????????本篇文章将MyBatis引入到贯穿项目中,并将学生和班级的关系在展示学生, 新增学生中体现。 ????????效果如下: 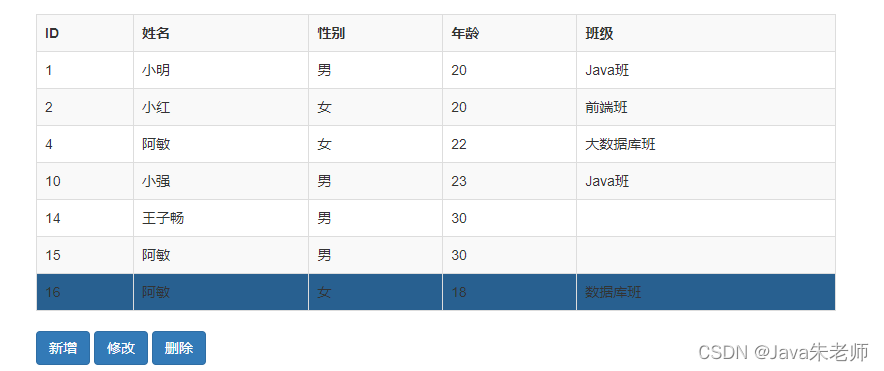 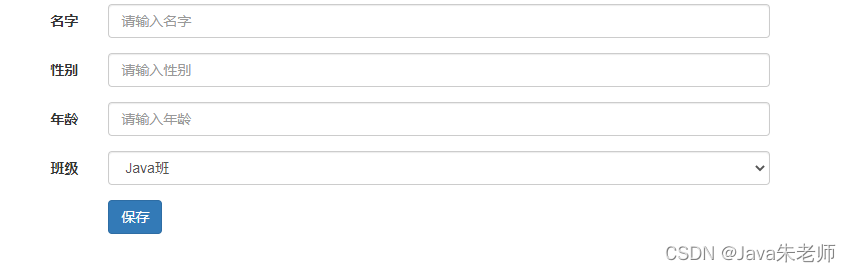 ????????项目当前使用的技术是Spring MVC+MyBatis,项目结构如下: 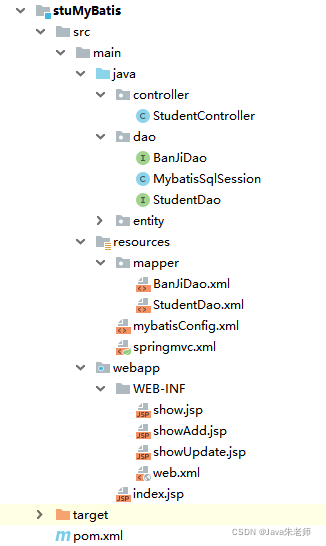 ???????? StudentDao.java:
package dao;
import entity.Student;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface StudentDao {
List<Student> searchAll();
Student search(int id);
int add(Student stu);
int update(Student stu);
int delete(int id);
}
BanJiDao.java:
package dao;
import entity.BanJi;
import java.util.List;
public interface BanJiDao {
List<BanJi> searchAll();
}
StudentDao.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC
"-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="dao.StudentDao">
<select id="searchAll" resultMap="stuAndBj">
select s.*,bj.name as bj_name from student as s left join banji as bj on s.bj_id=bj.id
</select>
<resultMap id="stuAndBj" type="entity.Student">
<id column="id" property="id"/>
<result column="name" property="name"/>
<result column="gender" property="gender"/>
<result column="age" property="age"/>
<association property="bj" column="bj_id" javaType="entity.BanJi">
<id column="bj_id" property="id"/>
<result column="bj_name" property="name"/>
</association>
</resultMap>
<select id="search" resultType="entity.Student">
select * from student where id=#{id}
</select>
<insert id="add">
insert into student (name,gender,age,bj_id) values(#{name},#{gender},#{age},#{bj.id})
</insert>
<update id="update">
update student set name=#{name},gender=#{gender},age=#{age} where id=#{id}
</update>
<delete id="delete">
delete from student where id=#{id}
</delete>
</mapper>
BanJiDao.xml:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC
"-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="dao.BanJiDao">
<select id="searchAll" resultType="entity.BanJi">
select * from banji
</select>
</mapper>
StudentController.xml:
package controller;
import dao.BanJiDao;
import dao.MybatisSqlSession;
import dao.StudentDao;
import entity.BanJi;
import entity.Student;
import org.apache.ibatis.session.SqlSession;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import java.util.List;
@Controller
public class StudentController {
@RequestMapping("show")
public ModelAndView search() {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
StudentDao stuDao = sqlSession.getMapper(StudentDao.class);
List<Student> list = stuDao.searchAll();
ModelAndView mv = new ModelAndView("show");
mv.addObject("stus", list);
sqlSession.close();
return mv;
}
@RequestMapping("showAdd")
public ModelAndView showAdd() {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
BanJiDao bjDao=sqlSession.getMapper(BanJiDao.class);
List<BanJi> bjList=bjDao.searchAll();
ModelAndView mv = new ModelAndView("showAdd");
mv.addObject("bjs",bjList);
return mv;
}
@RequestMapping("add")
public String add(Student stu) {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
StudentDao stuDao = sqlSession.getMapper(StudentDao.class);
int flag = stuDao.add(stu);
sqlSession.commit();
sqlSession.close();
if (flag>0) {
return "redirect:show.do";
}
return null;
}
@RequestMapping("showUpdate")
public ModelAndView showUpdate(int id) {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
StudentDao stuDao = sqlSession.getMapper(StudentDao.class);
Student stu = stuDao.search(id);
ModelAndView mv = new ModelAndView("showUpdate");
mv.addObject("stu", stu);
sqlSession.commit();
sqlSession.close();
return mv;
}
@RequestMapping("update")
public String update(Student stu) {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
StudentDao stuDao = sqlSession.getMapper(StudentDao.class);
int flag = stuDao.update(stu);
sqlSession.commit();
sqlSession.close();
if (flag>0) {
return "redirect:show.do";
}
return null;
}
@RequestMapping("delete")
public String delete(int id) {
SqlSession sqlSession = MybatisSqlSession.getSqlSession();
StudentDao stuDao = sqlSession.getMapper(StudentDao.class);
int flag = stuDao.delete(id);
sqlSession.commit();
sqlSession.close();
if (flag>0) {
return "redirect:show.do";
}
return null;
}
}
show.jsp:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" %>
<%@page import="java.util.List,entity.*" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<link rel="stylesheet"
href="https://cdn.staticfile.org/twitter-bootstrap/3.3.7/css/bootstrap.min.css">
<style>
#container {
width: 800px;
margin: 20px auto;
}
#container .selected {
background: #286090;
}
</style>
<script src="https://cdn.staticfile.org/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function () {
var id = 0;
$("#showAdd").click(function () {
location.href = "showAdd.do";
})
$(".data").click(function () {
id = $(this).children().eq(0).text();
$(".data").removeClass("selected")
$(this).addClass("selected")
})
$("#showUpdate").click(function () {
if (id > 0) {
location.href = "showUpdate.do?id="+id;
}else{
alert("请选中一条数据")
}
})
$("#delete").click(function () {
if (id > 0) {
location.href = "delete.do?id="+id;
}else{
alert("请选中一条数据")
}
})
})
</script>
</head>
<body>
<%
List<Student> list = (List<Student>) request.getAttribute("stus");
%>
<div id="container">
<table class="table table-striped table-bordered table-hover">
<tr>
<th>ID</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
<th>班级</th>
</tr>
<%
for (int i = 0; i < list.size(); i++) {
%>
<tr class="data">
<td><%=list.get(i).getId()%>
</td>
<td><%=list.get(i).getName()%>
</td>
<td><%=list.get(i).getGender()%>
</td>
<td><%=list.get(i).getAge()%>
</td>
<td><%=list.get(i).getBj()!=null?list.get(i).getBj().getName():""%>
</td>
</tr>
<%
}
%>
</table>
<button type="button" class="btn btn-primary" id="showAdd">新增</button>
<button type="button" class="btn btn-primary" id="showUpdate">修改</button>
<button type="button" class="btn btn-primary" id="delete">删除</button>
</div>
</body>
</html>
showAdd.jsp:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" %>
<%@page import="java.util.List,entity.*" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<link rel="stylesheet"
href="https://cdn.staticfile.org/twitter-bootstrap/3.3.7/css/bootstrap.min.css">
<style>
#container {
width: 800px;
margin: 20px auto;
}
</style>
</head>
<body>
<%
List<BanJi> bjList = (List<BanJi>) request.getAttribute("bjs");
%>
<div id="container">
<form class="form-horizontal" action="add.do">
<div class="form-group">
<label class="col-sm-2 control-label">名字</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="name" placeholder="请输入名字">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">性别</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="gender" placeholder="请输入性别">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">年龄</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="age" placeholder="请输入年龄">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">班级</label>
<div class="col-sm-10">
<select class="form-control" name="bj.id">
<% for (int i = 0; i < bjList.size(); i++) {%>
<option value="<%=bjList.get(i).getId()%>"><%=bjList.get(i).getName()%></option>
<%}%>
</select>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<button type="submit" class="btn btn-primary">保存</button>
</div>
</div>
</form>
</div>
</body>
</html>
|