全局配置文件
全局配置文件能够对一些默认配置值进行修改,Spring Boot使用一个application.properties或者application.yaml的文件配置文件,该文件存放在src/main/resource目录或者类路径的/config。
application.properties配置文件
使用Spring Initializer方式构建Spring Boot项目,会在resource目录下生成自动生成一个空的application.properties文件,Spring Boot项目启动时会自动加载application.properties文件。 我们可以在application.properties文件中定义Spring Boot项目的相关属性。示例代码如下:
server.address=80
server.port=8443
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.config.additional-location=spring.config.location=
spring.config.name=application
示例: (1)使用Spring Initializr方式创建一个名为peizhi的Spring Boot项目,在Dependencies依赖找那个选择Web依赖 (2)在com.itheima的包下创建一个properties的包。在该包下创建两个实体类Pet和Person Pet.java
package com.itheima.properties;
public class Pet {
private String type;
private String name;
public String getType(){
return type;
}
public void setType(String type){
this.type=type;
}
public String getName(){
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Pet{" +
"type='" + type + '\'' +
", name='" + name + '\'' +
'}';
}
}
Person.java
package com.itheima.properties;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
import java.util.*;
@Component
@ConfigurationProperties(prefix = "person")
public class Person {
private int id;
private String name;
private List hobby;
private String[] family;
private Map map;
private Pet pet;
public void setId(int id) {
this.id = id;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getHobby() {
return hobby;
}
public void setHobby(List hobby) {
this.hobby = hobby;
}
public String[] getFamily() {
return family;
}
public void setFamily(String[] family) {
this.family = family;
}
public Map getMap() {
return map;
}
public void setMap(Map map) {
this.map = map;
}
public Pet getPet() {
return pet;
}
public void setPet(Pet pet) {
this.pet = pet;
}
@Override
public String toString() {
return "Person{" +
"id=" + id +
", name='" + name + '\'' +
", hobby=" + hobby +
", family=" + Arrays.toString(family) +
", map=" + map +
", pet=" + pet +
'}';
}
}
其中@ConfigurationProperties(prefix = “person”)注解的作用是将配置文件中以person开头的属性值通过setter方法注入实体类对应的属性中。@Component注解的作用事件之前注入属性指的Person类对象作为Bean组件放到Spring让其中。 (3)打开resource目录下的application.properties配置文件。
person.id=1
person.name=tom
person.hobby=play,read,sleep
person.family=father,mother
person.map.k1=v1
person.map.k2=v2
person.pet.type=dog
person.pet.name=kity
(4)在项目测试类Chapter02ApplicationTests中引入Person实体类Bean,并进行输出测试
package com.itheima;
import com.itheima.properties.Person;
import org.junit.runner.RunWith;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
@SpringBootTest
class PeizhiApplicationTests {
@Autowired
private Person person;
@Test
void contextLoads() {
System.out.println(person);
}
}

application.yaml配置文件
YAML文件格式是Spring Boot支持的一种JSON超集文件格式,是一种更加直观且容易被计算机识别数据的序列化格式。 YAML文件配置属性的写法 (1)value值为普通数据类型(数字、字符串、布尔等) 当YAML配置文件中配置属性值为普通数据类型时,可以直接配置对应的属性值,同时对于字符串类型的属性值,不需要额外加添加引号。示例代码如下:
server:
port:8081
path:/hello
上述代码用于配置server和path属性,port和path属于同一层级 (2)value值为数组和单列集合 当value值为数组和单列集合类型时,主要有两种书写方法,缩进写法和行内写法 缩进写法的表示形式,示例代码如下:
person:
hobby:
-play
-read
-sleep
或者用如下示例形式
person:
hobby:
play,
read,
sleep
上述代码使用两种形式为person对象的属性hobby赋值。 在YAML配置文件中,可以使用缩写式写法简化为行内式写法
person:
hobby:[play,read,sleep]
使用行内式写法中的[]可以省略,程序会自动匹配校对属性的值 (3)value值为Map集合和对象 当YAML配置文件件中配置的属性值为Maori集合或对象类型时,YAMl配置文件个是分为两种书写形式(缩进式写法和行内式写法) 缩进式写法
person:
mao:
k1:v1
k2:v2
行内式写法
person
map:{k1:v1,k2:v2}
在YAML配置文件的属性值为Map集合或对象类型时缩进式写法的形式按照YAML文件格式编写即可,行内式写法的属性值要用大括号包含。 application.properties配置文件的优先级要高于application.yaml配置文件。 示例: (1)在peizhi项目的resources目录下创建一个application.yaml配置文件,该该配置文件中设置Person对象的属性值
person:
id: 2
name: 张三
hobby: [sing,read,sleep]
family: [father,mother]
map: {k1: v1,k2: v2}
pet: {type:cat,name:tom}
(2)注释掉之前application.properties的内容。因为application.properties的优先级比application.yaml高。 (3)打开测试类,执行测试方法contextLoads()对该Person对象进行输出打印。

配置文件属性值的注入
使用Spring Boot全局配置文件配置属性时,如果配置的属性时SpringBoot默认属性。那么Spring Boot内部会自动扫描并读取属性值,如果配置属性时用户自定义属性,必须在程序中注入这些配置属性才可生效,Spring Boot支持多种注入配置文件属性的方式。
使用@ConfigurationProperties注入属性
Spring Boot提供的@ConfigurationProperties直接用来快速、方便的将配置文件中的属性值批量注入某个Bean对象的多个对应属性中。 示例代码:
@Component
@ConfigurationProperties(prefix = "person")
public class Person {
private int id;
public void setId(int id) {
this.id = id;
}
}
上述代码使用@Component和@ConfigurationProperties(prefix=“person”)将配置的没个属性映射到person类属性中。
使用@Value注入属性
@Value注解式Spring框架提供的,用来读取配置文件中的属性值,并逐个注入Bean对象的对应属性中,Spring Boot框架对Spring框架中的@Value注解进行了默认继承,在Spring Boot中还可以使用该注解读取和注入配置文件的属性值。 示例代码如下:
@Component
public class person{
@Value("${person.id}")
private int id;
}
示例: (1)在com.itheima.properties下创建一个新实体类Student
public class Student {
@Value("${person.id}")
private int id;
@Value("${person.name}")
private String name;
private List hobby;
private String[] family;
private Map map;
private Pet pet;
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", hobby=" + hobby +
", family=" + Arrays.toString(family) +
", map=" + map +
", pet=" + pet +
'}';
}
}
(2)在PeizhiApplicationTests测试类中添加测试方法studentTest
@Autowired
private Student student;
@Test
public void studentTest(){
System.out.println(student);
}
运行测试方法 
两种注解对比分析
对比点 | @ConfigurationProperties | @Value |
---|
底层框架 | Spring Boot | Spring | 功能 | 批量注入配置文件中的属性 | 单个注入 | setter方法 | 需要 | 不需要 | 复杂类型属性注入 | 支持 | 不支持 | 松散绑定 | 支持 | 不支持 | JSR303数据校验 | 支持 | 不支持 | SpEL | 不支持 | 支持 |
具体说明 (1)底层框架: @ConfigurationProperties注解式Spring Boot框架自带的,而@Value注解式Spring框架支持的,SpringBoot框架对Spring进行了默认支持 (2)功能: @ConfigurationProperties能够将配置文件中的属性批量注入Bean对象,而@Value只能单个注入 (3)属性setter方法 使用@ConfigurationProperties注解进行配置文件属性值读取注入时,还必须为每一个属性设置setter方法,通过对应的注解才能够将配置文件中的属性一一匹配注入对应的Bean属性上。@Value完全不需要为属性设置setter方法,该注解会先通过表达式读取配置文件中属性值,然后自动注入下方法的Bean属性上。 (4)复杂类型属性注入 @ConfigurationProperties和@Value都能注入配置文件中的属性,@ConfigurationProperties支持复杂类型属性注入,而@Value不支持。 (5)松散绑定 松散绑定:比如我的yaml中写的last-name,这个和代码中的lastName是一样的, - 后面跟着的字母默认是大写的,也就是-和我们的小写驼峰匹配。松散绑定的语法代码:
person.firstName=james //标准写法,对应Person类属性名
person.first-Name=james //使用横线“-”分隔多个单词
person.first_Name=james //使用下划线进行分隔
PERSON.FIRSTNAME=james //使用大小写格式,
(6)JSR303数据校验 @ConfigurationProperties注解进行配置文件属性值注入时,支持JSR303数据校验,主要作用是校验配置文件中注入对应的Bean属性是否符合相关值的规则。 (7)SQEL表达式 @Value注解注入时配置文件属性时,支持SQEL表达式,即“#{xx}” 示例代码:
@Value{“#5*2”}
private int id;
上述代码在不使用配置文件的情况下,直接使用@Value注解支持的SQE了表达式注入Bean属性而@ConfigurationProperties支持。 综上所述 当只是针对某一个业务需求,要引入配置文件中的个别属性值,推荐使用@Value。 当针对某个JavaBean类,需要大量注入属性值,推荐使用@ConfigurationProperties注解。
Spring Boot自定义配置
使用@PropertySource加载配置文件
加载自定义配置文件可以使用@Property和@Configuration注解实现@PropertySource注解可以指定自定义配置文件的位置和名称,@Configuration注解可以将实体类指定为自定义配置类,如果需要将自定义配置文件中的属性值注入实体类属性,可以使用@ConfigurationProperties或@Value注入属性值。 示例: (1)打开Spring Boot在peizhi项目下创建一个test.properties配置文件
test.id=10
test.name=test
(2)在com.itheima.properties包自定义一个配置类MyProperties
package com.itheima.properties;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySource("classpath:test.properties")
@EnableConfigurationProperties(MyProperties.class)
@ConfigurationProperties(prefix = "test")
public class MyProperties {
private int id;
private String name;
public void setId(int id) {
this.id = id;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "MyProperties{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
其中MyProperties是一个自定义配置类,其中有4个注解 1.@Configuration:该注解用于表示当前类是一个自定义配置类,该类会作为Bean组件添加到Spring容器中,这里等同于@Controller注解 2.@PropertySource(“classpath:test.properties”)注解制定了自定义配置文件的位置和名称,此示例表示自定义配置文件为classpath类路径下的test.properties文件 3.@ConfigurationProperties(prefix = “test”)该注解将上述自定义配置文件test.properties中以test开头的属性值注入该配置类属性中 4.@EnableConfigurationProperties(MyProperties.class)注解表示开启对应配置类MyProperties的属性注入功能,该注解式配合@ConfigurationProperties使用的,如果自定义配置类使用了@Component注解而非@Configuration这届,那么@EnableConfigurationProperties(MyProperties.class)可以省略 (3)打开测试类在测试类中添加测试方法
@Autowired
private MyProperties myProperties;
@Test
public void myPropertiestTest(){
System.out.println(myProperties);
}
(4)运行测试方法 
使用@importResource加载XML配置文件
@importResource注解标注在一个配置类上,通常放置在应用启动类上,使用时需要指定XML配置文件的路径和名称。 示例 (1)在peizhi中新建一个com.itheima.config的包,并在该包下创建一个类MyServlet该类中不需要任何编码 (2)打开resources目录,在该目录下创建一个名为beans.xml的自定义配置文件,在该配置文件中将MyService配置为Bean
<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myService" class="com.itheima.config.MyService"/>
</beans>
(3)在启动类上添加@importResource注解来指定XML文件位置
package com.itheima;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ImportResource;
@ImportResource("classpath:beans.xml")
@SpringBootApplication
public class PeizhiApplication {
public static void main(String[] args) {
SpringApplication.run(PeizhiApplication.class, args);
}
}
(3)打开测试类添加测试方法applicationContextTest
@Autowired
private ApplicationContext applicationContext;
@Test
public void applicationContextTest(){
System.out.println(applicationContext.containsBean("myService"));
}
运行代码查看输出结果 
使用@Configuration编写自定义配置类
使用@Configuration注解可以指定配置类,它的作用和XML配置是一样的,配置类中的@Bean注解方法返回的对象都将作为Bean注入Spring容器,默认情况下,使用@Bean注解的方法名就是组件名 示例: (1)在com.itheima.config包下创建一个类MyConfig,并使用@Configuration注解将该声明一个配置类
package com.itheima.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfig {
@Bean
public MyService myService(){
return new MyService();
}
}
(2)注释掉之前在PeizhiApplication中的@ImportResource(“classpath:beans.xml”) (3)运行测试方法applicationContextTest  说明使用自定义配置类的方式同样可以向Spring容器中添加和配置组件。
Profile多环境配置
实际开发中,程序通常需要部署到不同的运行环境中,不同的环境可能需要不同的环境配置,Spring Boot提供了多环境配置方式。分别是Profile文件多环境配置和@Profile注解多环境配置
使用Profile文件进行多环境配置
在Spring Boot框架中,使用Profile进行多环境配置是,该配置文件名必须满足application-{profile}.properties的格式,其中{profile}对应具体的环境标识,示例:
application-dev.properties //开发环境配置
application-test.properties //测试环境配置文件
application-prod.properties //生产环境配置文件
如果想要使用上述对应环境,只需要在SpringBoot的全局配置文件中激活spring.Profiles.active属性激活配置。以激活dev开发环境配置文件为例,在全局变量中application.properties中配置激活环境的属性,示例代码如下:
spring.profiles.active=dev
示例: (1)在resource目录下创建application-dev.properties,application-test.properties,application-prod.properties 分别在各个配置文件中配置不同的设置 application-dev.properties
server.port=8081
application-test.properties
server.port=8082
application-prod.properties
server.port=8083
(2)打开全局配置文件application.properties,在该配置文件中配置spring.properties.active属性选择激活Profile文件设置
spring.profiles.active=dev
(3)启动启动类PeizhiApplication 
使用@Profile注解进行多环境配置
除了使用Profile文件进行环境配置外还可以使用@Profile注解进行多环境配置。@Profile注解作用于类。并通过value属性指定配置环境。使用@Profile注解配置的环境同样需要在全局配置文件中激活 示例: (1)在com.itheima.config下创建一个接口DBConnector
package com.itheima.config;
public interface DBConnector {
public void configure();
}
(2)在com.itheima.config包下创建三个实现DBConnector几口的类,重写configure()方法,模拟连接配置不同的数据库的环境 DevDBConnector
package com.itheima.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
@Configuration
@Profile("dev")
public class DevDBConnector implements DBConnector{
@Override
public void configure() {
System.out.println("数据库配置环境dev");
}
}
ProdDBConnector
package com.itheima.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
@Configuration
@Profile("prod")
public class ProdDBConnector implements DBConnector{
@Override
public void configure() {
System.out.println("数据库配置环境prod");
}
}
TestDBConnector
package com.itheima.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
@Configuration
@Profile("test")
public class TestDBConnector implements DBConnector{
@Override
public void configure() {
System.out.println("数据库配置环境test");
}
}
(3)在全局变量中application.properties中设置spring。profile。active属性激活使用Profile注解构建多环境配置 (4)在com.itheima.config包下创建一个表示数据库连接配置的DBController类进行测试
package com.itheima.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DBController {
@Autowired
private DBConnector dbcConnector;
@GetMapping("/showDB")
public void shouDB(){
dbcConnector.configure();
}
}
(5)启动PeizhiApplication,访问http://localhost:8081/showDB  
随机值设置以及参数间引用
随机值设置
在SpringBoot配置文件中,随机值使用到了SpringBoot内嵌的RandomValuePropertySource类,对一些隐秘属性值户这测试用例属性值进行随机值注入。 随机值设置的语法格式为${random.xx},xx表时需要指定生成的随机数类型和范围,通用唯一标识码(UUID)或字符串,示例代码如下
my.string=${random.value} //配置随机字符串
my.number=${random.int} //配置随机的整数
my.bignumber=${random.long} //配置随机long类型数
my.uuid=${random.uuid} //配置随机UUID类型数
my.number.less.than.ten=${random.int(10)} //配置小于10的随机整数
my.number.in.range=${1024,65536} //配置范围在[1024,6536]之间的整数
参数间引用
在Spring Boot配置文件中,配置文件的属性值和可以进行参数间的引用定义域可以被引用,并且配置文件可以解析引用的属性值。 参数间引用的语法格式为${xx},xx表示先前在配置文件中已经配置过得属性名。示例
app.name=MyApp
app.decription=${app.name} is a Spring Boot application
在上述参数间引用设置示例中,先设置了“app.name=MyApp”将app.name属性设置为了MyApp接着,在app.description属性配置中,使用了${app.name}进行引用 示例: (1)在全局配置文件中分别通过随机值和参数间引用的方式添加两个测试属性,具体如下
tom.age=${random.int[10,20]}
tom.description= Tom的年龄可能是${tom.age}
(2)在测试类定义description属性并使用@Value注解注入tom.description属性,定义一个测试方法
@Value("${tom.description}")
private String description;
@Test
public void placeholderTest(){
System.out.println(description);
}
注直接启动会产生中文乱码 点击左上角的File下的setting 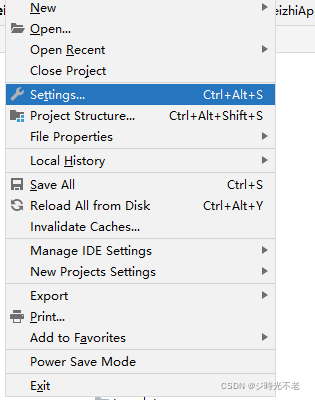 找到Editor下的File Encodings,配置如下 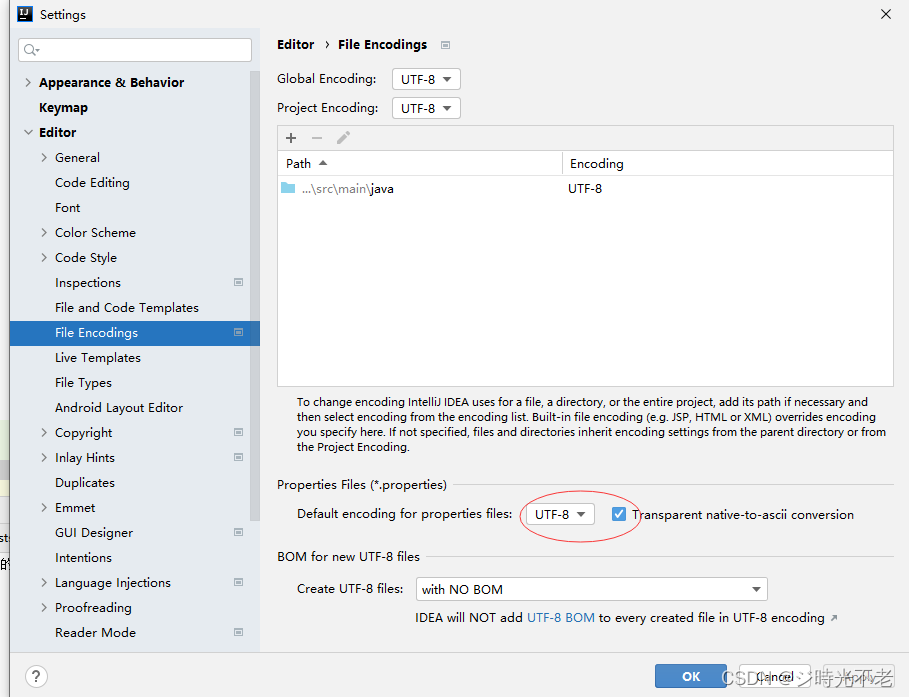 运行测试方法 
|