双冒号作用:
jdk1.8中lambda 经常需要操作函数式接口表达式,表达式也是需要人写出来的,如果有现成的,那么久不需要写了,这里的双冒号其实就是对方法体的一种引用。
以下是Java 8中方法引用的一些语法:
1、静态方法引用(static method)语法:classname::methodname 例如:Person::getAge 2、对象的实例方法引用语法:instancename::methodname 例如:System.out::println 3、对象的超类方法引用语法: super::methodname 4、类构造器引用语法: classname::new 例如:ArrayList::new 5、数组构造器引用语法: typename[]::new 例如: String[]:new
静态方法语法示例:
import java.util.Arrays;
import java.util.List;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ComponentScan("com.zhoufy")
public class Demo {
@Test
public void test() {
List<String> list = Arrays.asList("aaaa", "bbbb", "cccc");
list.forEach(Demo::print);
}
public static void print(String content){
System.out.println(content);
}
}
类实例方法语法使用示例:
import java.util.Arrays;
import java.util.List;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ComponentScan("com.zhoufy")
public class Demo {
@Test
public void test() {
List<String> list = Arrays.asList("aaaa", "bbbb", "cccc");
list.forEach(new Demo()::print);
}
public void print(String content){
System.out.println(content);
}
}
超类方法语法使用示例:
import java.util.Arrays;
import java.util.List;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ComponentScan("com.zhoufy")
public class Example extends BaseExample{
@Test
public void test() {
List<String> list = Arrays.asList("aaaa", "bbbb", "cccc");
list.forEach(super::print);
}
}
class BaseExample {
public void print(String content){
System.out.println(content);
}
}
类构造器语法使用示例:某个接口的内部类是个表达式,表达式借用对象的无惨构造器来完成。
无惨构造:
public class Example {
public Example() {
}
@Test
public void test() {
Runnable aNew = Example::new;
InterfaceExample com = Example::new;
InterfaceExample com2 = Example::new;
Example bean = com.create();
System.out.println(bean);
}
interface InterfaceExample{
Example create();
}
}
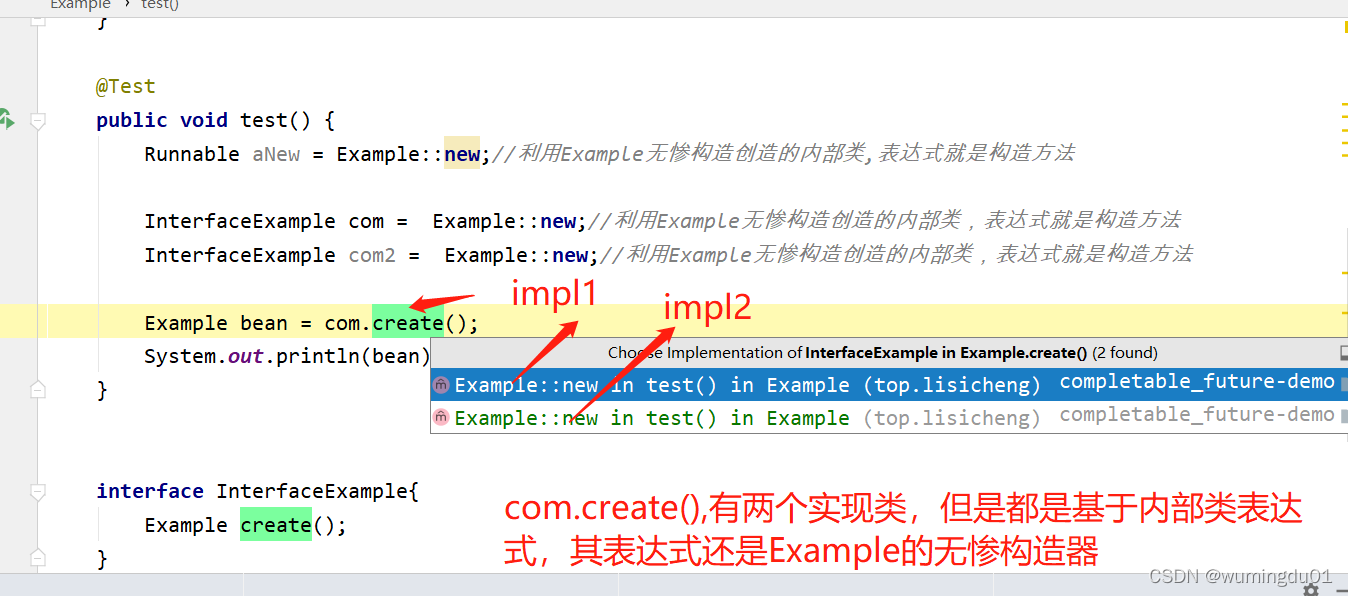
有参构造:
public class Example {
private String name;
Example(String name){
this.name = name;
}
@Test
public void test() {
InterfaceExample com = Example::new;
InterfaceExample com3 = (str)->{return new Example(str);};
Example bean = com.create("用的是Example的有参构造器当表达式");
System.out.println(bean);
}
interface InterfaceExample{
Example create(String name);
}
}
数组构造器语法使用例子:
package top.lisicheng;
import java.util.function.Function;
public class Exampl {
public Exampl[] Exampl(Integer integer) {
Exampl[] qqq = new Exampl[integer];
return qqq;
}
public static void main(String[] args) {
Function<Integer, Exampl[]> integerFunction1 = new Function<Integer, Exampl[]>() {
@Override
public Exampl[] apply(Integer integer) {
return new Exampl[integer];
}
};
Function<Integer, Exampl[]> integerFunction2 = Exampl[]::new;
Exampl[] array = integerFunction2.apply(4);
for(Exampl e:array){
System.out.println(e);
}
}
}
|