1.使用描述
1.1 将Stream的数据收集到集合中去
* collect 将数据收集到 集合 中去
* 1.将数据收集到【集合】中去
* 1.1 List 集合中去
* .collect(Collectors.toList());
* 1.2 Set 集合中去
* .collect(Collectors.toSet()); // set会自动去重
*
* 【补充:直接返回集合的具体实现类的写法】
* 【
* Collectors.toCollection(Supplier<C> collectionFactory) :
* 参数为 函数式接口 :Supplier
* 因此可以使用 Lambda 表达式的方式进行编码;也可以对Lambda表达式进行方法引用的重构
* 】
* 1.3 ArrayList 集合中去
* .collect(Collectors.toCollection(ArrayList::new));
* 1.4 HashSet 集合中去
* .collect(Collectors.toCollection(HashSet::new));
1.2 将Stream中的数据收集到数组中去
* collect 将数据收集到 数组 中去
* 2.将数据收集到【数组】中去
* 2.1 .toArray() : 返回值为 Object[]
* 2.2 .toArray(IntFunction<A[]> generator):
* 参数为 一个 Function 的函数式接口,作用是传入一个 数字类型的参数,然后返回一个数组,类型可以自定义!
* 案例 : .toArray(value -> new Integer[value+2]); // value 就是上级流中的元素个数,返回一个Integer类型的数组
2.案例代码
2.1 代码
package com.northcastle.I_stream;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class StreamCollect01 {
public static void main(String[] args) {
List<Integer> intList01 = Stream.of(1, 2, 400, 500, 12, 30,500)
.filter(num -> num > 50)
.collect(Collectors.toList());
System.out.println("intList01 = "+intList01);
Set<Integer> intSet01 = Stream.of(1, 2, 400, 500, 12, 30, 500)
.filter(num -> num > 50)
.collect(Collectors.toSet());
System.out.println("intSet01 = "+intSet01);
ArrayList<Integer> intList02 = Stream.of(1, 2, 400, 500, 12, 30, 500)
.filter(num -> num > 50)
.collect(Collectors.toCollection(ArrayList::new));
System.out.println("intList02 = "+intList02);
HashSet<Integer> intSet02 = Stream.of(1, 2, 400, 500, 12, 30, 500)
.filter(num -> num > 50)
.collect(Collectors.toCollection(HashSet::new));
System.out.println("intSet02 = "+intSet02);
Object[] intObjects01 = Stream.of(1, 2, 400, 500, 12, 30, 500)
.filter(num -> num > 50)
.toArray();
System.out.println("intObjects01 = "+ Arrays.toString(intObjects01));
Integer[] intObjects02 = Stream.of(1, 2, 400, 500, 12, 30, 500)
.filter(num -> num > 50)
.toArray(Integer[]::new);
System.out.println("intObject02 = "+Arrays.toString(intObjects02));
}
}
2.2 运行结果
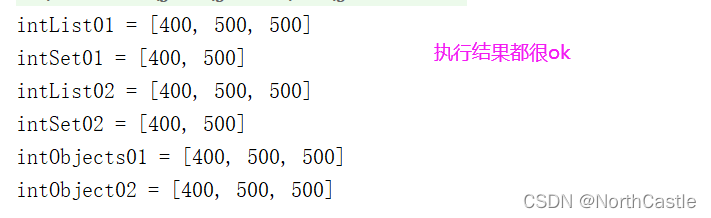
3.完成
Congratulations! You are one step closer to success!
|