java原码是经典的学习范例
Spring体系结构
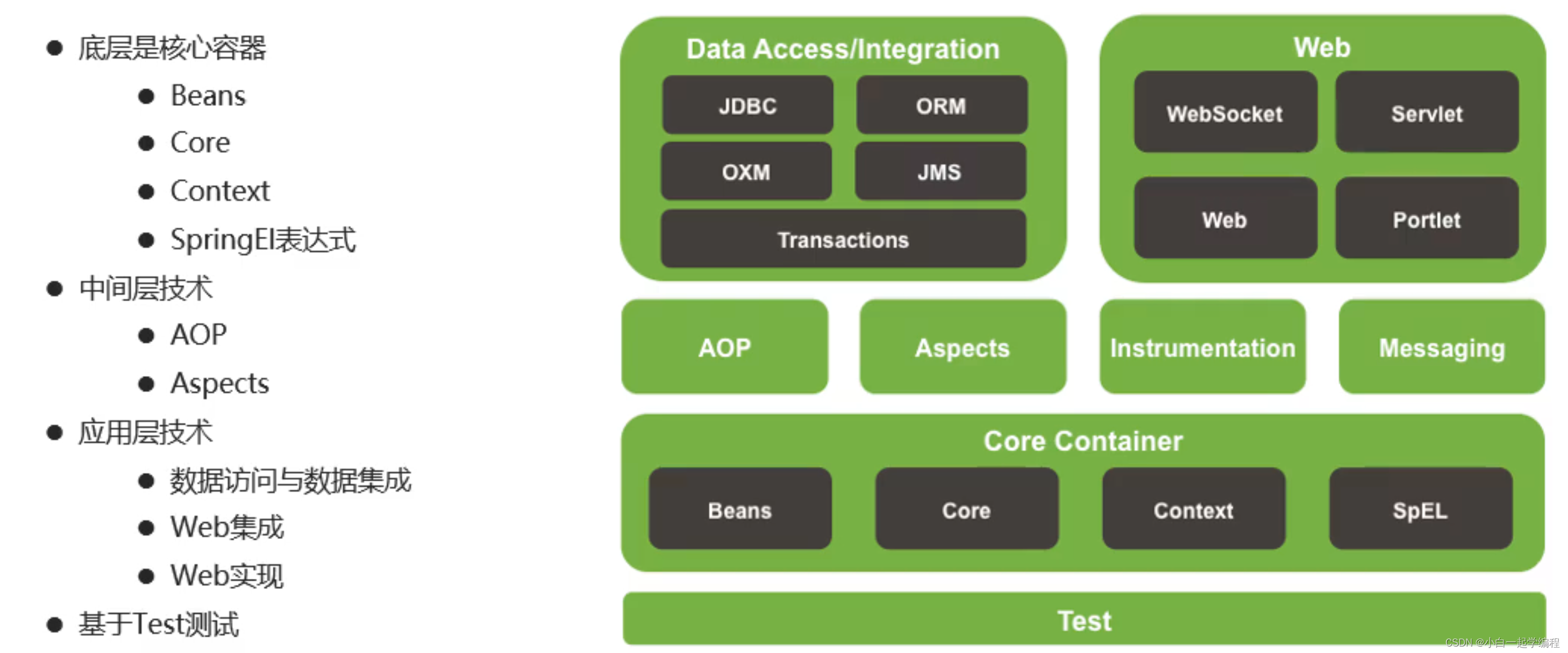
Spring发展
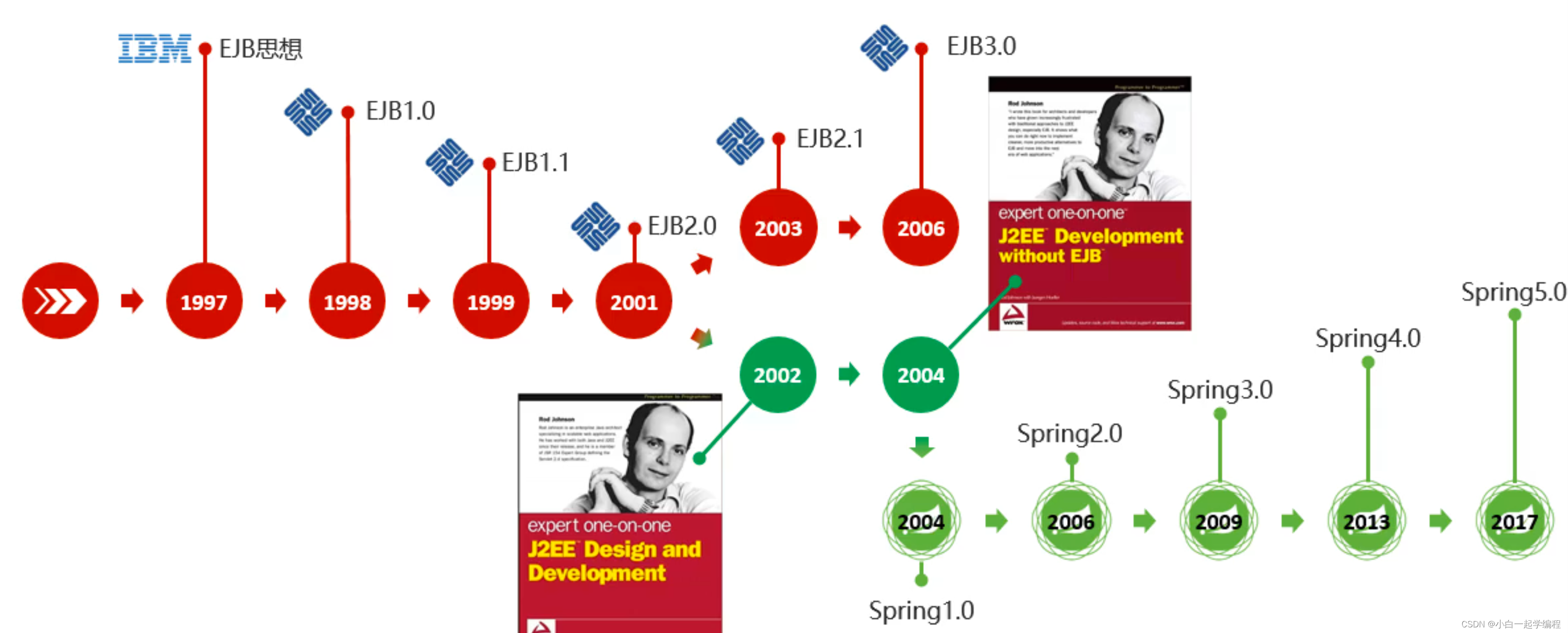
IoC简介
引例
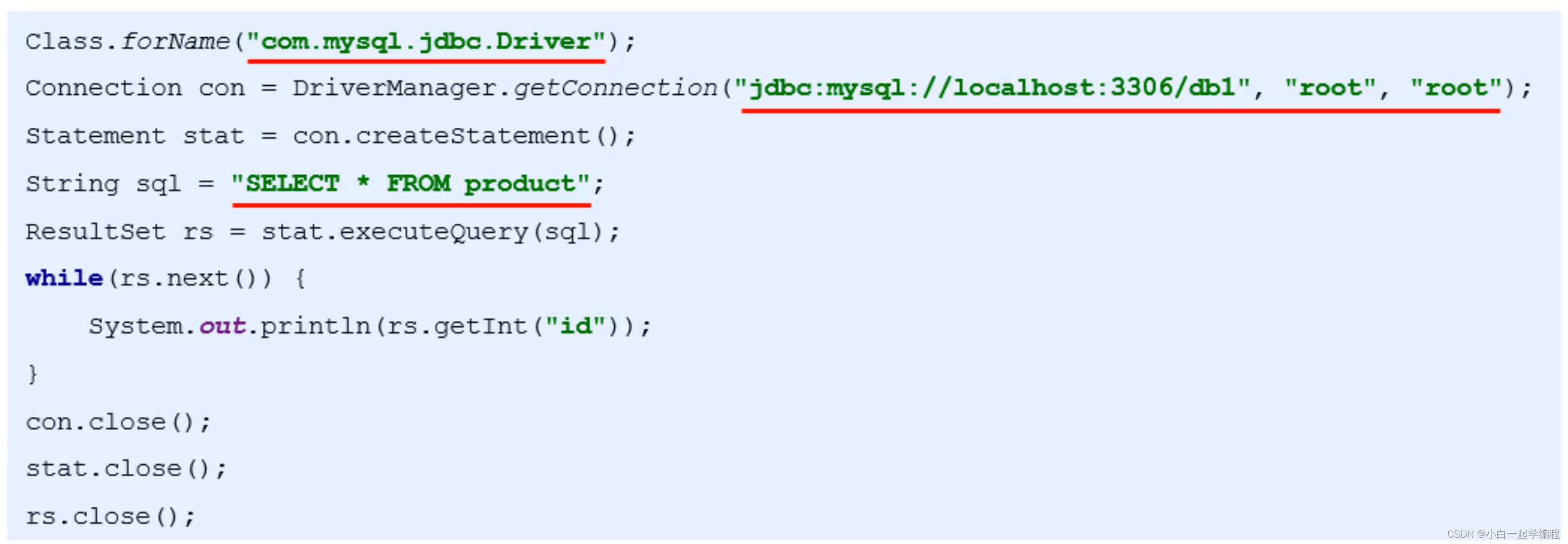 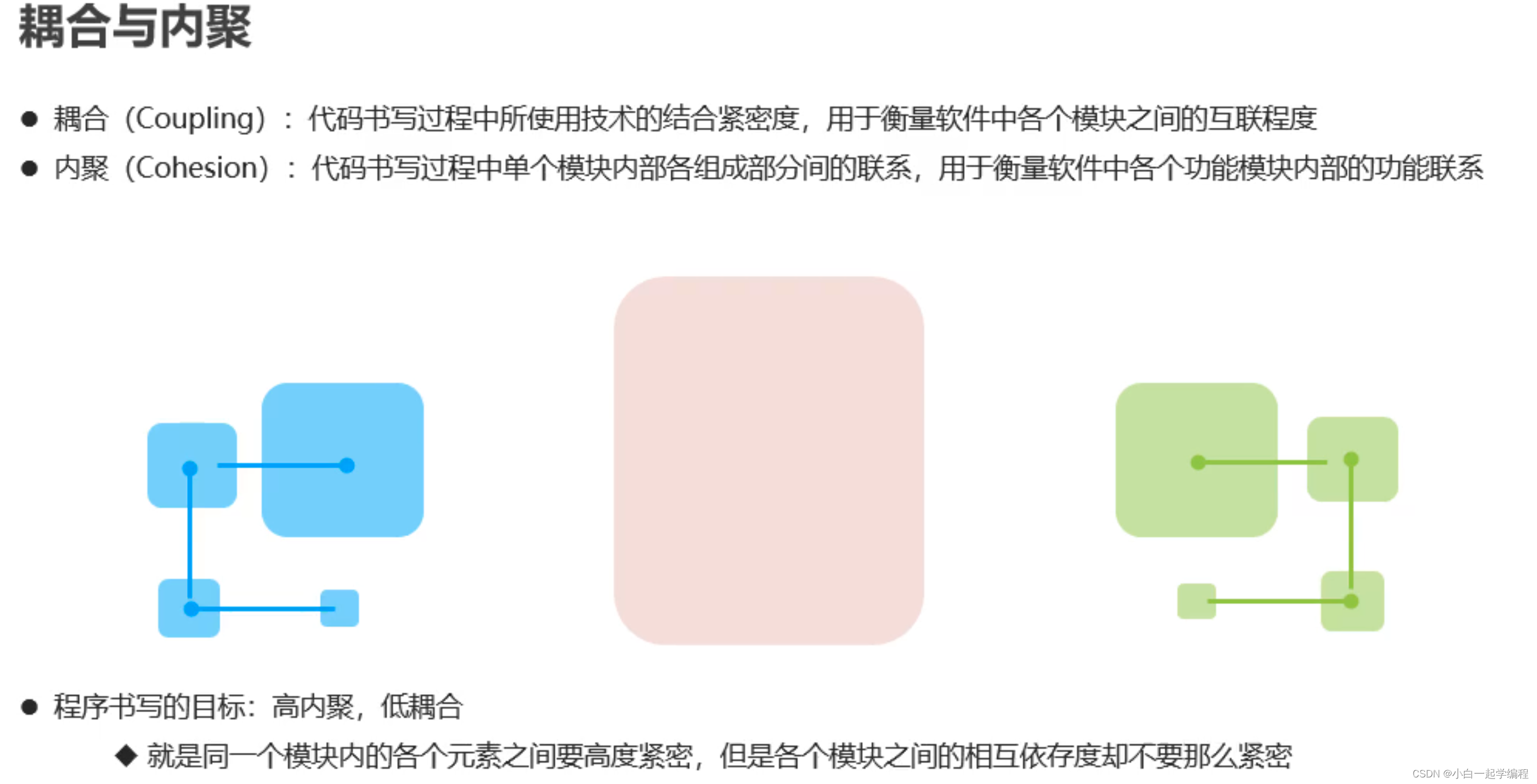 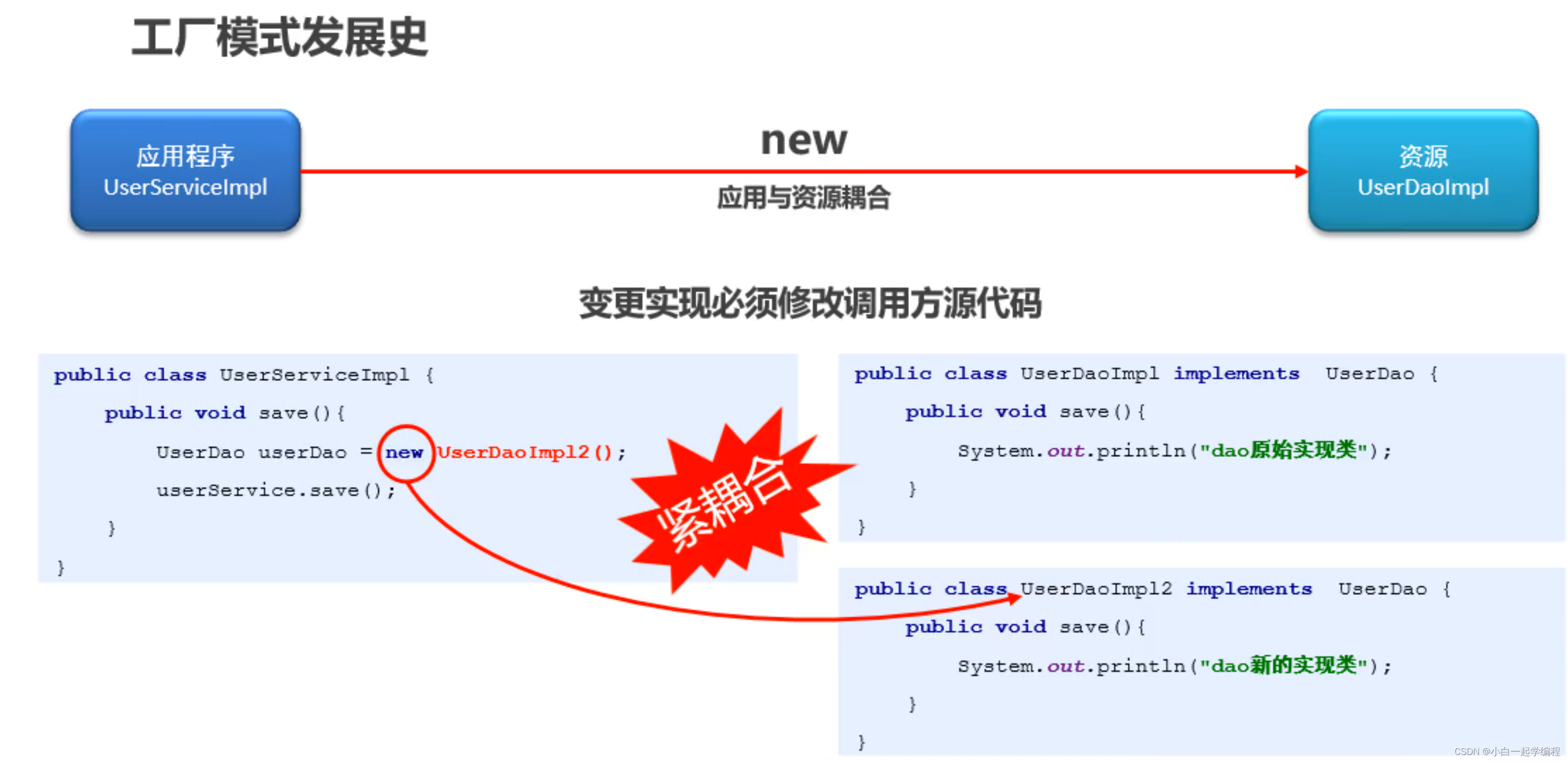
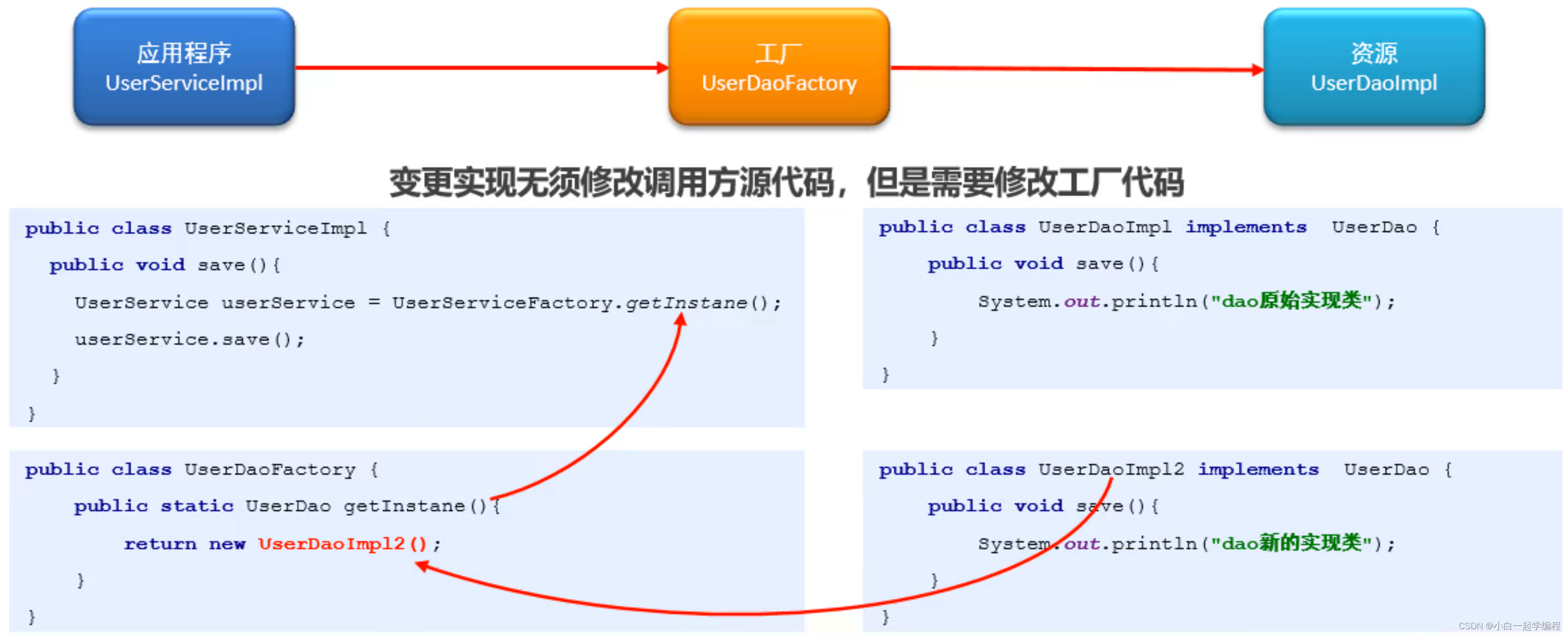 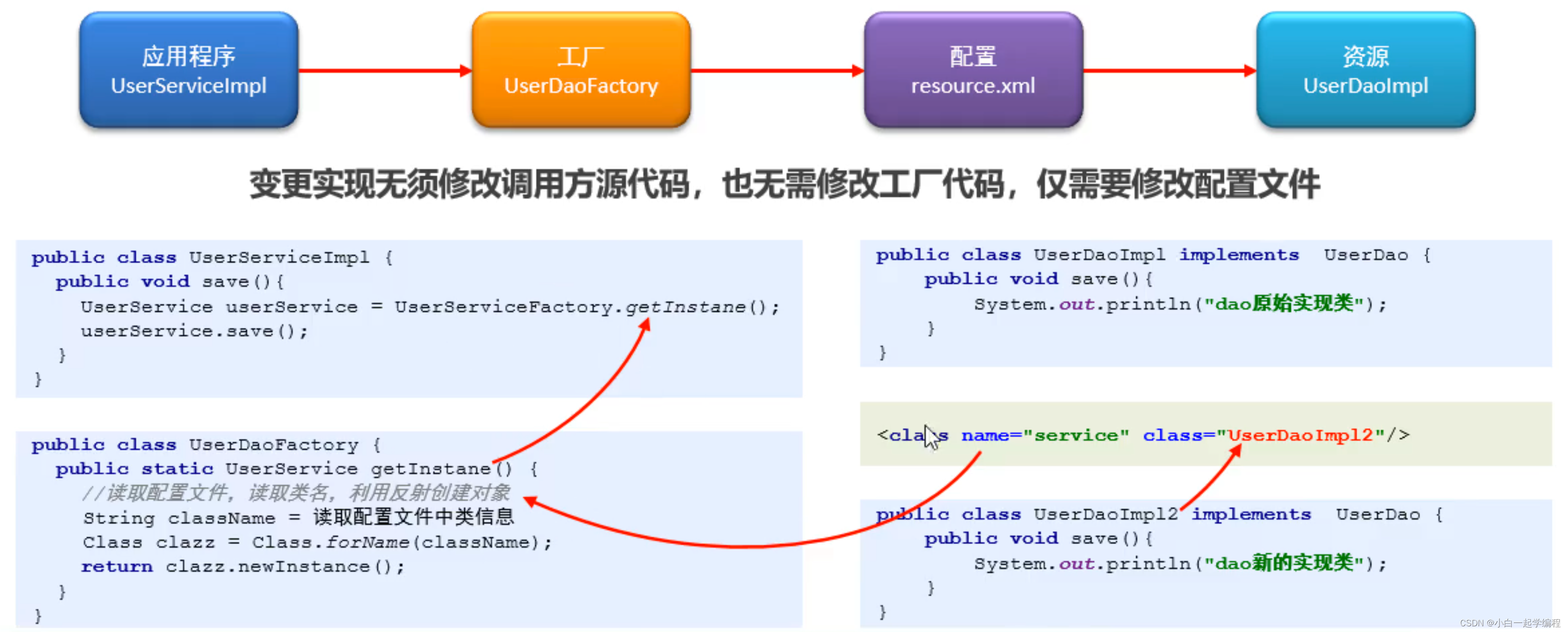
IoC概念
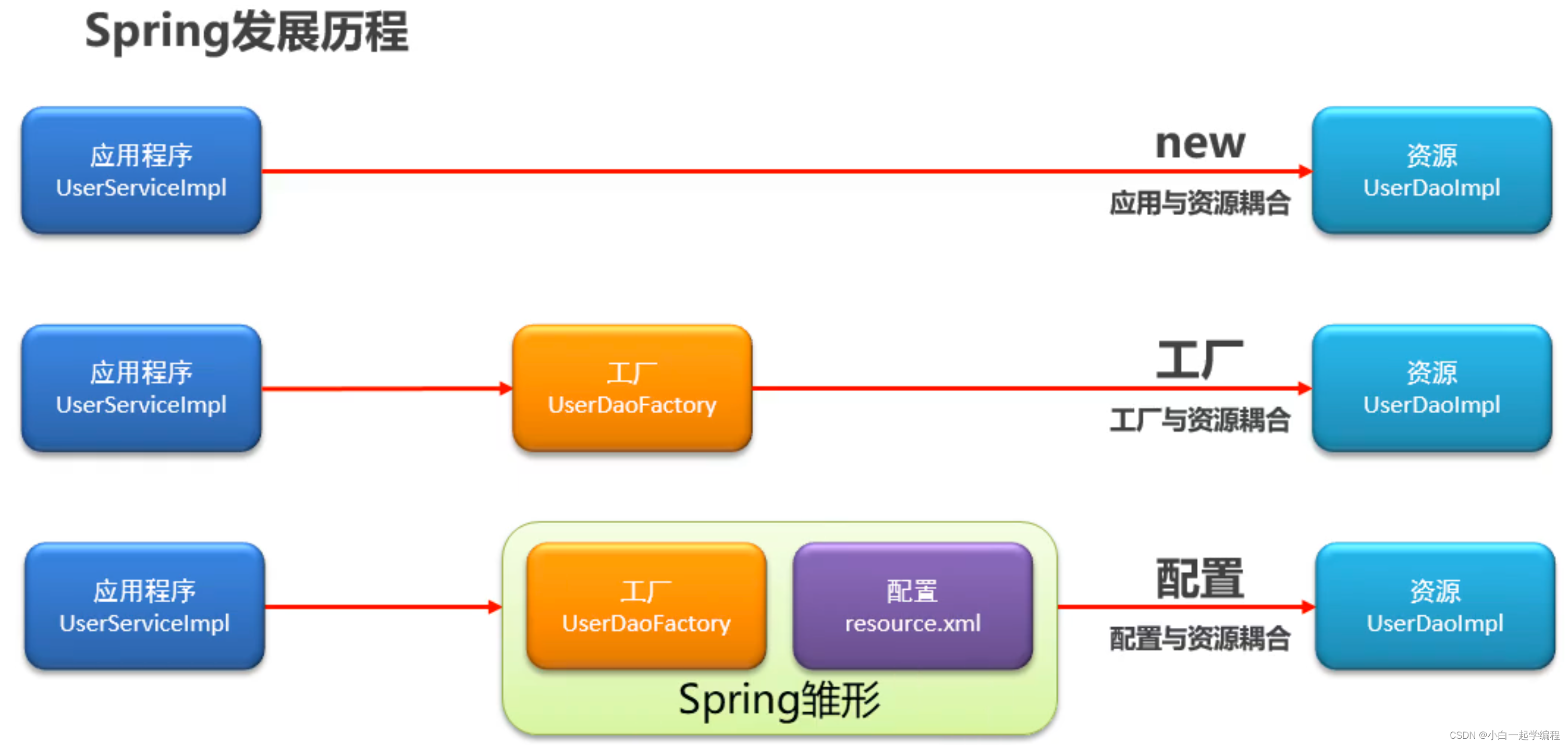 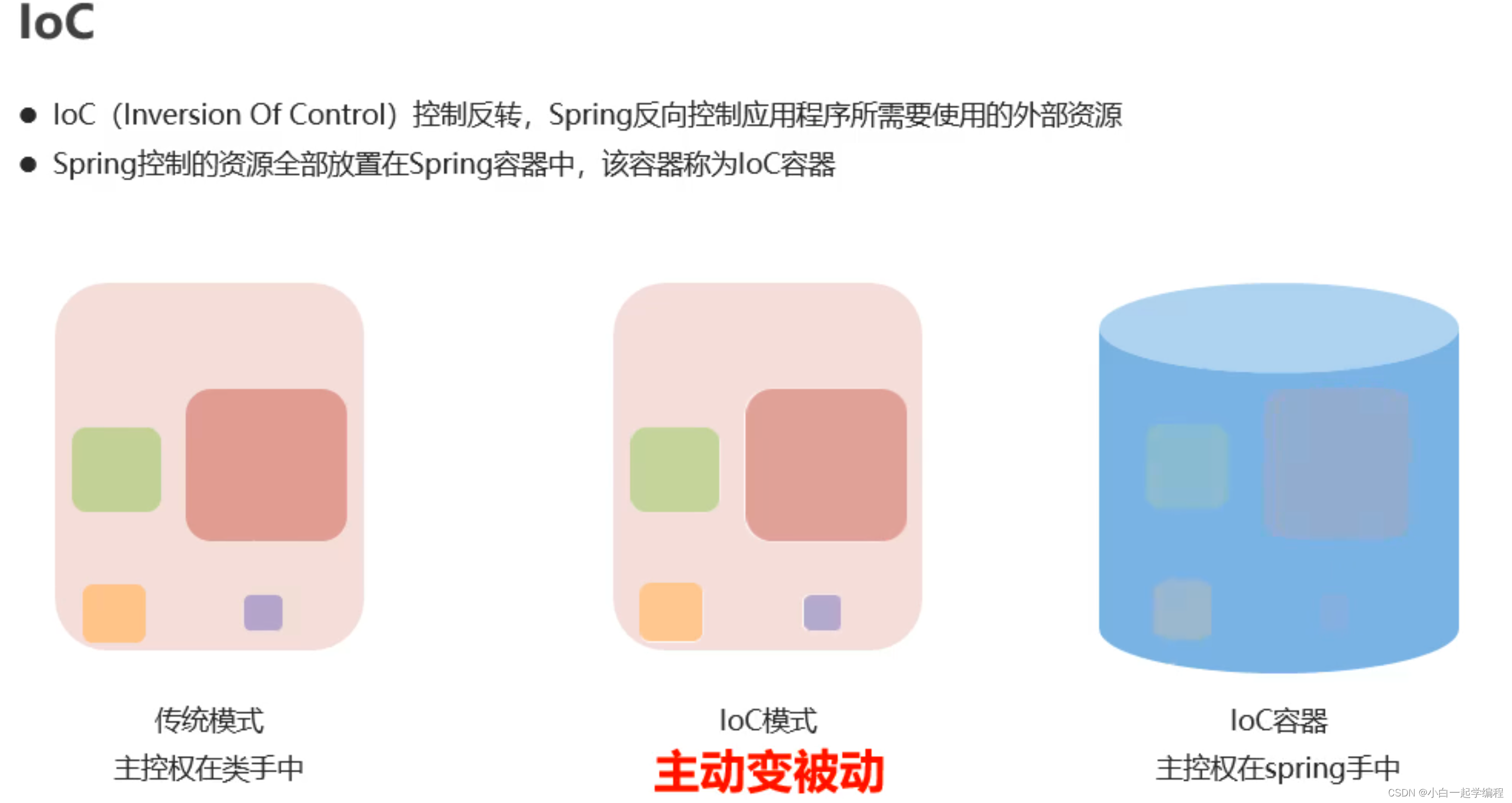
入门案例
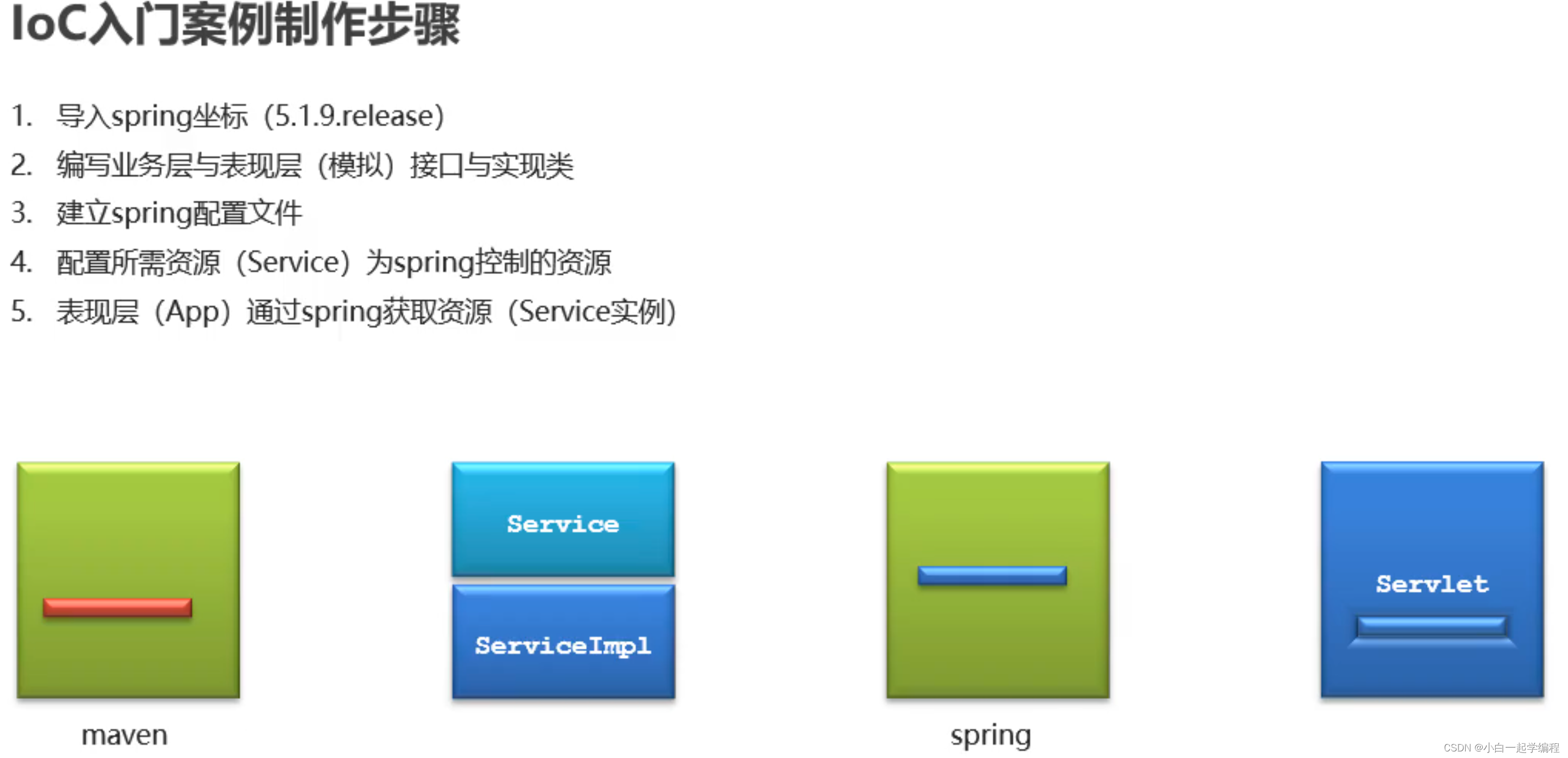
- pom.xml
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.1.9.RELEASE</version>
</dependency>
</dependencies>
- UserService & UserServiceImpl
package com.zs.service;
public interface UserService {
void save();
}
package com.zs.service.impl;
import com.zs.service.UserService;
public class UserServiceImpl implements UserService {
@Override
public void save() {
System.out.println("hello spring!!");
}
}
- 建立spring配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 1.创建spring控制的资源-->
<bean id="userService" class="com.zs.service.impl.UserServiceImpl"/>
</beans>
- 启动类
package com.zs;
import com.zs.service.UserService;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class UserApp {
public static void main(String[] args) {
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
UserService userService = (UserService) ctx.getBean("userService");
userService.save();
}
}
IoC配置(XML格式)
bean
基本属性(id,name,class)
作用: 定义spring中的资源,受此标签定义的资源将受到spring控制
<bean id="beanId" name="beanName1,beanNaem2" class="ClassName"/>
- id: bean的名称,通过id值获取bean
- class: bean的类型
- name: bean的名称,可以通过name值获取bean,用于多人配合时给bean起别名
采用的就是默认构造函数创建bean对象,此时如果类中没有默认构造函数,则对象无法创建
scope
作用:定义bean的作用范围
<bean scope="singleton"/>
默认就是单例的,单例时对象是在spring容器加载时创建,非单例时在对象创建时创建
- singleton: 设定创建出的对象保存在spring容器中,是一个单例的对象
- prototype: 设定创建出的对象保存在spring容器中,是一个非单例的对象
- request、session、applicaiton、websocket:设定创建出的对象放置在web容器对应的位置
bean生命周期(init-method,destroy-method)
作用:定义bean对象在初始化或销毁时完成的工作
<bean init-method="init" destroy-method="destroy"/>
bean对应的类中对应的具体方法名 init() ,destroy() 单例模式:只创建一次,init()做一次,ClassPathXmlApplicationContext 的close() 方法关闭时调用destroy() 非单例:创建一次,调一次,销毁方法不受spring管
工厂bean
factory-bean
作用:定义bean对象创建方式,使用静态工厂的形式创建bean,兼容早期遗留系统的升级工作
<bean id="userService4" class="com.zs.service.UserServiceFactory" factory-method="getService" />
public class UserServiceFactory {
public static UserService getService(){
return new UserServiceImpl();
}
}
factory-bean,factory-method
作用:定义bean对象创建方式,使用实例工厂的形式创建bean,兼容早期遗留系统的升级工作
<bean id="factoryBean" class="com.zs.service.UserServiceFactory2"/>
<bean id="userService5" factory-bean="factoryBean" factory-method="getService" />
public class UserServiceFactory {
public UserService getService(){
return new UserServiceImpl();
}
}
DI
DI依赖注入,应用程序运行依赖的资源由Spring为其提供,资源进入应用程序的方式称为注入 DI其实就是IoC容器,只是站在应用程序的角度的称呼
set注入(主流)
public class UserServiceImpl implements UserService {
private UserDao userDao;
private int num;
private Integer age;
private String name;
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void setNum(int num) {
this.num = num;
}
public void setAge(Integer age) {
this.age = age;
}
public void setName(String name) {
this.name = name;
}
@Override
public void save() {
System.out.println("hello spring!!");
userDao.save();
System.out.println(num + " " + age + " " + name);
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="userService" class="com.zs.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"/>
<property name="num" value="10"/>
<property name="age" value="10"/>
<property name="name" value="zs"/>
</bean>
<bean id="userDao" class="com.zs.dao.impl.UserDaoImpl"/>
</beans>
构造注入(了解)
public class UserServiceImpl implements UserService {
private UserDao userDao;
private int num;
private Integer age;
private String name;
public UserServiceImpl() {
}
public UserServiceImpl(UserDao userDao, int num, Integer age, String name) {
this.userDao = userDao;
this.num = num;
this.age = age;
this.name = name;
}
<bean id="userService" class="com.zs.service.impl.UserServiceImpl">
<constructor-arg name="userDao" ref="userDao"/>
<constructor-arg name="num" value="10"/>
<constructor-arg name="age" value="10"/>
<constructor-arg name="name" value="zs"/>
</bean>
<bean id="userDao" class="com.zs.dao.impl.UserDaoImpl"/>
集合注入(array,list,set,map,props)
public class BookDaoImpl implements BookDao {
private ArrayList al;
private Properties properties;
private int[] arr;
private HashSet hs;
private HashMap hm;
public void setAl(ArrayList al) {
this.al = al;
}
public void setProperties(Properties properties) {
this.properties = properties;
}
public void setArr(int[] arr) {
this.arr = arr;
}
public void setHs(HashSet hs) {
this.hs = hs;
}
public void setHm(HashMap hm) {
this.hm = hm;
}
@Override
public void save() {
System.out.println("book dao running..");
System.out.println("ArrayList" + al);
System.out.println("Properties: " + properties);
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
System.out.println("HashSet: " + hs);
System.out.println("HashMap: " + hm);
}
}
<bean id="userService" class="com.zs.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"/>
<property name="bookDao" ref="bookDao"/>
</bean>
<bean id="userDao" class="com.zs.dao.impl.UserDaoImpl"/>
<bean id="bookDao" class="com.zs.dao.impl.BookDaoImpl">
<property name="al">
<list>
<value type="java.lang.String">12</value>
<value type="java.lang.String">zs</value>
</list>
</property>
<property name="properties">
<props>
<prop key="name">zs</prop>
<prop key="value">666</prop>
</props>
</property>
<property name="arr">
<array>
<value>12</value>
<value>13</value>
</array>
</property>
<property name="hs">
<set>
<value type="java.lang.String">age</value>
<value type="java.lang.String">name</value>
</set>
</property>
<property name="hm">
<map>
<entry key="age" value-type="java.lang.String" value="18"/>
<entry key="name" value="zs"/>
</map>
</property>
</bean>
properties文件
public class UserDaoImpl implements UserDao {
private String username;
private String password;
public void setUsername(String username) {
this.username = username;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public void save() {
System.out.println(username + "\t" + password);
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:*.properties"/>
<bean id="userService" class="com.zs.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"/>
<property name="bookDao" ref="bookDao"/>
</bean>
<bean id="userDao" class="com.zs.dao.impl.UserDaoImpl">
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</bean>
username=zs666
password=123adfasdf
团队开发
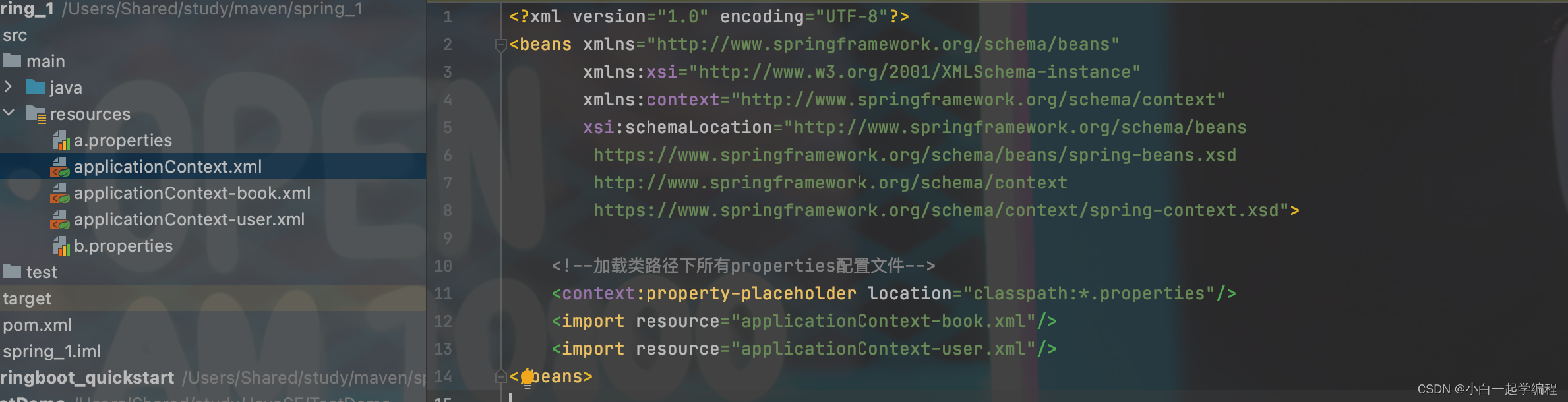 applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:*.properties"/>
<import resource="applicationContext-book.xml"/>
<import resource="applicationContext-user.xml"/>
</beans>
applicationContext-book.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<bean id="bookDao" class="com.zs.dao.impl.BookDaoImpl">
<property name="al">
<list>
<value type="java.lang.String">12</value>
<value type="java.lang.String">zs</value>
</list>
</property>
<property name="properties">
<props>
<prop key="name">zs</prop>
<prop key="value">666</prop>
</props>
</property>
<property name="arr">
<array>
<value>12</value>
<value>13</value>
</array>
</property>
<property name="hs">
<set>
<value type="java.lang.String">age</value>
<value type="java.lang.String">name</value>
</set>
</property>
<property name="hm">
<map>
<entry key="age" value-type="java.lang.String" value="18"/>
<entry key="name" value="zs"/>
</map>
</property>
</bean>
</beans>
applicationContext-user.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:*.properties"/>
<bean id="userService" class="com.zs.service.impl.UserServiceImpl">
<property name="userDao" ref="userDao"/>
<property name="bookDao" ref="bookDao"/>
</bean>
<bean id="userDao" class="com.zs.dao.impl.UserDaoImpl">
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</bean>
</beans>
ApplicationContext
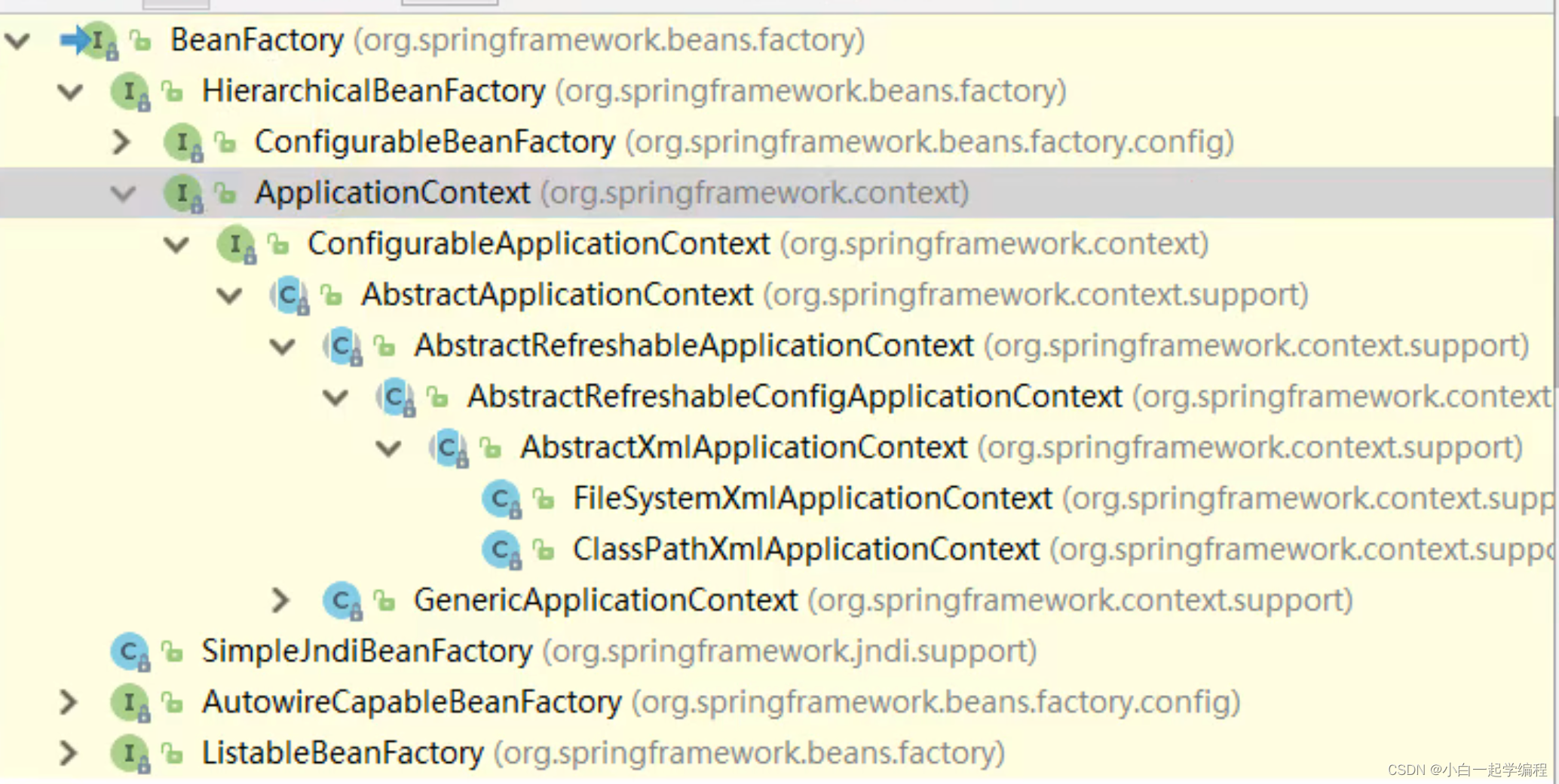
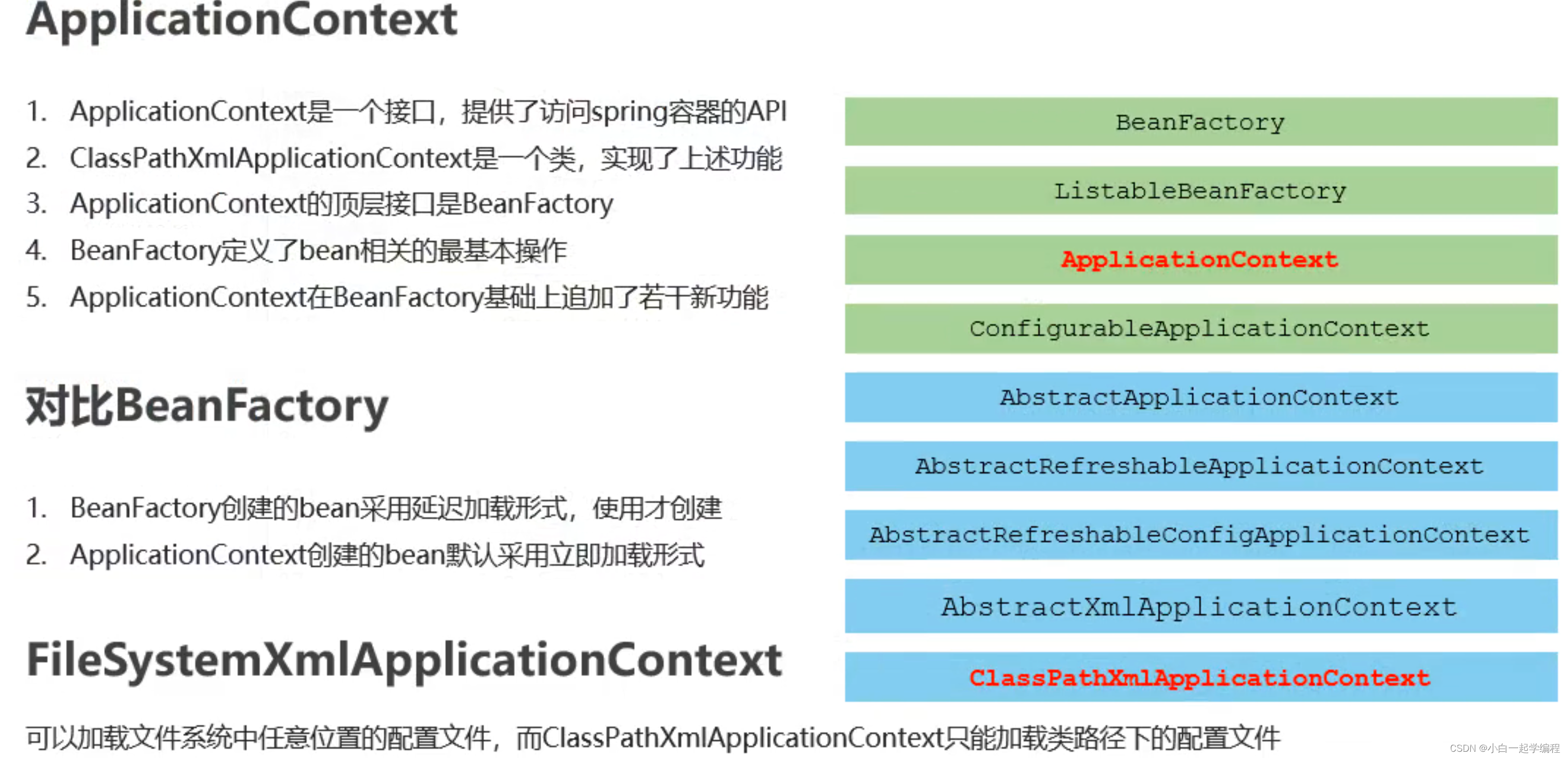 
第三方资源配置
Druid数据源
测试
DruidDataSource druidDataSource = new DruidDataSource();
System.out.println(druidDataSource);
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost3306/community"/>
<property name="username" value="iplat62"/>
<property name="password" value="iplat62"/>
</bean>
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>spring_1</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.1.9.RELEASE</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.21</version>
</dependency>
</dependencies>
<repositories>
<repository>
<id>aliyun-maven</id>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>aliyun-maven</id>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</pluginRepository>
</pluginRepositories>
</project>
综合案例
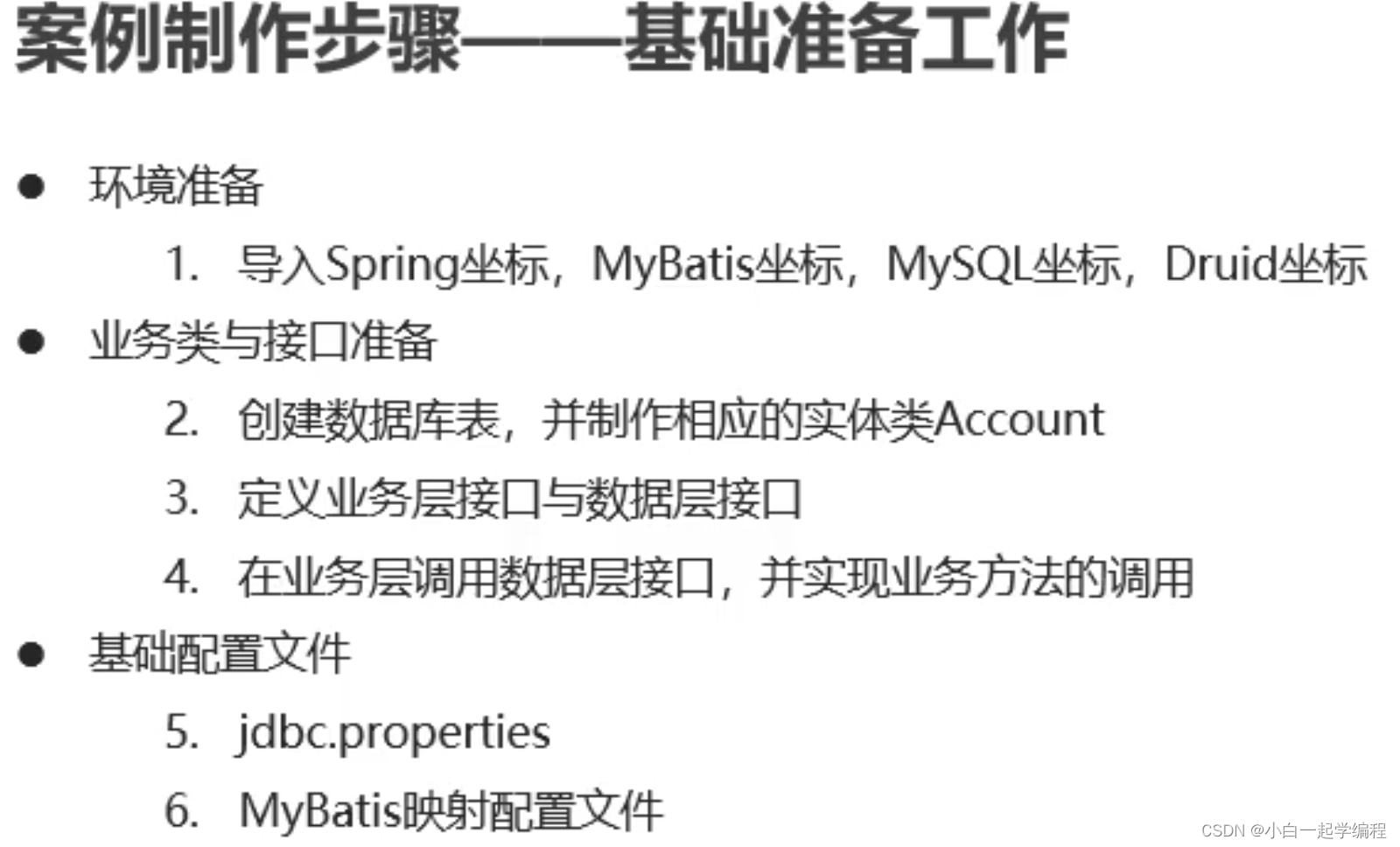 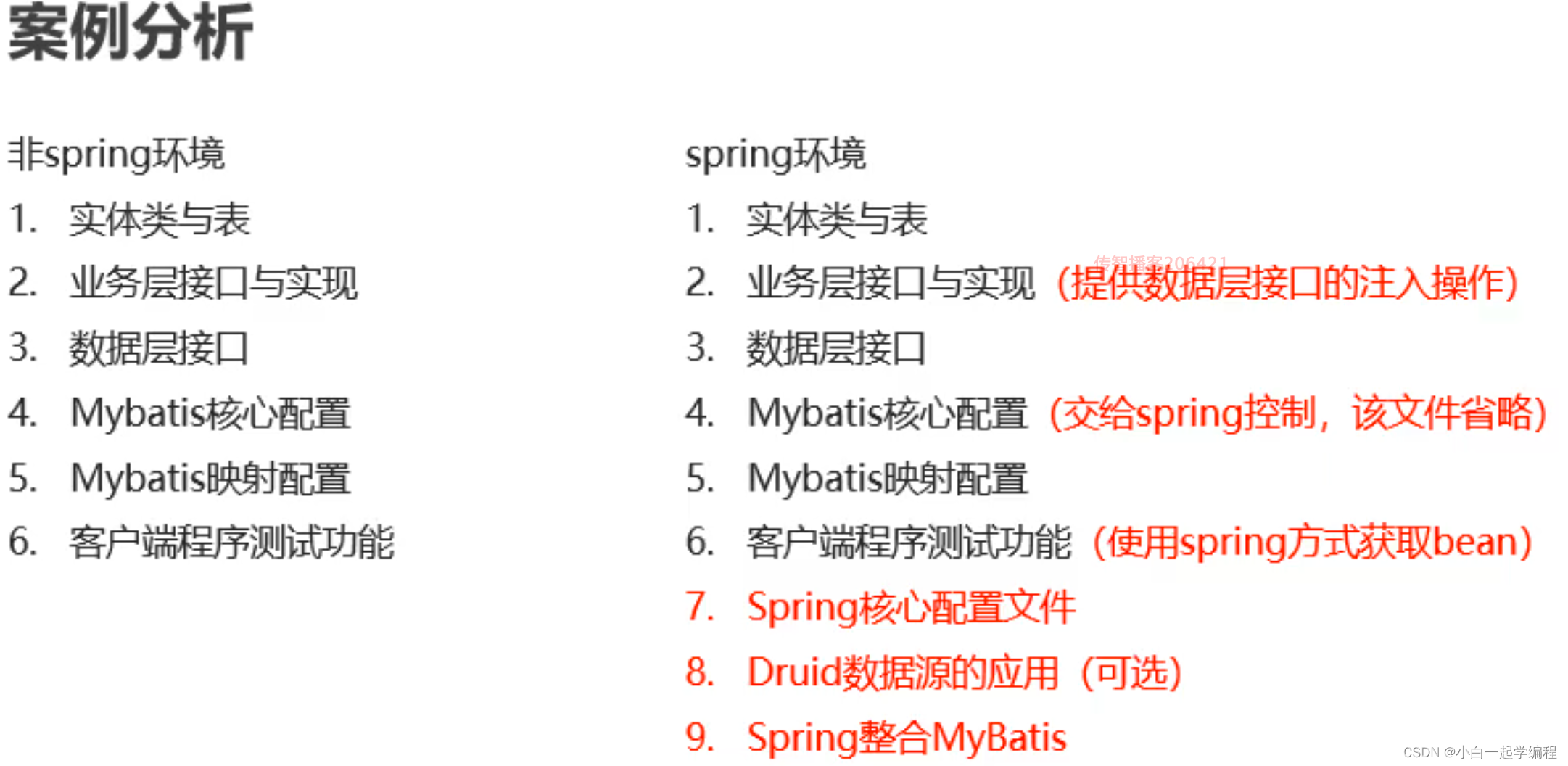
基础准备
坐标
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>spring_1</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.1.9.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.1.9.RELEASE</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.21</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.3</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
</dependencies>
<repositories>
<repository>
<id>aliyun-maven</id>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>aliyun-maven</id>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</pluginRepository>
</pluginRepositories>
</project>
业务类接口
数据表
create table account(
id int primary key auto_increment,
name varchar(40),
money float
)character set utf8 collate utf8_general_ci;
insert into account(name,money) values('aaa',1000);
insert into account(name,money) values('bbb',1000);
insert into account(name,money) values('ccc',1000);
业务类,接口
AccountDao
package com.zs.dao;
import com.zs.domain.Account;
import java.util.List;
public interface AccountDao {
void save(Account account);
void delete(Integer id);
void update(Account account);
List<Account> findAll();
Account findById(Integer id);
}
AccountService
package com.zs.service;
import com.zs.domain.Account;
import java.util.List;
public interface AccountService {
void save(Account account);
void delete(Integer id);
void update(Account account);
List<Account> findAll();
Account findById(Integer id);
}
AccountServiceImpl
package com.zs.service.impl;
import com.zs.dao.AccountDao;
import com.zs.domain.Account;
import com.zs.service.AccountService;
import java.util.List;
public class AccountServiceImpl implements AccountService {
private AccountDao accountDao;
public void setAccountDao(AccountDao accountDao) {
this.accountDao = accountDao;
}
@Override
public void save(Account account) {
accountDao.save(account);
}
@Override
public void delete(Integer id) {
accountDao.delete(id);
}
@Override
public void update(Account account) {
accountDao.update(account);
}
@Override
public List<Account> findAll() {
return accountDao.findAll();
}
@Override
public Account findById(Integer id) {
return accountDao.findById(id);
}
}
基础配置文件
jdbc.properties
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/testdb
jdbc.username=iplat62
jdbc.password=iplat62
SqlMapConfig.xml.bak :该文件被spring替换成bean
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="jdbc.properties"></properties>
<typeAliases>
<package name="com.zs.domain"/>
</typeAliases>
<environments default="mysql">
<environment id="mysql">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<package name="com.zs.dao"/>
</mappers>
</configuration>
AccountDao.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.zs.dao.AccountDao">
<select id = "findById" resultType="account" parameterType="int">
select * from account where id = #{id}
</select>
<select id="findAll" resultType="account">
select * from account
</select>
<insert id="save" parameterType="account">
insert into account(name,money) values(#{name},#{money})
</insert>
<delete id="delete" parameterType="int">
delete from account where id = #{id}
</delete>
<update id="update" parameterType="account">
update account set name = #{name},money=#{money} where id=#{id}
</update>
</mapper>
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:*.properties"/>
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<bean id="accountService" class="com.zs.service.impl.AccountServiceImpl">
<property name="accountDao" ref="accountDao"/>
</bean>
<bean class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.zs.domain"/>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.zs.dao"/>
</bean>
</beans>
测试类
package com.zs;
import com.alibaba.druid.pool.DruidDataSource;
import com.zs.domain.Account;
import com.zs.service.AccountService;
import com.zs.service.UserService;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
public class UserApp {
public static void main(String[] args) {
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
AccountService accountService = (AccountService) ctx.getBean("accountService");
Account byId = accountService.findById(1);
List<Account> all = accountService.findAll();
System.out.println(byId);
all.forEach(System.out::println);
}
}
常见问题
Exception in thread "main" org.apache.ibatis.binding.BindingException: Invalid bound statement (not found): com.zs.dao.AccountDao.findById 文件位置应该放到对应包下,对应包名需要注意是否只建立了一个包(下图是正确位置的截图) 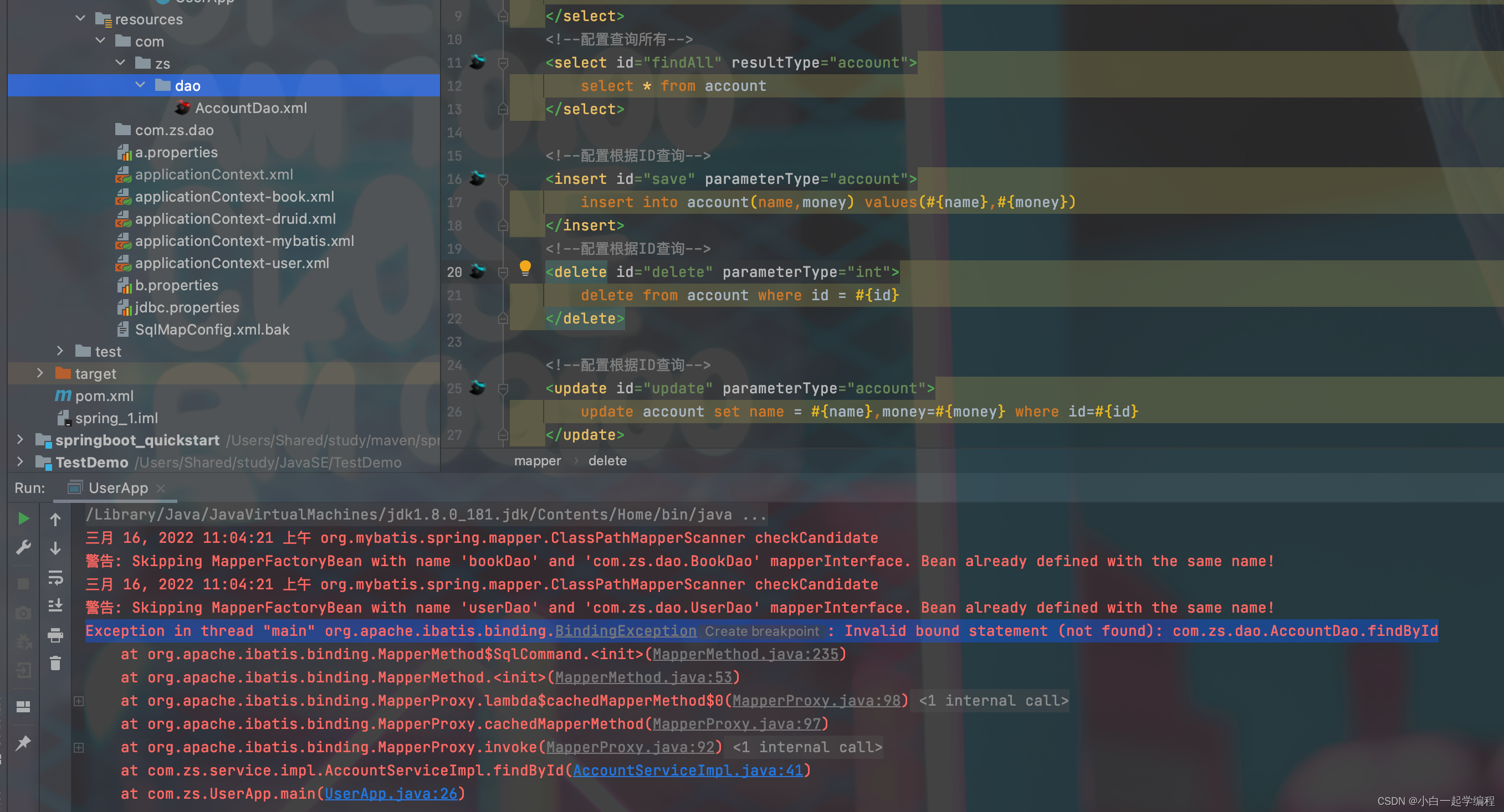
|