1. 策略模式代替if else if
2.优点:
1.策略模式符合开闭原则
2.避免使用多重条件转义语句 比如: if else if switch 语句
3. 使用策略模式可以提高算法的保密性和安全性
3. 缺点
1.客户端需要知道所有的策略 并且知道使用那一个策略
2.策略过多会增加 维护难度。
4. 类图
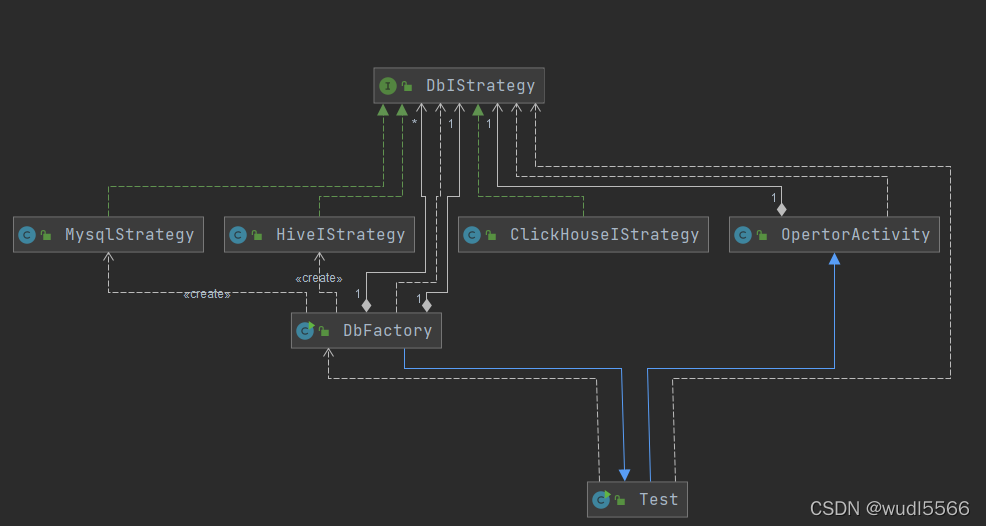
5.实现代码:
5.1 DbIStrategy
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:29
* @description:
* @modified By:
* @version: 1.0
*/
public interface DbIStrategy {
/**
* 初始化数据库
*/
void initDb();
}
MysqlStrategy
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:23
* @description: MYSQL 的策略实现
* @modified By:
* @version: 1.0
*/
public class MysqlStrategy implements DbIStrategy {
public void initDb() {
System.out.println("mysql 的策略类");
}
}
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:42
* @description:
* @modified By:
* @version: 1.0
*/
public class ClickHouseIStrategy implements DbIStrategy{
public void initDb() {
System.out.println("Click House 的实现策略");
}
}
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:24
* @description:
* @modified By:
* @version: 1.0
*/
public class HiveIStrategy implements DbIStrategy {
public void initDb() {
System.out.println("hive 的策略实现----------------------->");
}
}
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:31
* @description:
* @modified By:
* @version: 1.0
*/
public class OpertorActivity {
private DbIStrategy iStrategy;
public OpertorActivity(DbIStrategy iStrategy) {
this.iStrategy = iStrategy;
}
public void execute() {
iStrategy.initDb();
}
}
package com.wudl.design.trategy.upgrade;
import java.util.HashMap;
import java.util.Map;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:32
* @description:
* @modified By:
* @version: 1.0
*/
public class DbFactory extends Test {
private static Map<String, DbIStrategy> map = new HashMap<String, DbIStrategy>();
private static final DbIStrategy EMPTY = new MysqlStrategy();
static {
map.put("mysql", new MysqlStrategy());
map.put("hive",new HiveIStrategy());
map.put("clickhouse",new HiveIStrategy());
}
public static DbIStrategy getPromotionStrategy(String promotionKey){
DbIStrategy strategy = map.get(promotionKey);
return strategy == null ? EMPTY : strategy;
}
}
package com.wudl.design.trategy.upgrade;
/**
* @author :wudl
* @date :Created in 2022-04-13 0:41
* @description:
* @modified By:
* @version: 1.0
*/
public class Test {
public static void main(String[] args) {
String str = "Hvie";
DbIStrategy promotionStrategy = DbFactory.getPromotionStrategy(str);
promotionStrategy.initDb();
}
}
|