https://blog.csdn.net/weixin_45481406/article/details/110292130
什么是Feign
??Feign 是Spring Cloud Netflix 组件中的一个轻量级RESTFul的HTTP服务客户端,实现了负载均衡和Rest调用的开源框架,封装了Ribbon和RestTemplate;实现了WebService的面向接口编程,进一步降低了项目的耦合度。Feign内置了Ribbon,用来做客户端负载均衡调用服务注册中心的服务。Feign本身并不支持Spring MVC注解,它有一套自己的注解,为了使用更方便,Spring Cloud 孵化了OpenFeign。Feign是一种声明式、模板化的HTTP客户端,仅在consumer中使用。Feign的使用方式是:使用Feign的注解定义接口,调用这个接口,就可以调用服务注册中心的服务
Feign解决的问题
??Feign旨在使编写Java HTTP客户端变得更加容易,Feign 简化了RestTemplate代码,实现了Ribbon负载均衡,使代码变得更加简洁,也减少了客户端调用的代码,使用Feign实现负载均衡是首选方案。只要你创建一个接口,然后在上面添加注解即可 ??Feign是声明式调用服务组件,其核心是:像调用本地方法一样调用远程方法,无感知远程HTTP请求
- 它解决了让开发者调用远程接口就像调用本地方法一样的体验,开发者完全感知不到这是远程方法,更感知不到这是个HTTP请求,无需关注与远程交互的细节,更无需关注分布式环境开发
- 它像Dubbo一样,Consumer直接调用provider接口方法,而不需要通过常规的Http Client构造请求在解析返回数据
Feign VS OpenFeign
??OpenFeign是Spring Cloud 在 Feign 的基础上支持Spring MVC 注解,如:@RequestMapping等。OpenFeign的 @FeignClient 可以解析SpringMVC的 @RequestMapping 注解下的接口,并通过动态代理的方式产生实现类,实现类中做负载均衡并调用远程服务
Feign 入门案例
??本次案例使用Eureka注册中心,相关的注册中心和服务提供者可以参考 服务注册与发现-Eureka(三) 这篇文章,本文就不在重复搭建环境,主要演示服务消费者的代码demo
服务消费者
1. 导入jar包
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
2. 配置Eureka
server:
port: 8006
spring:
application:
name: eureka-feign-demo
eureka:
instance:
hostname: ${spring.cloud.client.ip-address}:${server.port}
prefer-ip-address: true
instance-id: ${spring.cloud.client.ip-address}:${server.port}
client:
register-with-eureka: true
fetch-registry: true
service-url:
defaultZone: http://root:123456@localhost:8002/eureka/,http://root:123456@localhost:8001/eureka/
registry-fetch-interval-seconds: 10
3. 声明需要调用的微服务接口
@FeignClient("eureka-provider-demo")
public interface ProviderServiceFeign {
@GetMapping("/list")
Object list();
}
4. 服务消费者接口调用远程服务
@Service
public class ConsumerDemoService implements IConsumerDemoService {
@Autowired
private ProviderServiceFeign providerServiceFeign;
@Override
public List<DemoEntity> list() {
return (List<DemoEntity>) providerServiceFeign.list();
}
}
5. 启动类中开启feign功能,启动测试
@SpringBootApplication
@EnableFeignClients
public class EurekaFeignDemoApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaFeignDemoApplication.class, args);
}
}
??访问消费者,可以正确获取到远程数据 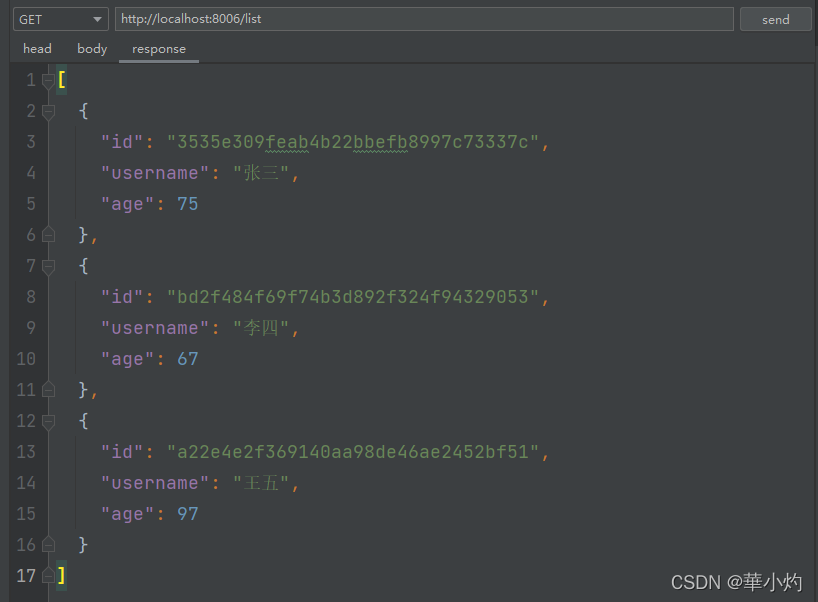
Feign 负载均衡
??Feign封装了Ribbon,自然也就进程了负载均衡的功能,默认采用轮询。由于 Netflix 对于 Ribbon 的维护已经暂停,所以 Spring Cloud 对于负载均衡建议使用由其自己定义的 Spring Cloud LoadBalancer。对于Spring Cloud LoadBalancer 的使用非常简单。 ??注意:从 Spring Cloud Netflix 3.0 以后的版本开始,就已经没有ribbon的依赖,它的负载均衡是依靠的Spring Cloud的负载均衡策略,并且只有两种:轮询策略 和 随机策略,实现的是 ReactorServiceInstanceLoadBalancer 接口,有兴趣的可以去看看源码 
public class LoadBalancerConfig {
@Bean
ReactorLoadBalancer<ServiceInstance> randomLoadBalancer(Environment environment,
LoadBalancerClientFactory loadBalancerClientFactory) {
String name = environment.getProperty(LoadBalancerClientFactory.PROPERTY_NAME);
return new RandomLoadBalancer(loadBalancerClientFactory
.getLazyProvider(name, ServiceInstanceListSupplier.class),
name);
}
}
??注意:这个类不能使用 @Configuration 注解,否则负载均衡器会失效并抛出空指针异常 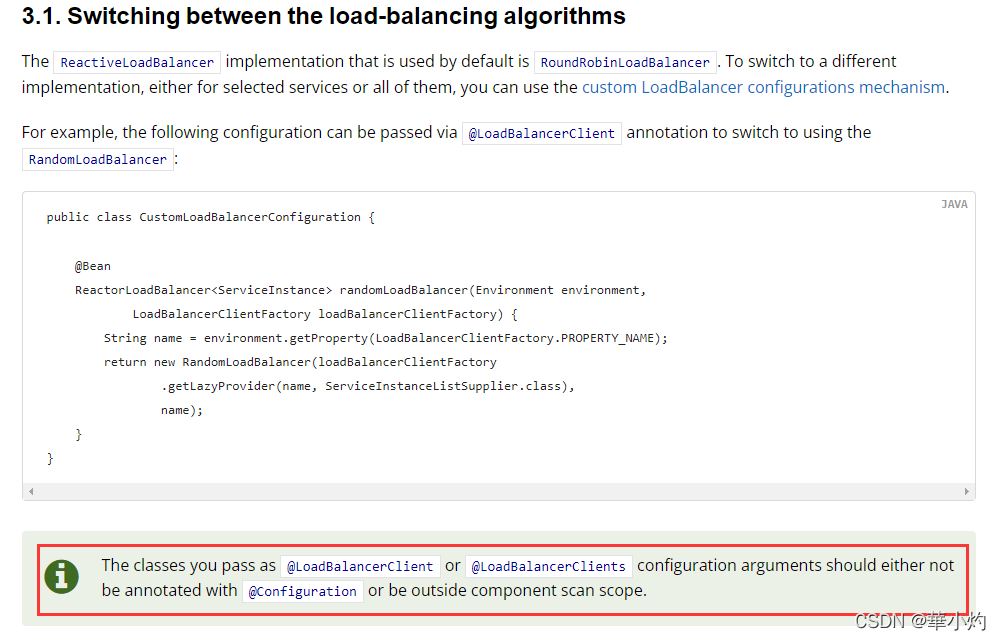
Feign 性能优化
Gzip压缩
??Gzip是一种数据格式,采用deflate算法压缩数据;Gzip是一种流行的文件压缩算法,应用十分广泛,尤其在Linux平台。当Gzip压缩一个纯文本文件时,效果非常明显,大约可以减少70%以上的文件大小。 ??Gzip的作用:网络数据经过压缩后实际降低了网络传输的字节数,做明显的好处就是可以加快网页加载的速度。网页加载速度的加快的好处不言而喻。除了节省流量,改善用户体验外,另一个潜在的好处就是Gzip与搜索引擎的抓取工具有着更好的关系。 HTTP协议源于压缩传输的规定
- 客户端向服务端请求中带有 Accept-Encoding:gzip、deflate 字段,向服务器表示客户端支持的压缩格式,如果不发送该消息头,服务端默认是不会压缩的
- 服务端在接收到请求后,如果发现请求头中含有 Accept-Encoding 字段,并且支持该类型的压缩,就会对响应报文压缩之后返回给客户端,并携带 Content-Encoding:gzip 消息头,表示响应的报文是根据该格式进行压缩的
- 客户端收到请求之后,先判断是否有 Content-Encoding 消息头,如果有,按照格式对报文进行解压,否则按正常报文处理
Gzip 压缩示例 ??局部:只配置consumer通过feign到provider的请求与响应的gzip压缩,即只在consumer和provider之间开启gzip压缩传输
feign:
compression:
request:
mime-types: text/xml,application/xml,application/json
min-request-size: 512
enabled: true
response:
enabled: true
??全局:对客户端浏览器的请求以及consumer对provider的请求与响应都实现gzip压缩,即浏览器-consumer-provider三者之间的传输都开启gzip压缩传输
server:
compression:
enabled: true
mime-types: text/html,text/plain,text/xml,application/xml,application/json
??开启测试,局部压缩没法演示,就只能看看全局配置后的压缩 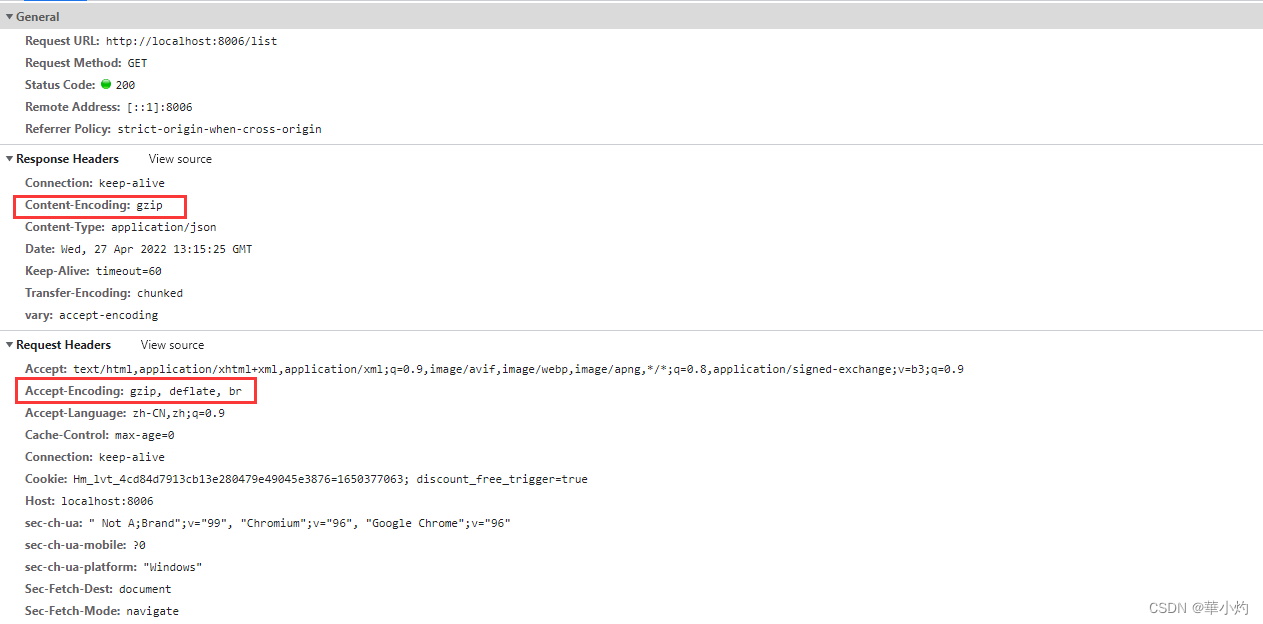
HTTP 连接池
??两台服务器建立http连接的过程是很复杂的,涉及到多个数据包的交换,很耗时间。HTTP的连接需要的3次握手4次挥手开销很大,这一开销对于大量的比较小的http消息来说更大。采用HTTP连接池可以节约大量的3次握手4次挥手,这样可以大大的提升吞吐量 ??Feign的HTTP客户端支持三种框架:HttpURLConnection、HttpClient、OkHttp
- 传统的HttpURLConnection是JDK自带的,并不支持连接池,如果要实现连接池的机制,还需要自己来管理连接对象。
??HttpClient封装了HTTP请求头、参数、内容体、响应等等,它不仅使客户端发送HTTP请求变得容易,而且也方便了 开发人员测试接口,既提高了开发的效率,又提高了代码的健壮性;另外高并发大量的请求网络的时候,也可以使用连接池提升吞吐量 1. 导入依赖 ??在consumer导入依赖
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>feign-httpclient</artifactId>
<version>11.8</version>
</dependency>
2. 配置 ??在consumer配置文件中开启httpClient
feign:
httpclient:
enabled: true
源码地址:https://gitee.com/peachtec/hxz-study
|