Webflux注解编程模型
SpringWebflux实现方式有两种:注解编程模型和函数式编程模型 使用注解编程模型方式,和之前SpringMVC使用相似,只需要把相关依赖配置到项目中,SpringBoot自动配置相关运行容器,默认情况下使用Netty服务器 说明: SpringMVC方式实现,同步阻塞的方式,基于SpringMVC+servlet+tomcat SpringWebflux方式实现,异步非阻塞方式,基于SpringWebflux+Reactor+Netty
- 创建springboot工程,引入webFlux相关依赖
 - 配置启动的端口号
 - 创建包和相关类(目录结构)
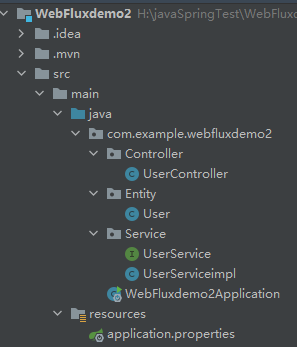 实体类User.java
package com.example.webfluxdemo2.Entity;
public class User {
private String name;
private String gender;
private int age;
public User(String name, String gender, int age) {
this.name = name;
this.gender = gender;
this.age = age;
}
public void setName(String name) {
this.name = name;
}
public void setGender(String gender) {
this.gender = gender;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public int getAge() {
return age;
}
}
接口UserService
package com.example.webfluxdemo2.Service;
import com.example.webfluxdemo2.Entity.User;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
public interface UserService {
Mono<User> getUserById(int id);
Flux<User> getAllUser();
Mono<Void> addUserInfo(Mono<User> userMono);
}
接口实现类UserServiceimpl
package com.example.webfluxdemo2.Service;
import com.example.webfluxdemo2.Entity.User;
import org.springframework.stereotype.Repository;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.util.HashMap;
import java.util.Map;
@Repository
public class UserServiceimpl implements UserService{
private final Map<Integer,User> user=new HashMap<>();
public UserServiceimpl(){
this.user.put(1,new User("sun","nan",18));
this.user.put(2,new User("tom","nv",23));
this.user.put(3,new User("jack","nan",25));
}
@Override
public Mono<User> getUserById(int id) {
return Mono.justOrEmpty(this.user.get(id));
}
@Override
public Flux<User> getAllUser() {
return Flux.fromIterable(this.user.values());
}
@Override
public Mono<Void> addUserInfo(Mono<User> userMono) {
return userMono.doOnNext(person->{
int id=user.size()+1;
this.user.put(id,person);
}).thenEmpty(Mono.empty());
}
}
UserController.java
package com.example.webfluxdemo2.Controller;
import com.example.webfluxdemo2.Entity.User;
import com.example.webfluxdemo2.Service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/user/{id}")
public Mono<User> getUserId(@PathVariable int id){
return userService.getUserById(id);
}
@GetMapping("/user")
public Flux<User> GetAllUser(){
return userService.getAllUser();
}
@GetMapping("/adduser")
public Mono<Void> addUserInfo(@RequestBody User user){
Mono<User> userMono=Mono.just(user);
return userService.addUserInfo(userMono);
}
}
运行效果 
|