- 创建Spring Boot 工程,并添加依赖
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-web-starter</artifactId>
<version>1.8.0</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.8</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
- pom.xml 的 build 下添加如下
<resources>
<resource>
<directory>src/main/resources</directory>
</resource>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
</resources>
- 创建实体类
@Data
public class User {
private Integer uid;
private String username;
private String password;
}
- UserMapper
@Mapper
@Repository
public interface UserMapper{
User getUserByUsername(String username);
}
- UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.springbootshiro.mapper.UserMapper">
<select id="getUserByUsername" resultType="com.example.springbootshiro.model.User">
select *
from user
where username = #{username}
</select>
</mapper>
- application.yml
spring:
datasource:
username: "root"
password: "123"
url: "jdbc:mysql://localhost:3306/mydb?serveTimezone=Asia/shanghai"
#Spring Boot 默认是不注入这些属性值的,需要自己绑定
#druid 数据源专有配置
initialSize: 5
minIdle: 5
maxActive: 20
maxWait: 60000
timeBetweenEvictionRunsMillis: 60000
minEvictableIdleTimeMillis: 300000
validationQuery: SELECT 1 FROM DUAL
testWhileIdle: true
testOnBorrow: false
testOnReturn: false
poolPreparedStatements: true
#mybatid配置
mybatis:
type-aliases-package: com/example/springbootshiro/model
mapper-locations: classpath:com/example/springbootshiro/mapper/*.xml
- UserRealm
public class UserRealm extends AuthenticatingRealm {
@Resource
UserMapper userMapper;
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
String username = (String) token.getPrincipal();
User user = userMapper.getUserByUsername(username);
if (user==null){
throw new UnknownAccountException();
}
/**
*SimpleAuthenticationInfo()参数:
*@param principal与指定领域关联的“主要”主体。(数据库中获取的对象)
*@param hashedCredentials验证给定主体的哈希凭据。(用户的密码)
*@param credentialsSalt对给定的hashedCredentials进行哈希时使用的盐(加密盐)
*@param realmName获取主体和凭据的领域。(从客户端接受的用户名)
*/
SimpleAuthenticationInfo info = new SimpleAuthenticationInfo(user,user.getPassword(),null,username);
return info;
}
}
- ShiroConfig
@Configuration
public class ShiroConfig {
@Bean
public UserRealm realm() {
return new UserRealm();
}
@Bean
public ShiroFilterChainDefinition filterChainDefinition() {
DefaultShiroFilterChainDefinition shiroFilterChainDefinition = new DefaultShiroFilterChainDefinition();
/**
* 注意顺序
* 不需要认证的放在前面
*/
shiroFilterChainDefinition.addPathDefinition("/login", "anon");//放行
shiroFilterChainDefinition.addPathDefinition("/register", "anon");
shiroFilterChainDefinition.addPathDefinition("/**", "authc");//拦截
return shiroFilterChainDefinition;
}
@Bean("securityManager")
@Primary
public DefaultWebSecurityManager securityManager(UserRealm userRealm) {
DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager();
securityManager.setRealm(userRealm);
return securityManager;
}
}
- 测试
@RestController
public class UserController {
@PostMapping("/login")
public String Login(String username, String password) {
UsernamePasswordToken token = new UsernamePasswordToken(username, password);
try {
SecurityUtils.getSubject().login(token);
} catch (UnknownAccountException e) {
return e.getMessage();
}
User principal = (User) SecurityUtils.getSubject().getPrincipal();
return "200 "+principal.toString();
}
}
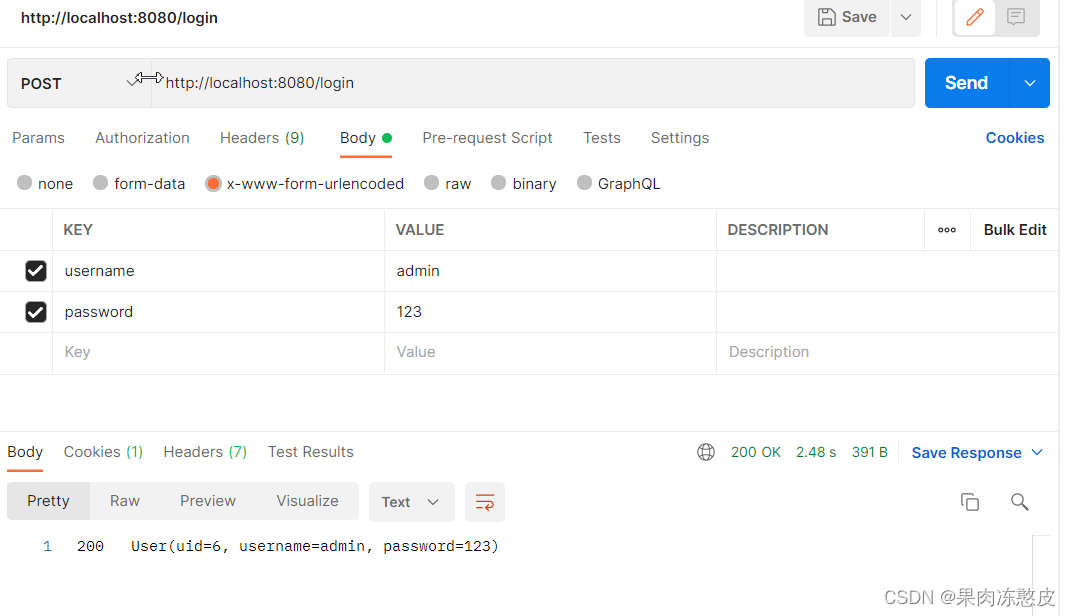 运行成功!
|