SpringBoot RedisTemplate opsForList
- 1 SpringBoot RedisTemplate
- 1 Long leftPush(K key, V value)
- 2 Long leftPush(K key, V pivot, V value)
- 2 Long leftPushAll(K, Collection)
- 3 Long leftPushAll(K, V...)
- 4 Long leftPushIfPresent(K, V)
- 5 V leftPop(K key)
- 6 List\ leftPop(K key, long count)
- 7 V leftPop(K, long, TimeUnit)
- 8 Long rightPush(K, V)
- 9 Long rightPush(K, V, V)
- 10 Long rightPushAll(K, Collection)
- 11 Long rightPushAll(K, V...)
- 12 Boolean rightPushIfPresent(K, V)
- 13 rightPop(K)
- 14 rightPop(K, long)
- 15 rightPop(K, long, TimeUnit)
- 16 V rightPopAndLeftPush(K sourceKey, K destinationKey)
- 17 rightPopAndLeftPush(K, K, long, TimeUnit)
- 18 V (K key, long index)
- 19 Long indexOf(K key, V value)
- 20 lastIndexOf(K key, V value)
spring-data-redis-3.0.0-M2.jar
@Autowired
private RedisTemplate redisTemplate;
@Override
public ListOperations<K, V> opsForList() {
return listOps;
}
类型 | Value |
---|
String | 字符串 | List | 列表 | Hash | 字典 | Set | 无序集合 | zset(Sorted Set) | 有序集合 |
1 SpringBoot RedisTemplate
1 SpringBoot RedisTemplate opsForValue 2 SpringBoot RedisTemplate opsForHash 3 SpringBoot RedisTemplate opsForList
操作 | 作用 |
---|
push 操作 | leftpush是在list的左侧添加,即列表的头部,right是在list的右侧侧添加,即在列表的尾部。 | pop 操作 | 和push一样。pop是获取一个元素(leftpop:先入后出,rightpop:先入先出),并且删除这个元素。 | range | 查看某个元素 |
1 Long leftPush(K key, V value)
K 是否存在 | 操作 | 返回 | 解释 |
---|
是 | 添加 | 添加成功后的list的长度 | 先添加的位于栈底 | 否 | 创建 | 添加成功后的list的长度 | 先添加的位于栈底 |
@Test
public void leftPush() {
Long value = redisTemplate.opsForList().leftPush("OpsForList", "M");
System.out.println(value);
}
@Test
public void leftPush() {
redisTemplate.opsForList().leftPush("OpsForList", "A");
redisTemplate.opsForList().leftPush("OpsForList", "B");
redisTemplate.opsForList().leftPush("OpsForList", "C");
}
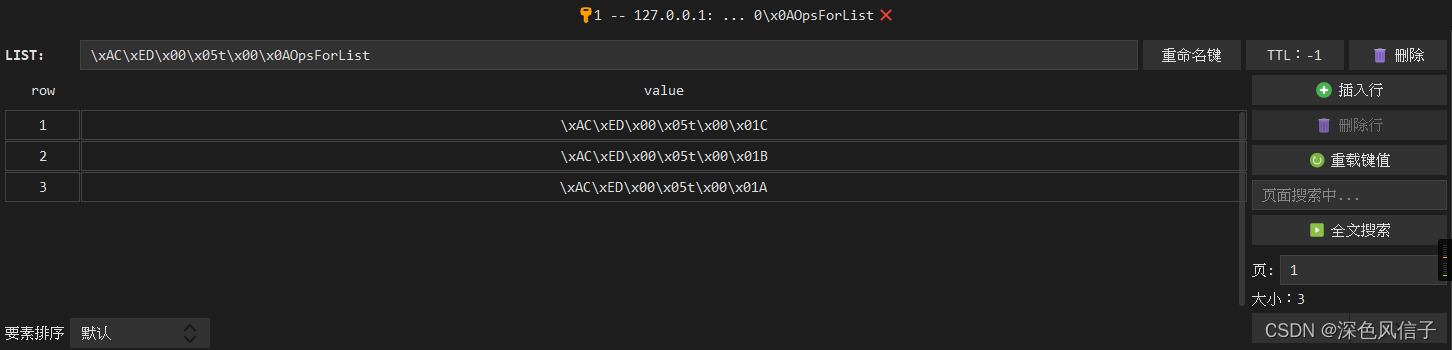
2 Long leftPush(K key, V pivot, V value)
pivot 是否存在 | 操作 | 返回 |
---|
是 | 添加到 pivot 前面 | 添加成功后的list的长度 | 否 | 不做操作 | -1 |
@Test
public void leftPush() {
Long value = redisTemplate.opsForList().leftPush("OpsForList", "A", "M-");
System.out.println(value);
}
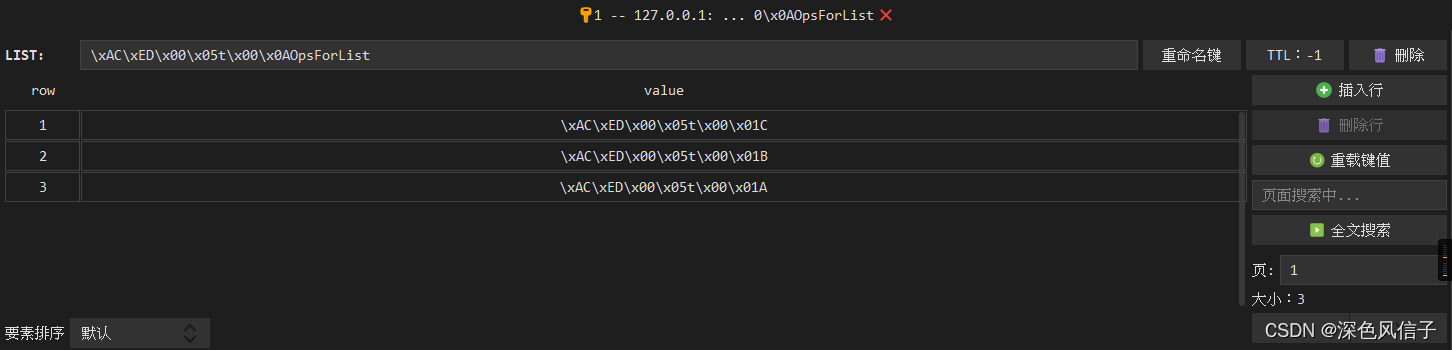 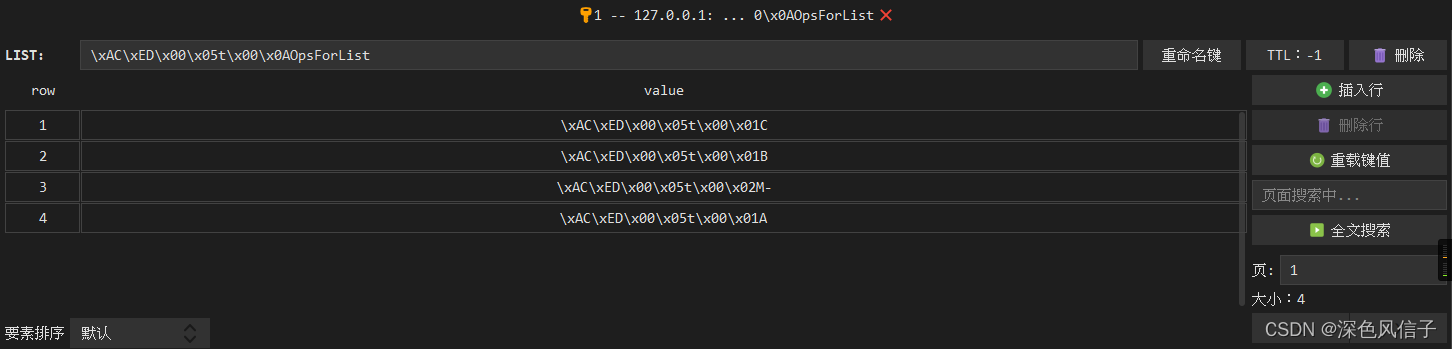
2 Long leftPushAll(K, Collection)
和1类似,一次left添加多个,返回添加成后的list的长度
3 Long leftPushAll(K, V…)
和1类似,一次left添加多个,返回添加成后的list的长度
4 Long leftPushIfPresent(K, V)
K 是否存在 | 操作 | 返回 |
---|
是 | 添加 | 返回添加成后的list的长度 | 否 | 不添加 | 0 |
@Test
public void leftPushIfPresent() {
Long value1 = redisTemplate.opsForList().leftPushIfPresent("OpsForList", "KV");
System.out.println(value1);
Long value2 = redisTemplate.opsForList().leftPushIfPresent("OpsForList-A", "B");
System.out.println(value2);
}
5
0
5 V leftPop(K key)
K 是否存在 | 操作 | 返回 |
---|
是 | 获取左边第一个元素(栈顶元素)并移除 | 左边第一个元素 | 否 | 不做操作 | null |
@Test
public void leftPop() {
String value = (String) redisTemplate.opsForList().leftPop("OpsForList");
System.out.println(value);
}
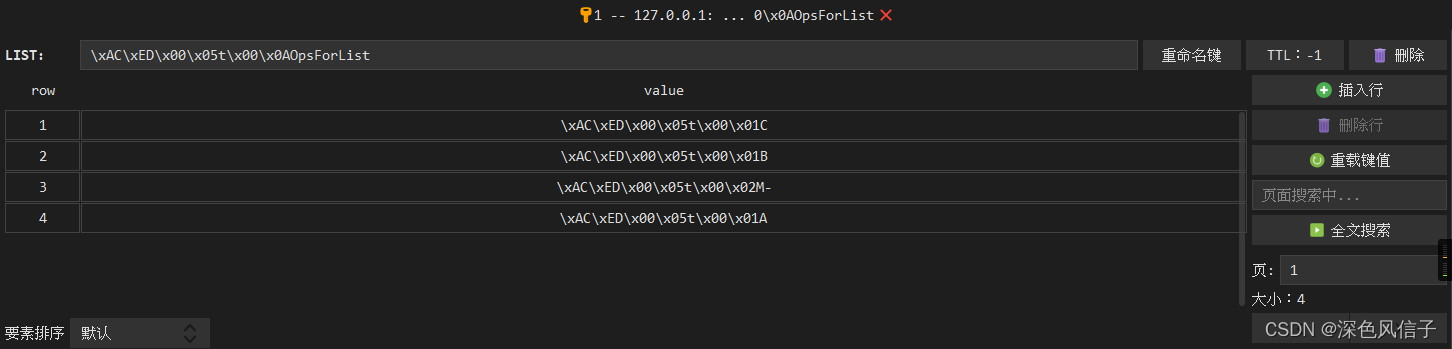 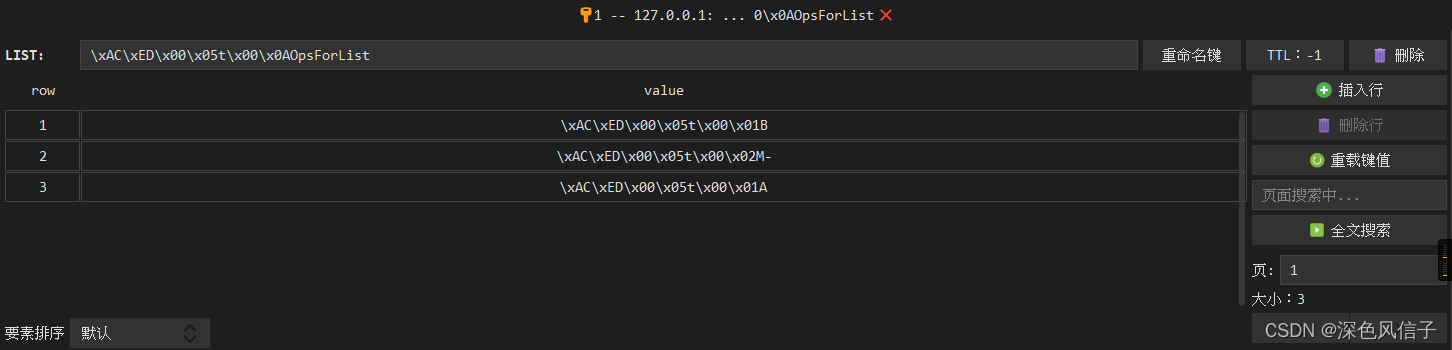
6 List<V> leftPop(K key, long count)
K 是否存在 | 操作 | 返回 |
---|
是 | 获取左边count个元素(栈顶count个元素)并移除 | 左边count个元素 | 否 | 不做操作 | 空 List |
@Test
public void leftPop() {
List<Object> value = redisTemplate.opsForList().leftPop("OpsForList", 2);
System.out.println(value);
}
[C, B]
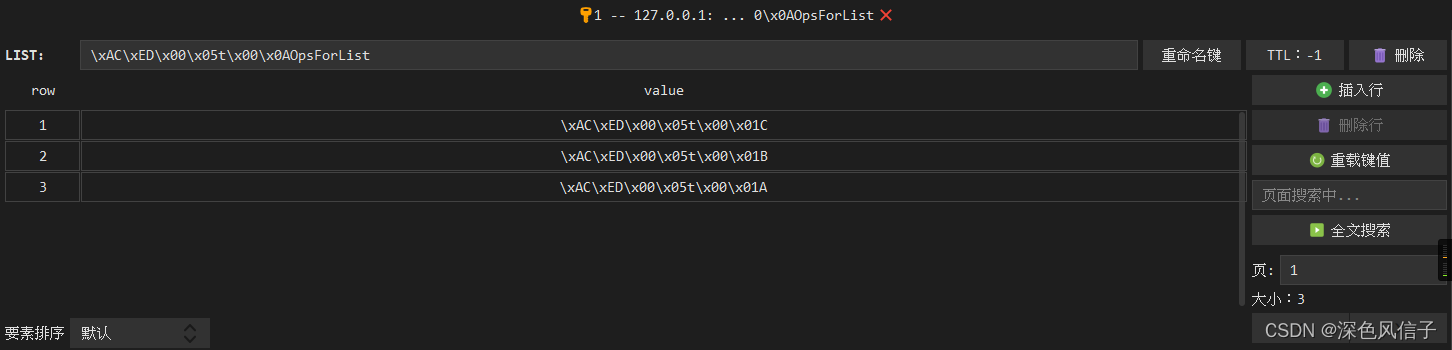 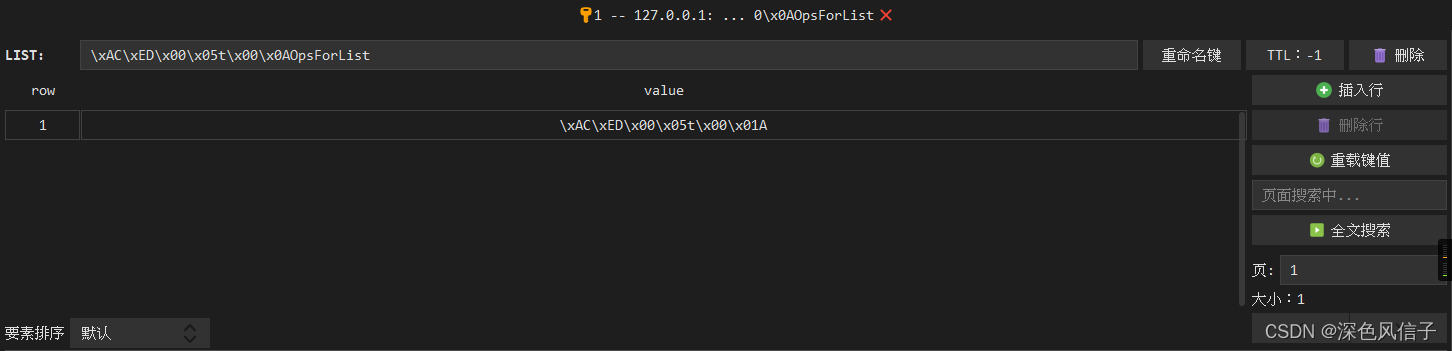
7 V leftPop(K, long, TimeUnit)
K 是否存在 | 操作 | 返回 |
---|
是 | 为左边第一个元素(栈顶元素)设置超时时间 | 左边第一个元素 | 否 | 不做操作 | null |
@Test
public void leftPop() {
String value = (String) redisTemplate.opsForList().leftPop("OpsForList", 5, TimeUnit.SECONDS);
System.out.println(value);
}
C
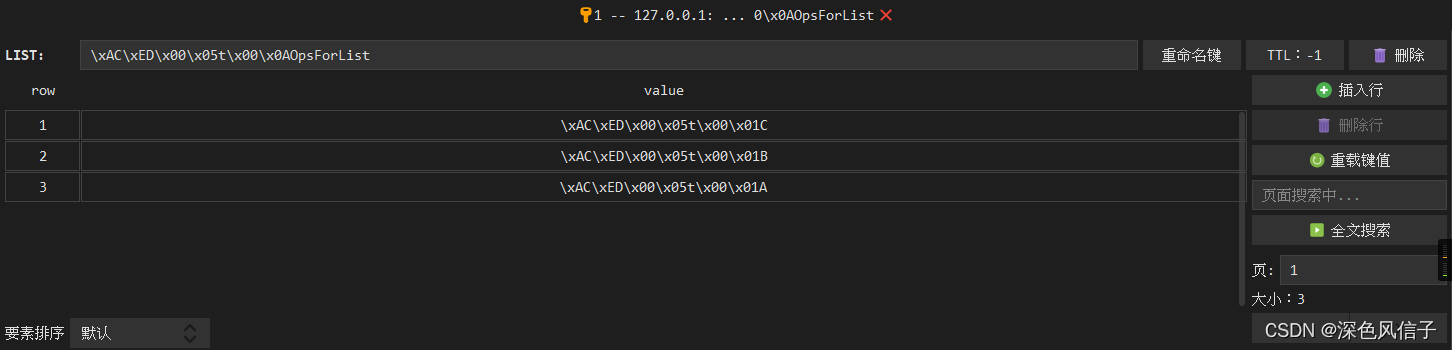 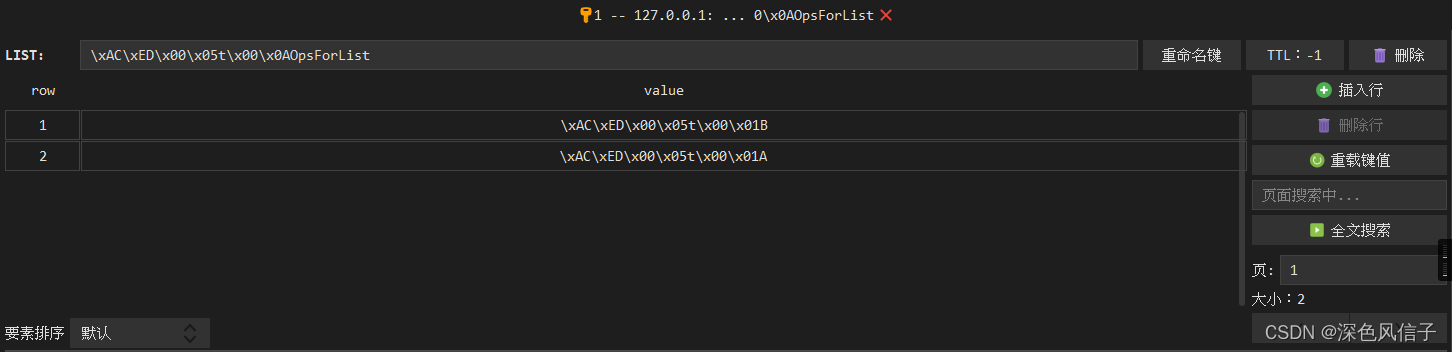
8 Long rightPush(K, V)
和left的一样,方向相反
9 Long rightPush(K, V, V)
和left的一样,方向相反
10 Long rightPushAll(K, Collection)
和left的一样,方向相反
11 Long rightPushAll(K, V…)
和left的一样,方向相反
12 Boolean rightPushIfPresent(K, V)
和left的一样,方向相反
13 rightPop(K)
和left的一样,方向相反
14 rightPop(K, long)
和left的一样,方向相反
15 rightPop(K, long, TimeUnit)
和left的一样,方向相反
16 V rightPopAndLeftPush(K sourceKey, K destinationKey)
sourceKey 和 destinationKey 是否一样 | 操作 | 返回 |
---|
是 | 移除右边的第一个元素,将该元素在左边增加 | 右边的第一个元素 | 否 | 创建新的键值对,destinationKey为key,右边的第一个元素为value | 右边的第一个元素 |
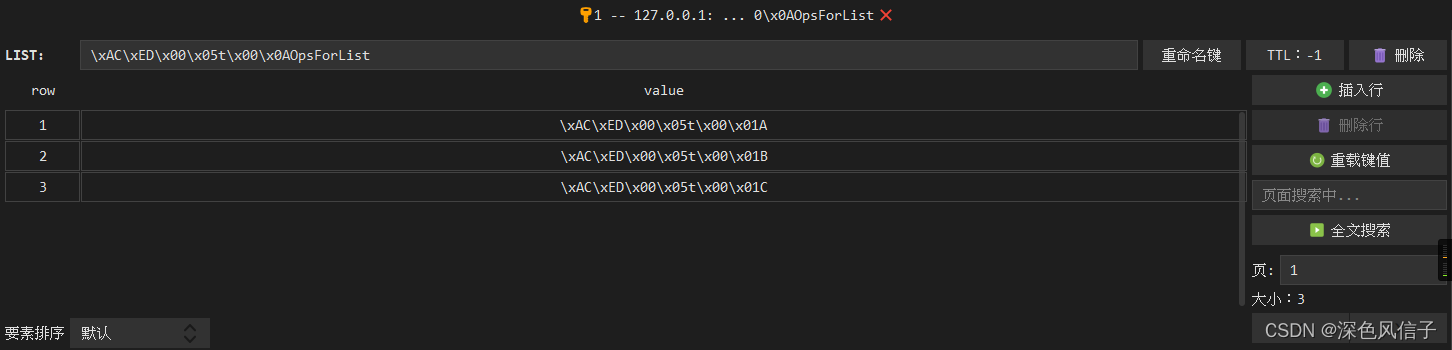 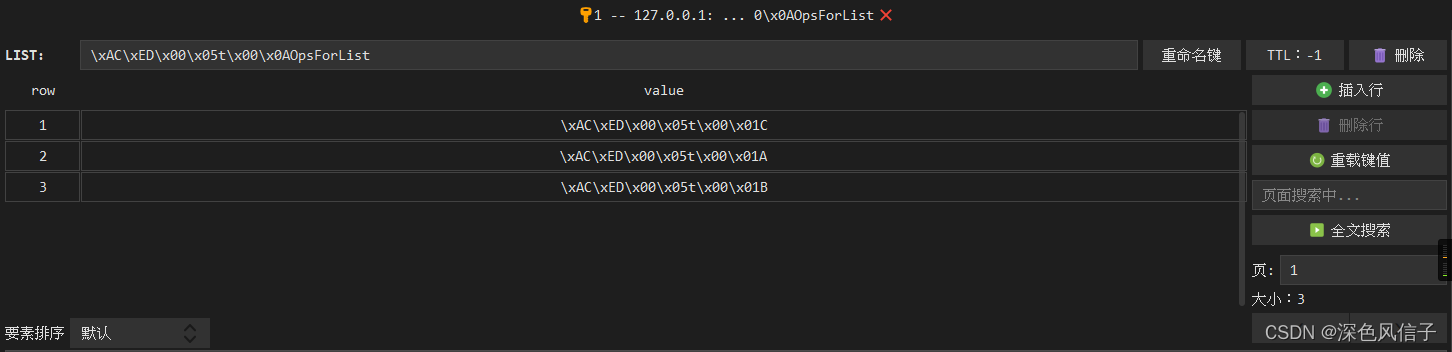
17 rightPopAndLeftPush(K, K, long, TimeUnit)
移除集合中右边的元素在等待的时间里,同时在左边添加元素,如果超过等待的时间仍没有元素则退出。
@Test
public void rightPopAndLeftPush() {
String value = (String) redisTemplate.opsForList().rightPopAndLeftPush("OpsForList", "OpsForList", 5, TimeUnit.SECONDS);
System.out.println(value);
}
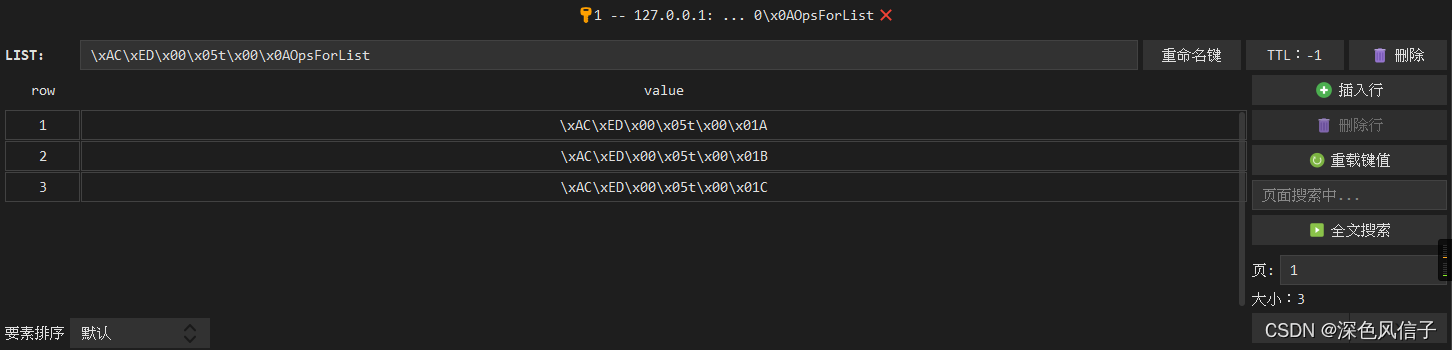
18 V (K key, long index)
K 是否存在 | index 是否超过 | 返回 |
---|
是 | 否 | index位置对应的value | 是 | 是 | null | 否 | - | null |
@Test
public void index() {
String value = (String) redisTemplate.opsForList().index("OpsForList", 0);
System.out.println(value);
}
A
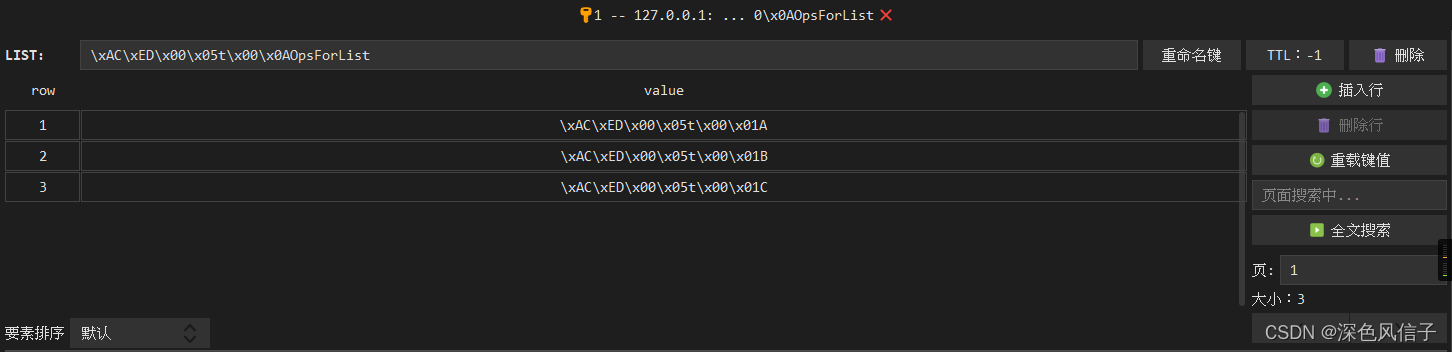
19 Long indexOf(K key, V value)
K 是否存在 | value 是否存在 | 返回 |
---|
是 | 是 | 第一个 value 的 index | 是 | 否 | null | 否 | - | null |
@Test
public void indexOf() {
Long value = redisTemplate.opsForList().indexOf("OpsForList", "A");
System.out.println(value);
}
0
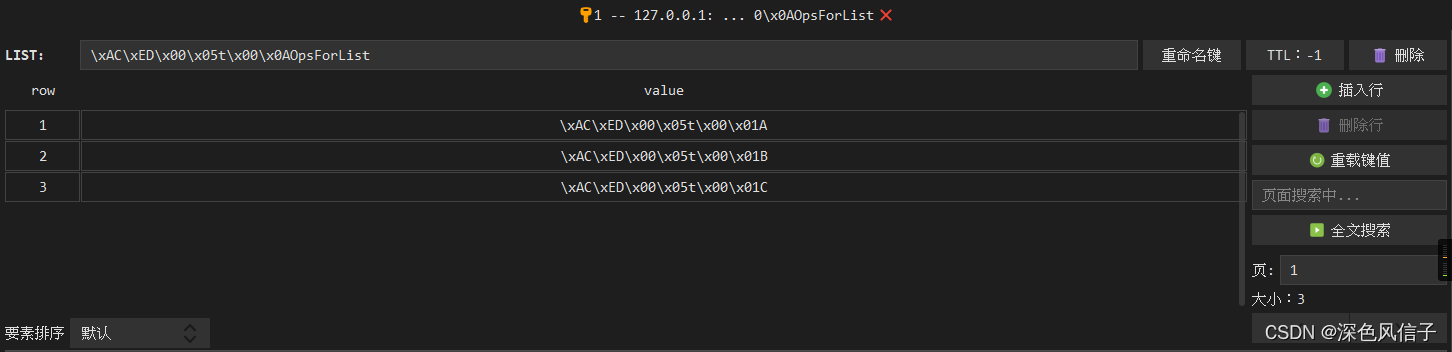
20 lastIndexOf(K key, V value)
K 是否存在 | value 是否存在 | 返回 |
---|
是 | 是 | 第最后一个 value 的 index | 是 | 否 | null | 否 | - | null |
@Test
public void indexOf() {
Long value = redisTemplate.opsForList().lastIndexOf("OpsForList", "A");
System.out.println(value);
}
3
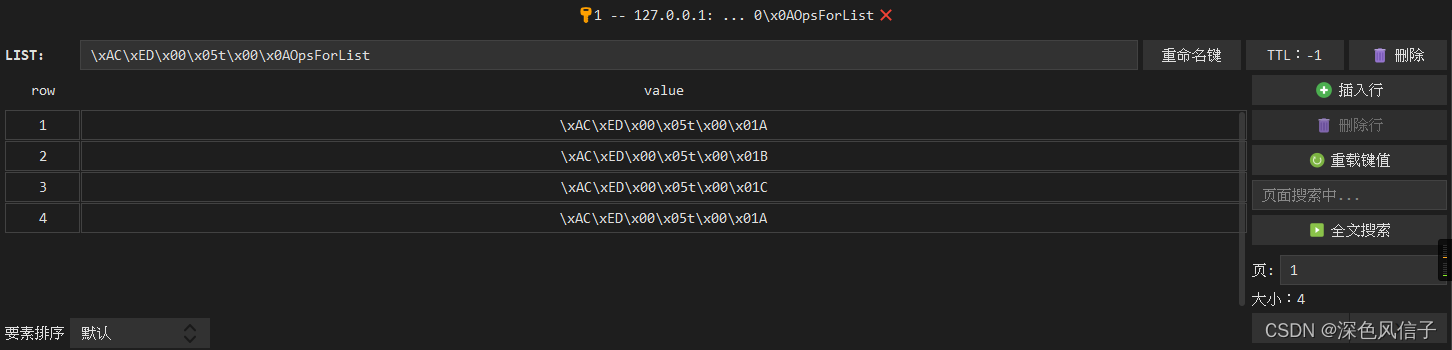 move(K, Direction, K, Direction) move(K, Direction, K, Direction, long, TimeUnit)
range(K, long, long) remove(K, long, Object)
set(K, long, V) size(K) trim(K, long, long)
更新中…
|