1.导入jar包
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
2.用户认证
1.通过配置类文件
spring.security.user.name=wanzghen
spring.security.user.password=wangzhen
?2.通过配置类
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//对密码进行加密
BCryptPasswordEncoder passwordEncoder=new BCryptPasswordEncoder();
String password=passwordEncoder.encode("123");
auth.inMemoryAuthentication().withUser("lucy").password(password).roles("");
}
//因为我们对密码进行了加密,所以登录的时候需要用到BCryptPasswordEncoder这个对象 我们需要手动创建
@Bean
PasswordEncoder password(){
return new BCryptPasswordEncoder();
}
}
3.自定义实现类
? 1.配置类
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
//自己定义的类
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//通过自己定义配置类
auth.userDetailsService(userDetailsService).passwordEncoder(password());
}
//因为我们对密码进行了加密,所以登录的时候需要用到BCryptPasswordEncoder这个对象 我们需要手动创建
@Bean
PasswordEncoder password(){
return new BCryptPasswordEncoder();
}
}
2.service类
@Service
public class MyUserDetailsSerice implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
//三个参数 用户名 密码 操作的权限(集合 并且不能为空)
// role为角色的名称
List<GrantedAuthority> auth= AuthorityUtils.commaSeparatedStringToAuthorityList("role");
return new User("lucy",new BCryptPasswordEncoder().encode("123"),auth);
}
}
4.查询数据库完成认证
? ?1.引入依赖
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
? 2.创建数据库表
? 3.创建实体类
? 4.创建mapper? 继承mp
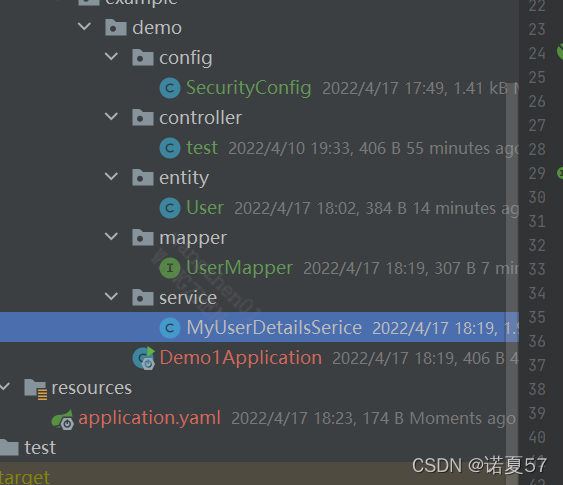
?配置类? ?调用我们自己写的service
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
//自己定义的类
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//通过自己定义配置类
auth.userDetailsService(userDetailsService).passwordEncoder(password());
}
//因为我们对密码进行了加密,所以登录的时候需要用到BCryptPasswordEncoder这个对象 我们需要手动创建
@Bean
PasswordEncoder password(){
return new BCryptPasswordEncoder();
}
}
service
@Service
public class MyUserDetailsSerice implements UserDetailsService {
@Resource
private UserMapper userMapper;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
//查询数据库
QueryWrapper<User> wrapper=new QueryWrapper<>();
wrapper.eq("username",username);
//判断是否为空
User users=userMapper.selectOne(wrapper);
if(users==null){
throw new UsernameNotFoundException("用户不存在");
}
//三个参数 用户名 密码 操作的权限(集合 并且不能为空)
// role为角色的名称
List<GrantedAuthority> auth= AuthorityUtils.commaSeparatedStringToAuthorityList("role");
//三个参数 用户名 密码 操作的权限(集合 并且不能为空)
return new org.springframework.security.core.userdetails.User(users.getUsername(),new BCryptPasswordEncoder().encode(users.getPassword()),auth);
}
}
3.自定义用户登录界面
?1.在配置类中配置
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
//自己定义的类
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//通过自己定义配置类
auth.userDetailsService(userDetailsService).passwordEncoder(password());
}
//因为我们对密码进行了加密,所以登录的时候需要用到BCryptPasswordEncoder这个对象 我们需要手动创建
@Bean
PasswordEncoder password(){
return new BCryptPasswordEncoder();
}
//----------------------------------------------------------------------------------
@Override
protected void configure(HttpSecurity http) throws Exception {
http.formLogin()
.loginPage("/Login.html") //登录页面设置
.loginProcessingUrl("/user/login") //登录访问路径
.defaultSuccessUrl("/test/index").permitAll() //登录成功跳转路径
.and().authorizeRequests()
.antMatchers("/","/test/hello","/user/login").permitAll().anyRequest().authenticated() //设置哪些路径可以直接访问不需要认证
.and().csrf().disable();//关闭csrf防护
}
}
2.添加登录界面Login.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/user/login" method="post">
用户名:<input type="text" name="username">
<br/>
密码:<input type="text" name="password">
<br/>
<input type="submit" value="login">
</form>
</body>
</html>
4.基于角色或者权限进行访问控制
?1.修改配置类
@Override
protected void configure(HttpSecurity http) throws Exception {
http.formLogin()
.loginPage("/Login.html") //登录页面设置
.loginProcessingUrl("/user/login") //登录访问路径
.defaultSuccessUrl("/test/index").permitAll() //登录成功跳转路径
.and().authorizeRequests()
.antMatchers("/","/test/hello","/user/login").permitAll() //设置哪些路径可以直接访问不需要认证
//当前登录的用户具有amdins的权限,才能访问/test/index 的路径
.antMatchers("/test/index").hasAuthority("admins")
.anyRequest().authenticated()
.and().csrf().disable();//关闭csrf防护
}

2.在MyUserDetailsSerice设置用户的权限

3.单个权限和多个权限的区别
hasAnyAuthority("admins","manager")? ?多个权限? ?admins? 和? manager 都可以访问
5.基于角色访问控制
hasRole()? ?和hasAnyRole()?


?5.自定义403? 没有权限的页面
配置类添加? ? http.exceptionHandling().accessDeniedPage("/Unauth.html");
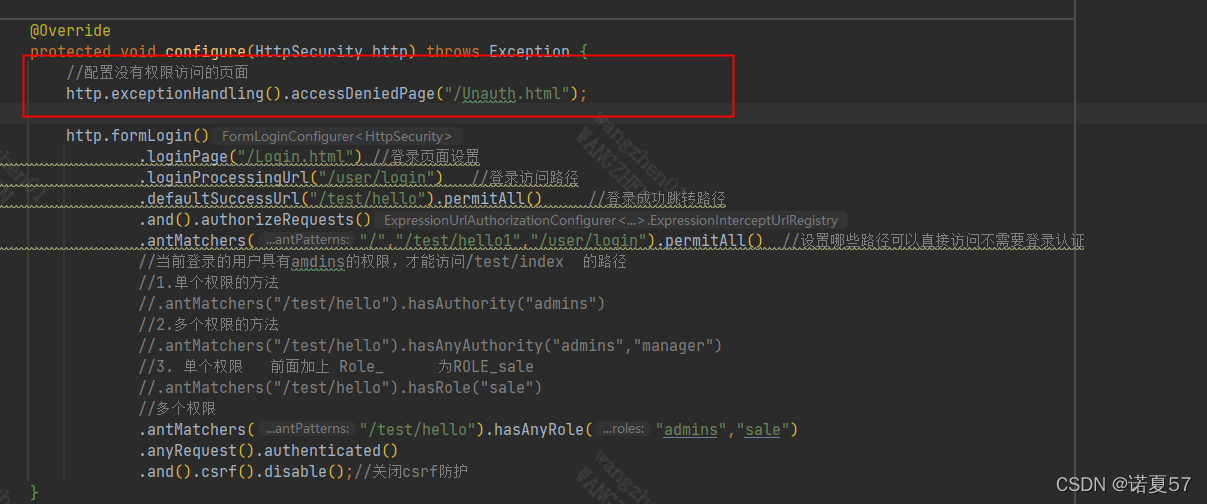
6.注解的使用?
|