???????????这是一款闯关的射击游戏,人物在不同的关 卡里面会触发不同的技能与对应的特效操作,有三个关卡与四个随机事件,每个关卡里面都会触发不同数量的怪物与能量血瓶,通过打败怪物与 到达特点时间点可通关
???????????效果图以及关卡里面的演示
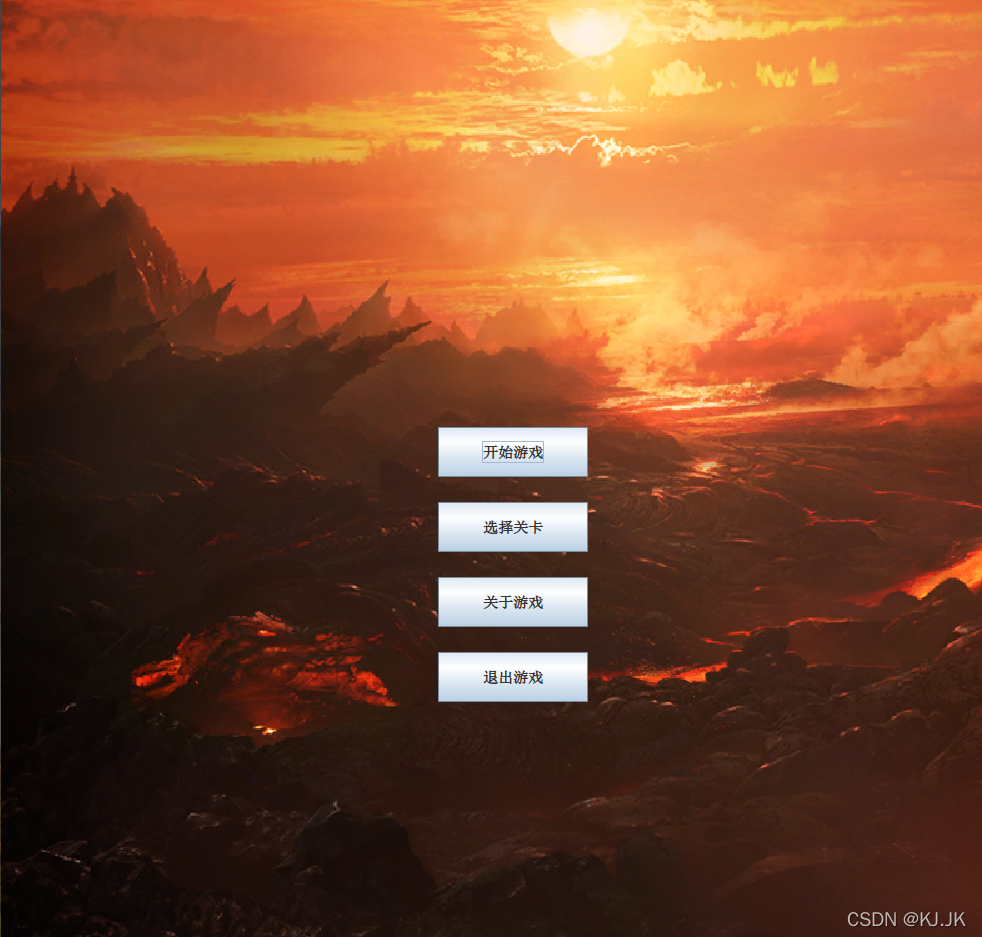 ????????????界面类
package game;
import java.awt.Image;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
class Main extends JFrame implements ActionListener {
Image im1 = (new ImageIcon("src/images/游戏缩小图标.jpeg")).getImage();
ImageIcon icon = new ImageIcon("src/images/bg.jpeg");
JButton btn1 = new JButton("开始游戏");
JButton btn2 = new JButton("选择关卡");
JButton btn3 = new JButton("关于游戏");
JButton btn4 = new JButton("退出游戏");
JPanel jpan = new JPanel();
JLabel jlbBackImg = new JLabel();
Main() {
this.setLayout(null);
this.setTitle("试炼之旅");
this.setIconImage(im1);
this.setBounds(0, 0, 800, 800);
this.setLocationRelativeTo(null);
jpan.setBounds(0, 0, 800, 800);
jpan.setLayout(null);
btn1.setBounds(350, 350, 120, 40);
btn2.setBounds(350, 410, 120, 40);
btn3.setBounds(350, 470, 120, 40);
btn4.setBounds(350, 530, 120, 40);
btn1.addActionListener(this);
btn2.addActionListener(this);
btn3.addActionListener(this);
btn4.addActionListener(this);
jlbBackImg.setBounds(0, 0, 800, 800);
jlbBackImg.setIcon(icon);
jpan.add(btn1);
jpan.add(btn2);
jpan.add(btn3);
jpan.add(btn4);
jpan.add(jlbBackImg);
this.add(jpan);
this.setResizable(false);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn1) {
this.dispose();
new Game();
}
if (e.getSource() == btn2) {
this.dispose();
new selectGame();
}
if (e.getSource() == btn3) {
int value = JOptionPane.showOptionDialog(this,
"关于游戏:第一关人物“WSAD”控制方向,“鼠标左键发射”,“按键Q消耗分数回血”" + "需要躲避周围射击出现的热旋。\n" + " "
+ "第二关人物1“WSAD”控制方向,“鼠标左键发射”,“按键Q消耗分数回血”,“按键K消耗能量释放技能”;“人物2箭头控制方向”,“右键发射攻击特定怪物”。\n"
+ " "
+ "第三关人物“WASD”控制方向,“鼠标左键发射”,“按键Q消耗分数回血”,“按键K消耗能量释放技能”,“达到1500分数后解锁右键射击,R键特殊功能会在第二个Boss解锁”\n"
+ " " + "“F1重新开始本关”" + "“F2”返回游戏主界面,“F3”退出游戏。游戏愉快!",
"试炼之旅", JOptionPane.YES_OPTION, JOptionPane.PLAIN_MESSAGE, null, null, null);
}
if (e.getSource() == btn4) {
System.exit(0);
}
}
public static void main(String[] args) {
new Main();
}
}
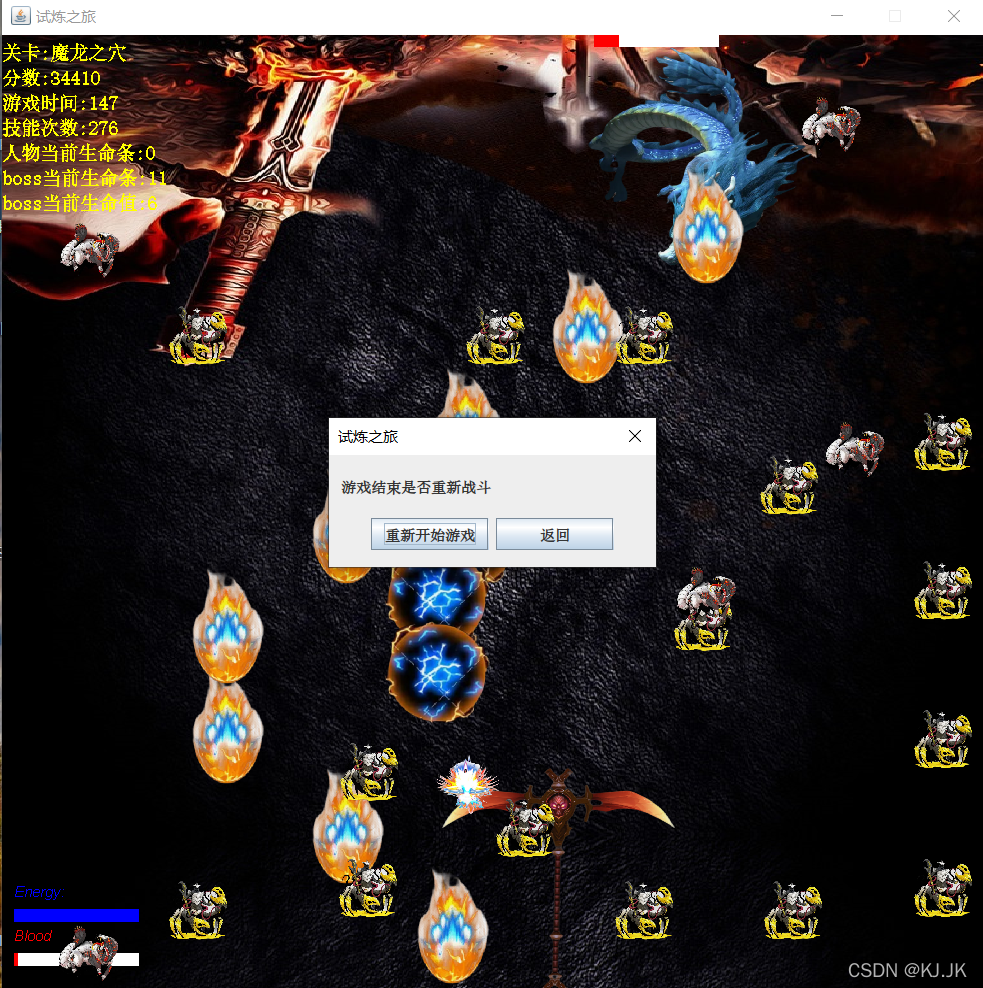 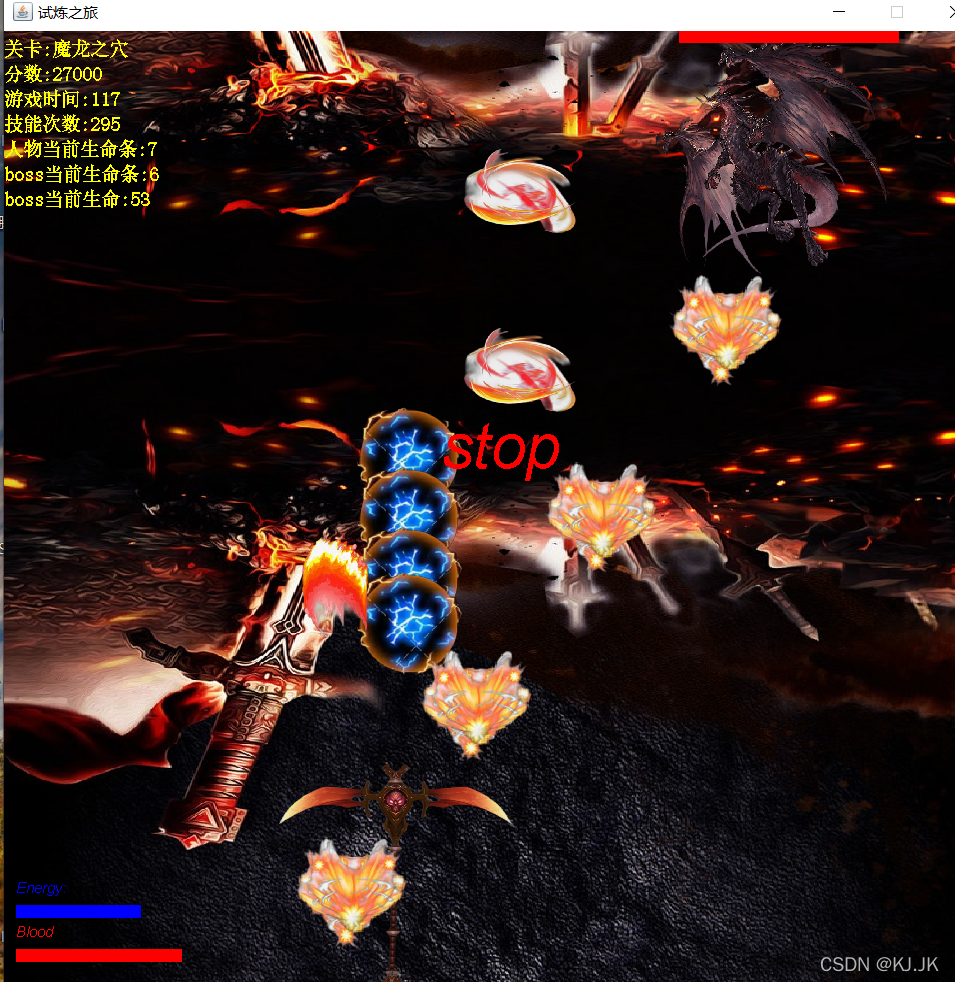 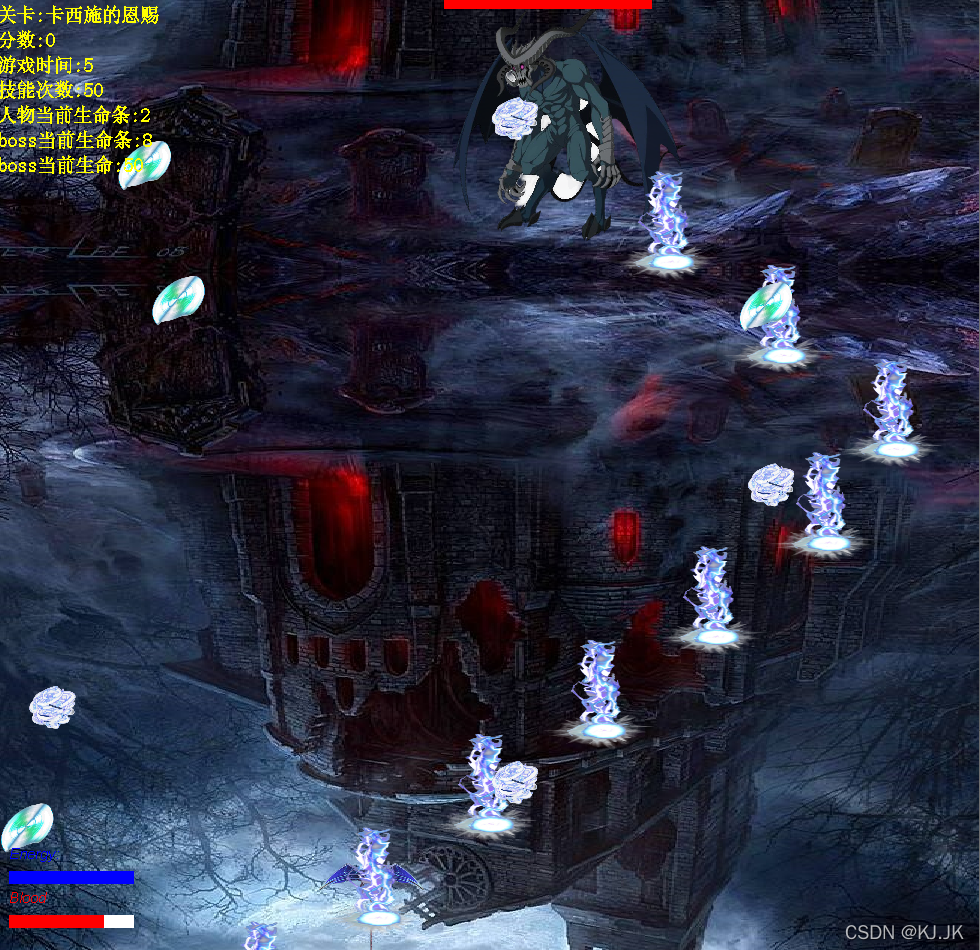 ???????????关卡的绘制类
@Override
public void paint(Graphics gh) {
if (gamerun == 1) {
if (bg1y >= 800) {
bg1y = -800;
}
if (bg2y >= 800) {
bg2y = -800;
}
bg1y += 10;
bg2y += 10;
gh.drawImage(ic1, bg1x, bg1y, 800, 800, null);
gh.drawImage(ic2, bg2x, bg2y, 800, 800, null);
man.move(gh);
gametimeBoss1.paintSelf2(gh);
for (int i = 0; i < shocks.size(); i++) {
shocks.get(i).drowshock(gh);
}
for (int i = 0; i < timebossbullets.size(); i++) {
timebossbullets.get(i).drowshock2(gh);
}
for (int i = 0; i < skills.size(); i++) {
skills.get(i).drowshock2(gh);
}
for (int i = 0; i < gb.length; i++) {
gb[i].drawShell(gh);
gb[i].drawShells(gh);
if (gb[i].getRect().intersects(man.getRect())) {
Explode explode = new Explode(man.x, man.y);
explode.draw(gh);
explodes.add(explode);
manlife1 -= 10;
break;
}
if (gb[i].getRect1().intersects(man.getRect())) {
Explode explode = new Explode(man.x, man.y);
explode.draw(gh);
explodes.add(explode);
manlife1 -= 10;
break;
}
}
相关的素材包 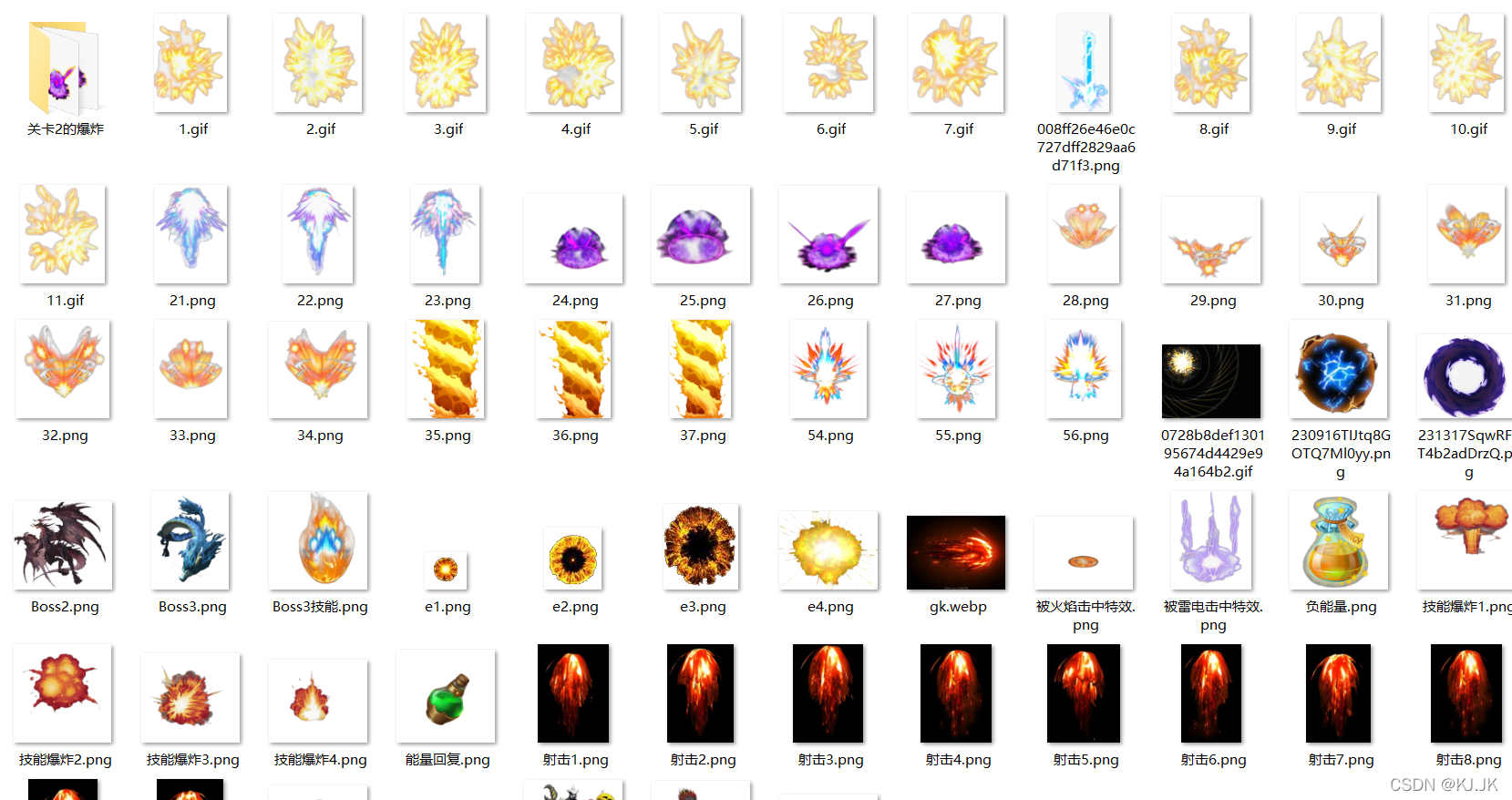
????????????随机Boss的子弹类
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
class gametimeboss {
Image ima = (new ImageIcon("src/timebossshock/随机事件.png")).getImage();
protected int speed = 15;
protected double degree = Math.random() * Math.PI * 5;
protected int Shellx = 300, Shelly = 300;
public Rectangle getRect() {
return new Rectangle(Shellx, Shelly, 35, 35);
}
public void drawShell(Graphics gh) {
Shellx += speed * Math.cos(degree);
Shelly += speed * Math.sin(degree);
if (Shellx < 0 || Shellx > 760) {
degree = Math.PI - degree;
}
if (Shelly < 0 || Shelly > 760) {
degree = -degree;
}
gh.drawImage(ima, Shellx, Shelly, 40, 40, null);
}
Image ima1 = (new ImageIcon("src/timebossshock/随机事件1.png")).getImage();
protected int speed1 = 12;
protected double degree1 = Math.random() * 10;
protected int Shellx1 = 400, Shelly1 = 250;
public Rectangle getRect1() {
return new Rectangle(Shellx, Shelly, 35, 35);
}
public void drawShells(Graphics gh) {
Shellx1 += speed1 * Math.cos(degree1);
Shelly1 += speed1 * Math.sin(degree1);
if (Shellx1 < 0 || Shellx1 > 760) {
degree1 = Math.PI - degree1;
}
if (Shelly1 < 0 || Shelly1 > 760) {
degree1 = -degree1;
}
gh.drawImage(ima1, Shellx1, Shelly1, 50, 50, null);
}
Image power2 = (new ImageIcon("src/bossimg/power2.png")).getImage();
protected int powerspeed = 10;
protected double powerdegree = Math.random() * 15;
protected int powerx = 400, powery = 250;
public Rectangle getRect2() {
return new Rectangle(powerx, powery, 30, 30);
}
public void drawpower(Graphics gh) {
powerx += powerspeed * Math.cos(powerdegree);
powery += powerspeed * Math.sin(powerdegree);
if (powerx < 0 || powerx > 760) {
powerdegree = Math.PI - powerdegree;
}
if (powery < 0 || powery > 760) {
powerdegree = -powerdegree;
}
gh.drawImage(power2, powerx, powery, 50, 50, null);
}
}
????????????部分的人物类
package game;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Rectangle;
import java.awt.event.KeyEvent;
import javax.swing.ImageIcon;
class Man3 {
protected int x = 300, y = 600;
protected boolean shang, xia, zuo, you;
protected boolean live = true;
final int speed = 12;
Image man3 = (new ImageIcon("src/images/man3.png")).getImage();
Image imgb = (new ImageIcon("src/images/特效1.png")).getImage();
public void move(Graphics gh) {
if (live) {
if (shang && y > 100) {
y -= speed;
}
if (xia && y < 680) {
y += speed;
}
if (zuo && x > 0) {
x -= speed;
}
if (you && x < 700) {
x += speed;
}
gh.drawImage(man3, x, y, 200, 200, null);
}
}
public Rectangle getRect() {
return new Rectangle(x, y, 100, 100);
}
public void move(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_W:
shang = true;
break;
case KeyEvent.VK_S:
xia = true;
break;
case KeyEvent.VK_A:
zuo = true;
break;
case KeyEvent.VK_D:
you = true;
break;
}
}
public void dismove(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_W:
shang = false;
break;
case KeyEvent.VK_S:
xia = false;
break;
case KeyEvent.VK_A:
zuo = false;
break;
case KeyEvent.VK_D:
you = false;
break;
}
}
}
需要源代码以及完整图片请点赞留言博主 就等你们来了,心动不如行动,赶快留言吧
作者:KJ.JK 本文仅用于交流学习,未经作者允许,禁止转载,更勿做其他用途,违者必究。 文章对你有所帮助的话,欢迎给个赞或者 star 呀,你的支持是对作者最大的鼓励,不足之处可以在评论区多多指正,交流学习呀
|