项目目录:
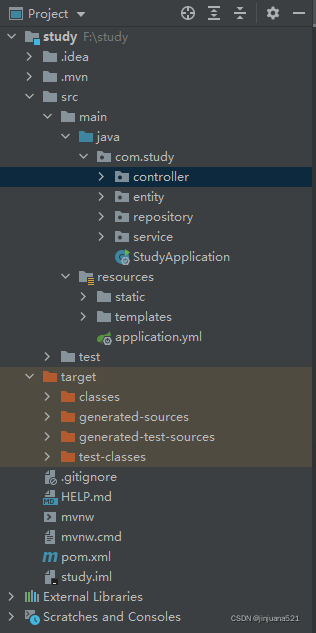
1.pom文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.jin</groupId>
<artifactId>study</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>study</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
2.application.yml文件?
##tomcat 端口
server:
port: 5008
##数据连接
spring:
datasource:
url: jdbc:mysql://192.168.100.168:5000/smart_line_xj?characterEncoding=utf8&useSSL=false
username: root
password: 123456
driver-class-name: com.mysql.cj.jdbc.Driver
#thymeleaf配置
thymeleaf:
prefix: classpath:/templates/
suffix: .html
mode: HTML5
encoding: UTF-8
content-type: text/html
cache: false
#jpa的指向工程
jpa:
hibernate:
ddl-auto: update
show-sql: true
3.创建实体类
(@Entity 注解实体类? ?@Table 注解匹配的数据库表名? )
(实现 Serializable)
(属性进行getter 、setter、toString)
package com.study.entity;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Table;
import java.io.Serializable;
@Entity
@Table(name = "tb_user")
public class Users implements Serializable {
private String username;
//密码
private String password;
//加密种子
private String salt;
private String email;
private String portrait;
private String realname;
private Integer state;
private String registration;
private String url;
private Long id;
public void setId(Long id) {
this.id = id;
}
@Id
public Long getId() {
return id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getSalt() {
return salt;
}
public void setSalt(String salt) {
this.salt = salt;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPortrait() {
return portrait;
}
public void setPortrait(String portrait) {
this.portrait = portrait;
}
public String getRealname() {
return realname;
}
public void setRealname(String realname) {
this.realname = realname;
}
public Integer getState() {
return state;
}
public void setState(Integer state) {
this.state = state;
}
public String getRegistration() {
return registration;
}
public void setRegistration(String registration) {
this.registration = registration;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
@Override
public String toString() {
return "Users{" +
"username='" + username + '\'' +
", password='" + password + '\'' +
", salt='" + salt + '\'' +
", email='" + email + '\'' +
", portrait='" + portrait + '\'' +
", realname='" + realname + '\'' +
", state=" + state +
", registration='" + registration + '\'' +
", url='" + url + '\'' +
", id=" + id +
'}';
}
}
4.创建接口类DAO,? JPA中用repository
(@Repository 注解repository类)
(?继承JpaRepository类 <实体类类型,实体类id类型>)
(无需写接口)
package com.study.repository;
import com.study.entity.Users;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UsersRepository extends JpaRepository <Users,Long>{
}
5.创建service
(@Service 注解service层? @Transactional 注解事务)
package com.study.service;
import com.study.entity.Users;
import com.study.repository.UsersRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
@Transactional
public class UsersService {
@Autowired
UsersRepository usersRepository;
public Users findUserById(Long id) {
return usersRepository.getById(id);
}
}
6.创建Controller (@Controller 注解Controller层)
(@Controller + @ResponseBody = @RestController? 注意 此处用??@Controller)
package com.study.controller;
import com.study.entity.Users;
import com.study.service.UsersService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
/**
* @Controller + @ResponseBody = @RestController
*/
@Controller
public class StudyController {
@Autowired
UsersService usersService;
@GetMapping("/users/{userid}")
public String findUserById(@PathVariable Long userid, Model model) {
Users user=usersService.findUserById(userid);
model.addAttribute("user", user);
return "index";
}
}
7.创建html
(注意:thymeleaf 文件放在? templates 文件夹下,css、js等文件放在static文件夹下)
<!DOCTYPE html >
<html lang="en" xmlns:th="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" />
<meta charset="utf-8" />
<title>Dashboard - Ace Admin</title>
</head>
<body class="no-skin">
<p th:text="${user.username}"> </p>
</body>
</html>
8.运行SpringBootApplication文件,这里名叫StudyApplication
访问地址:本机ip+application.yml文件设置的端口号+Controller中方法GetMapping的路径和参数? ? ? 出现查询结果:
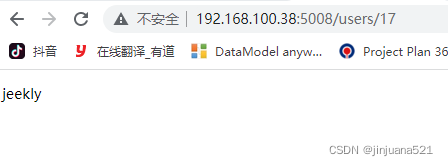
?
|