日期时间体系:一共8个类
- 第1代,jdk1.0:? ? ? ?Date, DateFormat,SimpleDateFormat
- 第2代,jdk1.1:? ? Calendar
- 第3代,jdk8.0:? ? ? ?LocalDate,LocalTime,LocalDateTime,DateTimeFormatter
Date类 java.util.Date 表示特定的时间,精确到毫秒 过时的能用,但是让你看着别扭。
1.Date类2个构造器:
- ? ? ?public Date()
- ? ? ?public Date(long date)//long自1970年的毫秒数。
2.Date类2个方法:
- ? ? ?long getTime();//把日期对象转换为对应的一个毫秒数
- ? ? ?Date类重写了toString(),返回结果为指定格式的字符串。
1969年 ===>unix系统
丹尼斯·里奇(1941年9月9日-2011年10月12日),C语言之父,UNIX之父
public class ExceptionDemo {
public static void main(String[] args) {
Date date = new Date(0L);
//由于我们处于东八区,所以我们的基准时间为1970年1月1日8:00:00
System.out.println(date);//Thu Jan 01 08:00:00 CST 1970
}
}
DateFormat是抽象类 java.text ? ? 针对Date对象进行格式化解析操作的工具类的父类
格式化: Date对象 -》String文本? ? ? ? ? public final String format(Date date) 解析:String文本-》 Date对象? ? ? ? ? ? ???public Date parse(String ?source)
SimpleDateFormat是DateFormat实现类 java.text? 构造器: 用给定的模式和默认语言环境的日期格式符号构造 。
public class ExceptionDemo {
public static void main(String[] args) throws ParseException {
Date date = new Date();
// Date对象的格式化
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日 HH时mm分ss秒SSS毫秒");
String format = sdf.format(date);
System.out.println(format);//2022年04月22日 11时48分01秒461毫秒
// Date对象的解析
String s = "2022年02月22日 22时22分22秒222毫秒";
// parse()会有编译时异常,得处理
Date parse = sdf.parse(s);
System.out.println(parse);//Tue Feb 22 22:22:22 CST 2022
// 返回描述此日期格式的模式字符串
String pattern = sdf.toPattern();
System.out.println(pattern);//yyyy年MM月dd日 HH时mm分ss秒SSS毫秒
}
}
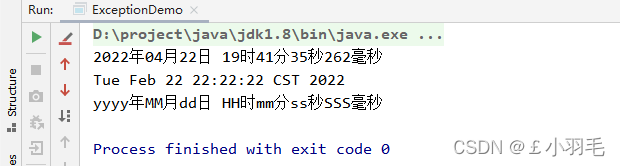
这样魔改一下也是不会发生异常的,因为sdf格式里要求的你都给提供了
不会发生异常:
String s=“2022年02月22日 22时22分22秒222毫秒”
SimpleDateFormat sdf=new SimpleDateFormat("yyyy年MM月dd日 HH时mm分ss秒");
sdf.parse(s);
Calendar 黄历类[抽象类] ?java.util.Calendar 第二代日期时间的工具类代表,居然有构造器
Calendar方法:
- ? ? public static Calendar getInstance();//获取Calendar类型对象
- ? ? int get(int field);
public class ExceptionDemo {
public static void main(String[] args) {
Calendar date = Calendar.getInstance();
int year = date.get(Calendar.YEAR);//都是静态常量
int month = date.get(Calendar.MONTH);//月份是0-11.
int day = date.get(Calendar.DAY_OF_MONTH);
System.out.println(year + "年" + (month + 1) + "月" + day + "日");//2022年4月22日
}
}
LocalDateTime ?java.time 针对第三代操作日期时间的工具类,构造器私有化
8个要掌握的方法
- LocalDateTime now();
- int getYear()
- int getMonthValue()//1月-12月
- int getDayOfMonth();//获取月当中的天
- int ? getHour()
- int ? getMinute()
- int ? getSecond()
- static LocalDateTime parse(CharSequence text,DateTimeFormatter formatter)
DateTimeFormatter ? ?java.time.format
针对第三代日期时间类进行格式化和解析,DateTimeFormatter 构造器私有化,
DateTimeFormatter的方法
- ????????static DateTimeFormatter ofPattern(String pattern)
- ????????String format(TemporalAccessor temporal))
- ????????TemporalAccessor parse(CharSequence text)
public class ExceptionDemo {
public static void main(String[] args) {
LocalDateTime ldt=LocalDateTime.now();
DateTimeFormatter dtf=DateTimeFormatter.ofPattern("yyyy年MM月dd日");
String format = dtf.format(ldt);//LocalDateTime是该参数TemporalAccessor接口的实现类
System.out.println(format);//2022年04月22日
System.out.println("==================");
String s="2202年02月02日";
LocalDate date = LocalDate.parse(s, dtf);//不会报错,因为s和dtf都只针对日期并没有时间
dtf.parse(s);//不可用
System.out.println(date);
LocalDateTime parse = LocalDateTime.parse(s, dtf);//会报错,因为字符串s中并没有时间,只有日期LocalDateTime是日期时间类
}
}
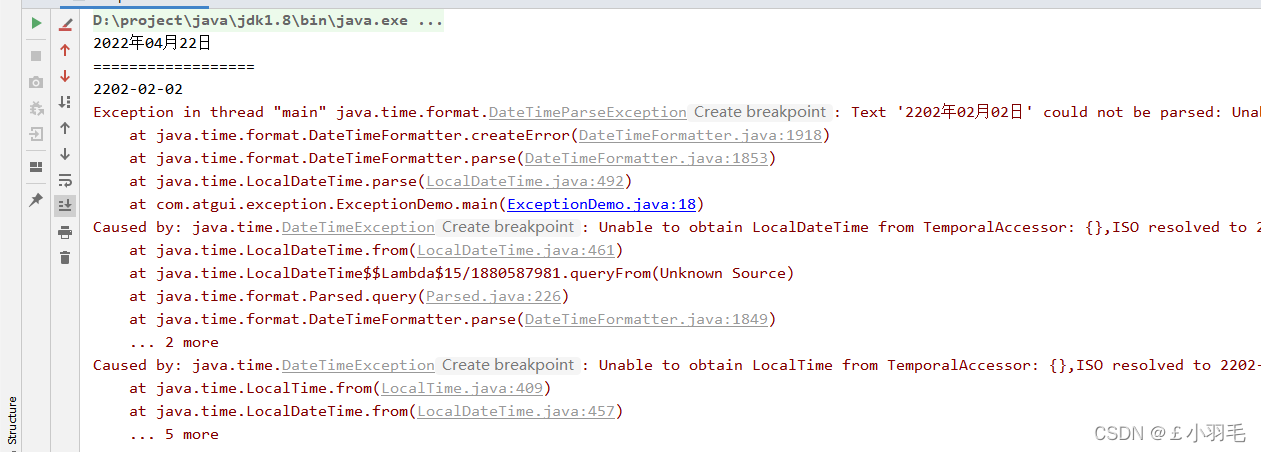
FIle类 ? ?java.io? 构造器:File(String pathname) //可以是相对路径和绝对路径
相对路径和绝对路径 绝对路径是一个完整的路径,从盘符开始。
相对路径是一个不完整的路径:是相对于项目project的可变路径。注意 File对象的构造器里写的是啥,则相对路径打印啥
- getAbsolutePath();
- getPath();//获取File对象的相对路径
- getName();//返回文件或目录的名称,就是它们的最后一级
- Long Length();//获取单纯文件的大小 ,以字节KB为单位
- isDirectory()//判断这个File对象是否是文件夹
- isFile();//判断这个File对象是否是文件
- exists();//测试File对象在硬盘是否真实存在
- File[] ?listFiles();//获取指定file对象下的所有目录和文件
----------
File对象的构造器里写的是啥,则相对路径打印啥
public class ExceptionDemo {
public static void main(String[] args) {
File file1=new File("D:\\atguigu\\PDF课件"); // 用\\起到转义作用
File file2=new File("D:\\atguigu\\PDF课件\\a.txt");
File file3=new File("a.txt");
System.out.println(file1.getPath());//D:\atguigu\PDF课件
System.out.println(file2.getPath());//D:\atguigu\PDF课件\a.txt
System.out.println(file3.getPath());//a.txt
}
}
经典用法,使用递归遍历多级文件夹
public class ExceptionDemo {
public static void main(String[] args) {
File file = new File("D:\\atguigu");// 用\\起到转义作用
print(file);
}
public static void print(File path){
//使用递归遍历多级文件夹
File[] files = path.listFiles();
for (int i = 0; i < files.length; i++) {
File file=files[i];
if(file.isFile()){
System.out.println("文件--"+file);
}else {
System.out.println("目录--"+file);
print(file);
}
}
}
}
效果如下:感谢我敬爱的沙老师给我这么多的资料包
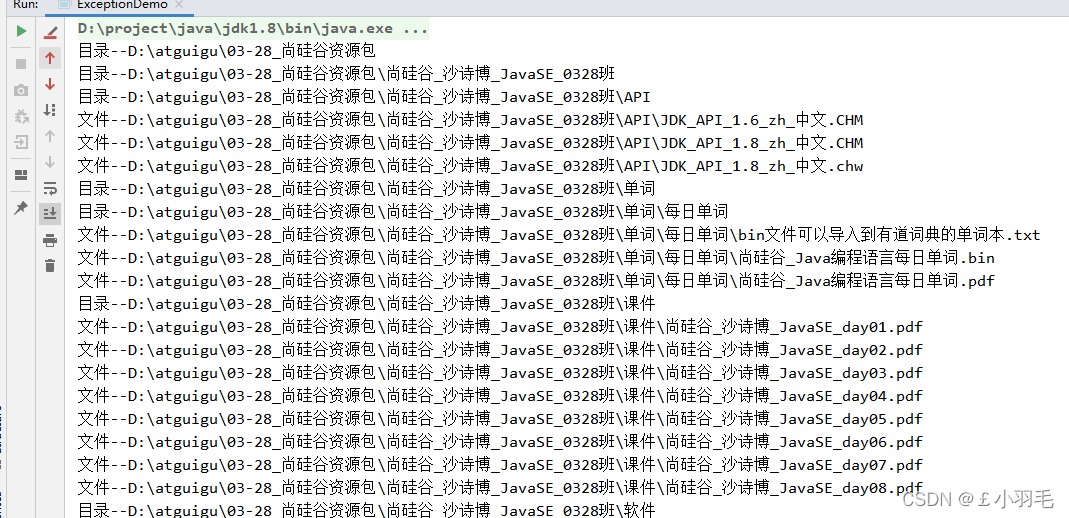
IO流:
根据数据流向进行分类:
????????输入流(其他设备->内存) ????????输出流:(内存->其他设备)
根据数据流向单位进行分类: ????????字节流:输入输出以字节为单位 ????????字符流:输入输出以字符为单位
上面俩俩组合后就是IO流的4大基类,这4个都是抽象类
字节输入流 InputStream 字节输出流 OutputStream 字符输入流 ?Reader 字符输出流 ?Writer
根据读写的内容再进行分类: 文件流(最基本的读写操作) ? ? ? ? ? ? ? ?文件字节输入流 ? ? ? ? ? ? ? ?文件字节输出流 ? ? ? ? ? ? ? ?文件字符输入流 ? ? ? ? ? ? ? ?文件字符输出流
缓冲流(高效流) ? ? ? ? ? ? ? ?缓冲字节输入流 ? ? ? ? ? ? ? ?缓冲字节输出流 ? ? ? ? ? ? ? ?缓冲字符输入流 ? ? ? ? ? ? ? ?缓冲字符输出流 转换流 ? ? ? ? ? ? ? ? 转换输入流 ? ? ? ? ? ? ? ? 转换输出流 对象流 ? ? ? ? ? ? ? ? 对象输入流 ? ? ? ? ? ? ? ? 对象输出流 标准流 ? ? ? ? ? ? ? ? 标准输入流 ? ? ? ? ? ? ? ? 标准输出流
|