学习目标:
准备工作:
- 注册阿里云账户
- 开通短信服务
- 申请签名和模板
- 拿到AccessKey
大概说一下测试和申请流程,输入https://www.aliyun.com/进入官网,然后注册账号,直接在搜索框输入短信服务就能找到,进入控制台,找到国内消息,申请签名和模板,   AccessKey:在右上角头像哪里 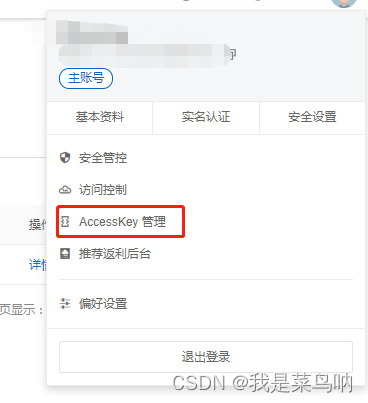
申请大概需要几个小时,个人用户一般很难通过,我个人申请被驳回很多次,最后用域名备案才通过
开始编写代码:
第一步先导入依赖
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.3.3</version>
</dependency>
第二步:在项目yml文件中配置阿里云短信的秘钥,秘钥在上面AccessKey管理可以拿到
aliyun:
sms:
regionId: default
accessKeyId: xxxxxxxxxxxxxxxxxxxxxxx
secret: xxxxxxxxxxxxxxxxxxxxxxx
第三步:去读取配置文件的参数秘钥,InitializingBean接口为bean提供了属性初始化后的处理方法
@Component
public class ConstantPropertiesUtils implements InitializingBean {
@Value("${aliyun.sms.regionId}")
private String regionId;
@Value("${aliyun.sms.accessKeyId}")
private String accessKeyId;
@Value("${aliyun.sms.secret}")
private String secret;
public static String REGION_Id;
public static String ACCESS_KEY_ID;
public static String SECRECT;
@Override
public void afterPropertiesSet() throws Exception {
REGION_Id=regionId;
ACCESS_KEY_ID=accessKeyId;
SECRECT=secret;
}
}
什么是验证码呢,就是后台随机生成的一段数字,为了方便就写了一个工具类获取验证码
package com.example.shiro.sys.utlis.smsSend;
import java.text.DecimalFormat;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Random;
public class RandomUtil {
private static final Random random = new Random();
private static final DecimalFormat fourdf = new DecimalFormat("0000");
private static final DecimalFormat sixdf = new DecimalFormat("000000");
public static String getFourBitRandom() {
return fourdf.format(random.nextInt(10000));
}
public static String getSixBitRandom() {
return sixdf.format(random.nextInt(1000000));
}
public static ArrayList getRandom(List list, int n) {
Random random = new Random();
HashMap<Object, Object> hashMap = new HashMap<Object, Object>();
for (int i = 0; i < list.size(); i++) {
int number = random.nextInt(100) + 1;
hashMap.put(number, i);
}
Object[] robjs = hashMap.values().toArray();
ArrayList r = new ArrayList();
for (int i = 0; i < n; i++) {
r.add(list.get((int) robjs[i]));
System.out.print(list.get((int) robjs[i]) + "\t");
}
System.out.print("\n");
return r;
}
}
准备工作都写完了,在编写controller来获取验证码,我这里还整合redis缓存,方便后面做验证码的有效期和校验,不整合redis也不影响发送验证码
@GetMapping("/getCode/{phone}")
public R getCode(@PathVariable String phone){
String code =(String) redisUtil.get(phone);
if(!StringUtils.isEmpty(code)){
return R.ok();
}
code = RandomUtil.getFourBitRandom();
boolean isSend = msgSendService.send(phone,code);
if(isSend){
redisUtil.set(phone,code, 120);
return R.ok();
}else{
return R.error("发送短信失败!!!");
}
}
直接看业务层具体的实现
@Override
public boolean send(String phone, String code) {
if(StringUtils.isEmpty(phone)){
return false;
}
DefaultProfile profile = DefaultProfile.
getProfile(ConstantPropertiesUtils.REGION_Id,
ConstantPropertiesUtils.ACCESS_KEY_ID,
ConstantPropertiesUtils.SECRECT);
IAcsClient client = new DefaultAcsClient(profile);
CommonRequest request = new CommonRequest();
request.setMethod(MethodType.POST);
request.setDomain("dysmsapi.aliyuncs.com");
request.setVersion("2017-05-25");
request.setAction("SendSms");
request.putQueryParameter("PhoneNumbers", phone);
request.putQueryParameter("SignName", "这里写你开通短信的签名");
request.putQueryParameter("TemplateCode", "模板code");
Map<String,Object> param = new HashMap();
param.put("code",code);
request.putQueryParameter("TemplateParam", JSONObject.toJSONString(param));
try {
CommonResponse response = client.getCommonResponse(request);
System.out.println(response.getData());
return response.getHttpResponse().isSuccess();
} catch (ServerException e) {
e.printStackTrace();
} catch (ClientException e) {
e.printStackTrace();
}
return false;
}
最后看能不能收到短信验证码,我配置了swagger-ui进行测试  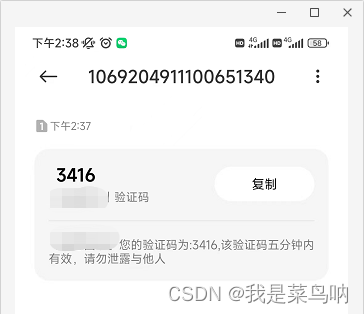
成功接收到,实现也很简单,代码复制就能使用
|