①:函数式接口(支持Lamda):(有且只有一个抽象方法的接口) 列举几个常用的
public static void main(String[] args) {
// 一个参数,一个返回值
Function<String, String> dd = i -> {
String ii = "My Name is";
return ii + i;
};
// 一个参数,无返回值
Consumer<String> ee = i -> System.out.println(i);
// 一个参数,返回布尔类型
Predicate<String> ff = i -> i.length() > 10;
// 没有参数,一个返回值
Supplier<String> gg = () -> "yes";
System.out.println(dd.apply("wenwenYU"));
ee.accept("CAI CAI WO SHI SHUI");
System.out.println(ff.test("1234567891234"));
System.out.println(gg.get());
}
个人理解:函数式接口更像是把代码逻辑块作为方法的参数。
②:Stream流
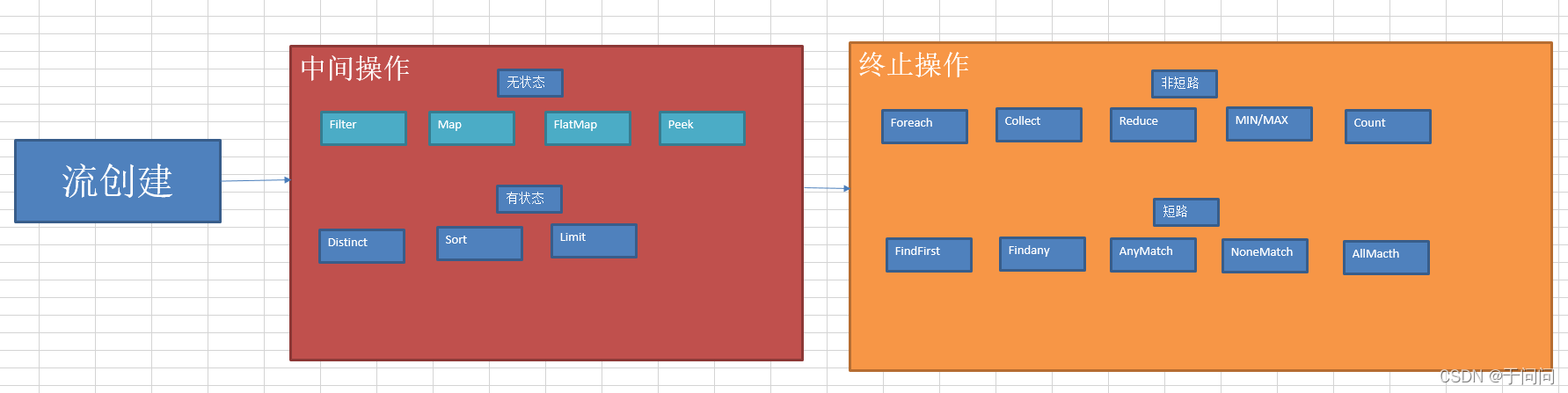
?一个Stream流操作:可以有N个中间操作,但只能有且只有一个终止操作。
假设一个场景:
一个List<Person> Person有两个属性String name,String code 现在要变成map<String,String> key为code,value为name
public static void main(String[] args) {
List<Person> personList =new ArrayList<>();
personList.add(new Person("于问问","988"));
personList.add(new Person("苗问问","988"));
personList.add(new Person("李问问","777"));
Map<String, String> personMap = personList.stream()
.collect(
Collectors.toMap(Person::getCode, Person::getName)
);
}
这样会不会有问题?答案是会有问题的。 Map的key值不能重复,现在于问问和苗问问的code号都是988
遇到这种情况转Map时就必须得有取舍了,苗问问和于问问只能留一个,那就留苗问问吧
Map<String, String> personMap = personList.stream()
.collect(Collectors.toMap(Person::getCode, Person::getName, (v1, v2) -> v2));
(v1,v2)->v2的意思也就是前者和后者传进来,只留后者
|