认证
- session 会话
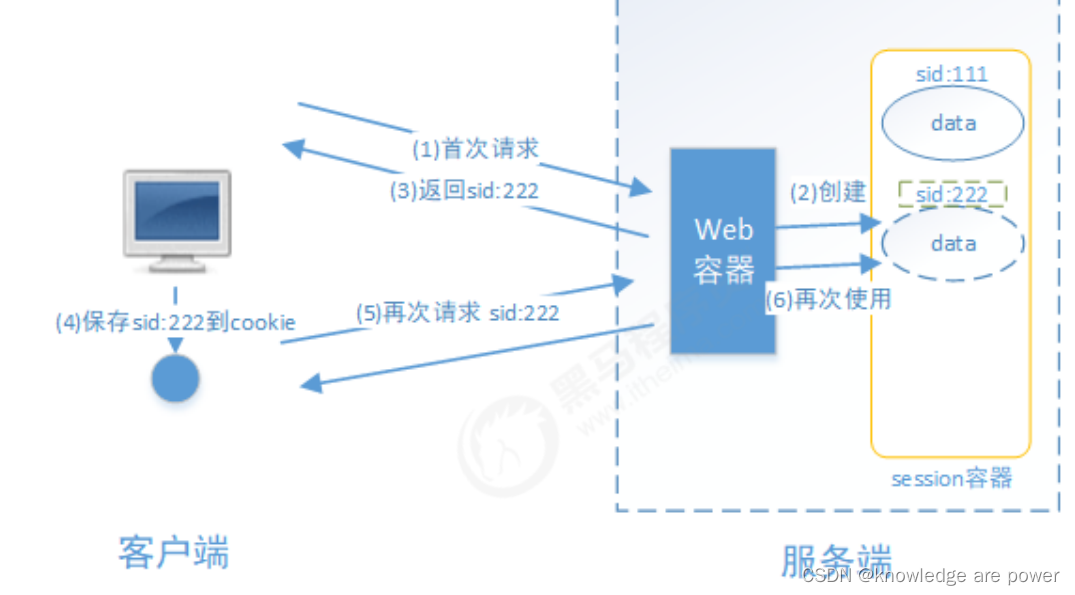
服务端需要保存会话session信息
客户端的 sesssion_id 需要存放到 cookie 中
当用户退出系统或session过期销毁时,客户端的session_id也就无效了
基于Session的认证机制由Servlet规范定制,Servlet容器已实现,
用户通过HttpSession的操作方法即可实现
方法 | 含义 |
---|
HttpSession getSession(Boolean create) | 获取当前HttpSession对象 | void setAttribute(String name,Object value) | 向session中存放对象 | object getAttribute(String name) | 从session中获取对象 | void removeAttribute(String name); | 移除session中对象 | void invalidate() | 使HttpSession失效 |
- token
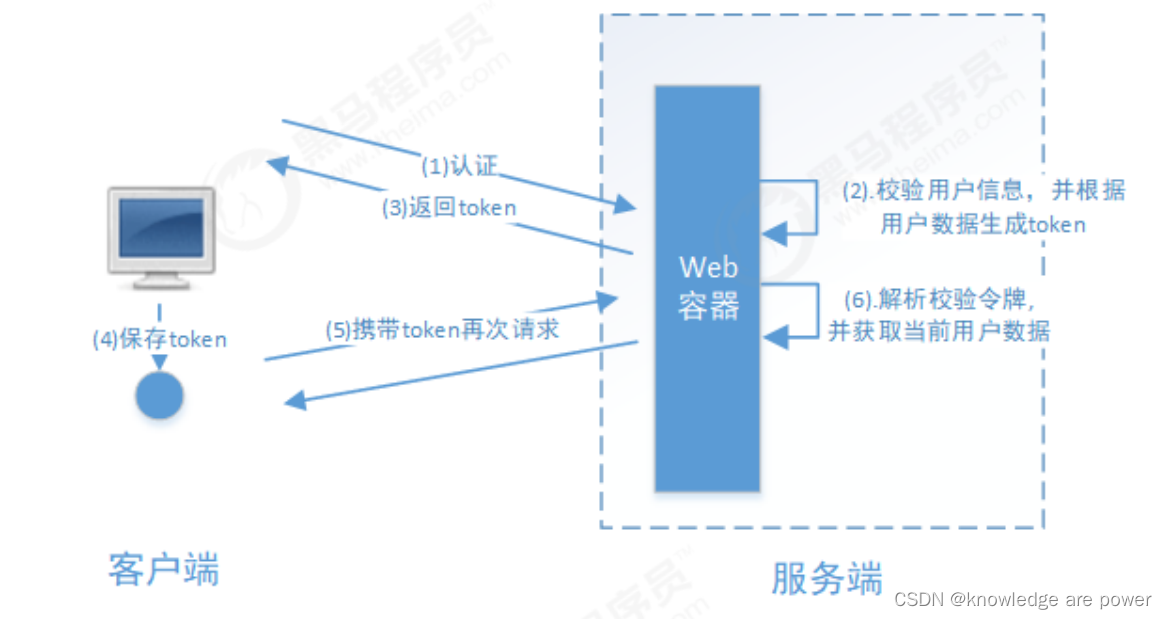
客户端可以放到 cookie 或 localStorage 等存储中
服务端不需要存储 token 信息
授权的数据模型
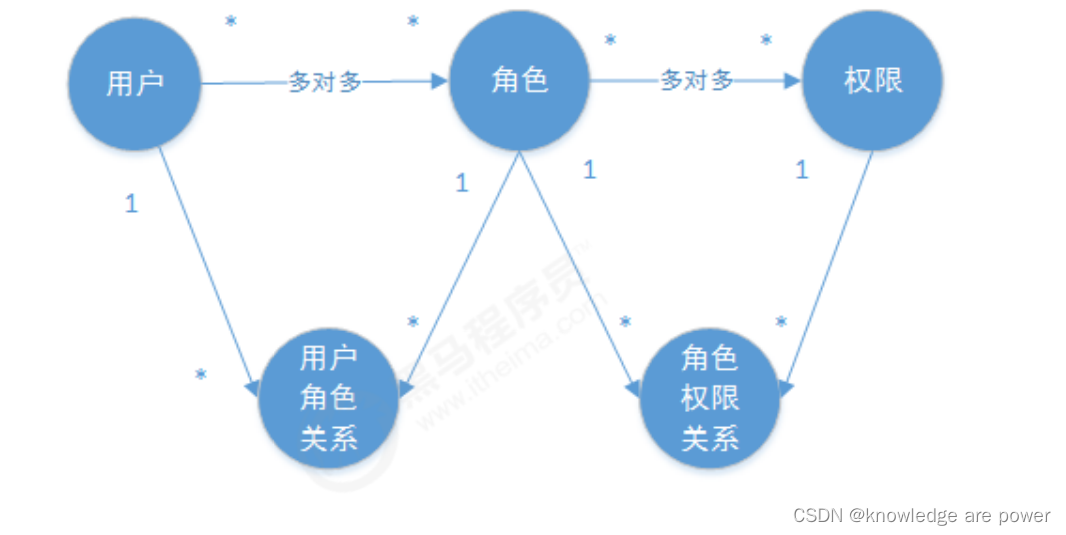
权限(权限id、权限标识、权限名称、资源名称、资源访问地址、...)
主体(用户id、账号、密码、...)
角色(角色id、角色名称、...)
主体(用户)和角色关系(用户id、角色id、...)
角色和权限关系(角色id、权限id、...)
RBAC
- Role-Based Access Control 是按角色进行授权
- Resource-Based Access Control 是按资源(或权限)进行授权(推荐)
SpringSecurity
- 提供默认的认证页面,可根据自己的需求定制自己的认证页面
- 认证流程
基于 UsernamePasswordAuthenticationFilter 实现
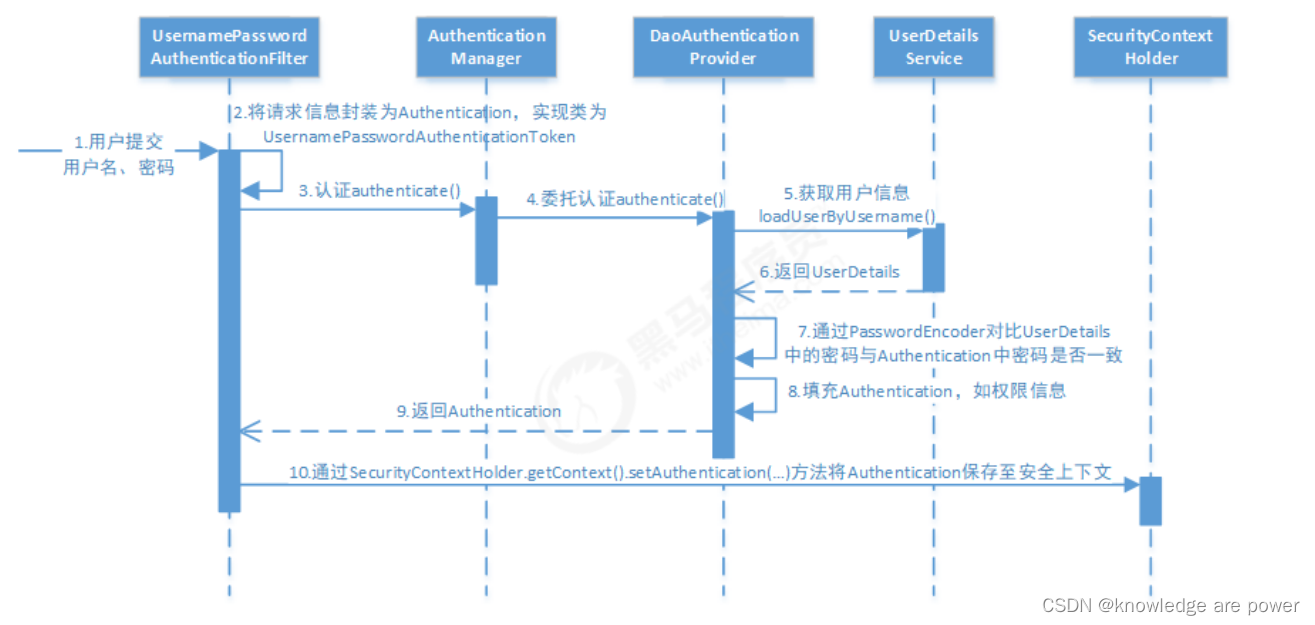
核心类
- WebSecurityConfigurerAdapter 自定义Security策略
- AuthenticationManagerBuilder 自定义Security策略
- @EnableWebSecurity 开启WebSecurity模式
核心依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
授权配置类编写
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().antMatchers("/").permitAll()
.antMatchers("/level1/**").hasRole("vip1")
.antMatchers("/level2/**").hasRole("vip2")
.antMatchers("/level3/**").hasRole("vip3");
http.formLogin();
}
}
- 无权限会跳转至登录页面进行认证,那么认证的数据来源从哪里来呢?
在上方的 SecurityConfig 中重写认证规则,主要包含 账户,密码,权限
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
我们要将前端传过来的密码进行某种方式加密
auth.inMemoryAuthentication().passwordEncoder(new BCryptPasswordEncoder())
.withUser("kuangshen").password(new BCryptPasswordEncoder().encode("123456")).roles("vip2","vip3")
.and()
.withUser("root").password(new BCryptPasswordEncoder().encode("123456")).roles("vip1","vip2","vip3")
.and()
.withUser("guest").password(new BCryptPasswordEncoder().encode("123456")).roles("vip1","vip2");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.logout();
http.logout().logoutSuccessUrl("/");
}
1:页面输入账号,密码
2:Security 根据用户名查询对应的用户信息,进行密码比对
|