SpringBoot快速搭建简易WebSocket系统(springboot整合thymeleaf模板引擎)二
maven依赖
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.websocket</groupId>
<artifactId>springboot_websocket</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.4.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
</dependencies>
<build>
<finalName>websocket</finalName>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
<encoding>utf-8</encoding>
</configuration>
</plugin>
</plugins>
</build>
</project>
用户实体类
package com.websocket.po;
public class User {
private String id;
private String username;
private String password;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
构建测试模拟数据
package com.websocket.po;
import java.util.ArrayList;
import java.util.List;
public class UserList {
public static List<User> getUserList() {
List<User> list=new ArrayList<>();
User user=new User();
user.setId("1");
user.setUsername("admin");
user.setPassword("123456");
list.add(user);
User user2=new User();
user2.setId("2");
user2.setUsername("zhangsan");
user2.setPassword("123456");
list.add(user2);
User user3=new User();
user3.setId("3");
user3.setUsername("lisi");
user3.setPassword("123456");
list.add(user3);
return list;
}
}
核心服务类
package com.websocket.service;
import java.io.IOException;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.ConcurrentHashMap;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.PathParam;
import javax.websocket.server.ServerEndpoint;
import org.springframework.stereotype.Component;
@Component
@ServerEndpoint(value = "/websocket/{userid}")
public class WebSocketService {
private Session session;
private String userid;
private static Map<String, WebSocketService> map = new ConcurrentHashMap<String, WebSocketService>();
@OnOpen
public void opOpen(@PathParam("userid") String userid, Session session) {
System.out.println("建立连接===============>");
this.session = session;
this.userid = userid;
userid=UUID.randomUUID().toString();
map.put(userid, this);
}
@OnClose
public void onClose() {
System.out.println("断开连接===============>");
map.remove(userid);
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("后台接收消息===========>"+message);
try {
if (this.userid == "0") {
sendMessageTo(message, this.userid);
} else {
sendMessageAll(message);
}
} catch (IOException e) {
e.printStackTrace();
}
}
public void sendMessageTo(String message, String userId) throws IOException {
for (WebSocketService item : map.values()) {
if (item.userid.equals(userId)) {
System.out.println("进入单个消息");
item.session.getAsyncRemote().sendText(message);
break;
}
}
}
public void sendMessageAll(String message) throws IOException {
for (WebSocketService item : map.values()) {
System.out.println("进入群发消息"+map.values().toString());
item.session.getAsyncRemote().sendText(message);
}
}
}
核心配置类
package com.websocket.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.server.standard.ServerEndpointExporter;
@Configuration
public class WebSocketConfig {
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
登陆Controller
package com.websocket.controller;
import java.util.List;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.websocket.po.User;
import com.websocket.po.UserList;
@Controller
public class LoginController {
@GetMapping("")
public String login (){
return "login";
}
@RequestMapping("/loginCheck")
public String loginCheck(String username, String password, HttpSession session, Model model){
System.out.println("登陆用户名:"+username);
List<User> user = UserList.getUserList();
for (User u : user) {
if(u.getUsername().equals(username) && u.getPassword().equals(password)){
session.setAttribute("user", u);
model.addAttribute("user", u);
System.out.println("=======================>【"+u.getUsername()+"】登陆成功!");
return "index.html";
}else{
System.out.println("=======================>用户名或密码错误!");
}
}
return "login";
}
}
package com.websocket.controller;
import java.io.IOException;
import java.util.UUID;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.websocket.service.WebSocketService;
@Controller
public class WebSocketController {
@Autowired
WebSocketService webSocketService;
@RequestMapping("/createOrder")
public @ResponseBody String createOrder() throws IOException {
webSocketService.sendMessageAll("你有新的订单,请及时处理========>" + UUID.randomUUID());
return "新增订单成功!";
}
@RequestMapping("/createOrder2")
public @ResponseBody String createOrder2() throws IOException {
webSocketService.sendMessageTo("你有新的订单,请及时处理========>" + UUID.randomUUID(),"1");
return "新增订单成功!";
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户登陆</title>
</head>
<body>
<h3>欢迎来的简易聊天系统</h3>
<form action="loginCheck">
用户名:<input name="username" type="text"/><br/>
密码:<input name="password" type="text"/><br/>
<input type="submit" value="登陆">
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>测试webSocket</title>
<input th:value="${user.id}" id="userId" type="text"/>
</head>
<body>
<script type="text/javascript">
var websocket = null;
var userId=document.getElementById("userId").value;
if ('WebSocket' in window) {
websocket = new WebSocket("ws://localhost:80/websocket/"+userId);
} else {
alert("该浏览器不支持WebSocket!")
}
websocket.onopen = function(event) {
console.log("建立连接!");
}
websocket.onclose = function(event) {
console.log("连接关闭!");
}
websocket.onmessage = function(event) {
console.log("收到消息!" + event.data);
document.getElementById("div").innerHTML += event.data + '<br/>';
}
websocket.onerror = function() {
alert("websocket发生错误!");
}
websocket.onbeforeunload = function() {
websocket.close();
}
function sendMessage() {
var sendmsg = document.getElementById("sendMsg").value;
websocket.send(sendmsg);
}
</script>
<h3>多人群发聊天</h3>
<div id="div"></div>
<input id="sendMsg" />
<button onclick="sendMessage();">消息发送</button>
</body>
</html>
测试运行结果
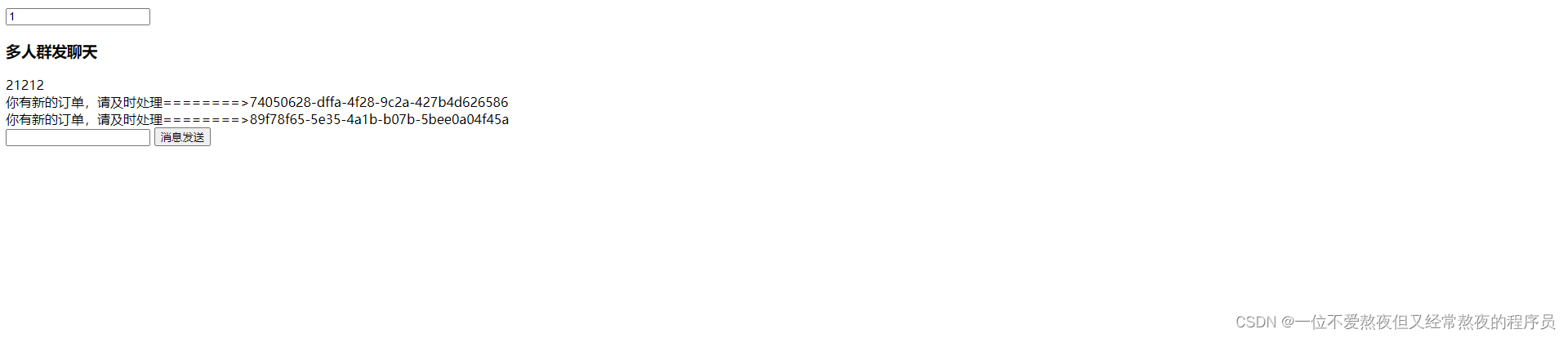 
|