application.properties文件中内容
spring.datasource.driverClassName=com.mysql.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/ssm spring.datasource.username=root spring.datasource.password=123456789 spring.datasource.type=com.alibaba.druid.pool.DruidDataSource mybatis.type-aliases-package=com.myspringboot.pojo
SpringBoot启动类
import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication @MapperScan("com.myspringboot.mapper") ?//@MapperScan 用户扫描MyBatis的Mapper接口 public class App { ?? ? ?? ?public static void main(String[] args) { ?? ??? ?SpringApplication.run(App.class, args); ?? ?} ?? ? ?? ?/** ?? ? * 启动应用app ?? ? * 1.controller定义page并调用service的方法 ?? ? * 2.service实例化mapper对象,并调用mapper的方法 ?? ? * 3.mapper调用mapper.xml中的sql语句 ?? ? */
}
Controller测试类如下:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.core.env.Environment; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
/** ?* @author Jeff.xie ?* ?* @RestController注解,相当于@Controller+@ResponseBody两个注解的结合,返回json数据不需要在方法前面加@ResponseBody注解了, ?* 但使用@RestController这个注解,就不能返回jsp,html页面,视图解析器无法解析jsp,html页面 ?* ?* 理解下面的注解: ?* @ResponseBody 表示该方法的返回结果直接写入 HTTP response body 中,一般在异步获取数据时使用【也就是AJAX】, ?* 在使用 @RequestMapping后,返回值通常解析为跳转路径,但是加上 @ResponseBody 后返回结果不会被解析为跳转路径,而是直接写入 HTTP response body 中。 ?* 比如异步获取 json 数据,加上 @ResponseBody 后,会直接返回 json 数据。 ?* @RequestBody 将 HTTP 请求正文插入方法中,使用适合的 HttpMessageConverter 将请求体写入某个对象。 ?* ?*/ @Controller @RequestMapping("/test") public class GetApplicationController { ?? ? ?? ?@Value("${spring.datasource.password}") ?? ?private String password; ?? ? ?? ?/** ?? ? * 第一种:通过注解Value获取 ?? ? * @return ?? ? */ ?? ?@RequestMapping("/value") ?? ?public String getByValue(Model model) { ?//获取属性成功 ?? ??? ?System.out.println(password);//获取属性成功 ?? ??? ?model.addAttribute("value",password); ?? ??? ?return "readApplication"; ?? ?} ?? ? ?? ?@Autowired ?? ?Environment env; ?? ? ?? ?/** ?? ? * 通过Enviroment类来获取 ?? ? * @return ?? ? */ ?? ?@RequestMapping("/env") ?? ?public String getByEnviroment(Model model) { ?? ??? ?String value = env.getProperty("spring.datasource.url"); ?? ??? ?model.addAttribute("value",value); ?? ??? ?System.out.println(value);//获取属性成功 ?? ??? ?return "readApplication"; ?? ?}
}
?
pom.xml文件内容
<project xmlns="http://maven.apache.org/POM/4.0.0" ?? ?xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" ?? ?xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> ?? ?<modelVersion>4.0.0</modelVersion> ?? ?<parent> ?? ??? ?<groupId>org.springframework.boot</groupId> ?? ??? ?<artifactId>spring-boot-starter-parent</artifactId> ?? ??? ?<version>1.5.10.RELEASE</version> ?? ?</parent> ?? ?<groupId>com.myspringboot</groupId> ?? ?<artifactId>MySpringBoot02</artifactId> ?? ?<version>0.0.1-SNAPSHOT</version> ?? ?<properties> ?? ??? ?<java.version>1.8</java.version> ?? ?</properties>
?? ?<dependencies> ?? ??? ?<!-- springBoot 的启动器 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>org.springframework.boot</groupId> ?? ??? ??? ?<artifactId>spring-boot-starter-web</artifactId> ?? ??? ?</dependency>? ?? ??? ?<!-- web 启动器 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>org.springframework.boot</groupId> ?? ??? ??? ?<artifactId>spring-boot-starter-thymeleaf</artifactId> ?? ??? ?</dependency>? ?? ??? ?<!-- Mybatis 启动器 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>org.mybatis.spring.boot</groupId> ?? ??? ??? ?<artifactId>mybatis-spring-boot-starter</artifactId> ?? ??? ??? ?<version>1.1.1</version> ?? ??? ?</dependency>? ?? ??? ??? ??? ?<!-- mysql 数据库驱动 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>mysql</groupId> ?? ??? ??? ?<artifactId>mysql-connector-java</artifactId> ?? ??? ?</dependency>? ?? ??? ??? ??? ?<!-- druid 数据库连接池 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>com.alibaba</groupId> ?? ??? ??? ?<artifactId>druid</artifactId> ?? ??? ??? ?<version>1.0.9</version> ?? ??? ?</dependency> ?? ??? ??? ??? ?<!--添加junit环境的jar包 --> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>org.springframework.boot</groupId> ?? ??? ??? ?<artifactId>spring-boot-starter-test</artifactId> ?? ??? ?</dependency> ?? ??? ?<dependency> ?? ??? ??? ?<groupId>org.springframework.boot</groupId> ?? ??? ??? ?<artifactId>spring-boot-configuration-processor</artifactId> ?? ??? ??? ?<optional>true</optional> ?? ??? ?</dependency> ?? ?</dependencies> </project>
readApplication.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"/> <title>read application</title> </head> <body> ?? ?read application <span th:text="${value}"></span> </body> </html>
测试
启动App.class
右键>Run as> Java Application
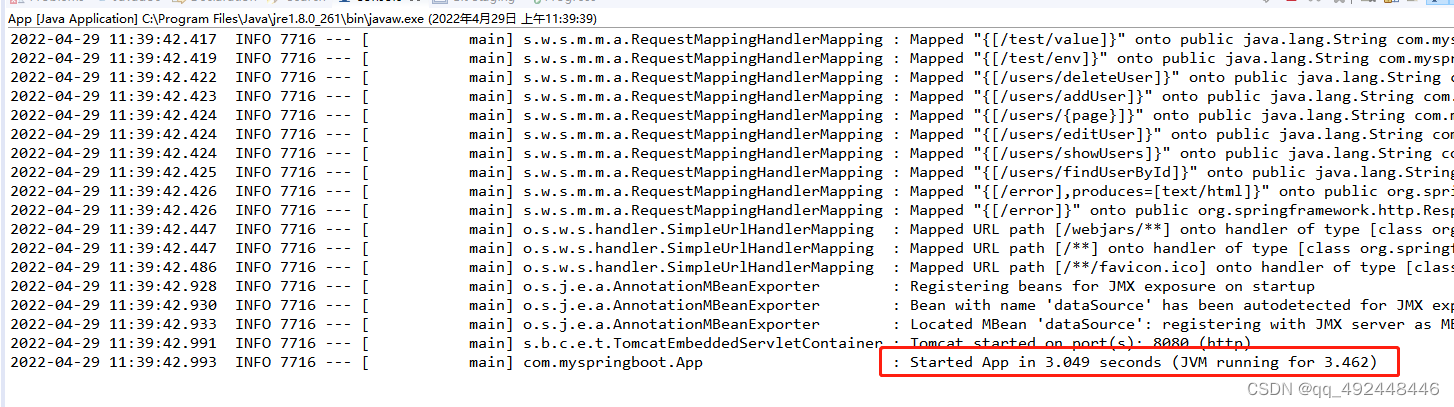
?
输入网址localhost:8080/test/value
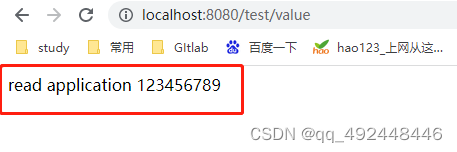
?输入网址localhost:8080/test/env
?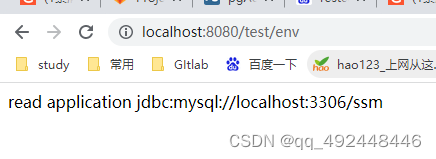
?
|