springboot:整合fastdfs
一、部署fastdfs
docker部署fastdfs
二、集成
依赖
<dependency>
<groupId>com.github.tobato</groupId>
<artifactId>fastdfs-client</artifactId>
<version>1.27.2</version>
</dependency>
配置类
@Configuration
@Import(FdfsClientConfig.class)
@EnableMBeanExport(registration = RegistrationPolicy.IGNORE_EXISTING)
public class FastDFSClientConfig {
}
yml配置
fdfs:
reqHost: 127.0.0.1
reqPort: 8899
so-timeout: 3000
connect-timeout: 1000
thumb-image:
width: 60
height: 60
tracker-list:
- 127.0.0.1:22122
工具类
package com.yolo.yoloblog.util;
import cn.hutool.core.util.StrUtil;
import com.github.tobato.fastdfs.domain.fdfs.MetaData;
import com.github.tobato.fastdfs.domain.fdfs.StorePath;
import com.github.tobato.fastdfs.domain.fdfs.ThumbImageConfig;
import com.github.tobato.fastdfs.domain.proto.storage.DownloadByteArray;
import com.github.tobato.fastdfs.exception.FdfsUnsupportStorePathException;
import com.github.tobato.fastdfs.service.FastFileStorageClient;
import lombok.Data;
import org.apache.commons.io.FilenameUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.Set;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
@Component
@Data
public class FastDFSUtil {
@Autowired
private FastFileStorageClient storageClient;
@Autowired
private ThumbImageConfig thumbImageConfig;
@Value("${fdfs.reqHost}")
private String reqHost;
@Value("${fdfs.reqPort}")
private String reqPort;
public String uploadFile(MultipartFile file) throws IOException {
StorePath path = storageClient.uploadFile(file.getInputStream(), file.getSize(),
FilenameUtils.getExtension(file.getOriginalFilename()), null);
return path.getFullPath();
}
public String uploadFile(File file) throws IOException {
FileInputStream inputStream = new FileInputStream(file);
StorePath path = storageClient.uploadFile(inputStream, file.length(),
FilenameUtils.getExtension(file.getName()), null);
return path.getFullPath();
}
public String uploadFileStream(InputStream is, long fileSize, String fileExtName) {
StorePath path = storageClient.uploadFile(is, fileSize, fileExtName, null);
return path.getFullPath();
}
public String uploadFile(byte[] bytes, long fileSize, String fileExtName) {
ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(bytes);
StorePath storePath = storageClient.uploadFile(byteArrayInputStream, fileSize, fileExtName, null);
return storePath.getFullPath();
}
public String uploadFile(String content, String fileExtName) {
byte[] buff = content.getBytes(StandardCharsets.UTF_8);
ByteArrayInputStream stream = new ByteArrayInputStream(buff);
StorePath storePath = storageClient.uploadFile(stream, buff.length, fileExtName, null);
return storePath.getFullPath();
}
public String[] upFileImage(InputStream is, long size, String fileExtName, Set<MetaData> metaData) {
StorePath storePath = storageClient.uploadImageAndCrtThumbImage(is, size, fileExtName, metaData);
String imagePath = storePath.getFullPath();
String thumbImagePath = thumbImageConfig.getThumbImagePath(imagePath);
return new String[]{imagePath,thumbImagePath};
}
public void deleteFile(String fileUrl) {
if (StrUtil.isEmpty(fileUrl)) {
return;
}
try{
StorePath storePath = StorePath.parseFromUrl(fileUrl);
storageClient.deleteFile(storePath.getGroup(), storePath.getPath());
} catch (FdfsUnsupportStorePathException e) {
e.printStackTrace();
}
}
public byte[] downloadFile(String fileUrl) {
if (StrUtil.isEmpty(fileUrl)) {
return null;
}
StorePath storePath = StorePath.parseFromUrl(fileUrl);
return storageClient.downloadFile(storePath.getGroup(), storePath.getPath(), new DownloadByteArray());
}
public InputStream download(String fileUrl ) {
if (StrUtil.isEmpty(fileUrl)) {
return null;
}
StorePath storePath = StorePath.parseFromUrl(fileUrl);
return storageClient.downloadFile(storePath.getGroup(), storePath.getPath(), (InputStream ins)->{
return ins;
});
}
public InputStream downloadFile(List<String> fileUrlList){
byte[] buffer = new byte[1024];
ByteArrayOutputStream baos = new ByteArrayOutputStream();
BufferedOutputStream fos = new BufferedOutputStream(baos);
ZipOutputStream zos = new ZipOutputStream(fos);
try{
for (String fileUrl:fileUrlList){
if (StrUtil.isNotEmpty(fileUrl)) {
StorePath storePath = StorePath.parseFromUrl(fileUrl);
byte[] bytes = storageClient.downloadFile(storePath.getGroup(), storePath.getPath(), new DownloadByteArray()) ;
String fileName = StrUtil.subSuf(fileUrl,fileUrl.lastIndexOf("/")+1);
zos.putNextEntry(new ZipEntry(fileName));
zos.write(bytes);
zos.closeEntry();
}
}
zos.close();
fos.flush();
InputStream is = new ByteArrayInputStream(baos.toByteArray());
return is;
}catch (Exception e){
e.printStackTrace();
}
return null;
}
public String getViewAccessUrl(String fileUrl) {
return "http://" + reqHost + ":" + reqPort + "/" + fileUrl;
}
}
控制器
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file) throws IOException {
String path = fastDFSUtil.uploadFile(file);
return fastDFSUtil.getViewAccessUrl(path);
}
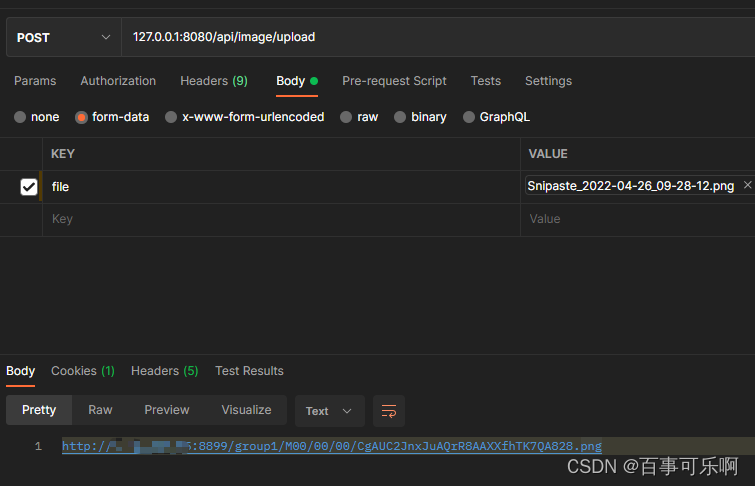
|