Spring整合MyBatis
一、项目框架
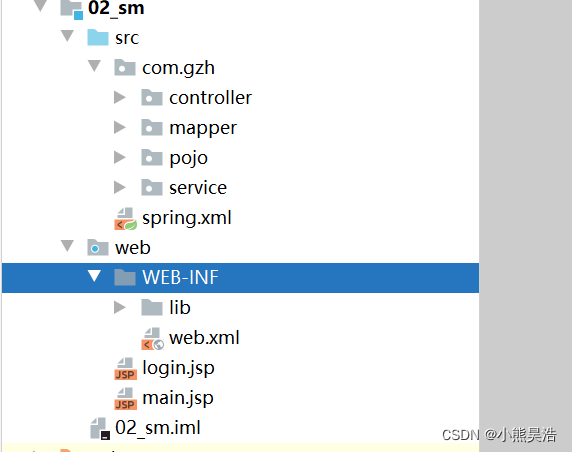](https://img-blog.csdnimg.cn/8791f4356f464e5fb0a4efe7ee37dcc5.png)
示例:这里是spring整合Mybatis之后的架构图
二、用Navicat在数据库中创建一张表t_user

三、 添加spring的一些jar包
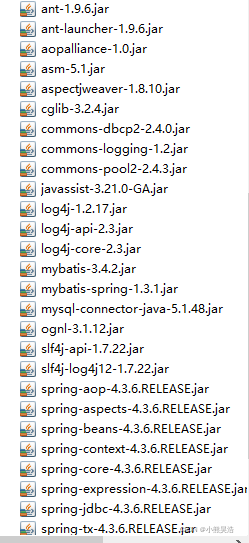
四、 编写实体类User
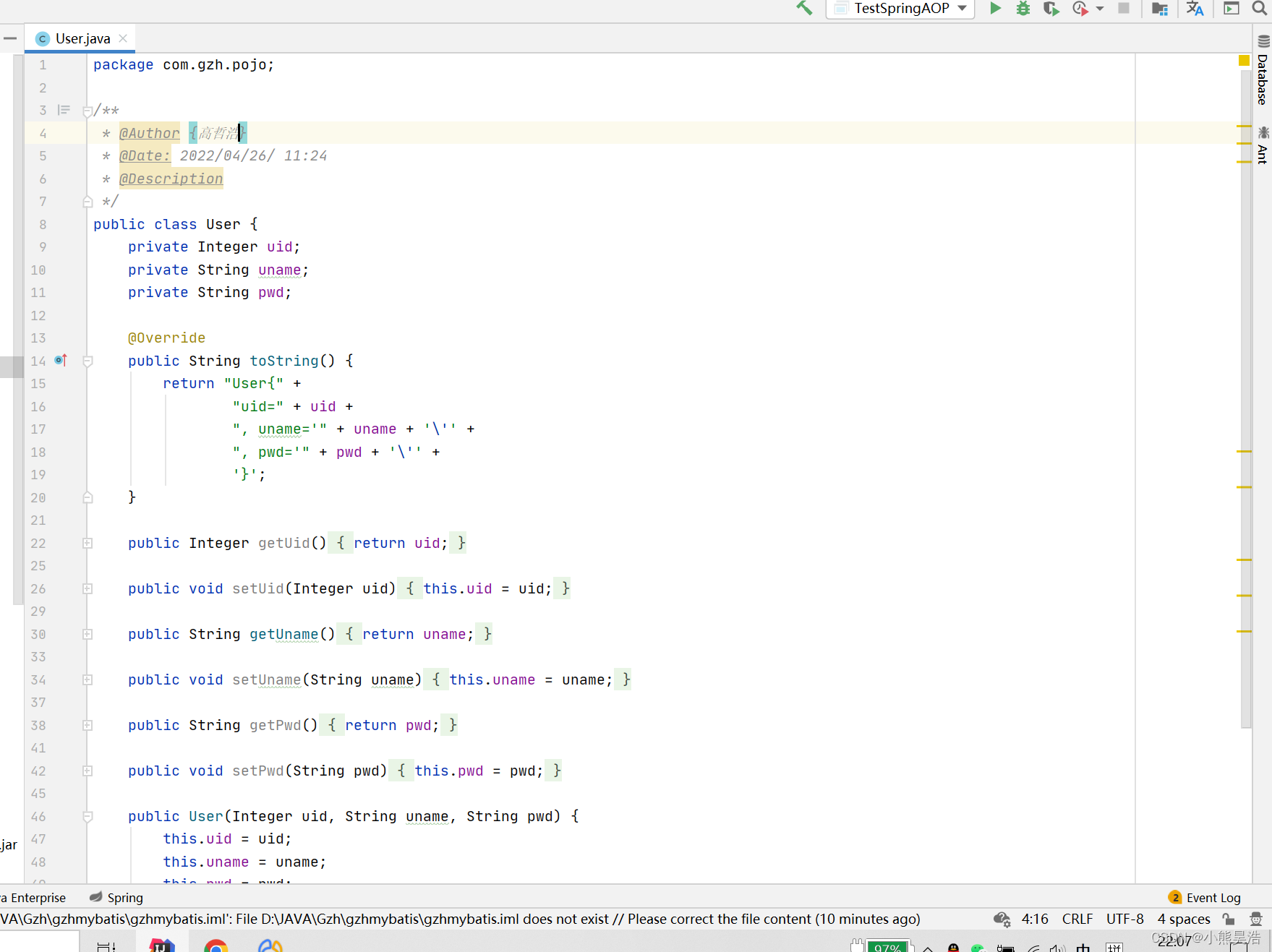
五、使用步骤编写UserMapper接口
package com.gzh.mapper;
import com.gzh.pojo.User;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface UserMapper {
@Select("select * from t_user where uname=#{uname} and pwd = #{pwd}")
User userLoginMapper(@Param("uname") String uname, @Param("pwd") String pwd);
@Select("select * from t_user")
List<User> selectAll();
}
六、写service接口和相应的实现类
1.service接口
package com.gzh.service;
import com.gzh.pojo.User;
import java.util.List;
public interface UserLoginService {
List<User> findAll();
}
2.实现类
package com.gzh.service.impl;
import com.gzh.mapper.UserMapper;
import com.gzh.pojo.User;
import com.gzh.service.UserLoginService;
import java.util.List;
public class UserLoginServiceImpl implements UserLoginService {
private UserMapper userMapper;
public UserMapper getUserMapper() {
return userMapper;
}
public void setUserMapper(UserMapper userMapper) {
this.userMapper = userMapper;
}
@Override
public List<User> findAll() {
List<User> users = userMapper.selectAll();
return users;
}
}
七.编写Spring配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://127.0.0.1:3306/502?useSSL=false&useUnicode=true&characterEncoding=utf8&serverTimezone=Asia/Shanghai"></property>
<property name="username" value="root"></property>
<property name="password" value="root"></property>
</bean>
<bean id="factory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
</bean>
<bean id="mapper" class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="sqlSessionFactory" ref="factory"></property>
<property name="basePackage" value="com.gzh.mapper"></property>
</bean>
<bean id="us" class="com.gzh.service.impl.UserLoginServiceImpl">
<property name="userMapper" ref="userMapper"></property>
</bean>
</beans>
八、编写测试方法
代码如下(示例):
package com.gzh.test;
import com.gzh.mapper.UserMapper;
import com.gzh.pojo.User;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
public class TestA {
public static void main(String[] args) {
ApplicationContext ac = new ClassPathXmlApplicationContext("spring.xml");
UserMapper mapper = (UserMapper) ac.getBean("userMapper");
List<User> users = mapper.selectAll();
for (User u : users){
System.out.println("user="+u);
}
}
}
总结: 不太会说,就这样吧
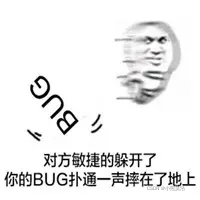
|