导入依赖
<!--jwt-->
<dependency>
<groupId>com.auth0</groupId>
<artifactId>java-jwt</artifactId>
<version>3.10.3</version>
</dependency>
编写jwt工具类 TokenUtils.java
package com.chen.utils;
import cn.hutool.core.date.DateUtil;
import cn.hutool.core.util.StrUtil;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import com.chen.pojo.Users;
import com.chen.service.Users1Service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.annotation.PostConstruct;
import javax.servlet.http.HttpServletRequest;
import java.util.Date;
@Component
public class TokenUtils {
private static Users1Service staticUser1Service;
@Autowired
private Users1Service users1Service;
@PostConstruct
public void setUsers1Service(){
staticUser1Service=users1Service;
}
public static String genToken(String userId,String sign){
return JWT.create().withAudience(userId)
.withExpiresAt(DateUtil.offsetHour(new Date(),2))
.sign(Algorithm.HMAC256(sign));
}
public static Users getCurrentUser() {
try {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
String token = request.getHeader("token");
if (StrUtil.isNotBlank(token)) {
String userId = JWT.decode(token).getAudience().get(0);
return staticUser1Service.getById(Integer.valueOf(userId));
}
} catch (Exception e) {
return null;
}
return null;
}
}
自定义异常 JwtInterceptor .java
package com.chen.config.interceptor;
import cn.hutool.core.util.StrUtil;
import com.auth0.jwt.JWT;
import com.auth0.jwt.JWTVerifier;
import com.auth0.jwt.algorithms.Algorithm;
import com.auth0.jwt.exceptions.JWTDecodeException;
import com.auth0.jwt.exceptions.JWTVerificationException;
import com.chen.common.Constants;
import com.chen.exception.ServiceException;
import com.chen.pojo.Users;
import com.chen.service.Impl.Users1ServiceImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.web.method.HandlerMethod;
import org.springframework.web.servlet.HandlerInterceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class JwtInterceptor implements HandlerInterceptor {
@Autowired
Users1ServiceImpl users1Service;
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
String token = request.getHeader("token");
if(!(handler instanceof HandlerMethod)){
return true;
}
if (StrUtil.isBlank(token)){
throw new ServiceException(Constants.CODE_401,"无token,请登录");
}
String userId;
try {
userId = JWT.decode(token).getAudience().get(0);
} catch (JWTDecodeException j) {
throw new ServiceException(Constants.CODE_401,"token验证失败");
}
Users user = users1Service.getById(userId);
if (user == null) {
throw new ServiceException(Constants.CODE_401,"用户不存在,请重新登录");
}
JWTVerifier jwtVerifier = JWT.require(Algorithm.HMAC256(user.getPassword())).build();
try {
jwtVerifier.verify(token);
} catch (JWTVerificationException e) {
throw new ServiceException(Constants.CODE_401,"用户不存在,请重新登录");
}
return true;
}
}
配置拦截器,约束拦截规则 InterceptorConfig .java
package com.chen.config;
import com.chen.config.interceptor.JwtInterceptor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class InterceptorConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(jwtInterceptor())
.addPathPatterns("/**")
.excludePathPatterns("/sys/login","/sys/register","/**/export","/**/import");
}
@Bean
public JwtInterceptor jwtInterceptor(){
return new JwtInterceptor();
}
}
最后调用方法获取当前用户
Users currentUser = TokenUtils.getCurrentUser();
System.out.println(currentUser.getNickname());
将token放在前端请求中
request.interceptors.request.use(config => {
config.headers['Content-Type'] = 'application/json;charset=utf-8';
let user=localStorage.getItem("user")?JSON.parse(localStorage.getItem("user")):{}
if (user) {
config.headers['token'] = user.token;
}
return config
}, error => {
return Promise.reject(error)
});
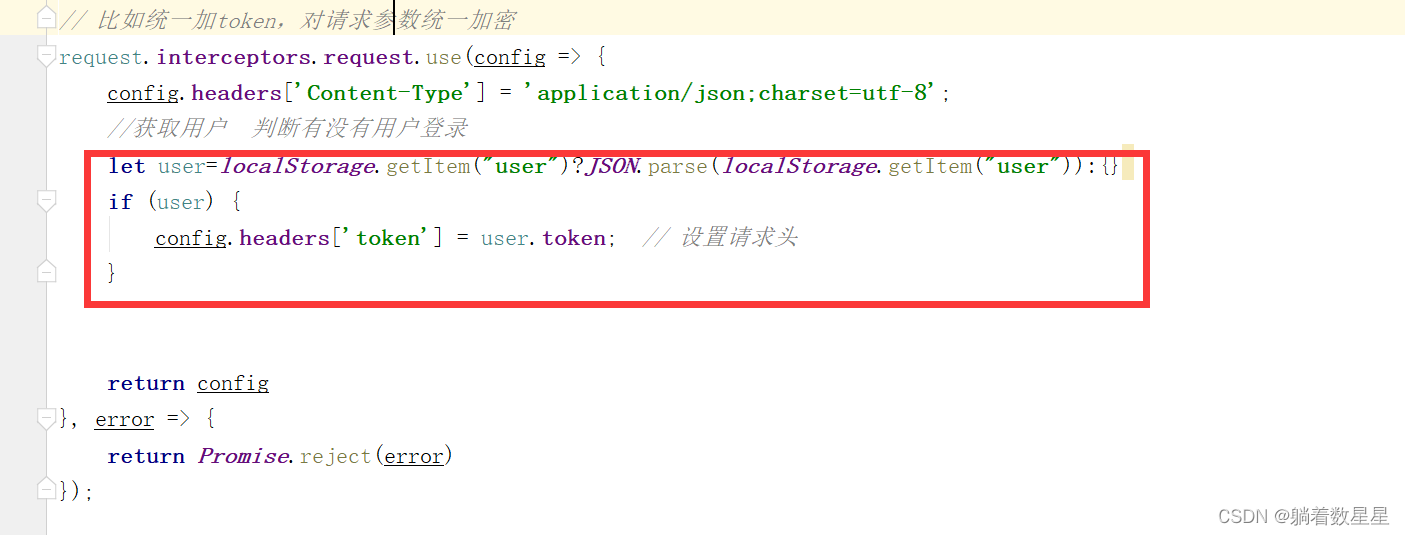
效果截图 
|