1.定义接口
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Dict {
String dictCode();
String dictText() default "";
}
2.定义切面
import com.alibaba.fastjson.JSONObject;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.ruoyi.common.annotation.Dict;
import com.ruoyi.common.core.domain.AjaxResult;
import com.ruoyi.common.core.domain.entity.SysDictData;
import com.ruoyi.common.core.page.TableDataInfo;
import com.ruoyi.common.page.PageDataInfo;
import com.ruoyi.common.utils.ObjConvertUtils;
import com.ruoyi.system.service.ISysDictTypeService;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.lang.reflect.Field;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Map;
@Aspect
@Component
@Slf4j
public class DictAspect {
private static String DICT_TEXT_SUFFIX = "Text";
@Autowired
private ISysDictTypeService dictTypeService;
@Pointcut("execution( * com.ruoyi.*.controller.huandian.*.*(..)) || execution( * com.ruoyi.*.controller.*.*(..)) ")
public void dict() {
}
@Around("dict()")
public Object doAround(ProceedingJoinPoint pjp) throws Throwable {
long time1 = System.currentTimeMillis();
Object result = pjp.proceed();
long time2 = System.currentTimeMillis();
long start = System.currentTimeMillis();
this.parseDictText(result);
long end = System.currentTimeMillis();
return result;
}
private void parseDictText(Object result) {
List<JSONObject> items = new ArrayList<>();
if (result instanceof AjaxResult) {
AjaxResult rr = (AjaxResult) result;
if (rr.getData() instanceof String) {
rr.setData(rr.getData());
} else {
rr.setData(getDataModel(rr.getData()));
}
} else if (result instanceof TableDataInfo) {
TableDataInfo rr = (TableDataInfo) result;
List<?> list = (List<?>) rr.getRows();
getData(items, list);
rr.setRows(items);
} else if (result instanceof PageDataInfo) {
PageDataInfo rr = (PageDataInfo) result;
List<?> list = (List<?>) rr.getData();
getData(items, list);
rr.setData(items);
}
}
private void getData(List<JSONObject> items, List<?> listData) {
List<?> list = listData;
for (Object record : list) {
ObjectMapper mapper = new ObjectMapper();
String json = "{}";
try {
json = mapper.writeValueAsString(record);
} catch (JsonProcessingException e) {
log.error("Json解析失败:" + e);
}
JSONObject item = JSONObject.parseObject(json);
for (Field field : ObjConvertUtils.getAllFields(record)) {
if (field.getAnnotation(Dict.class) != null) {
String dictType = field.getAnnotation(Dict.class).dictCode();
String text = field.getAnnotation(Dict.class).dictText();
String key = String.valueOf(item.get(field.getName()));
String textValue = translateDictValue(dictType, key);
if (!StringUtils.isBlank(text)) {
item.put(text, textValue);
} else {
item.put(field.getName() + DICT_TEXT_SUFFIX, textValue);
}
}
if ("java.util.Date".equals(field.getType().getName())
&& field.getAnnotation(JsonFormat.class) == null
&& item.get(field.getName()) != null) {
SimpleDateFormat aDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
item.put(field.getName(), aDate.format(new Date((Long) item.get(field.getName()))));
}
}
items.add(item);
}
}
private JSONObject getDataModel(Object data) {
ObjectMapper mapper = new ObjectMapper();
String json = "{}";
try {
json = mapper.writeValueAsString(data);
} catch (JsonProcessingException e) {
log.error("Json解析失败:" + e);
}
JSONObject item = JSONObject.parseObject(json);
for (Field field : ObjConvertUtils.getAllFields(data)) {
if (field.getAnnotation(Dict.class) != null) {
String dictType = field.getAnnotation(Dict.class).dictCode();
String text = field.getAnnotation(Dict.class).dictText();
String key = String.valueOf(item.get(field.getName()));
String textValue = translateDictValue(dictType, key);
if (!StringUtils.isBlank(text)) {
item.put(text, textValue);
} else {
item.put(field.getName() + DICT_TEXT_SUFFIX, textValue);
}
}
if ("java.util.Date".equals(field.getType().getName())
&& field.getAnnotation(JsonFormat.class) == null
&& item.get(field.getName()) != null) {
SimpleDateFormat aDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
item.put(field.getName(), aDate.format(new Date((Long) item.get(field.getName()))));
}
}
return item;
}
private String translateDictValue(String dictType, String key) {
if (StringUtils.isEmpty(key)) {
return null;
}
StringBuffer textValue = new StringBuffer();
String[] keys = key.split(",");
for (String k : keys) {
if (k.trim().length() == 0) {
continue;
}
String dict_label = "";
List<SysDictData> data = dictTypeService.selectDictDataByType(dictType);
if (null == data) {
return dict_label;
}
for (SysDictData sysDictData : data) {
if (sysDictData.getDictValue().equals(key)) {
dict_label = sysDictData.getDictLabel();
}
}
textValue.append(dict_label);
}
return textValue.toString();
}
}
3.工具类
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
@SuppressWarnings("ALL")
public class ObjConvertUtils {
public static Field[] getAllFields(Object object) {
Class<?> clazz = object.getClass();
List<Field> fieldList = new ArrayList<>();
while (clazz != null) {
fieldList.addAll(new ArrayList<>(Arrays.asList(clazz.getDeclaredFields())));
clazz = clazz.getSuperclass();
}
Field[] fields = new Field[fieldList.size()];
fieldList.toArray(fields);
return fields;
}
public static boolean isEmpty(Object object) {
if (object == null) {
return (true);
}
if ("".equals(object)) {
return (true);
}
if ("null".equals(object)) {
return (true);
}
return (false);
}
}
4.返回模型
import cn.hutool.http.HttpStatus;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.experimental.Accessors;
import java.io.Serializable;
@Data
@NoArgsConstructor
@AllArgsConstructor
@Accessors(chain = true)
@ApiModel("操作消息提醒")
public class AjaxResult<T> implements Serializable {
private static final long serialVersionUID = 7364644042368216173L;
@ApiModelProperty("状态码")
private int code;
@ApiModelProperty("返回内容")
private String msg;
@ApiModelProperty("数据对象")
private T data;
public AjaxResult(int code, String msg) {
this.code = code;
this.msg = msg;
}
public static AjaxResult<Void> success() {
return AjaxResult.success("操作成功");
}
public static <T> AjaxResult<T> success(T data) {
return AjaxResult.success("操作成功", data);
}
public static AjaxResult<Void> success(String msg) {
return AjaxResult.success(msg, null);
}
public static <T> AjaxResult<T> success(String msg, T data) {
return new AjaxResult<>(HttpStatus.HTTP_OK, msg, data);
}
public static AjaxResult<Void> error() {
return AjaxResult.error("操作失败");
}
public static AjaxResult<Void> error(String msg) {
return AjaxResult.error(msg, null);
}
public static <T> AjaxResult<T> error(String msg, T data) {
return new AjaxResult<>(HttpStatus.HTTP_INTERNAL_ERROR, msg, data);
}
public static AjaxResult<Void> error(int code, String msg) {
return new AjaxResult<>(code, msg, null);
}
}
5.使用 添加注解即可
@ApiModelProperty(value = "状态 0-使用中 1-已归还")
@Dict(dictCode = "orderStatus")
private Integer status;
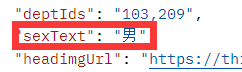 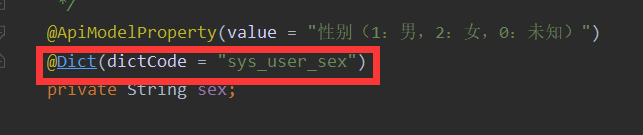
|