整合Junit
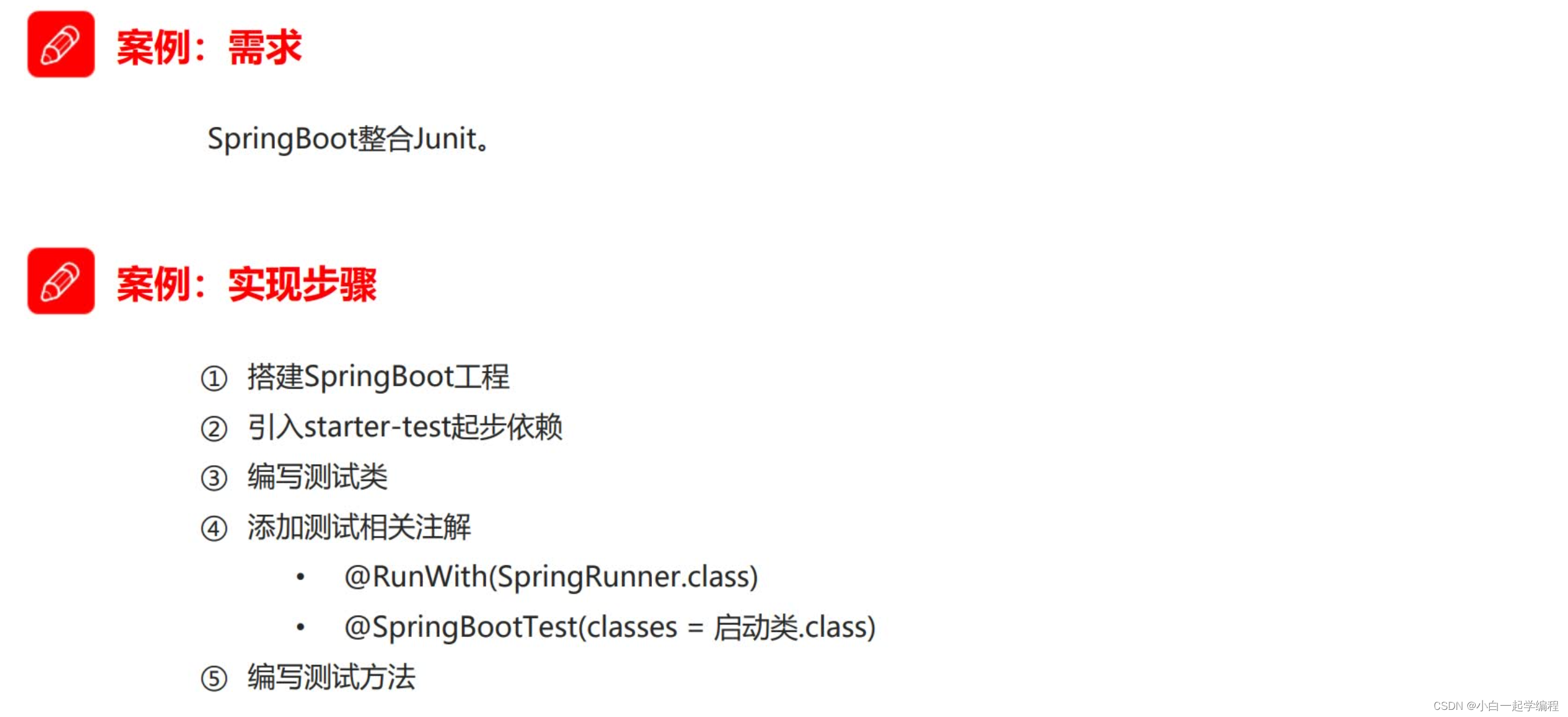
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
package com.example.test;
import com.example.springbootinit.SpringbootInitApplication;
import com.example.springbootinit.service.UserService;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.context.junit4.SpringRunner;
@SpringBootTest(classes = SpringbootInitApplication.class)
@RunWith(SpringRunner.class)
public class SpringbootInitApplicationTests {
@Autowired
private UserService userService;
@Test
public void test1() {
System.out.println(userService);
}
}
整合Mybatis
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
注解使用
@Mapper
public interface UserMapper {
@Select("select * from t_user")
public List<User> findall();
}
xml使用
@Mapper
public interface UserXmlMapper {
public List<User> findall();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.springbootinit.mapper.UserXmlMapper">
<select id="findall" resultType="user">
select * from testdb.t_user
</select>
</mapper>
配置
spring:
profiles:
active: dev
datasource:
url: jdbc:mysql:///testdb
username: iplat62
password: iplat62
driver-class-name: com.mysql.jdbc.Driver
mybatis:
mapper-locations: classpath:mapper/*Mapper.xml
type-aliases-package: com.example.springbootinit.domain
配置映射文件扫描
@MapperScan("com.example.springbootinit.mapper")
整合Redis
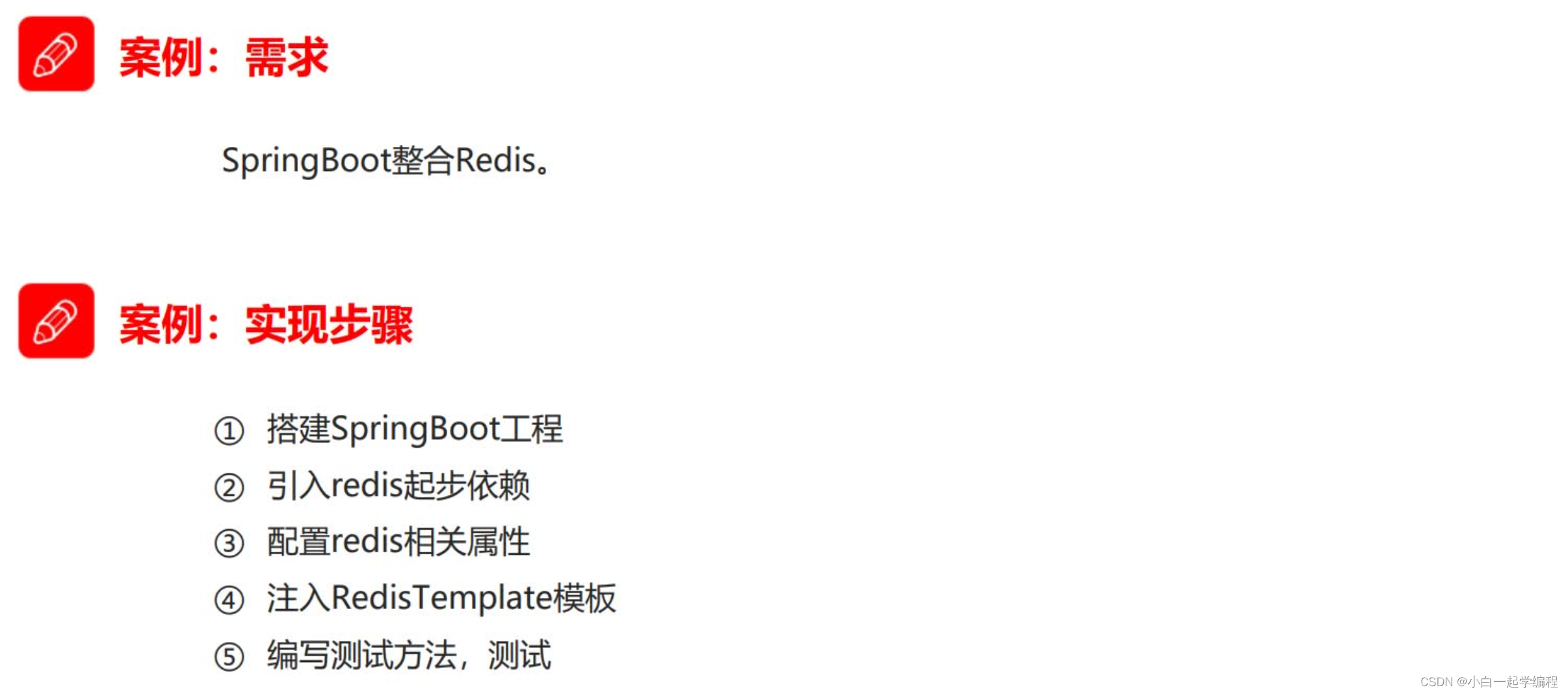
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
|