demo:账户转账
1、引入依赖
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.9.8.RC3</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.8.RC3</version>
</dependency>
2、配置spring.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<context:property-placeholder location="classpath:db.properties"/>
<context:component-scan base-package="service"/>
<bean id="transactionManager"
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="*"/>
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="pc"
expression="execution(* *.*.*(..))" />
<aop:advisor advice-ref="txAdvice" pointcut-ref="pc" />
</aop:config>
</beans>
3、数据层
AccountMapper.java
package mapper;
import org.apache.ibatis.annotations.Param;
public interface AccountMapper {
public int transAccount(@Param("userid") int userid, @Param("amount")float amount);
}
AccountMapper.xml
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.AccountMapper">
<update id="transAccount" >
update account
set balance=balance + #{amount}
where userid=#{userid}
</update>
</mapper>
4、服务层
AccountService.java
package service;
import org.apache.ibatis.annotations.Param;
public interface AccountService {
public void transfer(int inId,int outId,float amount);
}
AccountServiceImpl.java
package service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import dao.AccountDao;
@Service
public class AccountServiceImpl implements AccountService {
@Autowired
private AccountDao acc;
public void transfer(int inId, int outId, float amount) {
acc.transfer(inId, amount);
int a = 1 / 0;
acc.transfer(outId, -amount);
}
}
5、测试类
package lx56;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import service.AccountService;
public class MyTest {
@Test
public void test0() {
ApplicationContext ctx = new ClassPathXmlApplicationContext("spring.xml");
AccountService as = (AccountService) ctx.getBean("accountServiceImpl");
as.transfer(1, 2, 10);
}
}
6、测试结果
出现异常,由于添加了事务管理器,事务发生回滚,数据表中数据并没有发生改变。 
基于注解形式
<tx:annotation-driven transaction-manager="transactionManager"/>
在需要使用事务管理的方法前加上@Transactional 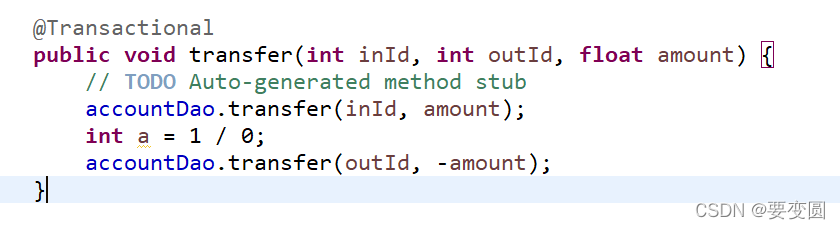
|