先写一个Controller输出信息
package com.example.thy;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.ArrayList;
import java.util.List;
@Controller
public class UserController {
@RequestMapping("/hello")
public String user(Model model){
User user = new User();
user.setId(11);
user.setUsername("张三");
user.setAddress("山东");
users.add(user);
model.addAttribute("user",user);
return "hello";
}
}
1.${}:变量表达式,用于访问的是容器上下文的变量,比如域变量:request域,session域,?如果没对象,和${} 基本上没区别
<div><td th:text="${user.id}"></td></div>
<div><td th:text="${user.username}"></td></div>
<div><td th:text="${user.address}"></td></div>
2.*{}:选择表达式,是选定的对象,不是整个环境的映射
<div><td th:text="*{user.id}"></td></div>
<div><td th:text="*{user.username}"></td></div>
<div><td th:text="*{user.address}"></td></div>
或者
<div th:object="${user}">
<div><td th:text="*{id}"></td></div>
<div><td th:text="*{username}"></td></div>
<div><td th:text="*{address}"></td></div>
</div>
上面的输出结果都是一样的

3.#{}:thymeleaf中的消息表达式、或者是资源表达式
message.properties
message=你好
hello.html
<div th:text="#{message}"></div>
4.@引用URL地址
<a th:href="@{/user/login}"></a>
5.项目的国际化
设置配置文件的编码格式
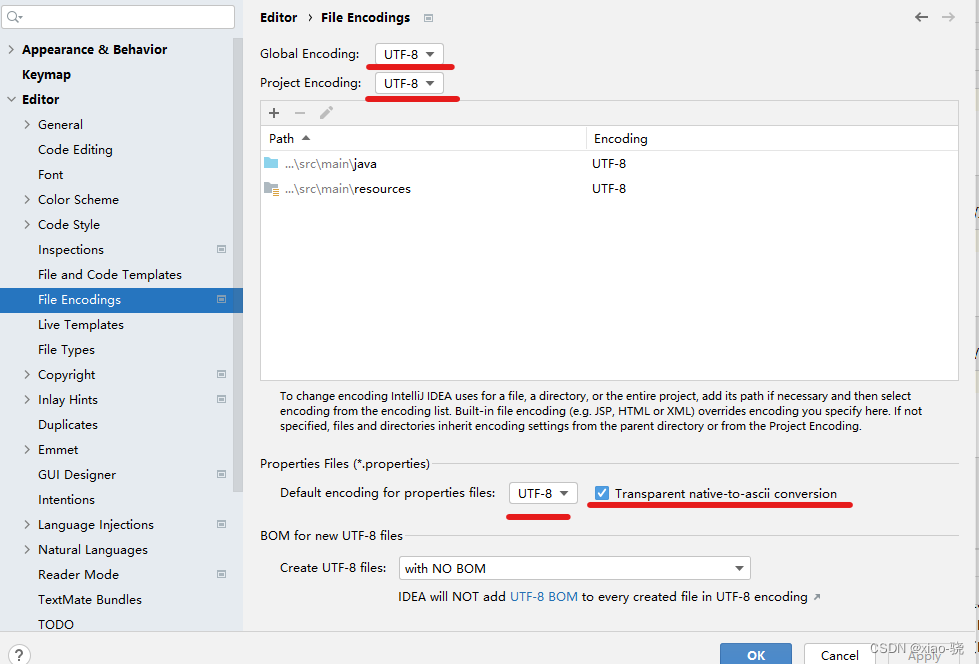
先resources创建i18n三个配置文件
login.properties login_en_US.properties login_zh_CN.properties
直接进入login.properties
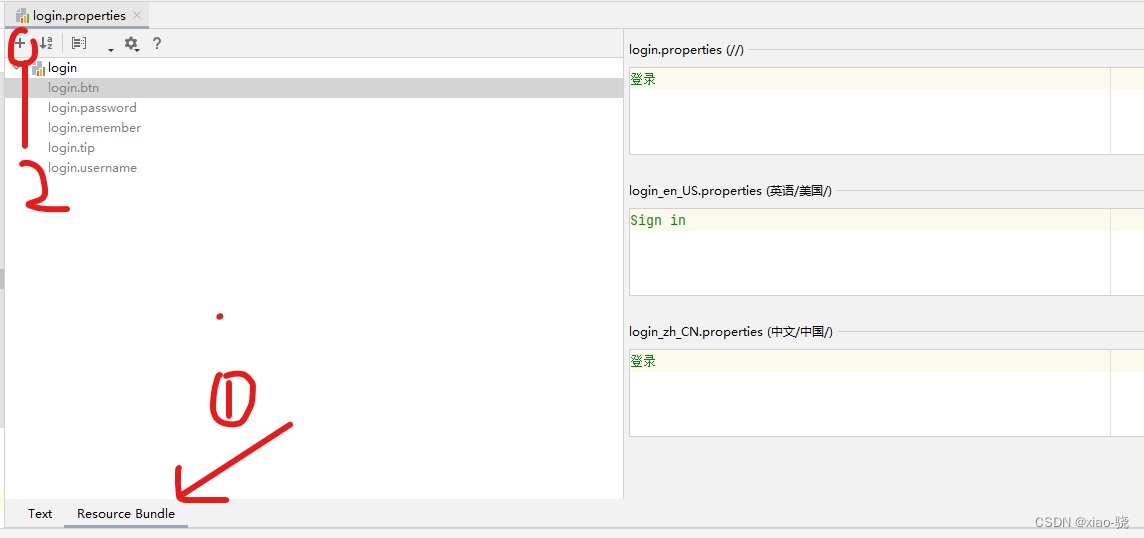
? ,如果没有这个,可能是因为,idea的版本是新的,需要安装插件,如下:
?
插件安装之后, 点击加号加上自己的内容
login.properties
login.btn=登录
login.password=密码
login.remember=记住我
login.tip=请登录
login.username=用户名
login_en_US.properties
login.btn=Sign in
login.password=Password
login.remember=Remember me
login.tip=Please sign in
login.username=Username
login_zh_CN.properties
login.btn=登录
login.password=密码
login.remember=记住我
login.tip=请登录
login.username=用户名
?在application.properties加载i18n?
spring.messages.basename=i18n.login
?或者application.yml加载i18n?
spring:
messages:
basename: i18n.login
?
?之后创建login.html,不要忘记加入xmlns:th="www.thymeleaf.org"
<!DOCTYPE html>
<html lang="en" xmlns:th="www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<input type="text" name="username" th:placeholder="#{login.username}" required="" autofocus="">
<input type="password" name="password" th:placeholder="#{login.password}" required="">
<!-- 这里传入参数不需要使用 ?使用 (key=value)-->
<a class="btn btn-sm" th:href="@{/login(l='zh_CN')}">中文</a>
<a class="btn btn-sm" th:href="@{/login(l='en_US')}">English</a>
<button type="submit" th:text="#{login.btn}"></button>
</body>
</html>
然后创建MyLocaleResolver
package com.example.thy;
import org.springframework.util.StringUtils;
import org.springframework.web.servlet.LocaleResolver;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Locale;
public class MyLocaleResolver implements LocaleResolver {
//解析请求
@Override
public Locale resolveLocale(HttpServletRequest request) {
String language = request.getParameter("l");
Locale locale = Locale.getDefault(); // 如果没有获取到就使用系统默认的
//如果请求链接不为空
if (!StringUtils.isEmpty(language)){
//分割请求参数
String[] split = language.split("_");
//国家,地区
locale = new Locale(split[0],split[1]);
}
return locale;
}
@Override
public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) {
}
}
?把国际化解析器添加到容器中
package com.example.thy;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.LocaleResolver;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Bean
public LocaleResolver localeResolver(){
return new MyLocaleResolver();
}
}
|