作为Java-web,平时总会在项目中涉及到文件上传,比如图片上传、Excel上传、PDF上传等等情况,今天以最普遍的图片上传为例,展示如下:
pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.4.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 第三方工具类 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
application.yml
server:
port: 6060
# 图片存储地址
img:
location: C:/Download
spring:
servlet:
multipart:
enabled: true
# 设置单文件最大值
max-file-size: 2MB
# 设置单次请求文件最大值
max-request-size: 10MB
# 设置文件预览路径
resources:
static-locations: file:${img.location}
业务逻辑
@RestController
@RequestMapping("/upload")
public class UploadController {
@Value("${img.location}")
private String imgLocation;
@Value("${spring.servlet.multipart.max-file-size}")
private String imgSize;
@PostMapping("/img")
public String uploadImg(@RequestParam("logo") MultipartFile file, HttpServletRequest request, HttpServletResponse response) throws IOException {
FileOutputStream fileOutputStream = null;
String newFileName = null;
if (file != null) {
//获取文件原始文件名
String filename = file.getOriginalFilename();
if (StringUtils.isNotBlank(filename)) {
String[] filenameArr = filename.split("\\.");
// 获取文件后缀名
String suffix = filenameArr[filenameArr.length - 1];
// 判断图片格式,判断时忽略大小写
if (!suffix.equalsIgnoreCase("png") && !suffix.equalsIgnoreCase("jpg") && !suffix.equalsIgnoreCase("jpeg")) {
throw new RuntimeException("图片格式不正确");
}
SimpleDateFormat df = new SimpleDateFormat("yyyyMMddHHmmss");//设置日期格式
String date = df.format(new Date());// new Date()为获取当前系统时间,也可使用当前时间戳
// 文件名重组 logo-uuid()随机字符-时间
newFileName = "logo-" + UUID.randomUUID() + date + "." + suffix;
// 图片最终保存位置 路径 + 文件名
String finalFilePath = imgLocation + File.separator + newFileName;
System.out.println(finalFilePath);
// 上传文件
File outFile = new File(finalFilePath);
// 判断文件夹是否存在
if (outFile.getParentFile() != null) {
// 创建文件夹
outFile.getParentFile().mkdirs();
}
// 文件输出保存到文件夹
fileOutputStream = new FileOutputStream(outFile);
InputStream inputStream = file.getInputStream();
IOUtils.copy(inputStream, fileOutputStream);
}
} else {
throw new RuntimeException("文件不能为空");
}
return "图片上传成功:查看地址为:ip:port" + newFileName;
}
private long parseSize(String size) {
size = size.toUpperCase();
return size.endsWith("KB")?Long.valueOf(size.substring(0, size.length() - 2)).longValue() * 1024L:(size.endsWith("MB")?Long.valueOf(size.substring(0, size.length() - 2)).longValue() * 1024L * 1024L:Long.valueOf(size).longValue());
}
}
全局异常类
/**
* @Description: 设置全局异常类,捕获异常
* 全局异常类,是在Controller执行之前被调用的
*/
@RestControllerAdvice
public class CommonExceptionHandler {
//单独捕获 文件上传过大的异常,可以配合 使用统一返回对象RestUtil(code,msg,data) 进行指定错误码、错误消息内容等
@ExceptionHandler(value = MaxUploadSizeExceededException.class)
public String Exception(MaxUploadSizeExceededException e) {
return "上传文件过大,不得超过2MB";
//return e.getMessage();
}
}
通过postman测试
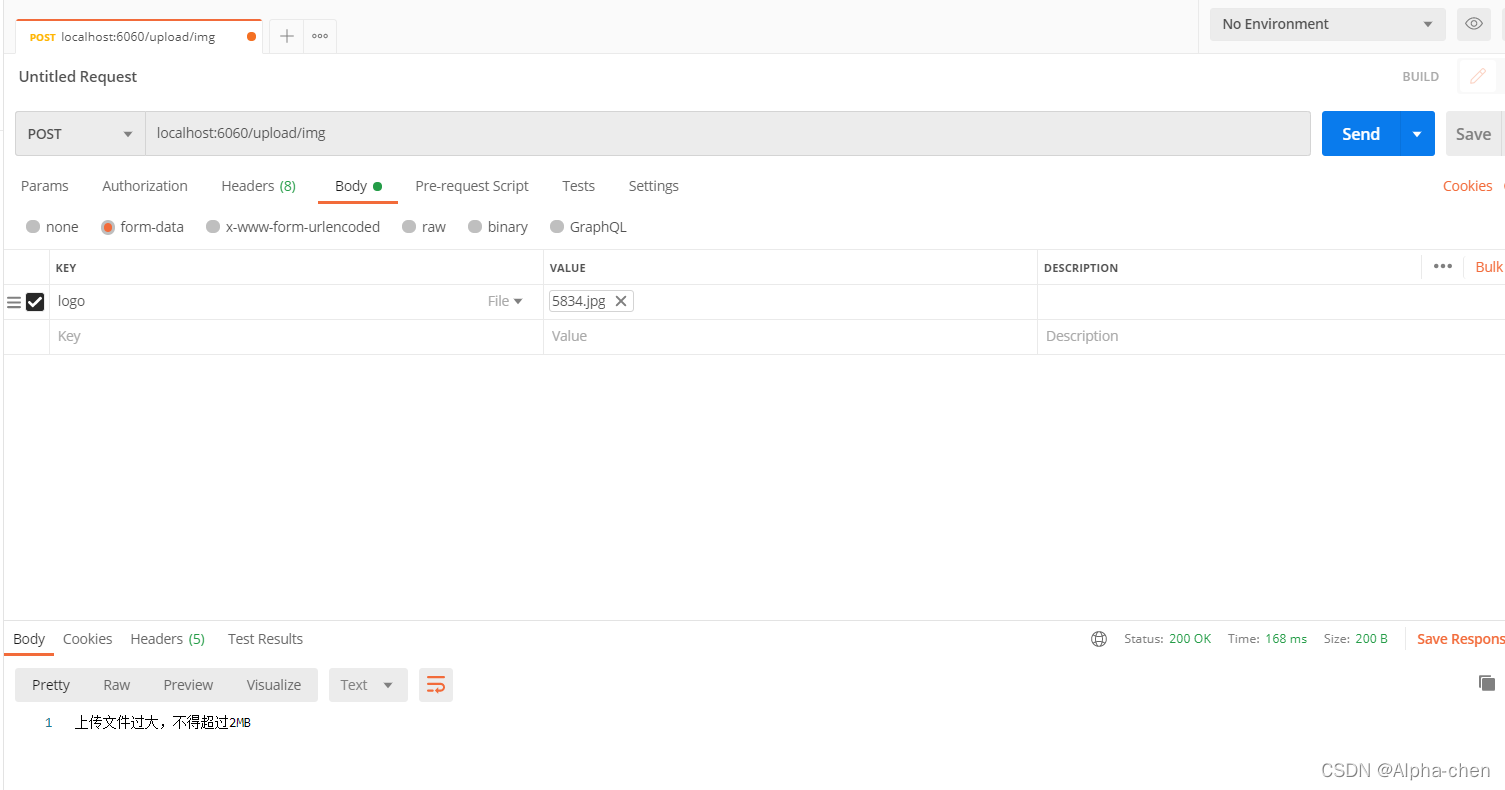
|