实现记事本————使用GUI
使用GUI组件实现记事本,要求有弹出窗口,以及模态和非模态对话框
代码实现
package GuIExample;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Win {
public static void main(String[] args) {
JFrame jFrame = new JFrame("记事本");
jFrame.setSize(800, 600);
jFrame.setLocationRelativeTo(null);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JTextArea jTextArea = new JTextArea();
jTextArea.setLineWrap(true);
JScrollPane scrollPane = new JScrollPane(jTextArea);
jFrame.add(scrollPane);
JMenuBar bar = new JMenuBar();
JMenu menuFile = new JMenu("文件(F)");
JMenuItem item1 = new JMenuItem("新建(N)");
item1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
newFile();
}
});
JMenuItem item2 = new JMenuItem("打开(O)");
item2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == item2) {
openFile();
}
}
});
JMenuItem item3 = new JMenuItem("保存(S)");
item3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == item3) {
saveFile();
}
}
});
JMenuItem item4 = new JMenuItem("另存为");
item4.addActionListener(new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
saveFile();
}
});
JMenuItem item5 = new JMenuItem("页面设置(U)");
JMenuItem item6 = new JMenuItem("打印(P)");
JMenuItem item7 = new JMenuItem("退出(X)");
menuFile.add(item1);
menuFile.add(item2);
menuFile.add(item3);
menuFile.add(item4);
menuFile.addSeparator();
menuFile.add(item5);
menuFile.add(item6);
menuFile.addSeparator();
menuFile.add(item7);
JMenu editMenu = new JMenu("编辑");
JMenu formatMenu = new JMenu("格式");
JMenu viewMenu = new JMenu("查看");
JMenu helpMenu = new JMenu("帮助");
bar.add(menuFile);
bar.add(editMenu);
bar.add(formatMenu);
bar.add(viewMenu);
bar.add(helpMenu);
jFrame.setJMenuBar(bar);
jFrame.setVisible(true);
}
private static void newFile() {
JDialog jDialog = new JDialog();
jDialog.setSize(260, 150);
jDialog.setLocationRelativeTo(null);
jDialog.setModal(true);
jDialog.setLayout(new GridLayout(2, 1));
JPanel panel1 = new JPanel();
JPanel panel2 = new JPanel();
JLabel jLabel = new JLabel("是否将更改保存到 无标题?");
JButton button1 = new JButton("保存(S)");
JButton button2 = new JButton("不保存(N)");
JButton button3 = new JButton("取消");
panel1.add(jLabel);
panel2.add(button1);
panel2.add(button2);
panel2.add(button3);
jDialog.add(panel1);
jDialog.add(panel2);
jDialog.setVisible(true);
}
private static void openFile() {
JFrame jFrame = new JFrame();
FileDialog jDialog = new FileDialog(jFrame);
jDialog.setSize(260, 150);
jDialog.setLocationRelativeTo(null);
jDialog.setModal(true);
jDialog.setVisible(true);
}
private static void saveFile() {
JFrame jFrame = new JFrame();
FileDialog jDialog = new FileDialog(jFrame);
jDialog.setSize(260, 150);
jDialog.setLocationRelativeTo(null);
jDialog.setModal(true);
JLabel jLabel = new JLabel("这是一个模态对话框");
jDialog.add(jLabel);
jDialog.setVisible(true);
}
}
运行结果
主页面
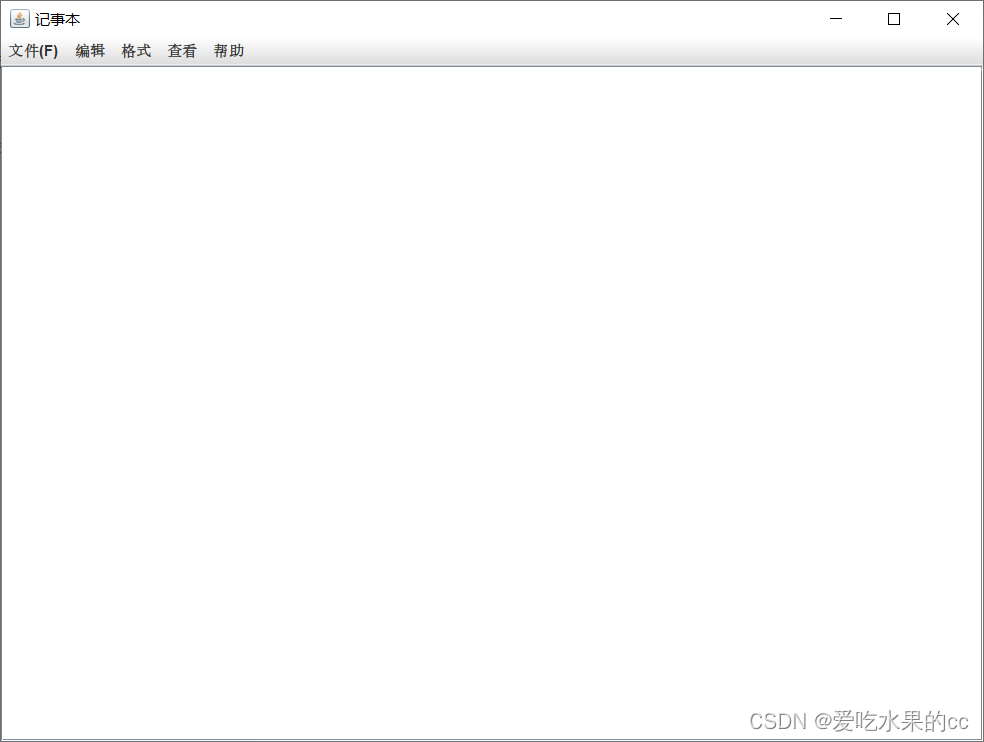
菜单栏
菜单相关组件实现
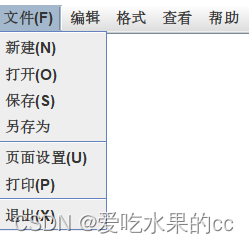
打开文件
仅仅就是一个窗口,未实现真正意义上的打开文件 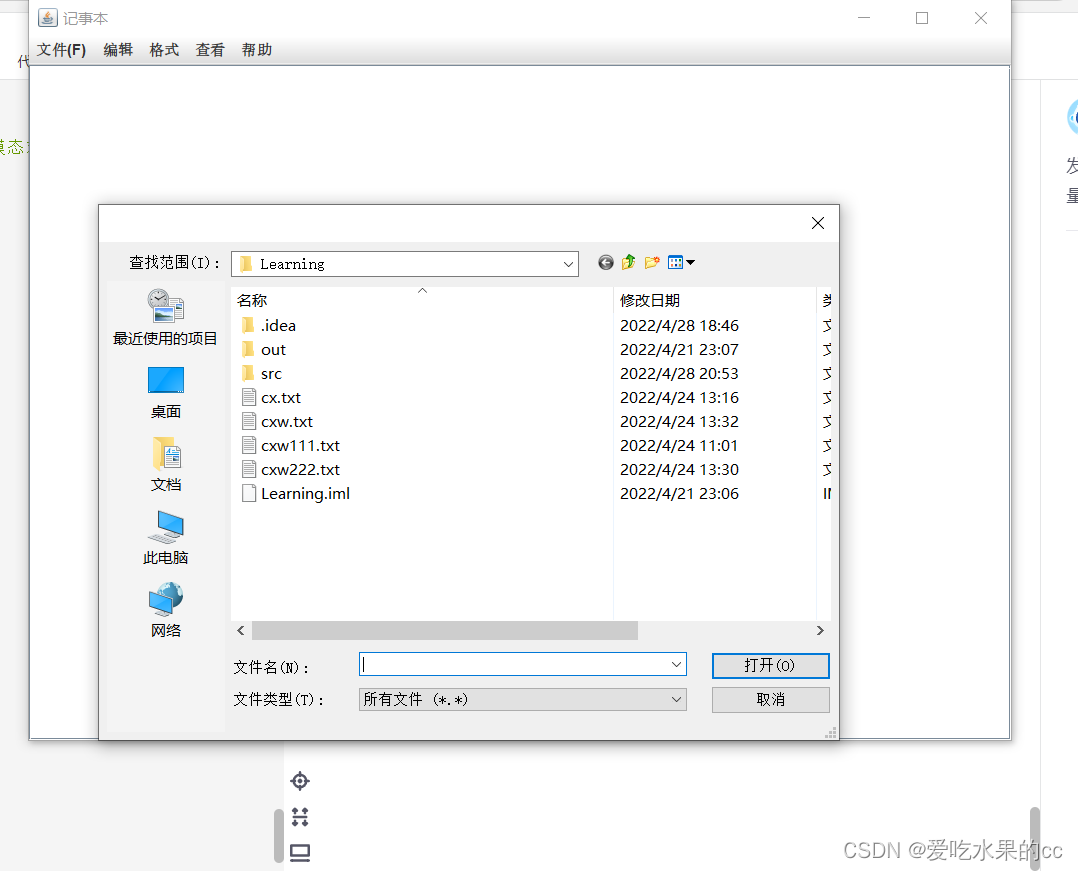
新建文件
未实现保存,不保存,取消的监听器功能,只是简单的UI界面 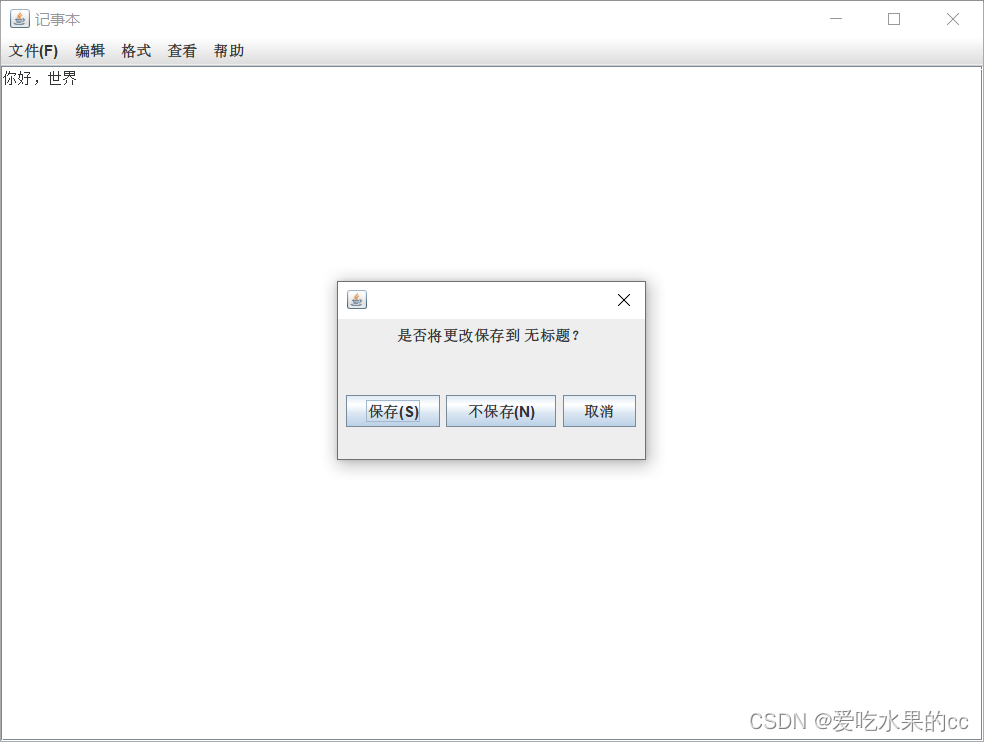
总结
这的意义在于实现GUI组件的运用和窗口的实现,JDialog窗口模态和非模态的区分。可以使用构造方法创建JDialog对象时设置为模态或非模态。也可以在创建对象后使用setModal()方法设置。传入参数为true时为模态窗口,false时为非模态窗口
模态窗口
用户需要处理完当前会话框时才可以使用其他窗口
private static void newFile() {
JDialog jDialog = new JDialog();
jDialog.setSize(260, 150);
jDialog.setLocationRelativeTo(null);
jDialog.setModal(true);
jDialog.setLayout(new GridLayout(2, 1));
JPanel panel1 = new JPanel();
JPanel panel2 = new JPanel();
JLabel jLabel = new JLabel("是否将更改保存到 无标题?");
JButton button1 = new JButton("保存(S)");
JButton button2 = new JButton("不保存(N)");
JButton button3 = new JButton("取消");
panel1.add(jLabel);
panel2.add(button1);
panel2.add(button2);
panel2.add(button3);
jDialog.add(panel1);
jDialog.add(panel2);
jDialog.setVisible(true);
}
非模态窗口
允许处理当前对话框时同时使用其他对话框,比如JFrame窗口
package GuIExample;
import javax.swing.*;
public class JFrameExample {
public static void main(String[] args) {
JFrame win = new JFrame("小明的窗口");
JButton bt = new JButton("我是按钮");
win.setLocation(400, 300);
win.setSize(300, 250);
win.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
win.setVisible(true);
}
}
欢迎大家指正,over 作者:爱吃水果的cc
|