public class Multithread {
private int QUEEN_NUM = 0;
private int[][] Checkerboard;
private Long COUNT = 0L;
public Multithread(int QUEEN_NUM) {
this.QUEEN_NUM = QUEEN_NUM;
this.Checkerboard = new int[QUEEN_NUM][QUEEN_NUM];
this.COUNT = 0L;
}
public boolean check(int row, int col) {
for (int i = row - 1; i >= 0; i--) {
if (Checkerboard[i][col] == 1)
return false;
}
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0; i--, j--) {
if (Checkerboard[i][j] == 1)
return false;
}
for (int i = row - 1, j = col + 1; i >= 0 && j < QUEEN_NUM; i--, j++) {
if (Checkerboard[i][j] == 1)
return false;
}
return true;
}
public Long calculate(int row,int i){
Checkerboard[row][i] = 1;
if (row == QUEEN_NUM - 1) {
COUNT++;
} else {
calculate(row + 1);
}
Checkerboard[row][i] = 0;
return COUNT;
}
public Long calculate(int row) {
for (int i = 0; i < QUEEN_NUM; i++) {
if (check(row, i)) {
Checkerboard[row][i] = 1;
if (row == QUEEN_NUM - 1) {
COUNT++;
} else {
calculate(row + 1);
}
Checkerboard[row][i] = 0;
}
}
// System.out.println("子线程结果:"+COUNT);
return COUNT;
}
}
import org.omg.PortableInterceptor.SYSTEM_EXCEPTION;
import java.util.ArrayList;
import java.util.concurrent.*;
public class Main {
public static int row = 16;
public static void main(String[] args) throws ExecutionException, InterruptedException {
ArrayList<Future<Long> > tasks = new ArrayList<>();
ExecutorService exec = Executors.newCachedThreadPool();
System.out.println("---------------------------多线程版本---------------------------");
long start = System.currentTimeMillis();
System.out.println("开始时间:"+start);
Long sum = 0L;
for(int i = 0;i<row;i++){
Calculate task = new Calculate(row,i);
Future<Long> future = exec.submit(task);
tasks.add(future);
// Calculate calculate = new Calculate(row,i);
// FutureTask<Long> task1 = new FutureTask<Long>(calculate);
// tasks.add(task1);
// new Thread(task1).start();
}
System.out.println("队列长度:"+tasks.size());
for(int i = 0;i<tasks.size();i++){
Future<Long> temp = tasks.get(i);
while(temp != null && !temp.isDone()){
// System.out.println("子线程"+i+"继续监听");
TimeUnit.MILLISECONDS.sleep(200);
}
System.out.println("子线程"+i+"已经运行结束");
sum+=temp.get();
}
long now = System.currentTimeMillis();
System.out.println("结束时间:"+now);
System.out.println("使用时间: "+(now-start)+" ms");
System.out.println("结果是:" + sum);
System.out.println();
exec.shutdown();
System.out.println("---------------------------非多线程版本---------------------------");
start = System.currentTimeMillis();
System.out.println("开始时间:"+start);
Multithread multithread = new Multithread(row);
sum = multithread.calculate(0);
now = System.currentTimeMillis();
System.out.println("结束时间:"+now);
System.out.println("使用时间: "+(now-start)+" ms");
System.out.println("结果是:" + sum);
System.out.println();
}
}
class Calculate implements Callable<Long>{
private int i = 0;
private int row = 0;
public Calculate(int row,int i) {
this.row = row;
this.i = i;
}
@Override
public Long call() throws Exception{
Multithread temp = new Multithread(this.row);
return temp.calculate(0,this.i);
}
}
执行结果:
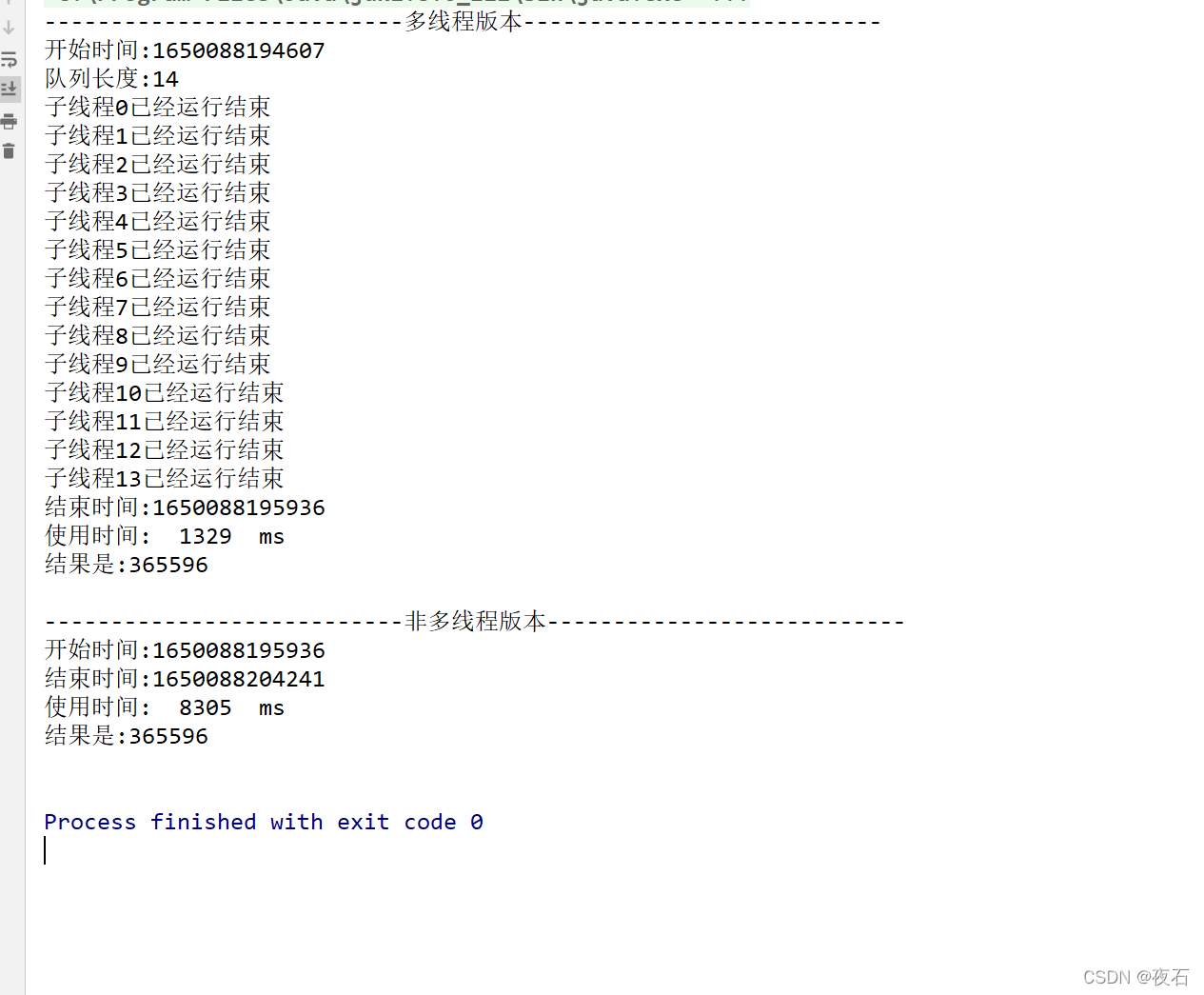
?
|