版权
版权所有,甲骨文和/或其附属公司版权所有。 甲骨文专有/机密。使用受许可条款约束。
包和依赖
package java.lang;
import java.lang.annotation.Native;
The Integer class wraps a value of the primitive type int in an object. An object of type Integer contains a single field whose type is int.
In addition, this class provides several methods for converting an int to a String and a String to an int, as well as other constants and methods useful when dealing with an int.
Implementation note: The implementations of the "bit twiddling" methods (such as highestOneBit and numberOfTrailingZeros) are based on material from Henry S. Warren, Jr.'s Hacker's Delight, (Addison Wesley, 2002).
Since:
JDK1.0
Author:
Lee Boynton, Arthur van Hoff, Josh Bloch, Joseph D. Darcy
Integer是在java.lang包下的 导入了Native依赖 Integer类将基本类型int的值包装在对象中。整型对象包含一个整型字段。 此外,该类还提供了几种将int转换为字符串和字符串转换为int的方法,以及处理int时有用的其他常量和方法。 实施说明:“比特旋转”方法(如highestOneBit和numberOfTrailingZeros)的实施基于小亨利·S·沃伦(Henry S.Warren,Jr.)的《黑客之乐》(Addison Wesley,2002)中的材料。 自: JDK1.0 作者: 李·博因顿、阿瑟·范霍夫、乔希·布洛赫、约瑟夫·D·达西
Native
再来看下导入的Native,它实际上是个接口
package java.lang.annotation;
@Documented
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.SOURCE)
public @interface Native {
}
指示可以引用定义常量值的字段 来自本机代码。 生成本机注释的工具可以将注释用作提示 头文件,以确定是否需要头文件,以及 如果是,它应该包含哪些声明。 @从1.8开始
注释
他有三个元注解
@Documented
@Documented: 将注解中的元素包含到Javadoc中
@Target(ElementType.FIELD)
@Target(ElementType.XXX):说明修饰的成员关系
constructor
描述构造器
Field
描述域
Local_variable
描述局部变量
method
描述方法
package
描述包
type
描述类、接口
@Retention(RetentionPolicy.SOURCE)
@Retention(RetentionPolicy.XXX):定义了注解被保留的时间长短
source
只在源码阶段保留,编译时被忽视
class
只被保留到编译进行时,不进JVM
Runtime
保留到程序进行时,会进JVM
总结
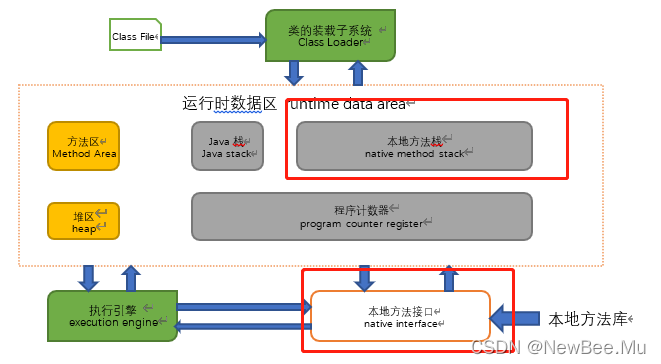 这个接口的作用就是调用本地方法库里面的用C语言写的方法
继承和实现
public final class Integer extends Number implements Comparable<Integer> {
可以看出,Integer继承了Number类,实现了Comparable接口
Number 类
package java.lang;
public abstract class Number implements java.io.Serializable {
public abstract int intValue();
public abstract long longValue();
public abstract float floatValue();
public abstract double doubleValue();
public byte byteValue() {
return (byte)intValue();
}
public short shortValue() {
return (short)intValue();
}
private static final long serialVersionUID = -8742448824652078965L;
}
抽象类{@code Number}是平台的超类 表示可转换为基本类型byte、double、float、int、long、short 从给定原语的特定Number实现类型由所讨论的Number实现定义。 对于平台类,转换通常类似于缩小原语转换或扩大原语转换 如《爪哇与贸易》中所定义;语言规范 用于在基元类型之间转换。因此,转换可能会发生可能会丢失有关数值整体大小的信息失去精度,甚至可能返回不同符号的结果 而不是输入。 有关详细信息,请参阅给定Number实现的文档 转换细节。 @作者李·博因顿 @作者阿瑟·范霍夫 @jls 5.1.2扩大原语转换 @jls 5.1.3缩小原语转换范围 @从JDK1.0开始
public abstract int intValue();
以整数形式返回指定数字的值,这可能涉及舍入或截断。 返回:转换为int类型后此对象表示的数值。 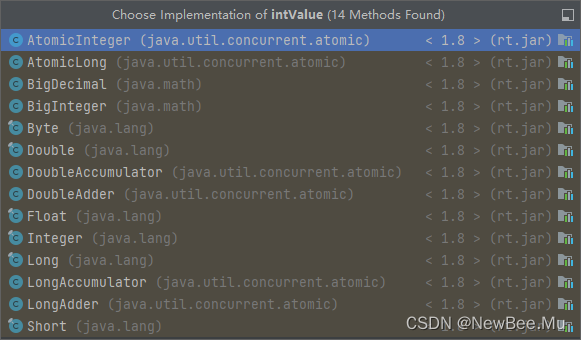
public abstract long longValue();
以long形式返回指定数字的值,这可能涉及舍入或截断。 返回:转换为long类型后此对象表示的数值。 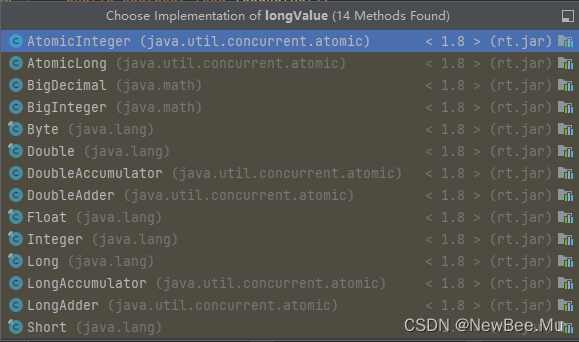
public abstract float floatValue();
以浮点形式返回指定数字的值,这可能涉及舍入。 返回:转换为float类型后,此对象表示的数值。 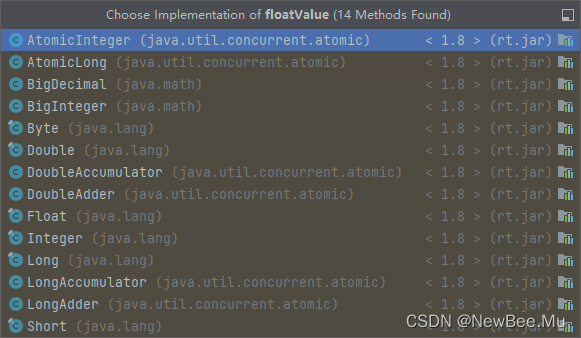
public abstract double doubleValue();
以双精度形式返回指定数字的值,这可能涉及舍入。 返回:转换为double类型后此对象表示的数值。 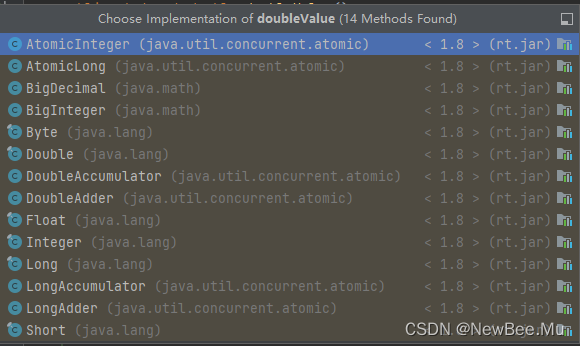
public byte byteValue()
public byte byteValue() {
return (byte)intValue();
}
形式返回指定数字的值,这可能涉及舍入或截断。 此实现将intValue转换的结果返回到一个字节。 返回:此对象在转换为字节类型后表示的数值。 自:JDK1.1. 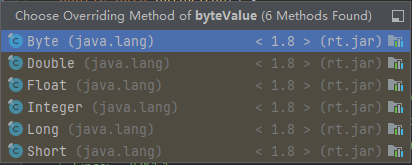
public short shortValue()
public short shortValue() {
return (short)intValue();
}
以短字符形式返回指定数字的值,这可能涉及舍入或截断。 此实现将intValue cast的结果返回给short。 返回:转换为short类型后此对象表示的数值。 自:JDK1.1.
private static final long serialVersionUID = -8742448824652078965L;
使用JDK 1.0.2中的serialVersionUID实现互操作性
|